10 React Best Practices Every Developer Should Follow
The industry leader in front-end development today is still React. Its robust abstractions, vibrant community, and rich ecosystem let developers create everything from enterprise-level programs to side projects. However, immense power can come with considerable complications. React apps may easily get unmanageable, inconsistent, or difficult to maintain if you neglect to stick to tried-and-true best practices. In order to create scalable, stable, effective, and clean React apps in 2025, every serious developer should follow these ten React best practices. 1. Build Small, Focused, and Reusable Components Why It Matters: It's component-based, React. Your user interface is essentially a tree of tiny, reusable parts. But only when those elements are single-responsibility and concentrated does it succeed. Avoid bloated “God components” that handle UI, logic, and side-effects all in one file. What You Should Do: One component = one purpose Break large components into smaller ones Reuse components across the app to avoid duplication Example: Instead of: which does everything, break it down into: ✅ Benefits: Easier to debug and test Encourages reuse Keeps files short and readable 2. Use Functional Components with Hooks (Over Class Components) The Evolution: Class components were commonplace before to React 16.8. Functional parts with hooks, however, are currently regarded as the best. Benefits: Cleaner syntax No need for this binding Hooks make state and lifecycle management modular and reusable Common Hooks to Master: | Hook | Purpose | |---------------------------|-----------------------------------------------------| | `useState` | Local state | | `useEffect` | Side effects (API calls, subscriptions) | | `useRef` | Persisting mutable values without re-renders | | `useContext` | Access global data without prop drilling | | `useReducer` | More complex state logic (Redux-like) | | `useCallback`, `useMemo` | Performance optimization | | **Custom Hooks** | Abstract logic into reusable pieces | Example: function Counter() { const [count, setCount] = useState(0); return setCount(count + 1)}>{count}; } 3. Lift State Up Thoughtfully (Avoid Prop Drilling) What’s Lifting State? The closest common ancestor should be lifted when two or more components require access to the same state. What to Avoid: Prop drilling is the process of inserting props through several unnecessary intermediary components. Tools to Help: React Context for lightweight shared state Zustand, Jotai, or Redux Toolkit for complex state across many components. Example: Instead of: Use: Rule: Lift state only as high as necessary — not higher. 4. Embrace Type Safety: TypeScript or PropTypes Why Type Checking Is Essential: This kind of JavaScript is dynamic. As your app grows, this adaptability may turn into a drawback. It provides a layer of protection using TypeScript (or PropTypes). Benefits of TypeScript: Catch bugs before runtime Enforce consistent interfaces Improve developer experience with autocomplete and inline docs TypeScript Example: type User = { id: number; name: string; email: string; }; function UserCard({ user }: { user: User }) { return {user.name}; } Use PropTypes only for simple apps. For anything real-world, go with TypeScript. 5. Create a Scalable File/Folder Structure Why Structure Matters: Untidy structures become uncontrollable. Onboarding is seamless and cooperation is facilitated by a scalable design. Popular Patterns: Feature-based structure Domain-driven structure Atomic design structure (atoms, molecules, organisms, etc.) Suggested Setup: src/ ├── components/ # Reusable UI components ├── features/ # Feature-specific modules │ └── auth/ │ └── dashboard/ ├── hooks/ # Custom hooks ├── contexts/ # Context providers ├── pages/ # Route-level pages (Next.js) ├── utils/ # Helper functions ├── assets/ # Images, fonts, etc. Keep a README.md in major folders to explain their structure. 6. Optimize Rendering: Memoize Where It Counts Why Optimize? When components' props or states change, React re-renders them. For big trees, this may get costly. Memoization Tools: React.memo() – skips re-renders of pure functional components useMemo() – caches expensive calculations useCallback() – memoizes callb
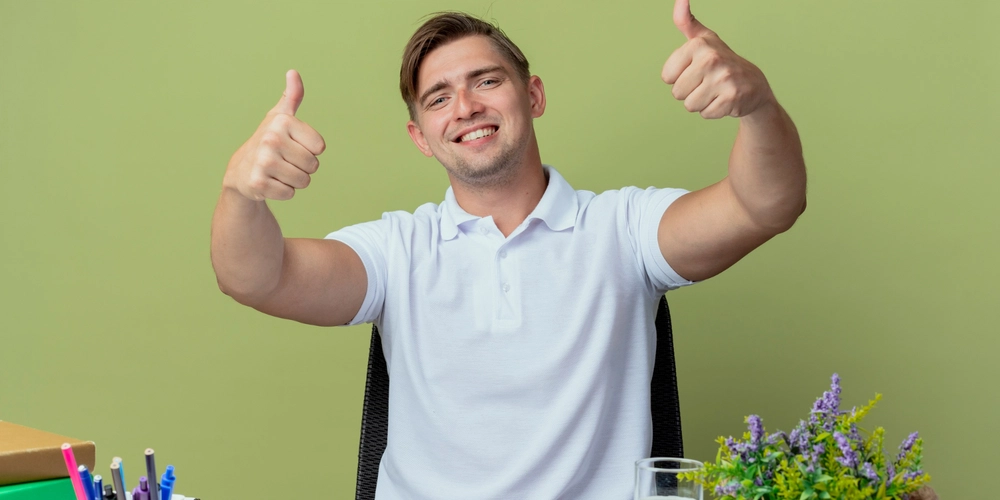
The industry leader in front-end development today is still React. Its robust abstractions, vibrant community, and rich ecosystem let developers create everything from enterprise-level programs to side projects.
However, immense power can come with considerable complications. React apps may easily get unmanageable, inconsistent, or difficult to maintain if you neglect to stick to tried-and-true best practices.
In order to create scalable, stable, effective, and clean React apps in 2025, every serious developer should follow these ten React best practices.
1. Build Small, Focused, and Reusable Components
Why It Matters:
It's component-based, React. Your user interface is essentially a tree of tiny, reusable parts. But only when those elements are single-responsibility and concentrated does it succeed.
Avoid bloated “God components” that handle UI, logic, and side-effects all in one file.
What You Should Do:
- One component = one purpose
- Break large components into smaller ones
- Reuse components across the app to avoid duplication
Example:
Instead of:
which does everything, break it down into:
✅ Benefits:
- Easier to debug and test
- Encourages reuse
- Keeps files short and readable
2. Use Functional Components with Hooks (Over Class Components)
The Evolution:
Class components were commonplace before to React 16.8. Functional parts with hooks, however, are currently regarded as the best.
Benefits:
- Cleaner syntax
No need for this binding
Hooks make state and lifecycle management modular and reusable
Common Hooks to Master:
| Hook | Purpose |
|---------------------------|-----------------------------------------------------|
| `useState` | Local state |
| `useEffect` | Side effects (API calls, subscriptions) |
| `useRef` | Persisting mutable values without re-renders |
| `useContext` | Access global data without prop drilling |
| `useReducer` | More complex state logic (Redux-like) |
| `useCallback`, `useMemo` | Performance optimization |
| **Custom Hooks** | Abstract logic into reusable pieces |
Example:
function Counter() {
const [count, setCount] = useState(0);
return ;
}
3. Lift State Up Thoughtfully (Avoid Prop Drilling)
What’s Lifting State?
The closest common ancestor should be lifted when two or more components require access to the same state.
What to Avoid:
Prop drilling is the process of inserting props through several unnecessary intermediary components.
Tools to Help:
- React Context for lightweight shared state
- Zustand, Jotai, or Redux Toolkit for complex state across many components.
Example:
Instead of:
Use:
Rule: Lift state only as high as necessary — not higher.
4. Embrace Type Safety: TypeScript or PropTypes
Why Type Checking Is Essential:
This kind of JavaScript is dynamic. As your app grows, this adaptability may turn into a drawback. It provides a layer of protection using TypeScript (or PropTypes).
Benefits of TypeScript:
- Catch bugs before runtime
- Enforce consistent interfaces
- Improve developer experience with autocomplete and inline docs
TypeScript Example:
type User = {
id: number;
name: string;
email: string;
};
function UserCard({ user }: { user: User }) {
return {user.name};
}
Use PropTypes only for simple apps. For anything real-world, go with TypeScript.
5. Create a Scalable File/Folder Structure
Why Structure Matters:
Untidy structures become uncontrollable. Onboarding is seamless and cooperation is facilitated by a scalable design.
Popular Patterns:
- Feature-based structure
- Domain-driven structure
- Atomic design structure (atoms, molecules, organisms, etc.)
Suggested Setup:
src/
├── components/ # Reusable UI components
├── features/ # Feature-specific modules
│ └── auth/
│ └── dashboard/
├── hooks/ # Custom hooks
├── contexts/ # Context providers
├── pages/ # Route-level pages (Next.js)
├── utils/ # Helper functions
├── assets/ # Images, fonts, etc.
Keep a README.md in major folders to explain their structure.
6. Optimize Rendering: Memoize Where It Counts
Why Optimize?
When components' props or states change, React re-renders them. For big trees, this may get costly.
Memoization Tools:
-
React.memo()
– skips re-renders of pure functional components -
useMemo()
– caches expensive calculations -
useCallback()
– memoizes callback functions to prevent child re-renders
Pro Tip:
Don’t blindly optimize. Use React Profiler to identify bottlenecks.
7. Write Reusable Custom Hooks
Why Custom Hooks?
Hooks are reusable containers for logic. Use a custom hook if you're repeating logic across components.
Example:
function useFetch(url) {
const [data, setData] = useState(null);
useEffect(() => {
fetch(url).then(res => res.json()).then(setData);
}, [url]);
return data;
}
Use it like:
const userData = useFetch('/api/user');
Best Practices:
Prefix with use
Keep hooks pure and composable
Place inside a hooks
/ folder
8. Implement Error Boundaries and Fallback UIs
React Is Not Bulletproof
An unresolved problem in one part might cause the user interface to collapse. React Error Boundaries provide for gentle fallbacks by catching them.
Example:
class ErrorBoundary extends React.Component {
state = { hasError: false };
static getDerivedStateFromError() {
return { hasError: true };
}
render() {
if (this.state.hasError) return Something went wrong.
;
return this.props.children;
}
}
Also use:
-
Suspense
with lazy loading - Try/catch in async functions
- External libraries like
react-error-boundary
9. Test Your Components: Focus on Behavior, Not Implementation
Why Testing Matters in React Apps
Building trust in your app is the goal of testing, not simply finding flaws. Testing in a contemporary React application guarantees that your user interface (UI) functions properly across a range of devices, states, and user interactions. As the project progresses, it preserves your logic, offers code-based documentation, and avoids regressions.
*Mindset Shift: * Think of tests as safety nets, not chores. They let you refactor boldly, knowing the core logic still works.
Types of Tests You Should Know
| Test Type | Purpose | Tools to Use |
|----------------|---------------------------------------------------|----------------------------------------------|
| Unit Tests | Test isolated components and logic | Jest, React Testing Library |
| Integration | Test how components work together | React Testing Library, Vitest |
| E2E Tests | Test full app behavior as a user would | Cypress, Playwright, TestCafe |
| Snapshot | Detect unintended UI changes (with caution) | Jest (with snapshot plugin) |
Recommended Tools & Ecosystem
- React Testing Library (RTL): tests your application using user interaction, with an emphasis on events, accessibility roles, and DOM.
- Jest: A battle-tested JavaScript testing framework with built-in mocking, assertions, and snapshots.
- Cypress or Playwright: To do thorough end-to-end (E2E) testing in authentic browser settings. really automates user flows.
- MSW (Mock Service Worker): Tests can mock API answers without requiring a backend.
Best Practices for Testing in React
1. Write tests for user behavior, not internal implementation
- Bad: Checking internal state or class names
- Good: Clicking a button and checking if the result shows up
2. Use getByRole, getByLabelText,
and getByText
over getByTestId
- This ensures your app is accessible and testable
3. Test edge cases:
- Empty states
- Slow API responses
- Invalid inputs
- Mobile viewport sizes (in E2E)
4. Keep tests fast and deterministic — avoid flaky tests by mocking APIs and using fake timers where needed
Sample Test Case (React Testing Library)
jsx
CopyEdit
test("increments counter on click", () => {
render( );
const button = screen.getByRole("button", { name: /count/i });
fireEvent.click(button);
expect(button).toHaveTextContent("1");
});
Golden Rule:
- Test what your users care about — behavior, accessibility, interaction.
- Avoid testing component internals — structure, state variables, or DOM tree shape.
10. Accessibility (a11y) Is a Must — Not a Maybe
Why Accessibility Matters
Being accessible is a fundamental duty, not a secondary consideration. Everyone should be able to use your app, including those with limitations. Equal access to your product is guaranteed by inclusive design, regardless of visual impairments, movement difficulties, or cognitive difficulties.
In many countries, accessibility is also a legal requirement (e.g., ADA in the U.S., EN 301 549 in Europe).
a11y Best Practices for React Developers
1. Use Semantic HTML
- Prefer native elements: , , ,
- Avoid divs for interactive UI — screen readers skip them
2. Ensure Keyboard Navigability
- Every interactive element should be reachable and operable via Tab and Enter
- Use tabindex thoughtfully (avoid tabindex="0" overload)
3. Add ARIA Attributes When Needed
- Use aria-label, aria-hidden, aria-live to give screen readers context
- But don’t overuse ARIA when semantic HTML can do the job
4. Provide Alt Text for Images
- Use meaningful alt="" for essential images
- Use alt="" to hide decorative ones
5. Color Contrast and Focus Indicators
- Ensure text has high contrast (check against WCAG AA/AAA)
- Don’t remove focus outlines — customize them if needed
6. Error Handling for Forms
- Use aria-describedby to link form errors
- Validate on blur or submit, not just onChange
Tools to Ensure a11y Compliance
- axe DevTools (Chrome Extension) — Real-time analysis with WCAG violations
- eslint-plugin-jsx-a11y — Lint for missing roles, alt texts, tab traps
- Lighthouse (Chrome/CI) — Score a11y in your audits
- Screen Readers: NVDA (Windows), VoiceOver (macOS), ChromeVox
Real-World Accessibility Audit Tips
- Navigate your entire app using just a keyboard
- Use a screen reader to browse common flows
- Test color contrast with tools like contrast-ratio.com
- Avoid animations that could trigger motion disorders (respect
prefers-reduced-motion
)
Final Takeaways: Build for People and Future-Proof Your Code
React gives you the tools. These practices give you the discipline.
- Clean, modular components.
- Modern features (hooks, TypeScript, context).
- Confident, behavior-based tests.
- Accessible and inclusive experiences.
- Scalable architecture and file structures.
- Safe error handling and performance tuning.
You are creating high-quality software that scales, endures, and delights when you include these approaches into your process.