day-17: Java Fundamentals: Variables, Methods, and Polymorphism
Variables in Java: 1. Primitive Data Types Data Type Default Value Example int 0 int age = 25; double 0.0 double price = 19.99; boolean false boolean isActive = true; char '\u0000' char grade = 'A'; 2. Reference Data Types Data Type Default Value Example String null String name = "John"; Arrays null int[] numbers = {1, 2, 3}; Methods in Java Methods are blocks of code that perform specific tasks. Parameters vs. Arguments public class EB_Assessment { // 'notice' is a parameter (defined in method) public void reading(String notice) { System.out.println(notice); } public static void main(String[] args) { EB_Assessment meter = new EB_Assessment(); // "Smart Meter" is an argument (passed when calling) meter.reading("Smart Meter"); } } Parameter: Variable in method definition (String notice) Argument: Actual value passed when calling ("Smart Meter") Method Overloading Same method name but different parameters: class Calculator { // Adds two numbers public void add(int a, int b) { System.out.println(a + b); } // Adds three numbers (Overloaded) public void add(int a, int b, int c) { System.out.println(a + b + c); } public static void main(String[] args) { Calculator calc = new Calculator(); calc.add(10, 20); // Calls first add() calc.add(10, 20, 30); // Calls second add() } } Key Points: Must change number/type of parameters Return type alone doesn't qualify as overloading Polymorphism in Java "Same method name, different implementations." 1). Method Overloading (Compile-Time Polymorphism) class Printer { void print(String text) { /* Print text */ } void print(String text, int copies) { /* Print multiple copies */ } } Method Overriding (Runtime Polymorphism) class Animal { void sound() { System.out.println("Animal sound"); } } class Dog extends Animal { @Override void sound() { System.out.println("Bark!"); } } Key Differences Concept Method Overloading Method Overriding Definition Same name, different parameters Subclass redefines parent method Binding Compile-time Runtime Inheritance Not required Required Note: The formatting and structure were optimized for clarity with AI assistance. --------------------------- End of the Blog -------------------------------
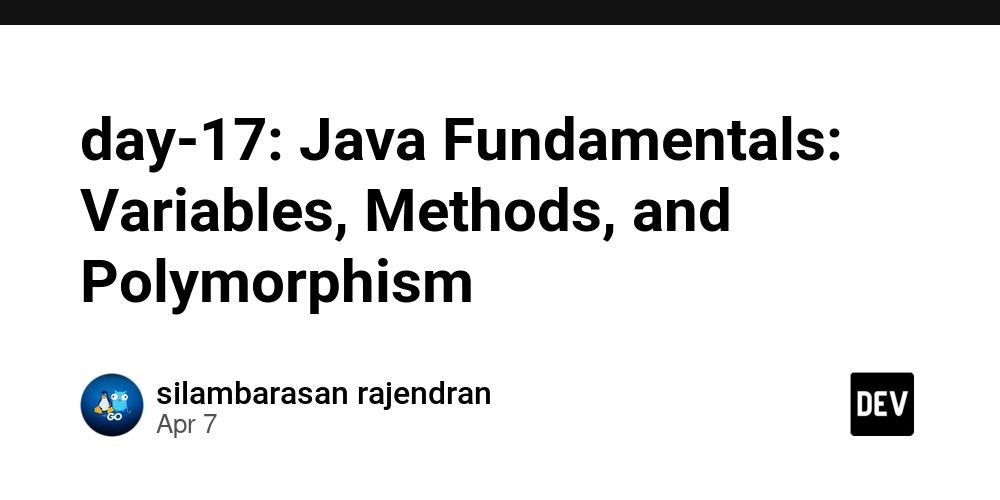
Variables in Java:
1. Primitive Data Types
Data Type Default Value Example
int 0 int age = 25;
double 0.0 double price = 19.99;
boolean false boolean isActive = true;
char '\u0000' char grade = 'A';
2. Reference Data Types
Data Type Default Value Example
String null String name = "John";
Arrays null int[] numbers = {1, 2, 3};
Methods in Java
Methods are blocks of code that perform specific tasks.
Parameters vs. Arguments
public class EB_Assessment {
// 'notice' is a parameter (defined in method)
public void reading(String notice) {
System.out.println(notice);
}
public static void main(String[] args) {
EB_Assessment meter = new EB_Assessment();
// "Smart Meter" is an argument (passed when calling)
meter.reading("Smart Meter");
}
}
Parameter: Variable in method definition (String notice)
Argument: Actual value passed when calling ("Smart Meter")
Method Overloading
Same method name but different parameters:
class Calculator {
// Adds two numbers
public void add(int a, int b) {
System.out.println(a + b);
}
// Adds three numbers (Overloaded)
public void add(int a, int b, int c) {
System.out.println(a + b + c);
}
public static void main(String[] args) {
Calculator calc = new Calculator();
calc.add(10, 20); // Calls first add()
calc.add(10, 20, 30); // Calls second add()
}
}
Key Points:
Must change number/type of parameters
Return type alone doesn't qualify as overloading
Polymorphism in Java
"Same method name, different implementations."
1). Method Overloading (Compile-Time Polymorphism)
class Printer {
void print(String text) { /* Print text */ }
void print(String text, int copies) { /* Print multiple copies */ }
}
- Method Overriding (Runtime Polymorphism)
class Animal {
void sound() { System.out.println("Animal sound"); }
}
class Dog extends Animal {
@Override
void sound() { System.out.println("Bark!"); }
}
Key Differences
Concept Method Overloading Method Overriding
Definition Same name, different parameters Subclass redefines parent method
Binding Compile-time Runtime
Inheritance Not required Required
Note: The formatting and structure were optimized for clarity with AI assistance.
--------------------------- End of the Blog -------------------------------