Static
Static Block in Java A static block (also called a static initialization block) is a block of code inside a Java class that runs when the class is loaded into memory (before the main() method or any object creation). It is used to initialize static variables or perform one-time setup tasks for the class. Key Features: Executes only once when the class is first loaded by the JVM. Runs before main() or any instance creation. Can have multiple static blocks (they execute in the order they appear). Cannot access non-static (instance) members (since no object exists yet). Syntax static { // Code to be executed when the class loads } Why Use a Static Block? Initialize static variables (especially if computation is needed). Load native libraries (e.g., System.loadLibrary()). Perform one-time setup (e.g., database connection setup). Example 1: Basic Static Block class Test { static int x; // Static block runs when class loads static { x = 10; System.out.println("Static block executed. x = " + x); } } public class Main { public static void main(String[] args) { System.out.println("Main method starts."); System.out.println("Test.x = " + Test.x); // x already initialized } } Output: Static block executed. x = 10 Main method starts. Test.x = 10 The static block runs before main(). Example 2: Multiple Static Blocks class Test { static int a; static int b; static { a = 5; System.out.println("First static block: a = " + a); } static { b = a * 2; System.out.println("Second static block: b = " + b); } } public class Main { public static void main(String[] args) { System.out.println("a = " + Test.a + ", b = " + Test.b); } } Output: First static block: a = 5 Second static block: b = 10 a = 5, b = 10 Static blocks execute in the order they are defined. Example 3: Static Block for Loading Libraries class Database { static { System.out.println("Loading database driver..."); // Simulate loading a JDBC driver try { Class.forName("com.mysql.jdbc.Driver"); } catch (ClassNotFoundException e) { e.printStackTrace(); } } static void connect() { System.out.println("Connected to database."); } } public class Main { public static void main(String[] args) { Database.connect(); // Static block runs first } } Output: Loading database driver... Connected to database. The static block ensures the driver is loaded before any database operation. When to Use Static Block? Scenario Example Initialize static variables with complex logic static { PI = 3.14159; } Load external libraries System.loadLibrary("nativeLib"); One-time setup before any instance is created Database driver setup Key Takeaways Runs only once when the class is loaded. Executes before main() and instance creation. Cannot access instance variables/methods (since no object exists). Useful for static initializations (e.g., constants, resources). Would you like an example with static blocks in inheritance? Let me know!
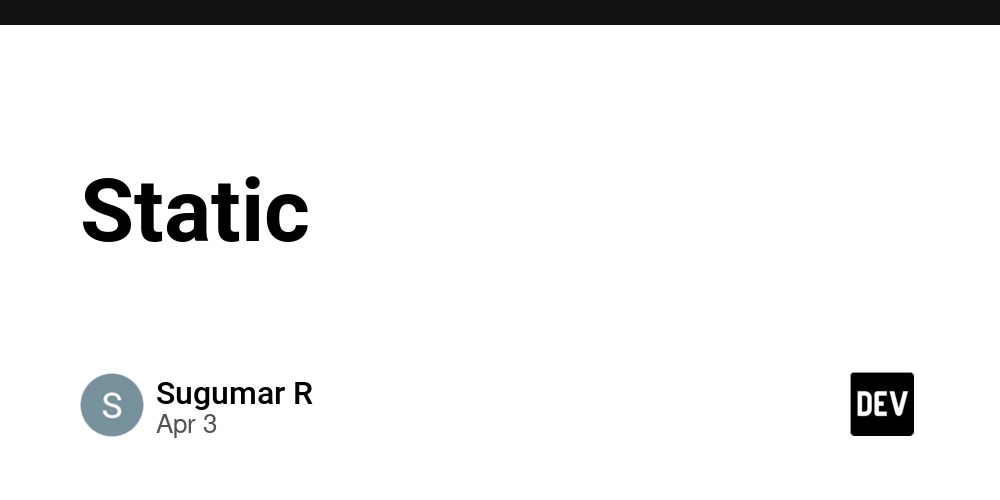
Static Block in Java
A static block (also called a static initialization block) is a block of code inside a Java class that runs when the class is loaded into memory (before the main()
method or any object creation). It is used to initialize static variables or perform one-time setup tasks for the class.
Key Features:
- Executes only once when the class is first loaded by the JVM.
-
Runs before
main()
or any instance creation. - Can have multiple static blocks (they execute in the order they appear).
- Cannot access non-static (instance) members (since no object exists yet).
Syntax
static {
// Code to be executed when the class loads
}
Why Use a Static Block?
- Initialize static variables (especially if computation is needed).
- Load native libraries (e.g.,
System.loadLibrary()
). - Perform one-time setup (e.g., database connection setup).
Example 1: Basic Static Block
class Test {
static int x;
// Static block runs when class loads
static {
x = 10;
System.out.println("Static block executed. x = " + x);
}
}
public class Main {
public static void main(String[] args) {
System.out.println("Main method starts.");
System.out.println("Test.x = " + Test.x); // x already initialized
}
}
Output:
Static block executed. x = 10
Main method starts.
Test.x = 10
- The static block runs before
main()
.
Example 2: Multiple Static Blocks
class Test {
static int a;
static int b;
static {
a = 5;
System.out.println("First static block: a = " + a);
}
static {
b = a * 2;
System.out.println("Second static block: b = " + b);
}
}
public class Main {
public static void main(String[] args) {
System.out.println("a = " + Test.a + ", b = " + Test.b);
}
}
Output:
First static block: a = 5
Second static block: b = 10
a = 5, b = 10
- Static blocks execute in the order they are defined.
Example 3: Static Block for Loading Libraries
class Database {
static {
System.out.println("Loading database driver...");
// Simulate loading a JDBC driver
try {
Class.forName("com.mysql.jdbc.Driver");
} catch (ClassNotFoundException e) {
e.printStackTrace();
}
}
static void connect() {
System.out.println("Connected to database.");
}
}
public class Main {
public static void main(String[] args) {
Database.connect(); // Static block runs first
}
}
Output:
Loading database driver...
Connected to database.
- The static block ensures the driver is loaded before any database operation.
When to Use Static Block?
Scenario | Example |
---|---|
Initialize static variables with complex logic | static { PI = 3.14159; } |
Load external libraries | System.loadLibrary("nativeLib"); |
One-time setup before any instance is created | Database driver setup |
Key Takeaways
- Runs only once when the class is loaded.
-
Executes before
main()
and instance creation. - Cannot access instance variables/methods (since no object exists).
- Useful for static initializations (e.g., constants, resources).
Would you like an example with static blocks in inheritance? Let me know!