Event Loop Monitoring and Performance Analysis
Event Loop Monitoring and Performance Analysis Introduction In the world of JavaScript, understanding the event loop is crucial for performance optimization and debugging. The event loop allows asynchronous operations and concurrency in a single-threaded environment. Monitoring its performance can help developers detect bottlenecks, identify runaway processes, and improve the responsiveness of applications. This comprehensive article delves into event loop monitoring and performance analysis, exploring its history, technical intricacies, best practices, and real-world use cases. Moreover, it will provide deep insights into edge cases and advanced implementations for senior developers aiming to master the art of JavaScript performance tuning. Historical Context JavaScript was conceived in 1995 by Brendan Eich while working at Netscape. Over the years, it has transformed from a simple scripting language for web browsers into a powerful and multifaceted tool that powers entire applications. The introduction of Node.js in 2009 extended JavaScript beyond browsers, allowing developers to run JavaScript on servers and facilitating the non-blocking I/O models that the language broadly supports. In its early adoption, JavaScript's event-driven nature led to the formation of the event loop concept as an essential mechanism for handling asynchronous operations without blocking the single-threaded execution model. Understanding how the event loop operates has now become a crucial component within JavaScript’s ecosystem, particularly with the widespread usage of single-page applications (SPAs) and real-time web applications. Technical Overview of the Event Loop Before diving into monitoring and analysis, let's understand how the event loop works: Call Stack: It maintains the execution stack of function calls. When a function is invoked, it is pushed onto the stack and popped once it completes. Web APIs (in browsers): They help to manage asynchronous operations like timers (setTimeout, setInterval), AJAX calls, and events. When these operations complete, they send their respective callbacks to the callback queue. Callback Queue: It holds messages or callbacks waiting to be executed. When the call stack is empty, the event loop pushes the first callback from the queue onto the stack for execution. Microtask Queue: This queue is prioritized over the callback queue. Microtasks (promises and MutationObserver callbacks) are processed before the next event loop cycle begins. Event Loop: The event loop continuously checks if the call stack is empty and moves callbacks from the callback queue and microtask queue onto it for execution. Understanding Event Loop Monitoring Event loop monitoring involves tracking the time it takes for the event loop to process different tasks (microtasks and macrotasks), helping identify performance bottlenecks. Methodology for Monitoring Using performance.now(): This method provides accurate timestamps that can be leveraged to track task execution times. Event Loop Tracing: In Node.js, you can utilize built-in modules such as perf_hooks to create performance snapshots. JavaScript Performance Profiling: Browsers provide tools (like Chrome DevTools) that allow developers to profile JavaScript execution and visualize the event loop activity. Code Examples Example 1: Basic Event Loop Simulation console.log('Start'); setTimeout(() => { console.log('Timeout callback'); }, 0); Promise.resolve().then(() => { console.log('Promise callback'); }); console.log('End'); Expected Output: Start End Promise callback Timeout callback Explanation: In this example, the promise callback is executed before the timeout callback due to the microtask queue's higher priority. Example 2: Event Loop with Complex Nesting setTimeout(() => { console.log('Timeout 1'); Promise.resolve().then(() => { console.log('Promise 1'); }); setTimeout(() => { console.log('Timeout 2'); }, 0); }, 0); Promise.resolve().then(() => { console.log('Promise 2'); }); Expected Output: Promise 2 Timeout 1 Promise 1 Timeout 2 Explanation: Even though the timeouts are non-blocking, the eventual tasks are processed in the order determined by the event loop. Example 3: Long-running Tasks Consider a scenario where you are performing a long computation on the main thread. console.log('Start long computation'); const start = performance.now(); while (performance.now() - start
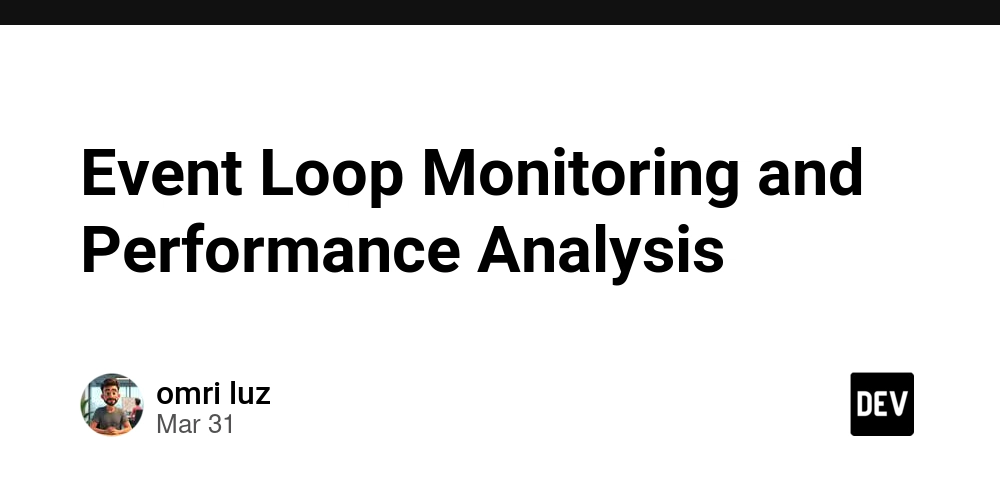
Event Loop Monitoring and Performance Analysis
Introduction
In the world of JavaScript, understanding the event loop is crucial for performance optimization and debugging. The event loop allows asynchronous operations and concurrency in a single-threaded environment. Monitoring its performance can help developers detect bottlenecks, identify runaway processes, and improve the responsiveness of applications. This comprehensive article delves into event loop monitoring and performance analysis, exploring its history, technical intricacies, best practices, and real-world use cases. Moreover, it will provide deep insights into edge cases and advanced implementations for senior developers aiming to master the art of JavaScript performance tuning.
Historical Context
JavaScript was conceived in 1995 by Brendan Eich while working at Netscape. Over the years, it has transformed from a simple scripting language for web browsers into a powerful and multifaceted tool that powers entire applications. The introduction of Node.js in 2009 extended JavaScript beyond browsers, allowing developers to run JavaScript on servers and facilitating the non-blocking I/O models that the language broadly supports.
In its early adoption, JavaScript's event-driven nature led to the formation of the event loop concept as an essential mechanism for handling asynchronous operations without blocking the single-threaded execution model. Understanding how the event loop operates has now become a crucial component within JavaScript’s ecosystem, particularly with the widespread usage of single-page applications (SPAs) and real-time web applications.
Technical Overview of the Event Loop
Before diving into monitoring and analysis, let's understand how the event loop works:
Call Stack: It maintains the execution stack of function calls. When a function is invoked, it is pushed onto the stack and popped once it completes.
Web APIs (in browsers): They help to manage asynchronous operations like timers (
setTimeout
,setInterval
), AJAX calls, and events. When these operations complete, they send their respective callbacks to the callback queue.Callback Queue: It holds messages or callbacks waiting to be executed. When the call stack is empty, the event loop pushes the first callback from the queue onto the stack for execution.
Microtask Queue: This queue is prioritized over the callback queue. Microtasks (promises and
MutationObserver
callbacks) are processed before the next event loop cycle begins.Event Loop: The event loop continuously checks if the call stack is empty and moves callbacks from the callback queue and microtask queue onto it for execution.
Understanding Event Loop Monitoring
Event loop monitoring involves tracking the time it takes for the event loop to process different tasks (microtasks and macrotasks), helping identify performance bottlenecks.
Methodology for Monitoring
Using
performance.now()
: This method provides accurate timestamps that can be leveraged to track task execution times.Event Loop Tracing: In Node.js, you can utilize built-in modules such as
perf_hooks
to create performance snapshots.JavaScript Performance Profiling: Browsers provide tools (like Chrome DevTools) that allow developers to profile JavaScript execution and visualize the event loop activity.
Code Examples
Example 1: Basic Event Loop Simulation
console.log('Start');
setTimeout(() => {
console.log('Timeout callback');
}, 0);
Promise.resolve().then(() => {
console.log('Promise callback');
});
console.log('End');
Expected Output:
Start
End
Promise callback
Timeout callback
Explanation: In this example, the promise callback is executed before the timeout callback due to the microtask queue's higher priority.
Example 2: Event Loop with Complex Nesting
setTimeout(() => {
console.log('Timeout 1');
Promise.resolve().then(() => {
console.log('Promise 1');
});
setTimeout(() => {
console.log('Timeout 2');
}, 0);
}, 0);
Promise.resolve().then(() => {
console.log('Promise 2');
});
Expected Output:
Promise 2
Timeout 1
Promise 1
Timeout 2
Explanation: Even though the timeouts are non-blocking, the eventual tasks are processed in the order determined by the event loop.
Example 3: Long-running Tasks
Consider a scenario where you are performing a long computation on the main thread.
console.log('Start long computation');
const start = performance.now();
while (performance.now() - start < 3000); // Simulate long computation
console.log('End long computation');
Performance Impact: This will block the call stack for 3 seconds, making the application unresponsive during that period. To optimize such tasks:
Edge Cases and Optimization
Edge Case: Too Many Microtasks
When using Promise
, if you create an excessive number of microtasks, it can cause performance degradation.
for (let i = 0; i < 10000; i++) {
Promise.resolve().then(() => {
// Do nothing, just enqueue
});
}
Monitoring Consideration: If you're not clearing the event loop or managing microtasks effectively, this can lead to an overwhelmed event loop.
Optimization Techniques
- Debouncing and Throttling: Implement these techniques for events like scroll and input to reduce excessive function calls.
- Web Workers: Offload heavy computations from the main thread using web workers. This way, you free up the event loop for UI updates.
-
Scheduling Tasks: Leverage
requestAnimationFrame
for animations or high-frequency updates, ensuring smoother rendering and responsiveness.
Real-World Use Cases
- Web Applications: Platforms like Google Docs use the event loop to ensure smooth editing experiences while handling real-time document synchronization.
- Gaming Engines: Fast-paced gaming relies on the event loop for rendering frames without blocking user interactions.
- Financial Applications: Stock trading platforms use the event loop and its monitoring for real-time data processing and UI responsiveness.
Performance Considerations
- Responsiveness Measures: Keep the UI thread free from heavy synchronous operations to maintain UI responsiveness.
- Microtasks vs. Macrotasks: Overuse of micro tasks can lead to starvation of macrotasks, ensuring a balanced mix is crucial.
Debugging Event Loop Issues
-
Monitoring Tools: Use tools like Node.js's
node --inspect
to explore event loop activity. - Timeline Analysis: Analyze timelines in browser dev tools to catch long running tasks.
- Runaway Promises: Watch for unhandled promise rejections that could intensify the event loop when numerous promises are pending.
Conclusion
Event loop monitoring and performance analysis is an essential aspect of advanced JavaScript programming. As applications grow in complexity and interactivity, understanding the intricacies of the event loop can dramatically improve application performance and user experience. By implementing strategies for monitoring the event loop, discovering bottlenecks, and practicing performance optimization, developers can ensure that their applications remain nimble, responsive, and user-friendly.
References
As you dive deeper into optimizing your JavaScript applications, keep this definitive guide at your side, ensuring you're always at the forefront of performance best practices.