Working with Authentication and Authorization in C#: A Developer’s Guide
As a C# developer, implementing authentication (verifying user identity) and authorization (controlling access to resources) is essential for building secure applications. The .NET ecosystem offers multiple tools and frameworks to handle these tasks. Below is an overview of common options and when to use them. 1. ASP.NET Core Identity What It Is: A built-in framework for managing user accounts, passwords, roles, and claims. Best For: Applications that store user data locally (e.g., traditional web apps with a user database). Pros: Integrated with ASP.NET Core. Supports customization (e.g., adding profile fields). Includes features like two-factor authentication and external logins. Cons: Requires maintaining a user database. 2. OAuth 2.0 / OpenID Connect What It Is: Standards for allowing users to log in via third-party providers (e.g., Google, Microsoft, Auth0). Best For: Apps where users should sign in with existing accounts (e.g., social media, enterprise SSO). Implementation: Use libraries like Microsoft.AspNetCore.Authentication.OpenIdConnect. Pros: No need to manage passwords. Scalable for large applications. Cons: Relies on third-party services. 3. JWT (JSON Web Tokens) What It Is: Tokens that securely transmit user information between parties. Best For: APIs, microservices, or stateless applications. Implementation: Generate tokens using System.IdentityModel.Tokens.Jwt and validate them via middleware. Pros: Lightweight and stateless. Works well with OAuth. Cons: Tokens must be securely stored and managed. 4. Windows Authentication What It Is: Uses Active Directory to authenticate domain users. Best For: Internal/intranet applications (e.g., corporate tools). Pros: Seamless for Windows users. No login forms required. Cons: Limited to Windows environments. 5. Role-Based vs. Policy-Based Authorization Role-Based: Grants access based on user roles (e.g., "Admin" or "User"). [Authorize(Roles = "Admin")] Policy-Based: Uses flexible rules (e.g., "Over18" or "CanEditPosts"). services.AddAuthorization(options => options.AddPolicy("Over18", policy => policy.RequireClaim("Age", "18"))); Best For: Role-based: Simple role checks. Policy-based: Complex rules (e.g., combining claims, roles, or custom logic). 6. Third-Party Services (Auth0, Firebase, Okta) What They Are: Managed solutions for authentication and authorization. Best For: Apps needing quick setup or advanced features (e.g., multi-factor auth). Pros: Reduce development time. Enterprise-grade security. Cons: Costs increase with user volume. Choosing the Right Option Scenario Recommended Solution Custom user database ASP.NET Core Identity External logins (e.g., Google) OAuth 2.0/OpenID Connect API/microservices JWT Corporate intranet app Windows Authentication Complex access rules Policy-Based Authorization Rapid development Third-party services (e.g., Auth0) Conclusion The best choice depends on your application’s needs. For example: Use ASP.NET Core Identity for full control over user data. Opt for JWT + OAuth if building a decoupled API. Leverage third-party services to save time and ensure security. Always prioritize security practices like HTTPS, token expiration, and input validation. By combining these tools, you can create a secure and scalable authentication system in your C# applications.
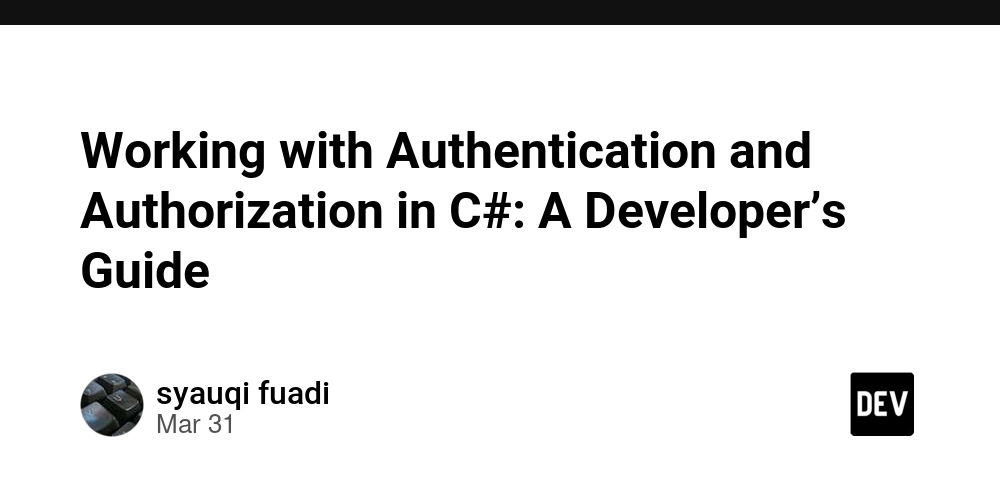
As a C# developer, implementing authentication (verifying user identity) and authorization (controlling access to resources) is essential for building secure applications. The .NET ecosystem offers multiple tools and frameworks to handle these tasks. Below is an overview of common options and when to use them.
1. ASP.NET Core Identity
What It Is: A built-in framework for managing user accounts, passwords, roles, and claims.
Best For: Applications that store user data locally (e.g., traditional web apps with a user database).
Pros:
- Integrated with ASP.NET Core.
- Supports customization (e.g., adding profile fields).
- Includes features like two-factor authentication and external logins. Cons: Requires maintaining a user database.
2. OAuth 2.0 / OpenID Connect
What It Is: Standards for allowing users to log in via third-party providers (e.g., Google, Microsoft, Auth0).
Best For: Apps where users should sign in with existing accounts (e.g., social media, enterprise SSO).
Implementation: Use libraries like Microsoft.AspNetCore.Authentication.OpenIdConnect
.
Pros:
- No need to manage passwords.
- Scalable for large applications. Cons: Relies on third-party services.
3. JWT (JSON Web Tokens)
What It Is: Tokens that securely transmit user information between parties.
Best For: APIs, microservices, or stateless applications.
Implementation: Generate tokens using System.IdentityModel.Tokens.Jwt
and validate them via middleware.
Pros:
- Lightweight and stateless.
- Works well with OAuth. Cons: Tokens must be securely stored and managed.
4. Windows Authentication
What It Is: Uses Active Directory to authenticate domain users.
Best For: Internal/intranet applications (e.g., corporate tools).
Pros:
- Seamless for Windows users.
- No login forms required. Cons: Limited to Windows environments.
5. Role-Based vs. Policy-Based Authorization
- Role-Based: Grants access based on user roles (e.g., "Admin" or "User").
[Authorize(Roles = "Admin")]
- Policy-Based: Uses flexible rules (e.g., "Over18" or "CanEditPosts").
services.AddAuthorization(options =>
options.AddPolicy("Over18", policy => policy.RequireClaim("Age", "18")));
Best For:
- Role-based: Simple role checks.
- Policy-based: Complex rules (e.g., combining claims, roles, or custom logic).
6. Third-Party Services (Auth0, Firebase, Okta)
What They Are: Managed solutions for authentication and authorization.
Best For: Apps needing quick setup or advanced features (e.g., multi-factor auth).
Pros:
- Reduce development time.
- Enterprise-grade security. Cons: Costs increase with user volume.
Choosing the Right Option
Scenario | Recommended Solution |
---|---|
Custom user database | ASP.NET Core Identity |
External logins (e.g., Google) | OAuth 2.0/OpenID Connect |
API/microservices | JWT |
Corporate intranet app | Windows Authentication |
Complex access rules | Policy-Based Authorization |
Rapid development | Third-party services (e.g., Auth0) |
Conclusion
The best choice depends on your application’s needs. For example:
- Use ASP.NET Core Identity for full control over user data.
- Opt for JWT + OAuth if building a decoupled API.
- Leverage third-party services to save time and ensure security.
Always prioritize security practices like HTTPS, token expiration, and input validation. By combining these tools, you can create a secure and scalable authentication system in your C# applications.