Style for control flow with validation checks
I find myself writing a lot of code like this: int myFunction(Person* person) { int personIsValid = !(person==NULL); if (personIsValid) { // do some stuff; might be lengthy int myresult = whatever; return myResult; } else { return -1; } } It can get pretty messy, especially if multiple checks are involved. In such cases, I've experimented with alternate styles, such as this one: int netWorth(Person* person) { if (Person==NULL) { return -1; } if (!(person->isAlive)) { return -1; } int assets = person->assets; if (assets==-1) { return -1; } int liabilities = person->liabilities; if (liabilities==-1) { return -1; } return assets - liabilities; } I'm interested in comments about the stylistic choices here. [Don't worry too much about the details of individual statements; it's the overall control flow that interests me.]
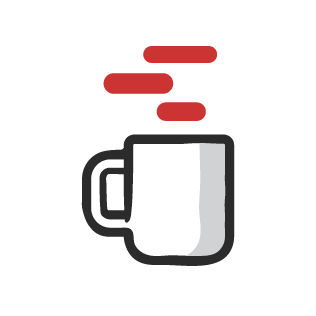
I find myself writing a lot of code like this:
int myFunction(Person* person) {
int personIsValid = !(person==NULL);
if (personIsValid) {
// do some stuff; might be lengthy
int myresult = whatever;
return myResult;
}
else {
return -1;
}
}
It can get pretty messy, especially if multiple checks are involved. In such cases, I've experimented with alternate styles, such as this one:
int netWorth(Person* person) {
if (Person==NULL) {
return -1;
}
if (!(person->isAlive)) {
return -1;
}
int assets = person->assets;
if (assets==-1) {
return -1;
}
int liabilities = person->liabilities;
if (liabilities==-1) {
return -1;
}
return assets - liabilities;
}
I'm interested in comments about the stylistic choices here. [Don't worry too much about the details of individual statements; it's the overall control flow that interests me.]