Stock Management System
Hi Guys! Let's Build a MERN Stack Application from scratch,A Stock Management System as part of this plan let's complete this step by step. Backend Setup 1.Initialize the project directory and split the folder by creating subfolders as client and server. And now we complete the server setup. code along with me in IDE called VSCode. mkdir stock-management-system && cd stock-management-system mkdir backend && cd backend npm init -y 2.After initializing the package.json install the backend dependencies that are required to build our project. npm install express mongoose dotenv cors jsonwebtoken bcryptjs multer nodemon 3.Maintain a directory structure in this format to address the code with no trouble and to maintain a clear view. backend/ │── models/ │ ├── Product.js │ ├── User.js │── routes/ │ ├── productRoutes.js │ ├── userRoutes.js │── controllers/ │ ├── productController.js │ ├── userController.js │── middleware/ │ ├── authMiddleware.js │── config/ │ ├── db.js │── uploads/ │── .env │── server.js │── package.json 4.Start coding Connect database db.js const mongoose = require('mongoose'); const connectDB = async () => { try { const conn = await mongoose.connect(process.env.MONGO_URI, { useNewUrlParser: true, useUnifiedTopology: true, }); console.log(`MongoDB Connected: ${conn.connection.host}`); } catch (error) { console.error(`Error: ${error.message}`); process.exit(1); } }; module.exports = connectDB; create model Product.js const mongoose = require('mongoose'); const productSchema = mongoose.Schema({ name: { type: String, required: true }, category: { type: String, required: true }, price: { type: Number, required: true }, stockQuantity: { type: Number, required: true }, itemsSold: { type: Number, default: 0 }, description: { type: String } }, { timestamps: true }); module.exports = mongoose.model('Product', productSchema); provide routes to specified model productRoute.js const express = require('express'); const { getProducts, createProduct } = require('../controllers/productController'); const router = express.Router(); router.get('/', getProducts); router.post('/', createProduct); module.exports = router; perform controller functions to perform actions on routes productController.js const Product = require('../models/Product'); exports.getProducts = async (req, res) => { const products = await Product.find({}); res.json(products); }; exports.createProduct = async (req, res) => { const { name, category, price, stockQuantity, description } = req.body; const product = new Product({ name, category, price, stockQuantity, description }); await product.save(); res.status(201).json(product); }; set up the server server.js const express = require('express'); const dotenv = require('dotenv'); const cors = require('cors'); const connectDB = require('./config/db'); const productRoutes = require('./routes/productRoutes'); dotenv.config(); connectDB(); const app = express(); app.use(cors()); app.use(express.json()); app.use('/api/products', productRoutes); app.listen(5000, () => console.log('Server running on port 5000')); app.get('/', (req, res) => { res.send('API is running...'); } ); module.exports = app; Start the Server npm start -> as you need to specify under the scripts inside the package.json file as "start": "nodemon server.js" If you Got to see the above result your server is live at port 5000. Test the API now U are all set to test the api created to implement the basic product specific model and product specific actions inorder to execute and test it using the api testing tools like Thunder Client or the Postman based on your preferences. I'm using Thunder client which is a VSCode extension, just install and proceed to continue along. 1.Provide a get request http://localhost:5000/ 2.Add a product based on model specified using post request. 3.Test the another route we have created, to get all the products. Explanation In API Testing of the CURD operations plays a crutial role which are defined in routes i.e. Controllers to post a product, the server is requesting a json body that is aligned with the model specified fields to be matched, as the client sends the response as described in image 2. const { name, category, price, stockQuantity, description } = req.body; 2.The response is printed with status code specified by the user. res.status(200).json(products); 3.And the get request of getting all the products with find query const products = await Product.find({}); For any errors U can even get the results in console log to know what is breaking, or U can use the middleware directly to address the errors app.use((err, req, res, next) => { const statusCode = res.statusCod
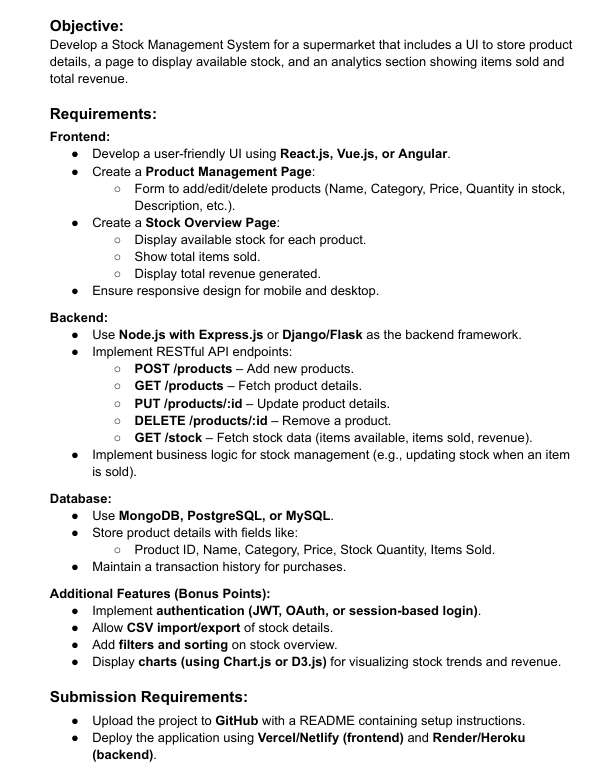
Hi Guys!
Let's Build a MERN Stack Application from scratch,A Stock Management System as part of this plan let's complete this step by step.
Backend Setup
1.Initialize the project directory and split the folder by creating subfolders as client and server.
And now we complete the server setup.
code along with me in IDE called VSCode.
mkdir stock-management-system && cd stock-management-system
mkdir backend && cd backend
npm init -y
2.After initializing the package.json install the backend dependencies that are required to build our project.
npm install express mongoose dotenv cors jsonwebtoken bcryptjs multer nodemon
3.Maintain a directory structure in this format to address the code with no trouble and to maintain a clear view.
backend/
│── models/
│ ├── Product.js
│ ├── User.js
│── routes/
│ ├── productRoutes.js
│ ├── userRoutes.js
│── controllers/
│ ├── productController.js
│ ├── userController.js
│── middleware/
│ ├── authMiddleware.js
│── config/
│ ├── db.js
│── uploads/
│── .env
│── server.js
│── package.json
4.Start coding
- Connect database db.js
const mongoose = require('mongoose');
const connectDB = async () => {
try {
const conn = await mongoose.connect(process.env.MONGO_URI, {
useNewUrlParser: true,
useUnifiedTopology: true,
});
console.log(`MongoDB Connected: ${conn.connection.host}`);
} catch (error) {
console.error(`Error: ${error.message}`);
process.exit(1);
}
};
module.exports = connectDB;
- create model
Product.js
const mongoose = require('mongoose');
const productSchema = mongoose.Schema({
name: { type: String, required: true },
category: { type: String, required: true },
price: { type: Number, required: true },
stockQuantity: { type: Number, required: true },
itemsSold: { type: Number, default: 0 },
description: { type: String }
}, { timestamps: true });
module.exports = mongoose.model('Product', productSchema);
- provide routes to specified model
productRoute.js
const express = require('express');
const { getProducts, createProduct } = require('../controllers/productController');
const router = express.Router();
router.get('/', getProducts);
router.post('/', createProduct);
module.exports = router;
- perform controller functions to perform actions on routes
productController.js
const Product = require('../models/Product');
exports.getProducts = async (req, res) => {
const products = await Product.find({});
res.json(products);
};
exports.createProduct = async (req, res) => {
const { name, category, price, stockQuantity, description } = req.body;
const product = new Product({ name, category, price, stockQuantity, description });
await product.save();
res.status(201).json(product);
};
- set up the server server.js
const express = require('express');
const dotenv = require('dotenv');
const cors = require('cors');
const connectDB = require('./config/db');
const productRoutes = require('./routes/productRoutes');
dotenv.config();
connectDB();
const app = express();
app.use(cors());
app.use(express.json());
app.use('/api/products', productRoutes);
app.listen(5000, () => console.log('Server running on port 5000'));
app.get('/', (req, res) => {
res.send('API is running...');
} );
module.exports = app;
- Start the Server
npm start
-> as you need to specify under the scripts inside the package.json
file as
"start": "nodemon server.js"
If you Got to see the above result your server is live at port 5000.
- Test the API
now U are all set to test the api created to implement the basic product specific model and product specific actions inorder to execute and test it using the api testing tools like Thunder Client or the Postman based on your preferences.
- I'm using Thunder client which is a VSCode extension, just install and proceed to continue along.
1.Provide a get request
http://localhost:5000/
2.Add a product based on model specified using post request.
3.Test the another route we have created, to get all the products.
Explanation
- In API Testing of the CURD operations plays a crutial role which are defined in routes i.e. Controllers
- to post a product, the server is requesting a json body that is aligned with the model specified fields to be matched, as the client sends the response as described in image 2.
const { name, category, price, stockQuantity, description } = req.body;
2.The response is printed with status code specified by the user.
res.status(200).json(products);
3.And the get request of getting all the products with find query
const products = await Product.find({});
For any errors U can even get the results in console log to know what is breaking, or U can use the middleware directly to address the errors
app.use((err, req, res, next) => {
const statusCode = res.statusCode ? res.statusCode : 500;
res.status(statusCode);
res.json({
message: err.message,
stack: process.env.NODE_ENV === 'production' ? null : err.stack,
});
}
Don't forget to setup the environment variables, .env
file
Hurray!