Writing Clean Code in Front-end: KISS, DRY, YAGNI, and Beyond
In the fast-paced world of software development, writing code that simply works is no longer enough. As systems grow more complex and teams become more collaborative, clean code becomes essential, not just for functionality, but for maintainability, scalability, and readability. Clean code isn’t just aesthetically pleasing. It’s a discipline. It reflects thoughtful engineering and a respect for both your future self and your fellow developers. In this article, focusing on the front-end developer, we’ll explore what clean code means and dig into three core principles that help us write better software: KISS, DRY, and YAGNI. KISS: Keep It Simple, Stupid The KISS principle reminds us not to overcomplicate. Complexity is the enemy of maintainability. KISS = Don't build a rocket ship when a bicycle will do. Avoid clever, convoluted solutions when a simpler approach would suffice. The goal is not to impress, but to communicate. Simplicity often requires more thought and a better understanding of the problem at hand, but it pays off long-term. Examples Don't do this const isAdmin = user?.roles?.includes('admin') && user?.permissions?.some(p => p.code === 'ALL_ACCESS') && user?.metadata?.active === true; Do this const isAdmin = checkUserIsAdmin(user); DRY: Don’t Repeat Yourself DRY is a fundamental principle in clean code: eliminate repetition. Repeated code is harder to maintain, more error-prone, and signals an opportunity for abstraction. Every piece of knowledge should have a single, unambiguous representation in your codebase. That could mean extracting common logic into a helper function, using inheritance or composition, or consolidating configuration in one place. But beware: Don’t Repeat Yourself doesn’t mean “abstract everything.” Over-abstraction can lead to confusion and make simple things harder to change. DRY is about meaningful consolidation—not premature generalization. Examples Don't do this Save Submit Send Do this const PrimaryButton = ({ children, ...props }) => ( {children} ); Save Submit Send YAGNI: You Ain’t Gonna Need It YAGNI is a mantra of agile and lean coding: Don’t write code until you absolutely need it. It’s tempting to try to "future-proof" software by adding features or flexibility that might be useful someday. But that leads to bloat, confusion, and wasted effort. YAGNI keeps us focused on solving today’s problems with clarity and purpose. Build only what is required. When the need truly arises, refactor or extend. Clean Code Is a Mindset These principles, KISS, DRY, and YAGNI, aren’t just checklists. They reflect a mindset of craftsmanship. Clean code comes from empathy: writing software that your colleagues (and future you) can understand, modify, and trust. Clean code is code that is easy to read, easy to understand, and easy to change. It’s not just about making things "look nice"—it's about writing code that others (and future-you) can work with confidently and efficiently. In frontend development, clean code ensures that your UI logic, components, styles, and data flows are predictable, modular, and maintainable. Whether you’re working with React, Vue, or vanilla JavaScript, the principles of clean code help you ship features faster, debug less, and collaborate better. Clean code is: Readable – Someone new to the codebase can understand what’s happening without asking questions. Simple: Avoids unnecessary complexity. Clear over clever. Consistent: Follows the same naming conventions, formatting, and structure across the project. Modular: Organized into small, single-purpose pieces (e.g., components, functions). Testable: Designed in a way that makes unit and integration testing easy. Descriptive: Uses meaningful names for variables, functions, and components. "Clean code always looks like it was written by someone who cares." — Robert C. Martin (Uncle Bob) In the world of frontend, that care translates to things like: Writing small, focused components Keeping business logic separate from UI rendering Using clear naming (e.g., SubmitButton instead of Btn1) Avoiding prop-drilling hell or unnecessary global state Writing styles that are easy to manage and extend Clean code makes everything smoother, from code reviews and onboarding to bug fixing and feature scaling. Conclusion Writing clean code isn’t an afterthought. It’s a foundational skill that distinguishes good developers from great ones. In the fast-evolving world of frontend development, where UI complexity grows and user expectations are high, clean code becomes even more critical. By embracing simplicity (KISS), avoiding repetition (DRY), and resisting the urge to over-engineer (YAGNI), we not only write code that works, we write code that scales, lasts, and empowers teams to move fast without breaking things. Clean code is al
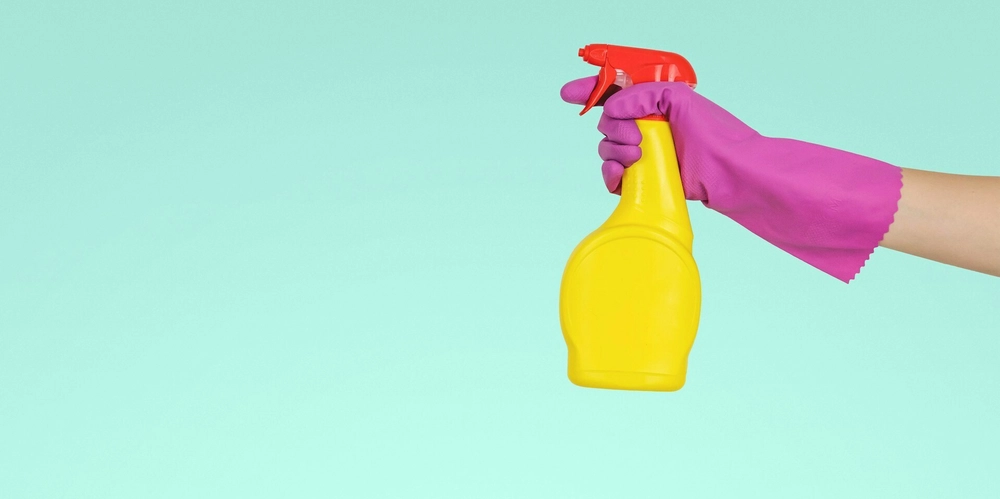
In the fast-paced world of software development, writing code that simply works is no longer enough. As systems grow more complex and teams become more collaborative, clean code becomes essential, not just for functionality, but for maintainability, scalability, and readability.
Clean code isn’t just aesthetically pleasing. It’s a discipline. It reflects thoughtful engineering and a respect for both your future self and your fellow developers. In this article, focusing on the front-end developer, we’ll explore what clean code means and dig into three core principles that help us write better software: KISS, DRY, and YAGNI.
KISS: Keep It Simple, Stupid
The KISS principle reminds us not to overcomplicate. Complexity is the enemy of maintainability.
KISS = Don't build a rocket ship when a bicycle will do.
Avoid clever, convoluted solutions when a simpler approach would suffice. The goal is not to impress, but to communicate. Simplicity often requires more thought and a better understanding of the problem at hand, but it pays off long-term.
Examples
Don't do this
const isAdmin = user?.roles?.includes('admin') && user?.permissions?.some(p => p.code === 'ALL_ACCESS') && user?.metadata?.active === true;
Do this
const isAdmin = checkUserIsAdmin(user);
DRY: Don’t Repeat Yourself
DRY is a fundamental principle in clean code: eliminate repetition. Repeated code is harder to maintain, more error-prone, and signals an opportunity for abstraction.
Every piece of knowledge should have a single, unambiguous representation in your codebase. That could mean extracting common logic into a helper function, using inheritance or composition, or consolidating configuration in one place.
But beware: Don’t Repeat Yourself doesn’t mean “abstract everything.” Over-abstraction can lead to confusion and make simple things harder to change. DRY is about meaningful consolidation—not premature generalization.
Examples
Don't do this
Do this
const PrimaryButton = ({ children, ...props }) => (
);
Save
Submit
Send
YAGNI: You Ain’t Gonna Need It
YAGNI is a mantra of agile and lean coding:
Don’t write code until you absolutely need it.
It’s tempting to try to "future-proof" software by adding features or flexibility that might be useful someday. But that leads to bloat, confusion, and wasted effort. YAGNI keeps us focused on solving today’s problems with clarity and purpose.
Build only what is required. When the need truly arises, refactor or extend.
Clean Code Is a Mindset
These principles, KISS, DRY, and YAGNI, aren’t just checklists. They reflect a mindset of craftsmanship. Clean code comes from empathy: writing software that your colleagues (and future you) can understand, modify, and trust.
Clean code is code that is easy to read, easy to understand, and easy to change. It’s not just about making things "look nice"—it's about writing code that others (and future-you) can work with confidently and efficiently.
In frontend development, clean code ensures that your UI logic, components, styles, and data flows are predictable, modular, and maintainable. Whether you’re working with React, Vue, or vanilla JavaScript, the principles of clean code help you ship features faster, debug less, and collaborate better.
Clean code is:
Readable – Someone new to the codebase can understand what’s happening without asking questions.
- Simple: Avoids unnecessary complexity. Clear over clever.
- Consistent: Follows the same naming conventions, formatting, and structure across the project.
- Modular: Organized into small, single-purpose pieces (e.g., components, functions).
- Testable: Designed in a way that makes unit and integration testing easy.
- Descriptive: Uses meaningful names for variables, functions, and components.
"Clean code always looks like it was written by someone who cares."
— Robert C. Martin (Uncle Bob)
In the world of frontend, that care translates to things like:
- Writing small, focused components
- Keeping business logic separate from UI rendering
- Using clear naming (e.g., SubmitButton instead of Btn1)
- Avoiding prop-drilling hell or unnecessary global state
- Writing styles that are easy to manage and extend
Clean code makes everything smoother, from code reviews and onboarding to bug fixing and feature scaling.
Conclusion
Writing clean code isn’t an afterthought. It’s a foundational skill that distinguishes good developers from great ones. In the fast-evolving world of frontend development, where UI complexity grows and user expectations are high, clean code becomes even more critical.
By embracing simplicity (KISS), avoiding repetition (DRY), and resisting the urge to over-engineer (YAGNI), we not only write code that works, we write code that scales, lasts, and empowers teams to move fast without breaking things.
Clean code is also an act of kindness toward your teammates, your future self, and anyone who’ll ever touch your codebase.
Remember: Code is read more often than it is written. Make yours worth reading.