10 Essential Laravel Helpers You Should Be Using
Laravel offers many global helper functions that make your code cleaner and easier to write. In this post, I’ll show you 10 helper functions I often use in real projects — they can help you write better and more readable code. class_basename() Get only the class name, without the namespace. class_basename(App\Models\User::class); // 'User' collect() Create a collection from an array great for working with data. collect([1, 2, 3])->map(fn($n) => $n * 2); // [2, 4, 6] str() and Str::of() Useful for working with strings in a fluent way. Str::of('Laravel')->lower()->append(' rocks!'); // 'laravel rocks!' throw_if() and throw_unless() Throw an exception if a condition is true or false. throw_if(!$user, new Exception('User not found')); value() Returns a value or runs a closure and returns the result. $value = value(fn() => 'Generated dynamically'); // 'Generated dynamically' retry() Try something again a few times until it works or fails. $response = retry(3, function () { return Http::get('https://api.example.com'); }, 100); tap() Run an action on a value and return the value. $user = tap(User::find(1), function ($user) { $user->logAccess(); }); blank() and filled() Check if a value is "empty" or "filled", more flexible than empty(). blank(''); // true filled('Laravel'); // true optional() Avoids errors when working with null objects. $user = null; $name = optional($user)->name; // null, no error data_get() Safely get a value from a nested array or object. $data = ['user' => ['name' => 'Ana']]; $name = data_get($data, 'user.name'); // 'Ana' Conclusion Laravel helpers are great tools for writing clean, expressive code. If you’re not using them yet, give them a try in your next project and feel the difference.
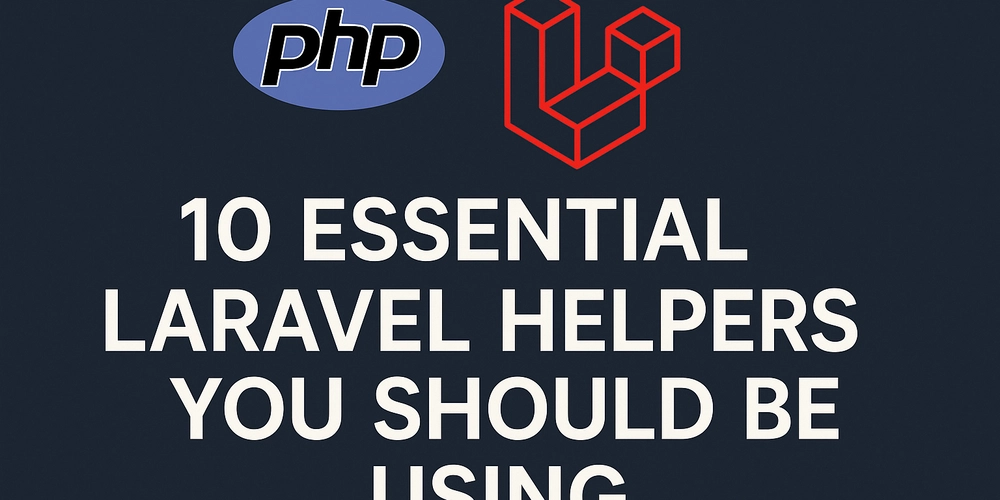
Laravel offers many global helper functions that make your code cleaner and easier to write. In this post, I’ll show you 10 helper functions I often use in real projects — they can help you write better and more readable code.
class_basename()
Get only the class name, without the namespace.
class_basename(App\Models\User::class); // 'User'
collect()
Create a collection from an array great for working with data.
collect([1, 2, 3])->map(fn($n) => $n * 2); // [2, 4, 6]
str() and Str::of()
Useful for working with strings in a fluent way.
Str::of('Laravel')->lower()->append(' rocks!'); // 'laravel rocks!'
throw_if() and throw_unless()
Throw an exception if a condition is true or false.
throw_if(!$user, new Exception('User not found'));
value()
Returns a value or runs a closure and returns the result.
$value = value(fn() => 'Generated dynamically');
// 'Generated dynamically'
retry()
Try something again a few times until it works or fails.
$response = retry(3, function () {
return Http::get('https://api.example.com');
}, 100);
tap()
Run an action on a value and return the value.
$user = tap(User::find(1), function ($user) {
$user->logAccess();
});
blank() and filled()
Check if a value is "empty" or "filled", more flexible than empty()
.
blank(''); // true
filled('Laravel'); // true
optional()
Avoids errors when working with null objects.
$user = null;
$name = optional($user)->name; // null, no error
data_get()
Safely get a value from a nested array or object.
$data = ['user' => ['name' => 'Ana']];
$name = data_get($data, 'user.name'); // 'Ana'
Conclusion
Laravel helpers are great tools for writing clean, expressive code. If you’re not using them yet, give them a try in your next project and feel the difference.