Tutorial 4: Functions and Closures in Swift - Building a Fart Button App
In this tutorial, we’ll explore functions and closures in Swift by building a fun and simple app: The Fart Button! This app will play different fart sounds when tapped, showcasing how functions and closures make our code modular and reusable. 1. Understanding Functions in Swift A function in Swift is a reusable block of code that performs a specific task. It can take parameters, return values, and even be passed around like variables. Basic Function Syntax func sayHello() { print("Hello, Swift!") } sayHello() // Output: Hello, Swift! Functions with Parameters and Return Values func addNumbers(_ a: Int, _ b: Int) -> Int { return a + b } let sum = addNumbers(5, 10) print(sum) // Output: 15 2. Introducing Closures Closures in Swift are anonymous functions (functions without a name) that can be assigned to variables or passed as arguments. Basic Closure Syntax let greet = { (name: String) in print("Hello, \(name)!") } greet("Swift") // Output: Hello, Swift! Closures as Function Parameters Closures are often used in completion handlers and event-driven programming. func performAction(action: () -> Void) { action() } performAction { print("Closure executed!") } // Output: Closure executed! 3. Let's Build: The Fart Button App! Now that we understand functions and closures, let’s build a simple iOS app using Swift and SwiftUI that plays different fart sounds when tapped. Project Setup Open Xcode and create a new SwiftUI App. Name it FartButtonApp. Add fart sound files (e.g., fart1.mp3, fart2.mp3, fart3.mp3) to your project. Building the UI Modify ContentView.swift to add a Fart Button that randomly plays a fart sound when tapped. import SwiftUI import AVFoundation struct ContentView: View { let fartSounds = ["fart1", "fart2", "fart3"] var body: some View { VStack { Text("Press the button for an epic fart!") .font(.headline) .padding() Button(action: { playRandomFart() }) { Image(systemName: "speaker.wave.3.fill") .resizable() .frame(width: 100, height: 100) .foregroundColor(.brown) .padding() } } } func playRandomFart() { if let randomFart = fartSounds.randomElement() { playSound(named: randomFart) } } func playSound(named soundName: String) { guard let url = Bundle.main.url(forResource: soundName, withExtension: "mp3") else { return } var player: AVAudioPlayer? do { player = try AVAudioPlayer(contentsOf: url) player?.play() } catch { print("Error playing sound: \(error)") } } } @main struct FartButtonApp: App { var body: some Scene { WindowGroup { ContentView() } } } 4. Breaking It Down playRandomFart(): Uses the randomElement() function to pick a random fart sound. playSound(named:): Uses AVAudioPlayer to play the selected sound file. The button’s action calls playRandomFart(), demonstrating how functions keep code modular. Using Closures for More Fun Let’s refactor playRandomFart() using a closure: func playRandomFart() { fartSounds.randomElement().map { playSound(named: $0) } } This makes the function more concise and leverages Swift’s higher-order functions. 5. Summary Functions allow code reuse and modularity. Closures let us pass behavior as arguments, making code flexible. We built a Fart Button App using these concepts to play random fart sounds! Next Steps Add a slider to control fart volume. Implement a timer for delayed fart explosions. Use haptic feedback for a more immersive experience. That’s it! You’ve learned functions and closures while building a ridiculous but fun app.
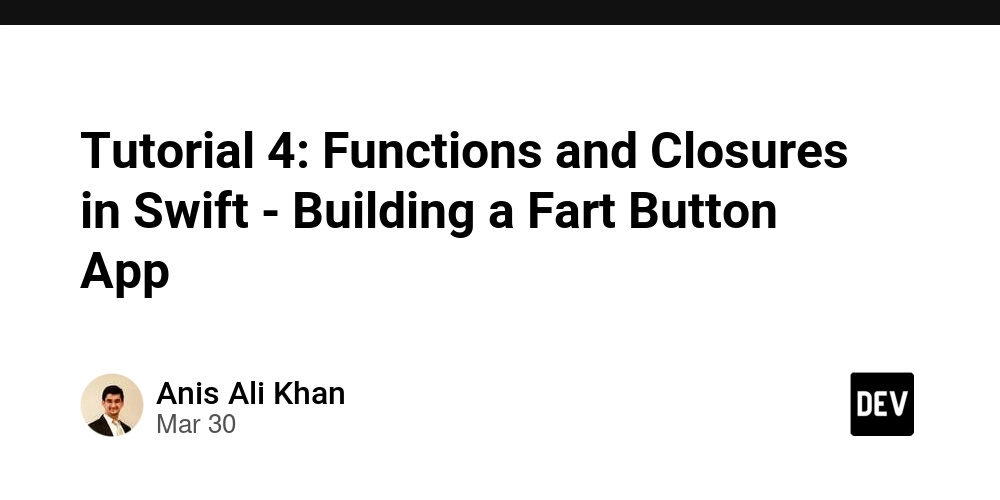
In this tutorial, we’ll explore functions and closures in Swift by building a fun and simple app: The Fart Button! This app will play different fart sounds when tapped, showcasing how functions and closures make our code modular and reusable.
1. Understanding Functions in Swift
A function in Swift is a reusable block of code that performs a specific task. It can take parameters, return values, and even be passed around like variables.
Basic Function Syntax
func sayHello() {
print("Hello, Swift!")
}
sayHello() // Output: Hello, Swift!
Functions with Parameters and Return Values
func addNumbers(_ a: Int, _ b: Int) -> Int {
return a + b
}
let sum = addNumbers(5, 10)
print(sum) // Output: 15
2. Introducing Closures
Closures in Swift are anonymous functions (functions without a name) that can be assigned to variables or passed as arguments.
Basic Closure Syntax
let greet = { (name: String) in
print("Hello, \(name)!")
}
greet("Swift") // Output: Hello, Swift!
Closures as Function Parameters
Closures are often used in completion handlers and event-driven programming.
func performAction(action: () -> Void) {
action()
}
performAction {
print("Closure executed!")
}
// Output: Closure executed!
3. Let's Build: The Fart Button App!
Now that we understand functions and closures, let’s build a simple iOS app using Swift and SwiftUI that plays different fart sounds when tapped.
Project Setup
- Open Xcode and create a new SwiftUI App.
- Name it FartButtonApp.
- Add fart sound files (e.g.,
fart1.mp3
,fart2.mp3
,fart3.mp3
) to your project.
Building the UI
Modify ContentView.swift
to add a Fart Button that randomly plays a fart sound when tapped.
import SwiftUI
import AVFoundation
struct ContentView: View {
let fartSounds = ["fart1", "fart2", "fart3"]
var body: some View {
VStack {
Text("Press the button for an epic fart!")
.font(.headline)
.padding()
Button(action: {
playRandomFart()
}) {
Image(systemName: "speaker.wave.3.fill")
.resizable()
.frame(width: 100, height: 100)
.foregroundColor(.brown)
.padding()
}
}
}
func playRandomFart() {
if let randomFart = fartSounds.randomElement() {
playSound(named: randomFart)
}
}
func playSound(named soundName: String) {
guard let url = Bundle.main.url(forResource: soundName, withExtension: "mp3") else { return }
var player: AVAudioPlayer?
do {
player = try AVAudioPlayer(contentsOf: url)
player?.play()
} catch {
print("Error playing sound: \(error)")
}
}
}
@main
struct FartButtonApp: App {
var body: some Scene {
WindowGroup {
ContentView()
}
}
}
4. Breaking It Down
-
playRandomFart()
: Uses therandomElement()
function to pick a random fart sound. -
playSound(named:)
: UsesAVAudioPlayer
to play the selected sound file. - The button’s action calls
playRandomFart()
, demonstrating how functions keep code modular.
Using Closures for More Fun
Let’s refactor playRandomFart()
using a closure:
func playRandomFart() {
fartSounds.randomElement().map { playSound(named: $0) }
}
This makes the function more concise and leverages Swift’s higher-order functions.
5. Summary
- Functions allow code reuse and modularity.
- Closures let us pass behavior as arguments, making code flexible.
- We built a Fart Button App using these concepts to play random fart sounds!
Next Steps
- Add a slider to control fart volume.
- Implement a timer for delayed fart explosions.
- Use haptic feedback for a more immersive experience.
That’s it! You’ve learned functions and closures while building a ridiculous but fun app.