5 Essential Java Profiling Tools for Diagnosing Performance Bottlenecks
As a best-selling author, I invite you to explore my books on Amazon. Don't forget to follow me on Medium and show your support. Thank you! Your support means the world! Performance optimization remains a critical aspect of Java application development. As applications grow in complexity, identifying bottlenecks becomes increasingly challenging. In this article, I'll explore five powerful profiling tools that can help diagnose performance issues in Java applications. Java performance profiling is essential for creating responsive and efficient applications. Through years of optimizing Java systems, I've found that the right profiling tools can drastically reduce the time spent hunting for performance issues. Let's examine five tools that have proven invaluable in my work. JProfiler JProfiler stands out as a comprehensive commercial profiling solution with an intuitive interface. It provides detailed insights into CPU usage, memory allocation, and thread behavior with minimal overhead. What makes JProfiler particularly effective is its ability to drill down into method-level details. When I faced performance issues in a complex enterprise application, JProfiler's call tree visualization immediately highlighted a recursive method that was consuming excessive CPU cycles. JProfiler can be integrated with your application in several ways: // Option 1: Add JProfiler agent to JVM arguments java -agentpath:/path/to/libjprofilerti.so=port=8849 -jar myapp.jar // Option 2: Programmatic control with JProfiler API import com.jprofiler.api.controller.Controller; public class PerformanceCriticalSection { public void executeTask() { // Start CPU recording before the critical section Controller.startCPURecording(true); // Your performance critical code performComplexCalculation(); // Stop recording when done Controller.stopCPURecording(); } } The tool excels at tracking memory allocation patterns. Its reference tracking capabilities helped me identify memory leaks by showing object retention paths—essentially revealing which objects were preventing garbage collection. Java Flight Recorder Java Flight Recorder (JFR) represents a different approach to profiling. Built into the JDK since version 11 (and available as a commercial feature in earlier versions), JFR collects diagnostic data with remarkably low overhead—often less than 1%. I've used JFR in production environments where traditional profilers would be too intrusive. The data is collected continuously in a circular buffer, and can be extracted without stopping the application. Here's how to start a JFR recording: // Start JFR from command line java -XX:StartFlightRecording=duration=60s,filename=recording.jfr MyApplication // Or programmatically in your code import jdk.jfr.*; public class FlightRecorderExample { public static void main(String[] args) throws Exception { // Configure recording Configuration config = Configuration.getConfiguration("default"); Recording recording = new Recording(config); // Start recording recording.start(); // Run your application code performWork(); // Stop and save the recording recording.stop(); recording.dump(Path.of("myrecording.jfr")); } } JFR captures events like garbage collection, thread contention, and file I/O. When analyzing the recordings with Java Mission Control, I've discovered hidden issues like excessive lock contention and inefficient file operations that weren't apparent in standard profiling. VisualVM VisualVM is a free, open-source tool that provides a visual interface for JVM monitoring. I appreciate its versatility—it combines profiling, heap dump analysis, and monitoring features in a single application. For teams working with limited budgets, VisualVM offers significant value. The interface is straightforward, allowing developers to quickly identify memory leaks and CPU hotspots without extensive training. Using VisualVM is as simple as connecting to a running JVM process: // Launch VisualVM separately and connect to your JVM process // Or add a custom MBean to enable specific monitoring import java.lang.management.ManagementFactory; import javax.management.*; public class CustomMonitoring implements CustomMonitoringMBean { private int transactionsProcessed = 0; public CustomMonitoring() throws Exception { MBeanServer mbs = ManagementFactory.getPlatformMBeanServer(); ObjectName name = new ObjectName("com.myapp:type=Monitoring"); mbs.registerMBean(this, name); } public void incrementTransactions() { transactionsProcessed++; } public int getTransactionsProcessed() { return transactionsProcessed; } } interface CustomMonitoringMBean { int getTransactionsProcessed(); } I've often used VisualVM's sampler for initial inv
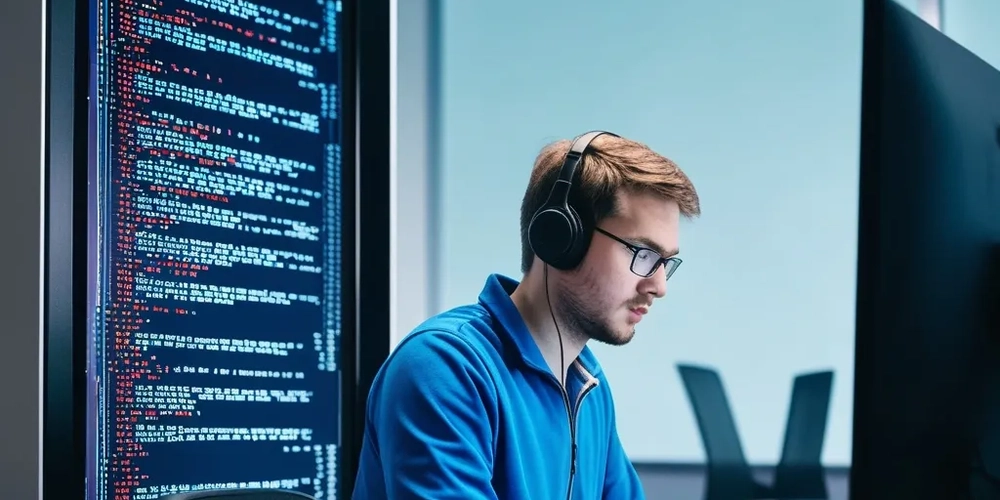
As a best-selling author, I invite you to explore my books on Amazon. Don't forget to follow me on Medium and show your support. Thank you! Your support means the world!
Performance optimization remains a critical aspect of Java application development. As applications grow in complexity, identifying bottlenecks becomes increasingly challenging. In this article, I'll explore five powerful profiling tools that can help diagnose performance issues in Java applications.
Java performance profiling is essential for creating responsive and efficient applications. Through years of optimizing Java systems, I've found that the right profiling tools can drastically reduce the time spent hunting for performance issues. Let's examine five tools that have proven invaluable in my work.
JProfiler
JProfiler stands out as a comprehensive commercial profiling solution with an intuitive interface. It provides detailed insights into CPU usage, memory allocation, and thread behavior with minimal overhead.
What makes JProfiler particularly effective is its ability to drill down into method-level details. When I faced performance issues in a complex enterprise application, JProfiler's call tree visualization immediately highlighted a recursive method that was consuming excessive CPU cycles.
JProfiler can be integrated with your application in several ways:
// Option 1: Add JProfiler agent to JVM arguments
java -agentpath:/path/to/libjprofilerti.so=port=8849 -jar myapp.jar
// Option 2: Programmatic control with JProfiler API
import com.jprofiler.api.controller.Controller;
public class PerformanceCriticalSection {
public void executeTask() {
// Start CPU recording before the critical section
Controller.startCPURecording(true);
// Your performance critical code
performComplexCalculation();
// Stop recording when done
Controller.stopCPURecording();
}
}
The tool excels at tracking memory allocation patterns. Its reference tracking capabilities helped me identify memory leaks by showing object retention paths—essentially revealing which objects were preventing garbage collection.
Java Flight Recorder
Java Flight Recorder (JFR) represents a different approach to profiling. Built into the JDK since version 11 (and available as a commercial feature in earlier versions), JFR collects diagnostic data with remarkably low overhead—often less than 1%.
I've used JFR in production environments where traditional profilers would be too intrusive. The data is collected continuously in a circular buffer, and can be extracted without stopping the application.
Here's how to start a JFR recording:
// Start JFR from command line
java -XX:StartFlightRecording=duration=60s,filename=recording.jfr MyApplication
// Or programmatically in your code
import jdk.jfr.*;
public class FlightRecorderExample {
public static void main(String[] args) throws Exception {
// Configure recording
Configuration config = Configuration.getConfiguration("default");
Recording recording = new Recording(config);
// Start recording
recording.start();
// Run your application code
performWork();
// Stop and save the recording
recording.stop();
recording.dump(Path.of("myrecording.jfr"));
}
}
JFR captures events like garbage collection, thread contention, and file I/O. When analyzing the recordings with Java Mission Control, I've discovered hidden issues like excessive lock contention and inefficient file operations that weren't apparent in standard profiling.
VisualVM
VisualVM is a free, open-source tool that provides a visual interface for JVM monitoring. I appreciate its versatility—it combines profiling, heap dump analysis, and monitoring features in a single application.
For teams working with limited budgets, VisualVM offers significant value. The interface is straightforward, allowing developers to quickly identify memory leaks and CPU hotspots without extensive training.
Using VisualVM is as simple as connecting to a running JVM process:
// Launch VisualVM separately and connect to your JVM process
// Or add a custom MBean to enable specific monitoring
import java.lang.management.ManagementFactory;
import javax.management.*;
public class CustomMonitoring implements CustomMonitoringMBean {
private int transactionsProcessed = 0;
public CustomMonitoring() throws Exception {
MBeanServer mbs = ManagementFactory.getPlatformMBeanServer();
ObjectName name = new ObjectName("com.myapp:type=Monitoring");
mbs.registerMBean(this, name);
}
public void incrementTransactions() {
transactionsProcessed++;
}
public int getTransactionsProcessed() {
return transactionsProcessed;
}
}
interface CustomMonitoringMBean {
int getTransactionsProcessed();
}
I've often used VisualVM's sampler for initial investigations. Unlike instrumentation-based profilers, the sampling approach minimizes overhead while still identifying major hotspots. When a microservice was experiencing intermittent slowdowns, VisualVM's thread dump analysis revealed a thread deadlock condition in the connection pool.
Async-profiler
Async-profiler takes a unique approach to Java profiling. It uses hardware performance counters and perf_events on Linux to capture stack traces with minimal overhead. This approach avoids the safepoint bias that affects many Java profilers.
I've found Async-profiler particularly valuable for CPU and allocation profiling in production systems. Unlike traditional profilers, it doesn't require JVM instrumentation, which means more accurate results without disturbing application behavior.
Using Async-profiler typically involves running it from the command line:
// Profiling CPU for 30 seconds
./profiler.sh -d 30 -f cpu-profile.html <pid>
// Profiling memory allocations
./profiler.sh -e alloc -d 30 -f alloc-profile.html <pid>
// For programmatic control, you can use the API
import one.profiler.AsyncProfiler;
public class ProfilingDemo {
public static void main(String[] args) throws Exception {
// Get profiler instance
AsyncProfiler profiler = AsyncProfiler.getInstance();
// Start CPU profiling
profiler.start(Event.CPU, 10000000); // 10ms sampling interval
// Run the code you want to profile
runComputation();
// Stop and generate flame graph
profiler.stop();
String report = profiler.dumpFlameGraph();
System.out.println(report);
}
}
The flame graphs generated by Async-profiler are particularly insightful. When analyzing a data processing pipeline, the flame graph clearly showed that string concatenation in a tight loop was consuming 40% of CPU time—a pattern that was easy to miss in traditional profilers.
YourKit
YourKit provides powerful memory analysis features that have saved me countless hours debugging memory issues. Its ability to track object creation and show exact allocation points in the code is particularly useful for complex applications.
YourKit excels at memory leak detection through its object retention view. When working on a large financial application, YourKit helped identify a cache that wasn't releasing references properly, causing gradual memory consumption.
Integration with YourKit is straightforward:
// Add YourKit agent to JVM arguments
java -agentpath:/path/to/libyjpagent.so=port=10001,disablestacktelemetry,disableexceptiontelemetry MyApp
// For targeted profiling, use the API
import com.yourkit.api.Controller;
public class MemoryAnalysisExample {
public void analyzeMemoryUsage() throws Exception {
Controller controller = new Controller();
// Take a memory snapshot before operation
controller.captureMemorySnapshot();
// Perform memory-intensive operation
processLargeDataSet();
// Take another snapshot to compare
controller.captureMemorySnapshot();
// Force garbage collection to identify retained objects
controller.forceGC();
controller.captureMemorySnapshot();
}
}
YourKit's CPU profiling has also proved valuable. Its call counting functionality shows exactly how many times each method is called, which helped me identify an unnecessarily repeated database query that was causing performance degradation.
Strategic Profiling Techniques
Beyond the tools themselves, effective profiling requires strategic thinking. I've developed a systematic approach to performance analysis:
- Start with high-level metrics to identify problem areas (CPU, memory, I/O, or network).
- Use targeted profiling to drill down into the specific bottleneck.
- Correlate findings with business metrics to prioritize improvements.
For CPU issues, focus on hot methods and call frequencies. In a recent project, profiling revealed that a seemingly innocent logging statement was being called millions of times in a high-throughput path.
// Before optimization
public void processTransaction(Transaction t) {
logger.debug("Processing transaction " + t.getId() + " for customer " + t.getCustomerId());
// Process transaction
}
// After optimization
public void processTransaction(Transaction t) {
if (logger.isDebugEnabled()) {
logger.debug("Processing transaction {} for customer {}", t.getId(), t.getCustomerId());
}
// Process transaction
}
For memory issues, examine allocation rates and object lifespans. Large objects with short lifespans can trigger frequent garbage collection, creating latency spikes. I've sometimes found that simple object pooling can significantly reduce GC pressure.
// Using an object pool for frequently created expensive objects
public class BufferPool {
private final ConcurrentLinkedQueue<ByteBuffer> pool = new ConcurrentLinkedQueue<>();
private final int bufferSize;
private final AtomicInteger created = new AtomicInteger(0);
public BufferPool(int bufferSize) {
this.bufferSize = bufferSize;
}
public ByteBuffer acquire() {
ByteBuffer buffer = pool.poll();
if (buffer == null) {
buffer = ByteBuffer.allocateDirect(bufferSize);
created.incrementAndGet();
}
return buffer;
}
public void release(ByteBuffer buffer) {
buffer.clear();
pool.offer(buffer);
}
public int getCreatedCount() {
return created.get();
}
}
Thread issues require careful examination of lock contention and thread states. YourKit and JProfiler both offer thread profiling features that have helped me identify synchronization bottlenecks.
In one case, profiling showed threads spending excessive time waiting on a synchronized map. Replacing it with a concurrent implementation provided an immediate performance boost:
// Before optimization
private final Map<String, UserSession> sessions = new HashMap<>();
public synchronized UserSession getSession(String id) {
return sessions.get(id);
}
public synchronized void addSession(String id, UserSession session) {
sessions.put(id, session);
}
// After optimization
private final ConcurrentHashMap<String, UserSession> sessions = new ConcurrentHashMap<>();
public UserSession getSession(String id) {
return sessions.get(id);
}
public void addSession(String id, UserSession session) {
sessions.put(id, session);
}
Choosing the Right Tool
Each profiling tool has its strengths. I select tools based on:
- JProfiler when I need comprehensive analysis with an intuitive interface
- Java Flight Recorder for production monitoring with minimal overhead
- VisualVM for quick investigations and team-wide accessibility
- Async-profiler when accuracy in production environments is paramount
- YourKit when memory leaks and complex object relationships need analysis
Sometimes, combining tools provides the most complete picture. I might use JFR for continuous monitoring, then deploy Async-profiler when a specific issue occurs, followed by detailed analysis with JProfiler in a development environment.
Performance Testing Integration
Integrating profiling with automated performance testing creates a powerful feedback loop. I've set up CI/CD pipelines that run JMeter tests with JFR enabled, automatically analyzing results to detect performance regressions.
For web applications, correlating backend profiling data with frontend metrics provides end-to-end visibility:
// Adding correlation IDs to track requests across systems
@WebFilter("/*")
public class PerformanceMonitoringFilter implements Filter {
private static final ThreadLocal<String> correlationId = new ThreadLocal<>();
@Override
public void doFilter(ServletRequest request, ServletResponse response, FilterChain chain)
throws IOException, ServletException {
HttpServletRequest req = (HttpServletRequest) request;
HttpServletResponse res = (HttpServletResponse) response;
// Extract or generate correlation ID
String requestId = req.getHeader("X-Correlation-ID");
if (requestId == null) {
requestId = UUID.randomUUID().toString();
}
correlationId.set(requestId);
// Add correlation ID to response
res.addHeader("X-Correlation-ID", requestId);
// Add to MDC for logging
MDC.put("correlationId", requestId);
try {
long startTime = System.nanoTime();
chain.doFilter(request, response);
long duration = System.nanoTime() - startTime;
// Log or record timing data with correlation ID
logger.info("Request {} completed in {} ms", requestId, duration / 1_000_000);
} finally {
MDC.remove("correlationId");
correlationId.remove();
}
}
}
Real-World Optimization Examples
The most significant performance improvements often come from fundamental algorithmic changes identified through profiling. In a document processing system, profiling revealed that a text analysis algorithm had O(n²) complexity:
// Before optimization: O(n²) complexity
public List<String> findRepeatedPhrases(String text) {
List<String> phrases = new ArrayList<>();
String[] words = text.split("\\s+");
for (int i = 0; i < words.length - 3; i++) {
String phrase = words[i] + " " + words[i+1] + " " + words[i+2];
// Check if phrase appears elsewhere in text
for (int j = i + 3; j < words.length - 2; j++) {
String compareTo = words[j] + " " + words[j+1] + " " + words[j+2];
if (phrase.equals(compareTo)) {
phrases.add(phrase);
break;
}
}
}
return phrases;
}
// After optimization: O(n) complexity using a HashMap
public List<String> findRepeatedPhrases(String text) {
Map<String, Integer> phraseCounts = new HashMap<>();
List<String> repeatedPhrases = new ArrayList<>();
String[] words = text.split("\\s+");
for (int i = 0; i < words.length - 2; i++) {
String phrase = words[i] + " " + words[i+1] + " " + words[i+2];
int count = phraseCounts.getOrDefault(phrase, 0) + 1;
phraseCounts.put(phrase, count);
if (count == 2) {
repeatedPhrases.add(phrase);
}
}
return repeatedPhrases;
}
In a database-driven application, profiling with YourKit showed excessive database calls. Implementing batch processing and caching dramatically improved throughput:
// Before optimization: individual database calls
public List<ProductInfo> getProductDetails(List<String> productIds) {
List<ProductInfo> results = new ArrayList<>();
for (String id : productIds) {
results.add(jdbcTemplate.queryForObject(
"SELECT * FROM products WHERE id = ?",
new Object[]{id},
productRowMapper));
}
return results;
}
// After optimization: batch query and caching
@Cacheable("productDetails")
public List<ProductInfo> getProductDetails(List<String> productIds) {
if (productIds.isEmpty()) {
return Collections.emptyList();
}
// Create placeholders for SQL IN clause
String placeholders = String.join(",", Collections.nCopies(productIds.size(), "?"));
return jdbcTemplate.query(
"SELECT * FROM products WHERE id IN (" + placeholders + ")",
productIds.toArray(),
productRowMapper);
}
Conclusion
Profiling tools provide the visibility needed to optimize Java applications effectively. Whether you're battling memory leaks, CPU bottlenecks, or thread contention issues, the right profiling strategy can guide you to the root cause.
I've found that performance optimization is rarely a one-time effort. Regular profiling as part of your development cycle helps maintain performance as applications evolve. The small investment in learning these tools pays enormous dividends in application quality and user satisfaction.
Remember that the most insightful profiling often happens in realistic environments with production-like data volumes and access patterns. With the low-overhead options available today, there's no reason not to incorporate performance profiling into your regular development practices.
By mastering these five profiling tools and developing a systematic approach to performance analysis, you'll be well-equipped to tackle even the most challenging performance problems in your Java applications.
101 Books
101 Books is an AI-driven publishing company co-founded by author Aarav Joshi. By leveraging advanced AI technology, we keep our publishing costs incredibly low—some books are priced as low as $4—making quality knowledge accessible to everyone.
Check out our book Golang Clean Code available on Amazon.
Stay tuned for updates and exciting news. When shopping for books, search for Aarav Joshi to find more of our titles. Use the provided link to enjoy special discounts!
Our Creations
Be sure to check out our creations:
Investor Central | Investor Central Spanish | Investor Central German | Smart Living | Epochs & Echoes | Puzzling Mysteries | Hindutva | Elite Dev | JS Schools
We are on Medium
Tech Koala Insights | Epochs & Echoes World | Investor Central Medium | Puzzling Mysteries Medium | Science & Epochs Medium | Modern Hindutva