Tutorial 21: Advanced Auto Layout Techniques for Responsive UIs
Table of Contents Introduction to Auto Layout Understanding Constraints and Safe Areas Adaptive Layout with Size Classes Dynamic Type and Accessibility Support Using Stack Views for Flexible Layouts Constraint Animations for Smooth UI Transitions Building an AR Depth Scanner with LiDAR and Auto Layout Testing and Debugging Auto Layout Issues Conclusion and Best Practices 1. Introduction to Auto Layout Auto Layout enables responsive UIs across different screen sizes by defining relationships between UI elements using constraints. Storyboard vs. Programmatic Auto Layout Storyboard: Visual approach, quick prototyping. Programmatic: More flexible, reusable components. let button = UIButton() button.translatesAutoresizingMaskIntoConstraints = false view.addSubview(button) NSLayoutConstraint.activate([ button.centerXAnchor.constraint(equalTo: view.centerXAnchor), button.centerYAnchor.constraint(equalTo: view.centerYAnchor), button.widthAnchor.constraint(equalToConstant: 200), button.heightAnchor.constraint(equalToConstant: 50) ]) 2. Understanding Constraints and Safe Areas Intrinsic Content Size: Determines UI element size. Priority & Compression Resistance: Manages element resizing. label.setContentHuggingPriority(.defaultHigh, for: .horizontal) label.setContentCompressionResistancePriority(.defaultLow, for: .horizontal) 3. Adaptive Layout with Size Classes Use size classes to differentiate between iPhone and iPad layouts. if traitCollection.horizontalSizeClass == .regular { print("Running on iPad or larger iPhone") } 4. Dynamic Type and Accessibility Support Use Dynamic Type for font scaling: label.font = UIFont.preferredFont(forTextStyle: .body) label.adjustsFontForContentSizeCategory = true 5. Using Stack Views for Flexible Layouts Stack Views automatically manage layout based on size constraints. let stackView = UIStackView(arrangedSubviews: [label, button]) stackView.axis = .vertical stackView.spacing = 10 stackView.distribution = .fillEqually view.addSubview(stackView) 6. Constraint Animations for Smooth UI Transitions Auto Layout constraints can be animated to create smooth transitions. UIView.animate(withDuration: 0.3) { self.buttonConstraint.constant += 50 self.view.layoutIfNeeded() } 7. Building an AR Depth Scanner with LiDAR and Auto Layout Why Use LiDAR? LiDAR (Light Detection and Ranging) is available on iPhone Pro models and enables accurate depth sensing. Setting Up an ARKit Project Create an ARKit App in Xcode. Add ARKit and RealityKit frameworks. import ARKit import UIKit class LiDARScannerViewController: UIViewController, ARSCNViewDelegate { let sceneView = ARSCNView() let depthLabel = UILabel() override func viewDidLoad() { super.viewDidLoad() setupSceneView() setupDepthLabel() } func setupSceneView() { sceneView.frame = view.bounds sceneView.delegate = self sceneView.session.run(ARWorldTrackingConfiguration()) view.addSubview(sceneView) } func setupDepthLabel() { depthLabel.translatesAutoresizingMaskIntoConstraints = false depthLabel.text = "Depth: 0m" depthLabel.textAlignment = .center view.addSubview(depthLabel) NSLayoutConstraint.activate([ depthLabel.centerXAnchor.constraint(equalTo: view.centerXAnchor), depthLabel.bottomAnchor.constraint(equalTo: view.safeAreaLayoutGuide.bottomAnchor, constant: -20) ]) } func renderer(_ renderer: SCNSceneRenderer, updateAtTime time: TimeInterval) { guard let frame = sceneView.session.currentFrame else { return } if let depthData = frame.sceneDepth?.depthMap { let avgDepth = depthData.mean() DispatchQueue.main.async { self.depthLabel.text = String(format: "Depth: %.2fm", avgDepth) } } } } extension CVPixelBuffer { func mean() -> Float { let width = CVPixelBufferGetWidth(self) let height = CVPixelBufferGetHeight(self) CVPixelBufferLockBaseAddress(self, .readOnly) let buffer = CVPixelBufferGetBaseAddress(self)! let sum = (0.. Float in let pixels = buffer.assumingMemoryBound(to: Float.self) return sum + pixels[row * width] } CVPixelBufferUnlockBaseAddress(self, .readOnly) return sum / Float(width * height) } } 8. Testing and Debugging Auto Layout Issues view.translatesAutoresizingMaskIntoConstraints = false NSLayoutConstraint.deactivate(view.constraints) 9. Conclusion and Best Practices Use Stack Views and Size Classes for flexible designs. Leverage Dynamic Type for accessibility. Animate Constraints for smooth UI transitions. Test with Xcode's Debugger for layout issues.
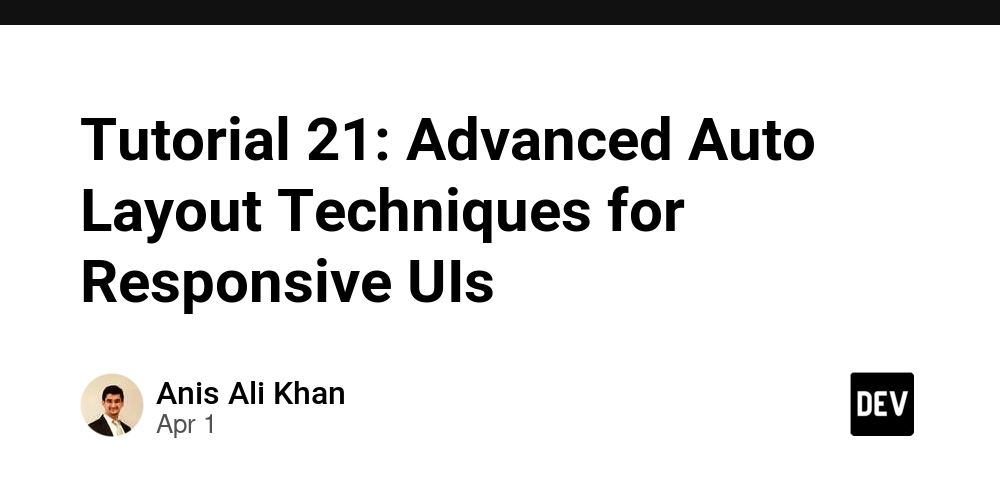
Table of Contents
- Introduction to Auto Layout
- Understanding Constraints and Safe Areas
- Adaptive Layout with Size Classes
- Dynamic Type and Accessibility Support
- Using Stack Views for Flexible Layouts
- Constraint Animations for Smooth UI Transitions
- Building an AR Depth Scanner with LiDAR and Auto Layout
- Testing and Debugging Auto Layout Issues
- Conclusion and Best Practices
1. Introduction to Auto Layout
Auto Layout enables responsive UIs across different screen sizes by defining relationships between UI elements using constraints.
Storyboard vs. Programmatic Auto Layout
- Storyboard: Visual approach, quick prototyping.
- Programmatic: More flexible, reusable components.
let button = UIButton()
button.translatesAutoresizingMaskIntoConstraints = false
view.addSubview(button)
NSLayoutConstraint.activate([
button.centerXAnchor.constraint(equalTo: view.centerXAnchor),
button.centerYAnchor.constraint(equalTo: view.centerYAnchor),
button.widthAnchor.constraint(equalToConstant: 200),
button.heightAnchor.constraint(equalToConstant: 50)
])
2. Understanding Constraints and Safe Areas
- Intrinsic Content Size: Determines UI element size.
- Priority & Compression Resistance: Manages element resizing.
label.setContentHuggingPriority(.defaultHigh, for: .horizontal)
label.setContentCompressionResistancePriority(.defaultLow, for: .horizontal)
3. Adaptive Layout with Size Classes
Use size classes to differentiate between iPhone and iPad layouts.
if traitCollection.horizontalSizeClass == .regular {
print("Running on iPad or larger iPhone")
}
4. Dynamic Type and Accessibility Support
Use Dynamic Type for font scaling:
label.font = UIFont.preferredFont(forTextStyle: .body)
label.adjustsFontForContentSizeCategory = true
5. Using Stack Views for Flexible Layouts
Stack Views automatically manage layout based on size constraints.
let stackView = UIStackView(arrangedSubviews: [label, button])
stackView.axis = .vertical
stackView.spacing = 10
stackView.distribution = .fillEqually
view.addSubview(stackView)
6. Constraint Animations for Smooth UI Transitions
Auto Layout constraints can be animated to create smooth transitions.
UIView.animate(withDuration: 0.3) {
self.buttonConstraint.constant += 50
self.view.layoutIfNeeded()
}
7. Building an AR Depth Scanner with LiDAR and Auto Layout
Why Use LiDAR?
LiDAR (Light Detection and Ranging) is available on iPhone Pro models and enables accurate depth sensing.
Setting Up an ARKit Project
- Create an ARKit App in Xcode.
- Add
ARKit
andRealityKit
frameworks.
import ARKit
import UIKit
class LiDARScannerViewController: UIViewController, ARSCNViewDelegate {
let sceneView = ARSCNView()
let depthLabel = UILabel()
override func viewDidLoad() {
super.viewDidLoad()
setupSceneView()
setupDepthLabel()
}
func setupSceneView() {
sceneView.frame = view.bounds
sceneView.delegate = self
sceneView.session.run(ARWorldTrackingConfiguration())
view.addSubview(sceneView)
}
func setupDepthLabel() {
depthLabel.translatesAutoresizingMaskIntoConstraints = false
depthLabel.text = "Depth: 0m"
depthLabel.textAlignment = .center
view.addSubview(depthLabel)
NSLayoutConstraint.activate([
depthLabel.centerXAnchor.constraint(equalTo: view.centerXAnchor),
depthLabel.bottomAnchor.constraint(equalTo: view.safeAreaLayoutGuide.bottomAnchor, constant: -20)
])
}
func renderer(_ renderer: SCNSceneRenderer, updateAtTime time: TimeInterval) {
guard let frame = sceneView.session.currentFrame else { return }
if let depthData = frame.sceneDepth?.depthMap {
let avgDepth = depthData.mean()
DispatchQueue.main.async {
self.depthLabel.text = String(format: "Depth: %.2fm", avgDepth)
}
}
}
}
extension CVPixelBuffer {
func mean() -> Float {
let width = CVPixelBufferGetWidth(self)
let height = CVPixelBufferGetHeight(self)
CVPixelBufferLockBaseAddress(self, .readOnly)
let buffer = CVPixelBufferGetBaseAddress(self)!
let sum = (0..<height).reduce(0.0) { (sum, row) -> Float in
let pixels = buffer.assumingMemoryBound(to: Float.self)
return sum + pixels[row * width]
}
CVPixelBufferUnlockBaseAddress(self, .readOnly)
return sum / Float(width * height)
}
}
8. Testing and Debugging Auto Layout Issues
view.translatesAutoresizingMaskIntoConstraints = false
NSLayoutConstraint.deactivate(view.constraints)
9. Conclusion and Best Practices
- Use Stack Views and Size Classes for flexible designs.
- Leverage Dynamic Type for accessibility.
- Animate Constraints for smooth UI transitions.
- Test with Xcode's Debugger for layout issues.