TS2308: Module {0} has already exported a member named '{1}'. Consider explicitly re-exporting to resolve the ambiguity
TS2308: Module {0} has already exported a member named '{1}'. Consider explicitly re-exporting to resolve the ambiguity Introduction to TypeScript and Types TypeScript is a strongly typed programming language that builds on JavaScript, adding static type definitions. Essentially, it acts as a superset of JavaScript, meaning that any valid JavaScript code is also valid TypeScript code, but TypeScript adds additional features (such as static type-checking, interfaces, and more). The primary goal of TypeScript is to catch errors at compile time, saving developers the hassle of runtime errors that are harder to debug. One cornerstone of TypeScript is types. A "type" is a way to tell TypeScript what kind of data a variable, function, or object is expected to handle. This could be something as simple as ensuring a string variable isn't accidentally treated as a number, or as complex as defining custom structures for objects. Here's a simple type example in TypeScript: function add(x: number, y: number): number { return x + y; } // Error: Argument of type 'string' is not assignable to parameter of type 'number' add(5, "text"); In the above code, TypeScript ensures that only numbers are passed to the add function, and will throw an error at compile time if the types don't match. This kind of enforcement is what makes TypeScript appealing for larger, scalable projects. If you're interested in learning more about TypeScript or using AI tools like GPTTeach to enhance your coding skills, subscribe to my blog for detailed guides. What is a Superset Language? TypeScript being called a superset of JavaScript means that all JavaScript features are available in TypeScript, but additional functionality is layered on top. Imagine it as an enhanced version of JavaScript that provides extra tools and safety. For instance, you can still write JavaScript code and gradually adopt TypeScript's features like types, enums, interfaces, etc. Example of JavaScript in TypeScript: // This code is valid JavaScript and TypeScript const greet = (name) => { console.log(`Hello ${name}`); }; greet("TypeScript"); Example of enhanced TypeScript functionality: // Now using type annotations in TypeScript const greet = (name: string): void => { console.log(`Hello ${name}`); }; // Type checking will prevent this error greet(123); // Error: Argument of type 'number' is not assignable to parameter of type 'string' The superset structure allows developers to adopt the parts of TypeScript they need without losing the ability to run their JavaScript code. Explaining the Error: TS2308: Module {0} has already exported a member named '{1}'. Consider explicitly re-exporting to resolve the ambiguity When working with TypeScript, you might encounter the error TS2308: Module {0} has already exported a member named '{1}'. Consider explicitly re-exporting to resolve the ambiguity. This error essentially means that you've defined or imported two or more members in a module (file), but their names conflict or overlap. TypeScript cannot resolve this conflict on its own and requires the developer to handle it explicitly. Let's break it down in simpler terms: "Module {0}" refers to the file causing the problem. The "member named '{1}'" refers to the specific export that's being duplicated. "Consider explicitly re-exporting" suggests that you solve the issue by restructuring or renaming your exports, so that TypeScript can clearly understand what you intend. In essence, this error often occurs when duplicate names are being exported or when imports have naming conflicts between modules. A Code Example That Causes TS2308 Imagine you have two files: moduleA.ts and moduleB.ts. Each of them exports an identical member called doSomething. moduleA.ts export function doSomething() { console.log("Module A - Doing something"); } moduleB.ts export function doSomething() { console.log("Module B - Doing something else"); } Now, in a third file, main.ts, you try to import both modules and directly re-export their members: main.ts export * from './moduleA'; export * from './moduleB'; TypeScript will throw TS2308: Module {0} has already exported a member named '{1}'. Consider explicitly re-exporting to resolve the ambiguity, because both moduleA and moduleB export a member named doSomething, and TypeScript doesn't know which one to use. How to Fix TS2308 There are several ways to fix this issue: 1. Explicit Re-Exports With Aliases You can rename the conflicting exports using aliases (a new name assigned to the export): export { doSomething as doSomethingFromA } from './moduleA'; export { doSomething as doSomethingFromB } from './moduleB'; Now, in other parts of your code, you can use these new names to avoid conflicts: import { doSomethingFromA, doSomethingFromB } from '
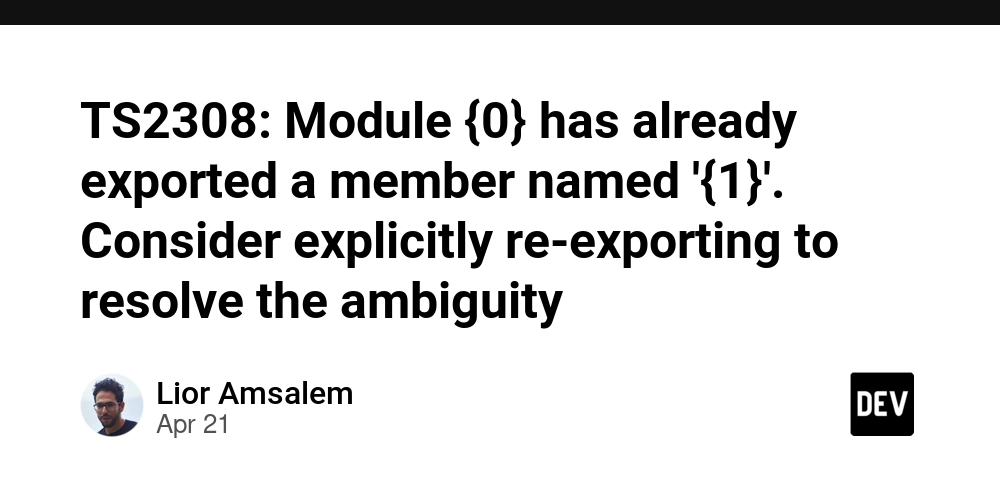
TS2308: Module {0} has already exported a member named '{1}'. Consider explicitly re-exporting to resolve the ambiguity
Introduction to TypeScript and Types
TypeScript is a strongly typed programming language that builds on JavaScript, adding static type definitions. Essentially, it acts as a superset of JavaScript, meaning that any valid JavaScript code is also valid TypeScript code, but TypeScript adds additional features (such as static type-checking, interfaces, and more). The primary goal of TypeScript is to catch errors at compile time, saving developers the hassle of runtime errors that are harder to debug.
One cornerstone of TypeScript is types. A "type" is a way to tell TypeScript what kind of data a variable, function, or object is expected to handle. This could be something as simple as ensuring a string variable isn't accidentally treated as a number, or as complex as defining custom structures for objects.
Here's a simple type example in TypeScript:
function add(x: number, y: number): number {
return x + y;
}
// Error: Argument of type 'string' is not assignable to parameter of type 'number'
add(5, "text");
In the above code, TypeScript ensures that only numbers are passed to the add
function, and will throw an error at compile time if the types don't match. This kind of enforcement is what makes TypeScript appealing for larger, scalable projects. If you're interested in learning more about TypeScript or using AI tools like GPTTeach to enhance your coding skills, subscribe to my blog for detailed guides.
What is a Superset Language?
TypeScript being called a superset of JavaScript means that all JavaScript features are available in TypeScript, but additional functionality is layered on top. Imagine it as an enhanced version of JavaScript that provides extra tools and safety. For instance, you can still write JavaScript code and gradually adopt TypeScript's features like types, enums, interfaces, etc.
Example of JavaScript in TypeScript:
// This code is valid JavaScript and TypeScript
const greet = (name) => {
console.log(`Hello ${name}`);
};
greet("TypeScript");
Example of enhanced TypeScript functionality:
// Now using type annotations in TypeScript
const greet = (name: string): void => {
console.log(`Hello ${name}`);
};
// Type checking will prevent this error
greet(123); // Error: Argument of type 'number' is not assignable to parameter of type 'string'
The superset structure allows developers to adopt the parts of TypeScript they need without losing the ability to run their JavaScript code.
Explaining the Error: TS2308: Module {0} has already exported a member named '{1}'. Consider explicitly re-exporting to resolve the ambiguity
When working with TypeScript, you might encounter the error TS2308: Module {0} has already exported a member named '{1}'. Consider explicitly re-exporting to resolve the ambiguity. This error essentially means that you've defined or imported two or more members in a module (file), but their names conflict or overlap. TypeScript cannot resolve this conflict on its own and requires the developer to handle it explicitly.
Let's break it down in simpler terms:
- "Module {0}" refers to the file causing the problem.
- The "member named '{1}'" refers to the specific export that's being duplicated.
- "Consider explicitly re-exporting" suggests that you solve the issue by restructuring or renaming your exports, so that TypeScript can clearly understand what you intend.
In essence, this error often occurs when duplicate names are being exported or when imports have naming conflicts between modules.
A Code Example That Causes TS2308
Imagine you have two files: moduleA.ts
and moduleB.ts
. Each of them exports an identical member called doSomething
.
moduleA.ts
export function doSomething() {
console.log("Module A - Doing something");
}
moduleB.ts
export function doSomething() {
console.log("Module B - Doing something else");
}
Now, in a third file, main.ts
, you try to import both modules and directly re-export their members:
main.ts
export * from './moduleA';
export * from './moduleB';
TypeScript will throw TS2308: Module {0} has already exported a member named '{1}'. Consider explicitly re-exporting to resolve the ambiguity, because both moduleA
and moduleB
export a member named doSomething
, and TypeScript doesn't know which one to use.
How to Fix TS2308
There are several ways to fix this issue:
1. Explicit Re-Exports With Aliases
You can rename the conflicting exports using aliases (a new name assigned to the export):
export { doSomething as doSomethingFromA } from './moduleA';
export { doSomething as doSomethingFromB } from './moduleB';
Now, in other parts of your code, you can use these new names to avoid conflicts:
import { doSomethingFromA, doSomethingFromB } from './main';
doSomethingFromA(); // Outputs: "Module A - Doing something"
doSomethingFromB(); // Outputs: "Module B - Doing something else"
2. Export Individual Members
Instead of re-exporting everything (export *
), explicitly export only specific members to avoid conflicts.
export { doSomething } from './moduleA'; // Only export this from moduleA
// Do not re-export 'doSomething' from moduleB to avoid ambiguity
3. Combine and Rename Duplicates
If the functionalities are related, you might consider merging the two into a new function or object and exporting that:
import { doSomething as doSomethingA } from './moduleA';
import { doSomething as doSomethingB } from './moduleB';
export const doSomething = () => {
doSomethingA();
doSomethingB();
};
Important to Know!
Always use explicit imports and exports when working with multiple contributors or third-party libraries in large projects. This makes your code easier to debug and reduces chances of namespace conflicts.
If the error TS2308: Module {0} has already exported a member named '{1}'. Consider explicitly re-exporting to resolve the ambiguity surfaces, check for
export *
statements first, as they’re the most common cause of ambiguity.Refactoring file organization can often prevent issues like TS2308. Keep your modules focused and stick to single-purpose exports where possible.
Frequently Asked Questions (FAQs)
1. What does "explicitly re-exporting" mean?
Explicit re-exporting refers to renaming, restructuring, or individually selecting what to export from a module to prevent conflicts or ambiguity.
Example:
export { memberName as aliasName } from './module';
2. Why doesn’t TypeScript handle duplicate exports automatically?
TypeScript cannot resolve naming conflicts reliably because it doesn't know the intent of the programmer. For clarity and safety, you must resolve conflicts manually.
3. Is using export *
a bad practice?
Not necessarily, but it’s risky when dealing with modules that may have duplicate export names. It’s better to explicitly define exports when possible in larger codebases.
By understanding errors like TS2308: Module {0} has already exported a member named '{1}'. Consider explicitly re-exporting to resolve the ambiguity, you’ll save yourself from many hours of debugging in your TypeScript journey. Keep practicing and refining your TypeScript skills!