1. What is API Design? An API (Application Programming Interface) allows two software systems to communicate with each other. API Design means structuring the way clients (apps, users, services) request and receive data. Good API design is essential for scalability, usability, security, and future growth. 2. Fundamental Principles of API Design "Good API design follows simplicity, clarity, consistency, and predictability." The four sacred principles: Simplicity: Keep APIs intuitive and minimal. Consistency: Maintain uniform naming, patterns, and conventions. Clarity: Make APIs readable and self-explanatory. Predictability: Clients should predict behavior based on structure. 3. Understanding REST Principles (Core Foundations) REST (Representational State Transfer) is the most common architecture for modern APIs. It relies on standard HTTP methods to interact with resources. Key Concepts: Term Meaning Resource Object (e.g., User, Order, Product) Endpoint URL where the resource can be accessed HTTP Methods GET, POST, PUT, PATCH, DELETE Stateless Each request must contain all the needed info Common REST HTTP Methods: Method Purpose GET Retrieve data POST Create a new resource PUT Update (replace) an existing resource PATCH Update (partial) a resource DELETE Remove a resource Tip: APIs should manipulate resources, not actions. Bad Example: POST /createUser Good Example: POST /users 4. API Versioning (Prepare for the Future) Never design an API without planning versioning. It allows you to update APIs without breaking existing clients. Common Versioning Strategies: Strategy Example URL versioning GET /api/v1/users Header versioning Accept: application/vnd.api.v1+json Query parameter /api/users?version=1 Best Practice: Use URL versioning. It is clear, simple, and cache-friendly. 5. URL Design Best Practices (Clear and Consistent) Your URL should speak clearly what it serves. Rules: Use nouns, not verbs: /users, /orders Use plural names: /products not /product Nest logically: /users/{userId}/orders Use hyphens not underscores: /user-profile Keep URLs lowercase Examples: Purpose URL List all users GET /api/v1/users Retrieve a user GET /api/v1/users/{userId} Create new user POST /api/v1/users Update user PUT /api/v1/users/{userId} Delete user DELETE /api/v1/users/{userId} 6. API Documentation Standards (Communicate Clearly) An undocumented API is a dead API. Good documentation allows developers to use your API without confusion. Professional Documentation Tools: OpenAPI Specification (Swagger) — Industry standard Postman API Docs Redoc Minimum Documentation Requirements: API Endpoints Request/Response Examples Status Codes and Error Responses Authentication Requirements Data Schemas (Fields, Types, Descriptions)
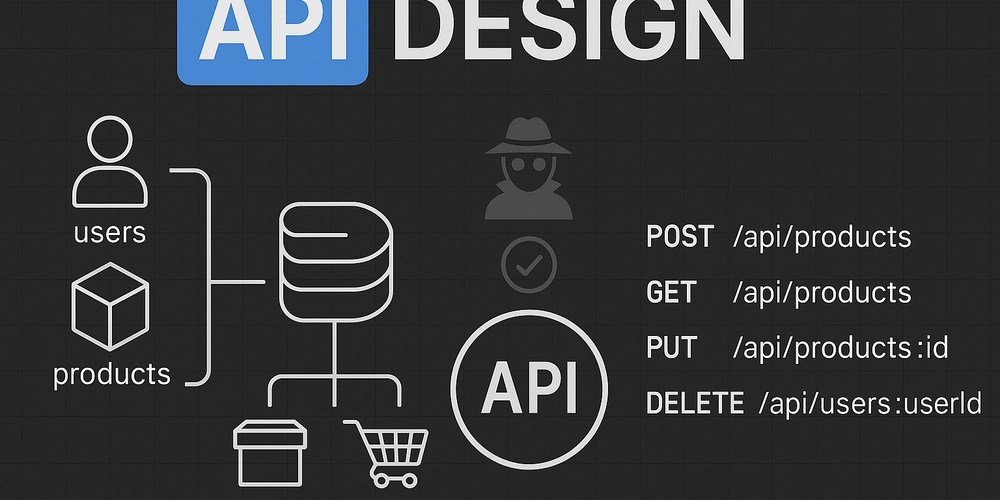
1. What is API Design?
An API (Application Programming Interface) allows two software systems to communicate with each other. API Design means structuring the way clients (apps, users, services) request and receive data. Good API design is essential for scalability, usability, security, and future growth.
2. Fundamental Principles of API Design
"Good API design follows simplicity, clarity, consistency, and predictability."
The four sacred principles:
Simplicity: Keep APIs intuitive and minimal.
Consistency: Maintain uniform naming, patterns, and conventions.
Clarity: Make APIs readable and self-explanatory.
Predictability: Clients should predict behavior based on structure.
3. Understanding REST Principles (Core Foundations)
REST (Representational State Transfer) is the most common architecture for modern APIs.
It relies on standard HTTP methods to interact with resources.
Key Concepts:
Term | Meaning |
---|---|
Resource | Object (e.g., User, Order, Product) |
Endpoint | URL where the resource can be accessed |
HTTP Methods | GET, POST, PUT, PATCH, DELETE |
Stateless | Each request must contain all the needed info |
Common REST HTTP Methods:
Method | Purpose |
---|---|
GET | Retrieve data |
POST | Create a new resource |
PUT | Update (replace) an existing resource |
PATCH | Update (partial) a resource |
DELETE | Remove a resource |
Tip: APIs should manipulate resources, not actions.
Bad Example:
POST /createUser
Good Example:
POST /users
4. API Versioning (Prepare for the Future)
Never design an API without planning versioning.
It allows you to update APIs without breaking existing clients.
Common Versioning Strategies:
Strategy | Example |
---|---|
URL versioning | GET /api/v1/users |
Header versioning | Accept: application/vnd.api.v1+json |
Query parameter | /api/users?version=1 |
Best Practice: Use URL versioning. It is clear, simple, and cache-friendly.
5. URL Design Best Practices (Clear and Consistent)
Your URL should speak clearly what it serves.
Rules:
Use nouns, not verbs:
/users
,/orders
Use plural names:
/products
not/product
Nest logically:
/users/{userId}/orders
Use hyphens not underscores:
/user-profile
Keep URLs lowercase
Examples:
Purpose | URL |
---|---|
List all users | GET /api/v1/users |
Retrieve a user | GET /api/v1/users/{userId} |
Create new user | POST /api/v1/users |
Update user | PUT /api/v1/users/{userId} |
Delete user | DELETE /api/v1/users/{userId} |
6. API Documentation Standards (Communicate Clearly)
An undocumented API is a dead API.
Good documentation allows developers to use your API without confusion.
Professional Documentation Tools:
OpenAPI Specification (Swagger) — Industry standard
Postman API Docs
Redoc
Minimum Documentation Requirements:
API Endpoints
Request/Response Examples
Status Codes and Error Responses
Authentication Requirements
Data Schemas (Fields, Types, Descriptions)