TS2306: File '{0}' is not a module
TS2306: File '{0}' is not a module TypeScript is a typed superset of JavaScript, adding optional static typing and extensive tooling capabilities to the base language. This means TypeScript builds on top of JavaScript, providing features such as type definitions, interfaces, enums, and more, to help developers catch errors early and write safer, more maintainable code. Types in TypeScript allow us to define the shape of data and ensure that we are using it correctly across our applications. For example, a variable can be typed to hold only a string, number, or even a more complex object, reducing runtime issues. If you're new to TypeScript or want to further explore its features, consider subscribing to blogs like this one or trying AI tools such as GPTeach to learn how to code. What Are Types in TypeScript? Types are a way to define the shape or structure of data in TypeScript. They allow developers to specify what kind of data should be passed around in their code. For example: let age: number = 25; // age must hold numbers let name: string = "John"; // name must hold strings By enabling strong typing, TypeScript catches mistakes at compile time, significantly reducing bugs. For more advanced scenarios, we can define custom types like objects, unions, arrays, etc., to make the structure of our code explicit and predictable: type User = { name: string; age: number; isAdmin: boolean; }; const user: User = { name: "Alice", age: 30, isAdmin: true, }; By enforcing types, TypeScript ensures consistency in how we handle and use data. Now let’s move on to the main subject of this article: TS2306: File '{0}' is not a module. This error can sometimes appear when working with TypeScript, and understanding the cause is essential for debugging it properly. Understanding TS2306: File '{0}' is not a module When the TypeScript compiler encounters the error TS2306: File '{0}' is not a module, it generally means that you're attempting to import a file as if it were a module, but TypeScript cannot recognize it as such. This issue often arises due to incorrectly defined TypeScript types or missing export declarations in the file you’re trying to import. Let’s analyze this error more deeply and walk through its behavior step by step. When Does the TS2306: File '{0}' is not a module Error Happen? Here’s a common scenario that can trigger this error: // utils.ts function add(a: number, b: number): number { return a + b; } If you try to import this function without explicitly exporting it: // app.ts import { add } from "./utils"; You’ll see the error TS2306: File './utils' is not a module, because the file utils.ts doesn’t actually export anything. TypeScript doesn’t recognize it as a module. How to Fix TS2306: File '{0}' is not a module? There are several ways to resolve this error depending on the root cause. Let’s go through some of them: 1. Add an Export to the File If the file you’re trying to import from doesn’t have an export, add one: // utils.ts export function add(a: number, b: number): number { return a + b; } Now, the module can be safely imported: // app.ts import { add } from "./utils"; console.log(add(2, 3)); Important to know! In TypeScript, every file that has at least one export or import is considered a module. Files without these keywords are treated as scripts, and you cannot import them. 2. Ensure Correct Export Syntax Using incorrect export syntax can also cause TS2306: File '{0}' is not a module. If you’re using a default export, ensure you import it correctly: // utils.ts export default function add(a: number, b: number): number { return a + b; } Correct usage: // app.ts import add from "./utils"; console.log(add(2, 3)); Incorrect usage (triggers TS2306): // app.ts import { add } from "./utils"; // Incorrect when using default export 3. Check Type Definition Files (.d.ts) When working with third-party libraries or external files, this error might also occur if the library doesn’t have proper type definitions. You’ll need to ensure you have the required @types package installed or manually define the module in a .d.ts file: // types.d.ts declare module "some-library" { export function someFunction(): void; } Important to know! If you’re working with plain JavaScript files in a TypeScript project, you might face this error because TypeScript doesn't automatically infer types or module definitions for .js files. Use declare module in these cases to define the module manually. FAQs About TS2306: File '{0}' is not a module 1. Can I import from a file without export in TypeScript? No. In TypeScript, modules must export something for them to be imported. Otherwise, you’ll see the error TS2306: File '{0}' is not a module. Add an export to make the file a val
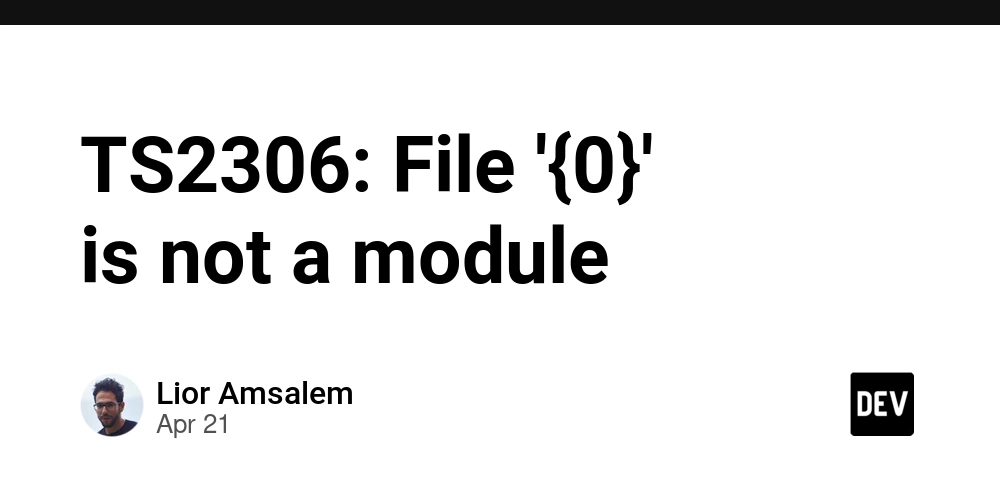
TS2306: File '{0}' is not a module
TypeScript is a typed superset of JavaScript, adding optional static typing and extensive tooling capabilities to the base language. This means TypeScript builds on top of JavaScript, providing features such as type definitions, interfaces, enums, and more, to help developers catch errors early and write safer, more maintainable code. Types in TypeScript allow us to define the shape of data and ensure that we are using it correctly across our applications. For example, a variable can be typed to hold only a string
, number
, or even a more complex object, reducing runtime issues.
If you're new to TypeScript or want to further explore its features, consider subscribing to blogs like this one or trying AI tools such as GPTeach to learn how to code.
What Are Types in TypeScript?
Types are a way to define the shape or structure of data in TypeScript. They allow developers to specify what kind of data should be passed around in their code. For example:
let age: number = 25; // age must hold numbers
let name: string = "John"; // name must hold strings
By enabling strong typing, TypeScript catches mistakes at compile time, significantly reducing bugs.
For more advanced scenarios, we can define custom types like objects, unions, arrays, etc., to make the structure of our code explicit and predictable:
type User = {
name: string;
age: number;
isAdmin: boolean;
};
const user: User = {
name: "Alice",
age: 30,
isAdmin: true,
};
By enforcing types, TypeScript ensures consistency in how we handle and use data.
Now let’s move on to the main subject of this article: TS2306: File '{0}' is not a module. This error can sometimes appear when working with TypeScript, and understanding the cause is essential for debugging it properly.
Understanding TS2306: File '{0}' is not a module
When the TypeScript compiler encounters the error TS2306: File '{0}' is not a module, it generally means that you're attempting to import a file as if it were a module, but TypeScript cannot recognize it as such. This issue often arises due to incorrectly defined TypeScript types or missing export declarations in the file you’re trying to import.
Let’s analyze this error more deeply and walk through its behavior step by step.
When Does the TS2306: File '{0}' is not a module Error Happen?
Here’s a common scenario that can trigger this error:
// utils.ts
function add(a: number, b: number): number {
return a + b;
}
If you try to import this function without explicitly exporting it:
// app.ts
import { add } from "./utils";
You’ll see the error TS2306: File './utils' is not a module, because the file utils.ts
doesn’t actually export anything. TypeScript doesn’t recognize it as a module.
How to Fix TS2306: File '{0}' is not a module?
There are several ways to resolve this error depending on the root cause. Let’s go through some of them:
1. Add an Export to the File
If the file you’re trying to import from doesn’t have an export
, add one:
// utils.ts
export function add(a: number, b: number): number {
return a + b;
}
Now, the module can be safely imported:
// app.ts
import { add } from "./utils";
console.log(add(2, 3));
Important to know!
In TypeScript, every file that has at least one export
or import
is considered a module. Files without these keywords are treated as scripts, and you cannot import them.
2. Ensure Correct Export Syntax
Using incorrect export syntax can also cause TS2306: File '{0}' is not a module. If you’re using a default
export, ensure you import it correctly:
// utils.ts
export default function add(a: number, b: number): number {
return a + b;
}
Correct usage:
// app.ts
import add from "./utils";
console.log(add(2, 3));
Incorrect usage (triggers TS2306):
// app.ts
import { add } from "./utils"; // Incorrect when using default export
3. Check Type Definition Files (.d.ts
)
When working with third-party libraries or external files, this error might also occur if the library doesn’t have proper type definitions. You’ll need to ensure you have the required @types
package installed or manually define the module in a .d.ts
file:
// types.d.ts
declare module "some-library" {
export function someFunction(): void;
}
Important to know!
If you’re working with plain JavaScript files in a TypeScript project, you might face this error because TypeScript doesn't automatically infer types or module definitions for .js
files. Use declare module
in these cases to define the module manually.
FAQs About TS2306: File '{0}' is not a module
1. Can I import from a file without export
in TypeScript?
No. In TypeScript, modules must export something for them to be imported. Otherwise, you’ll see the error TS2306: File '{0}' is not a module. Add an export
to make the file a valid module.
2. Why am I getting this error with a third-party library?
This usually occurs because the library is missing type definitions. Install the necessary @types
package or define the module manually in a .d.ts
file.
3. Does this error only occur in TypeScript projects?
Yes, TS2306: File '{0}' is not a module is specific to TypeScript. JavaScript’s behavior regarding imports is less strict, so this error doesn’t apply there.
Key Points to Remember
Here’s a quick checklist to avoid and resolve the TS2306: File '{0}' is not a module error:
-
Ensure files have an
export
: A file withoutexport
is not considered a module. - Use correct import/export syntax: Match the import style based on whether the file uses named or default exports.
- Verify type definitions: For external libraries, make sure the necessary types are installed or defined.
-
Check for
.d.ts
files: Manually define missing modules for untyped JavaScript libraries if needed.
By following these steps, you should be able to resolve this error and ensure smooth TypeScript development.
In conclusion, TS2306: File '{0}' is not a module is an error that primarily stems from type definition or module export issues, but it’s easy to fix once you identify the root cause. Understanding how modules work in TypeScript and following best practices in export/import syntax can save you a lot of debugging time in the long run.