The Detailed Execution Pipeline of .NET
.NET is a powerful framework that enables developers to build a wide range of applications, from web and desktop to cloud and mobile. To truly understand how .NET applications run, it’s essential to explore the execution pipeline that transforms source code into a running application. This blog provides an in-depth yet easy-to-understand breakdown of the .NET execution pipeline. 1. Writing and Compiling the Source Code Everything starts with writing the source code using languages like C#, F#, or VB.NET. This code is then compiled using the respective language compiler (e.g., Roslyn for C#). Compilation Process: The compiler converts the source code into Intermediate Language (IL) (previously known as Microsoft Intermediate Language — MSIL). The IL code is stored in an assembly (DLL or EXE file) along with metadata describing the code’s structure. At this stage, the code is still platform-independent and not yet machine-executable. 2. Assembly Loading and Verification When the application starts, the Common Language Runtime (CLR) loads the compiled assemblies into memory. The CLR performs verification to ensure that the IL code adheres to type safety and security rules. Key Steps: Class Loader: Loads required classes from the assemblies. Metadata Processing: Reads type information and dependencies. Code Access Security (CAS): Ensures that the code has the necessary permissions. If the IL passes verification, the next step is Just-In-Time (JIT) compilation. 3. Just-In-Time (JIT) Compilation .NET uses a Just-In-Time Compiler (JIT) to convert IL into machine code specific to the target CPU architecture. Types of JIT Compilation: Normal JIT: Compiles methods on demand when they are first called and stores them in memory. Pre-JIT (Ahead-of-Time — AOT Compilation): Converts the entire assembly into native code before execution (e.g., Native AOT in .NET 7+). EconoJIT: Optimized for memory usage but does not cache compiled methods. Once compiled, the machine code remains in memory for subsequent executions to improve performance. 4. Garbage Collection and Memory Management .NET has an automated Garbage Collector (GC) that efficiently manages memory by cleaning up unused objects. .NET classifies objects into generations (Gen 0, Gen 1, Gen 2) to optimize memory reclamation. The GC runs periodically to free up memory occupied by unreachable objects. Large objects are allocated in the Large Object Heap (LOH) and cleaned up less frequently. This automatic memory management helps developers avoid common issues like memory leaks and dangling pointers. 5. Execution and Optimization After JIT compilation, the code is executed. The CLR continuously optimizes performance using: Inlining: Frequently used methods are directly inserted into the caller to reduce function call overhead. Tiered Compilation: Initially, methods are compiled quickly, and later, they are optimized further for performance. Just-in-time Profiling: CLR analyzes execution patterns and optimizes the code accordingly. These optimizations make .NET applications faster and more efficient over time. 6. Exception Handling and Error Management .NET has a robust exception-handling mechanism that ensures runtime errors are properly handled. Try-Catch-Finally blocks prevent application crashes. Unhandled exceptions are caught by the default exception handler in the CLR. Developers can use custom exception handling frameworks to log and recover from errors gracefully. 7. Deployment and Execution in Different Environments .NET applications can run in various environments: Standalone Deployment: The application includes the required runtime and dependencies. Framework-Dependent Deployment: The application requires .NET to be installed on the machine. Cloud Deployment: Runs in containers or serverless platforms like Azure Functions. Cross-Platform Execution: With .NET Core/.NET 5+, applications can run on Windows, Linux, and macOS. Final Thoughts Understanding the execution pipeline of .NET is crucial for optimizing performance and troubleshooting issues effectively. From writing code to JIT compilation, memory management, and runtime optimizations, every step ensures smooth and efficient execution.
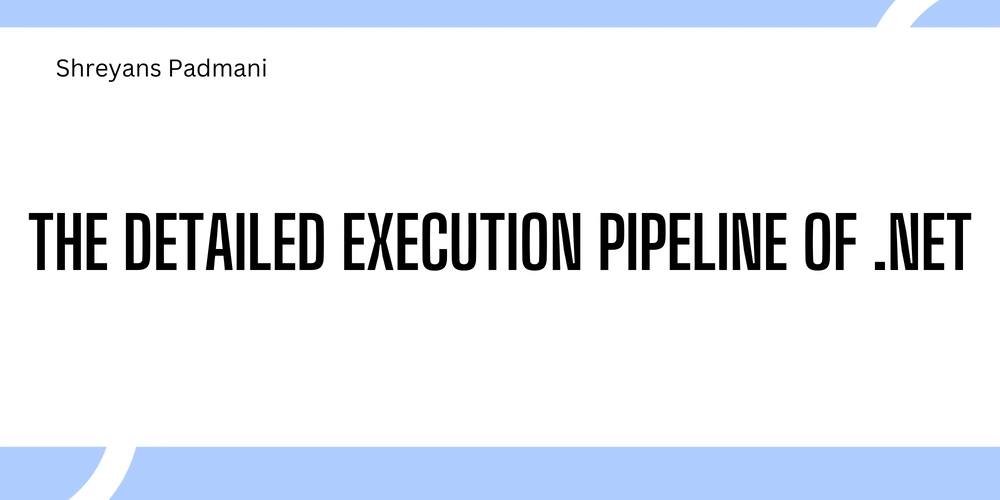
.NET is a powerful framework that enables developers to build a wide range of applications, from web and desktop to cloud and mobile. To truly understand how .NET applications run, it’s essential to explore the execution pipeline that transforms source code into a running application. This blog provides an in-depth yet easy-to-understand breakdown of the .NET execution pipeline.
1. Writing and Compiling the Source Code
Everything starts with writing the source code using languages like C#, F#, or VB.NET. This code is then compiled using the respective language compiler (e.g., Roslyn for C#).
Compilation Process:
- The compiler converts the source code into Intermediate Language (IL) (previously known as Microsoft Intermediate Language — MSIL).
- The IL code is stored in an assembly (DLL or EXE file) along with metadata describing the code’s structure.
At this stage, the code is still platform-independent and not yet machine-executable.
2. Assembly Loading and Verification
When the application starts, the Common Language Runtime (CLR) loads the compiled assemblies into memory. The CLR performs verification to ensure that the IL code adheres to type safety and security rules.
Key Steps:
- Class Loader: Loads required classes from the assemblies.
- Metadata Processing: Reads type information and dependencies.
- Code Access Security (CAS): Ensures that the code has the necessary permissions.
If the IL passes verification, the next step is Just-In-Time (JIT) compilation.
3. Just-In-Time (JIT) Compilation
.NET uses a Just-In-Time Compiler (JIT) to convert IL into machine code specific to the target CPU architecture.
Types of JIT Compilation:
- Normal JIT: Compiles methods on demand when they are first called and stores them in memory.
- Pre-JIT (Ahead-of-Time — AOT Compilation): Converts the entire assembly into native code before execution (e.g., Native AOT in .NET 7+).
- EconoJIT: Optimized for memory usage but does not cache compiled methods.
Once compiled, the machine code remains in memory for subsequent executions to improve performance.
4. Garbage Collection and Memory Management
.NET has an automated Garbage Collector (GC) that efficiently manages memory by cleaning up unused objects.
- .NET classifies objects into generations (Gen 0, Gen 1, Gen 2) to optimize memory reclamation.
- The GC runs periodically to free up memory occupied by unreachable objects.
- Large objects are allocated in the Large Object Heap (LOH) and cleaned up less frequently.
This automatic memory management helps developers avoid common issues like memory leaks and dangling pointers.
5. Execution and Optimization
After JIT compilation, the code is executed. The CLR continuously optimizes performance using:
- Inlining: Frequently used methods are directly inserted into the caller to reduce function call overhead.
- Tiered Compilation: Initially, methods are compiled quickly, and later, they are optimized further for performance.
- Just-in-time Profiling: CLR analyzes execution patterns and optimizes the code accordingly.
These optimizations make .NET applications faster and more efficient over time.
6. Exception Handling and Error Management
.NET has a robust exception-handling mechanism that ensures runtime errors are properly handled.
- Try-Catch-Finally blocks prevent application crashes.
- Unhandled exceptions are caught by the default exception handler in the CLR.
- Developers can use custom exception handling frameworks to log and recover from errors gracefully.
7. Deployment and Execution in Different Environments
.NET applications can run in various environments:
- Standalone Deployment: The application includes the required runtime and dependencies.
- Framework-Dependent Deployment: The application requires .NET to be installed on the machine.
- Cloud Deployment: Runs in containers or serverless platforms like Azure Functions.
- Cross-Platform Execution: With .NET Core/.NET 5+, applications can run on Windows, Linux, and macOS.
Final Thoughts
Understanding the execution pipeline of .NET is crucial for optimizing performance and troubleshooting issues effectively. From writing code to JIT compilation, memory management, and runtime optimizations, every step ensures smooth and efficient execution.