TS2305: Module 0 has no exported member 1
TS2305: Module '{0}' has no exported member '{1}' TypeScript is a statically typed superset of JavaScript, and it provides features like type definitions, enums, interfaces, and more. Unlike JavaScript, TypeScript enforces system-wide type safety, which makes it easier to write, debug, and maintain large-scale projects. At its core, TypeScript allows developers to define data types during development, reducing runtime errors and improving code readability. If you're eager to learn TypeScript or enhance your coding skills using AI tools, consider subscribing to our blog or exploring resources like GPTeach. It's a great way to accelerate your learning process! In this article, we will explore one of the common issues in TypeScript, the error TS2305: Module '{0}' has no exported member '{1}'. But first, let's discuss what types are to give you a foundation for understanding this kind of error. What Are Types in TypeScript? In TypeScript, types define the shape and behavior of data. Whether you're working with variables, functions, or objects, assigning a type ensures that the data fits the contract you've defined. Common types in TypeScript include string, number, boolean, array, object, and any. For example: let firstName: string = "John"; let age: number = 30; let isAdmin: boolean = true; Here, the firstName variable can only hold a string, age can only hold a number, and isAdmin can only hold a boolean. TypeScript prevents assigning a value of the wrong type, such as assigning a number to the firstName field. TS2305: Module '{0}' has no exported member '{1}' Now let’s break down the error TS2305: Module '{0}' has no exported member '{1}'. This error occurs when you try to import something that hasn’t been exported from a module. It usually means that the name you’re trying to import doesn’t exist in the source file or the package you're importing from. Understanding the Error: Modules in TypeScript (and JavaScript) allow us to split our code into smaller, reusable files. When a module exports a member (like a function, class, interface, or type), it's then available for other files to use. If you try to import something that the module doesn’t actually export, TypeScript throws the TS2305 error. Here’s what the error might look like: error TS2305: Module './utilities' has no exported member 'calculateSum'. This means that the module ./utilities doesn’t have an exported member named calculateSum. This might happen for reasons like misspelling, incorrect export statements, or wrong file paths. Example of Code That Causes the Error: // mathUtils.ts export function add(a: number, b: number): number { return a + b; } // main.ts import { calculateSum } from './mathUtils'; const result = calculateSum(5, 10); // Error: TS2305 console.log(result); In the above example, the error occurs because calculateSum is not defined or exported from mathUtils.ts. The actual exported member is add, but the code tries to import a non-existent function, causing TS2305: Module '{0}' has no exported member '{1}'. How to Fix TS2305 Here are the steps to resolve the error: Verify the Exported Members: Open the module file and check what members it exports. Ensure the name matches exactly. // mathUtils.ts export function add(a: number, b: number): number { return a + b; } Correct the Import Statement: Ensure that you’re importing a member that actually exists. Fix the incorrect import. import { add } from './mathUtils'; const result = add(5, 10); console.log(result); Check Module File Paths: Verify that the module path is accurate. Ensure the file is referenced correctly (./mathUtils in this case). Use Wildcard Imports as a Backup: If multiple exports exist, you can use a wildcard import. This allows you to access all members from a module in one go. import * as MathUtils from './mathUtils'; const result = MathUtils.add(5, 10); console.log(result); Important to Know! TypeScript is case-sensitive. Make sure the case (uppercase/lowercase characters) for imported members exactly matches their export. Wildcard imports (* as) can lead to cleaner imports if a module contains multiple exports. FAQs Q: What can cause TS2305 errors when you're confident about the exported member? Misspelled or mismatched import names. Incorrect module paths. The module you're importing may not have been compiled correctly. Q: Can TS2305 occur with third-party libraries? Yes, if the installed library version differs or if you import a non-existing member from the library (e.g., calling import { X } from "library" when "library" only exports Y). Q: How can I avoid these issues in larger projects? Use editor tools or IDEs with TypeScript support to auto-suggest the correct export names. Regularly review your file and mo
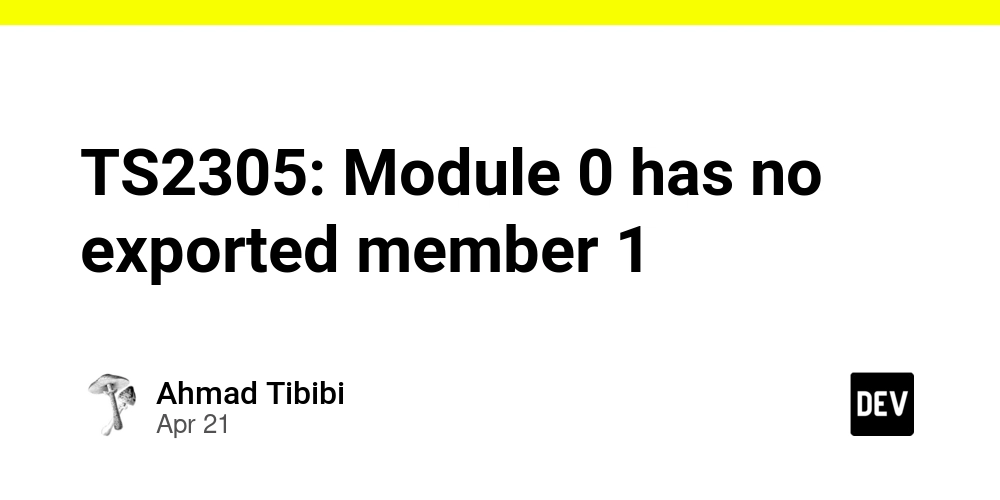
TS2305: Module '{0}' has no exported member '{1}'
TypeScript is a statically typed superset of JavaScript, and it provides features like type definitions, enums, interfaces, and more. Unlike JavaScript, TypeScript enforces system-wide type safety, which makes it easier to write, debug, and maintain large-scale projects. At its core, TypeScript allows developers to define data types during development, reducing runtime errors and improving code readability.
If you're eager to learn TypeScript or enhance your coding skills using AI tools, consider subscribing to our blog or exploring resources like GPTeach. It's a great way to accelerate your learning process!
In this article, we will explore one of the common issues in TypeScript, the error TS2305: Module '{0}' has no exported member '{1}'. But first, let's discuss what types are to give you a foundation for understanding this kind of error.
What Are Types in TypeScript?
In TypeScript, types define the shape and behavior of data. Whether you're working with variables, functions, or objects, assigning a type ensures that the data fits the contract you've defined. Common types in TypeScript include string
, number
, boolean
, array
, object
, and any
.
For example:
let firstName: string = "John";
let age: number = 30;
let isAdmin: boolean = true;
Here, the firstName
variable can only hold a string, age
can only hold a number, and isAdmin
can only hold a boolean. TypeScript prevents assigning a value of the wrong type, such as assigning a number to the firstName
field.
TS2305: Module '{0}' has no exported member '{1}'
Now let’s break down the error TS2305: Module '{0}' has no exported member '{1}'. This error occurs when you try to import something that hasn’t been exported from a module. It usually means that the name you’re trying to import doesn’t exist in the source file or the package you're importing from.
Understanding the Error:
Modules in TypeScript (and JavaScript) allow us to split our code into smaller, reusable files. When a module exports a member (like a function, class, interface, or type), it's then available for other files to use. If you try to import something that the module doesn’t actually export, TypeScript throws the TS2305
error.
Here’s what the error might look like:
error TS2305: Module './utilities' has no exported member 'calculateSum'.
This means that the module ./utilities
doesn’t have an exported member named calculateSum
. This might happen for reasons like misspelling, incorrect export statements, or wrong file paths.
Example of Code That Causes the Error:
// mathUtils.ts
export function add(a: number, b: number): number {
return a + b;
}
// main.ts
import { calculateSum } from './mathUtils';
const result = calculateSum(5, 10); // Error: TS2305
console.log(result);
In the above example, the error occurs because calculateSum
is not defined or exported from mathUtils.ts
. The actual exported member is add
, but the code tries to import a non-existent function, causing TS2305: Module '{0}' has no exported member '{1}'.
How to Fix TS2305
Here are the steps to resolve the error:
- Verify the Exported Members: Open the module file and check what members it exports. Ensure the name matches exactly.
// mathUtils.ts
export function add(a: number, b: number): number {
return a + b;
}
- Correct the Import Statement: Ensure that you’re importing a member that actually exists. Fix the incorrect import.
import { add } from './mathUtils';
const result = add(5, 10);
console.log(result);
Check Module File Paths:
Verify that the module path is accurate. Ensure the file is referenced correctly (./mathUtils
in this case).Use Wildcard Imports as a Backup:
If multiple exports exist, you can use a wildcard import. This allows you to access all members from a module in one go.
import * as MathUtils from './mathUtils';
const result = MathUtils.add(5, 10);
console.log(result);
Important to Know!
- TypeScript is case-sensitive. Make sure the case (uppercase/lowercase characters) for imported members exactly matches their export.
- Wildcard imports (
* as
) can lead to cleaner imports if a module contains multiple exports.
FAQs
Q: What can cause TS2305 errors when you're confident about the exported member?
- Misspelled or mismatched import names.
- Incorrect module paths.
- The module you're importing may not have been compiled correctly.
Q: Can TS2305 occur with third-party libraries?
Yes, if the installed library version differs or if you import a non-existing member from the library (e.g., calling import { X } from "library"
when "library"
only exports Y
).
Q: How can I avoid these issues in larger projects?
- Use editor tools or IDEs with TypeScript support to auto-suggest the correct export names.
- Regularly review your file and module structures.
Important to Know!
- When using third-party libraries, always check their documentation to see what they export.
- Type aliases, interfaces, and enums must all be explicitly exported if they’re intended to be imported into other files.
Summary of Important Things to Know:
-
TS2305: Module '{0}' has no exported member '{1}'
occurs because you're trying to import something that doesn’t exist in a module. - Always double-check the export statements in your module file.
- TypeScript will enforce strict checks, so small mistakes like typos or mismatched cases can lead to errors.
- Always ensure that your imports match exactly with the exported members.
With these guidelines, you should be able to fix and avoid the TS2305: Module '{0}' has no exported member '{1}' error in your TypeScript projects. Happy coding!