What Everyone’s Missing About React’s useDeferredValue Hook
Ever typed into a search bar in a React app, only to feel your keystrokes lag behind? Maybe you’ve built a feature where the UI needs to filter or display a large list of items in real time—and suddenly, everything feels slow and unresponsive. This isn’t just frustrating for users—it’s frustrating for developers. After all, React is known for being fast, right? Here’s the problem: React treats all updates as urgent by default, whether it’s a quick text input or a complex list render. That means when the app gets busy—especially with heavy components—the user experience starts to suffer. But here’s where the magic of modern React comes in. Back in 2022, React 18 introduced a new hook called useDeferredValue—a powerful tool designed to keep your app feeling responsive, even when it's doing heavy lifting behind the scenes. It doesn’t make rendering faster, but it makes the app feel faster by smartly deferring non-critical updates. In this post, we’re going to explore what most developers overlook about useDeferredValue, how it actually works, and how you can use it to smooth out your UI without rewriting your entire app. Let’s break it down. 1. It’s Not a Performance Booster—It’s a Perception Booster Let’s get this straight: It doesn’t make your app faster. It doesn’t reduce the rendering workload. It doesn’t skip any computations. What it does is make your app feel faster by allowing React to prioritize what’s most important: user input. Think of it like this: Instead of making the engine run faster, you’re just getting out of the driver’s way. Your app still processes that expensive re-render of the filtered list—but it waits until React has handled the urgent stuff, like the next character the user types. 2. It Defers Work—It Doesn’t Cancel It When you use useDeferredValue, you’re telling React: “Hey, this value matters—but don’t drop everything else to update it right now.” React takes that signal and delays rendering the dependent components until it finishes more urgent updates. Important: The work still happens. You’re not avoiding the cost—you’re rescheduling it. 3. It Improves Perceived Responsiveness—Not Frame Rates React doesn’t just aim for faster rendering anymore. It aims for smoother interaction. That’s where useDeferredValue shines. Even if your component still takes 300ms to render, the user won’t feel that delay—because their input remains crisp, immediate, and unblocked. This is a shift from technical performance to user experience performance. And it’s a big one. 4. Most Demos Are Too Small to Show Its True Power You’ll find countless tutorials showing useDeferredValue with a tiny search bar and a list of 50 items. Cool for learning? Sure. But in real apps? You want to use this when you're dealing with: 10,000+ items in a filterable catalog Real-time data dashboards Chart visualizations based on user input Live previews of markdown, code, or design elements That’s where this hook proves its value. 5. It’s Easy to Misuse and Misjudge Here’s a common story: A dev wraps a value with useDeferredValue Nothing changes They assume the hook is useless But what’s often missing is context: Are you rendering something expensive enough to matter? Are you using useMemo or React.memo to avoid unnecessary recalculations? Are you using ReactDOM.createRoot() to enable concurrent rendering? Without those, useDeferredValue won’t help—and it’s not the hook’s fault. Summary If your React app feels sluggish during user input—especially when rendering large lists or complex components—you’re not alone. That lag usually comes from treating all updates as urgent, even when they don’t need to be. React 18’s useDeferredValue hook is here to help. It doesn’t speed up rendering—it makes your app feel faster by deferring non-urgent updates until after the user interactions are handled. In this post, we covered: Why useDeferredValue boosts perceived responsiveness, not actual speed How it lets React prioritize user input while deferring expensive renders Common mistakes developers make when using it—and how to avoid them Real-world scenarios where this hook truly shines (hint: not just tiny to-do apps) If your UI feels slow under pressure, useDeferredValue might be the simplest way to deliver a smoother, snappier experience—without overhauling your entire app.
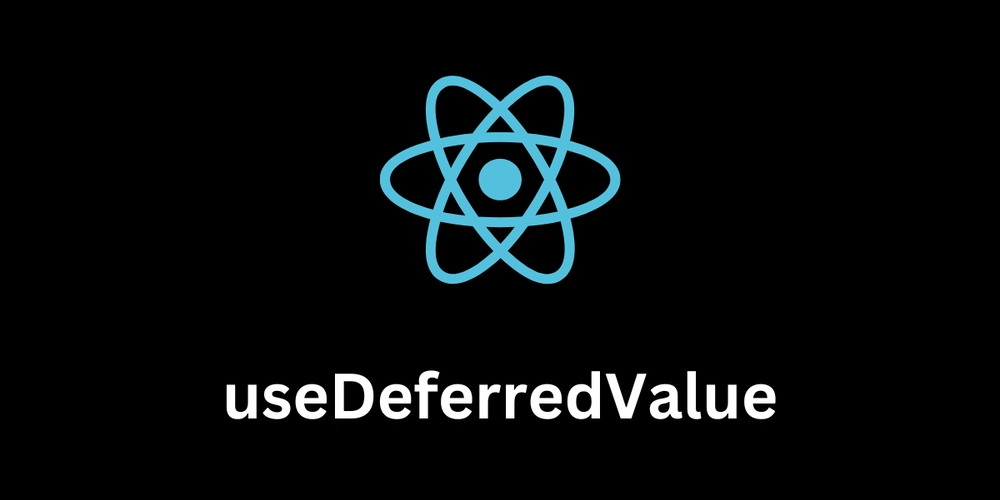
Ever typed into a search bar in a React app, only to feel your keystrokes lag behind? Maybe you’ve built a feature where the UI needs to filter or display a large list of items in real time—and suddenly, everything feels slow and unresponsive.
This isn’t just frustrating for users—it’s frustrating for developers. After all, React is known for being fast, right?
Here’s the problem: React treats all updates as urgent by default, whether it’s a quick text input or a complex list render. That means when the app gets busy—especially with heavy components—the user experience starts to suffer.
But here’s where the magic of modern React comes in.
Back in 2022, React 18 introduced a new hook called useDeferredValue—a powerful tool designed to keep your app feeling responsive, even when it's doing heavy lifting behind the scenes. It doesn’t make rendering faster, but it makes the app feel faster by smartly deferring non-critical updates.
In this post, we’re going to explore what most developers overlook about useDeferredValue, how it actually works, and how you can use it to smooth out your UI without rewriting your entire app.
Let’s break it down.
1. It’s Not a Performance Booster—It’s a Perception Booster
Let’s get this straight:
- It doesn’t make your app faster.
- It doesn’t reduce the rendering workload.
- It doesn’t skip any computations.
What it does is make your app feel faster by allowing React to prioritize what’s most important: user input.
Think of it like this:
Instead of making the engine run faster, you’re just getting out of the driver’s way.
Your app still processes that expensive re-render of the filtered list—but it waits until React has handled the urgent stuff, like the next character the user types.
2. It Defers Work—It Doesn’t Cancel It
When you use useDeferredValue, you’re telling React:
“Hey, this value matters—but don’t drop everything else to update it right now.”
React takes that signal and delays rendering the dependent components until it finishes more urgent updates.
Important:
The work still happens. You’re not avoiding the cost—you’re rescheduling it.
3. It Improves Perceived Responsiveness—Not Frame Rates
React doesn’t just aim for faster rendering anymore. It aims for smoother interaction.
That’s where useDeferredValue shines. Even if your component still takes 300ms to render, the user won’t feel that delay—because their input remains crisp, immediate, and unblocked.
This is a shift from technical performance to user experience performance. And it’s a big one.
4. Most Demos Are Too Small to Show Its True Power
You’ll find countless tutorials showing useDeferredValue with a tiny search bar and a list of 50 items. Cool for learning? Sure. But in real apps?
You want to use this when you're dealing with:
- 10,000+ items in a filterable catalog
- Real-time data dashboards
- Chart visualizations based on user input
- Live previews of markdown, code, or design elements
That’s where this hook proves its value.
5. It’s Easy to Misuse and Misjudge
Here’s a common story:
- A dev wraps a value with useDeferredValue
- Nothing changes
- They assume the hook is useless
But what’s often missing is context:
- Are you rendering something expensive enough to matter?
- Are you using useMemo or React.memo to avoid unnecessary recalculations?
- Are you using ReactDOM.createRoot() to enable concurrent rendering?
Without those, useDeferredValue won’t help—and it’s not the hook’s fault.
Summary
If your React app feels sluggish during user input—especially when rendering large lists or complex components—you’re not alone. That lag usually comes from treating all updates as urgent, even when they don’t need to be.
React 18’s useDeferredValue hook is here to help. It doesn’t speed up rendering—it makes your app feel faster by deferring non-urgent updates until after the user interactions are handled.
In this post, we covered:
- Why useDeferredValue boosts perceived responsiveness, not actual speed
- How it lets React prioritize user input while deferring expensive renders
- Common mistakes developers make when using it—and how to avoid them
- Real-world scenarios where this hook truly shines (hint: not just tiny to-do apps)
If your UI feels slow under pressure, useDeferredValue might be the simplest way to deliver a smoother, snappier experience—without overhauling your entire app.