10 Essential Steps to Secure a Node.js REST API
Building a REST API with Node.js is a straightforward process, but making it secure? That’s where things get tricky. In today’s world, APIs are prime targets for attacks, and even a single vulnerability can expose sensitive user data. Whether you’re handling authentication, managing user roles, or protecting against common exploits, security should always be a top priority. 1. Always Use HTTPS The first and most basic rule of API security is: never expose your API over HTTP. Always use HTTPS to encrypt data in transit, preventing man-in-the-middle (MITM) attacks. How to Implement HTTPS in Node.js If you're running your API on a local machine or development server, you can use a self-signed certificate for HTTPS: const https = require('https'); const fs = require('fs'); const express = require('express'); const app = express(); const options = { key: fs.readFileSync('server.key'), cert: fs.readFileSync('server.cert'), }; https.createServer(options, app).listen(3000, () => { console.log('Secure server running on https://localhost:3000'); }); In production, you should use Let’s Encrypt or a Cloudflare proxy to enforce HTTPS. If you’re deploying on AWS, Azure, or Google Cloud, use their built-in HTTPS services. 2. Implement API Authentication & Authorization Without proper authentication and authorization, your API is vulnerable to unauthorized access. JWT (JSON Web Token) for Authentication JWT is the industry standard for securing REST APIs. Here’s how you can implement JWT authentication in a Node.js API: Install jsonwebtoken: npm install jsonwebtoken Generate a token when a user logs in: const jwt = require('jsonwebtoken'); function generateToken(user) { return jwt.sign({ id: user.id, role: user.role }, 'your-secret-key', { expiresIn: '1h', }); } Protect your routes: function authenticateToken(req, res, next) { const token = req.headers['authorization']; if (!token) return res.status(403).json({ message: 'Access denied' }); jwt.verify(token, 'your-secret-key', (err, user) => { if (err) return res.status(401).json({ message: 'Invalid token' }); req.user = user; next(); }); } app.get('/protected', authenticateToken, (req, res) => { res.json({ message: 'This is a protected route' }); }); For better security, store your secret keys in environment variables instead of hardcoding them. 3. Use Rate Limiting APIs are often the target of brute-force attacks and DDoS attempts. Rate limiting restricts the number of requests a client can make within a time frame. How to Implement Rate Limiting in Express.js You can use express-rate-limit to limit requests: npm install express-rate-limit const rateLimit = require('express-rate-limit'); const limiter = rateLimit({ windowMs: 15 * 60 * 1000, // 15 minutes max: 100, // limit each IP to 100 requests per windowMs message: 'Too many requests, please try again later.', }); app.use(limiter); This prevents users from sending too many requests in a short period. 4. Validate and Sanitize User Input User input is a major attack vector for SQL injection, NoSQL injection, and XSS attacks. Always validate and sanitize input. Using Joi for Validation npm install joi const Joi = require('joi'); const userSchema = Joi.object({ username: Joi.string().alphanum().min(3).max(30).required(), email: Joi.string().email().required(), password: Joi.string().min(8).required(), }); app.post('/register', (req, res) => { const { error } = userSchema.validate(req.body); if (error) return res.status(400).json({ message: error.details[0].message }); res.json({ message: 'User registered successfully' }); }); Sanitizing Input Use express-validator for sanitization: npm install express-validator const { body, validationResult } = require('express-validator'); app.post('/login', [ body('email').isEmail().normalizeEmail(), body('password').trim().escape(), ], (req, res) => { const errors = validationResult(req); if (!errors.isEmpty()) return res.status(400).json({ errors: errors.array() }); res.json({ message: 'Login successful' }); }); 5. Protect Against SQL & NoSQL Injection If you’re using a database, prevent injection attacks by using parameterized queries. SQL Injection Prevention If using MySQL or PostgreSQL with mysql2 or pg: const db = require('mysql2/promise'); async function getUser(username) { const [rows] = await db.execute('SELECT * FROM users WHERE username = ?', [username]); return rows; } NoSQL Injection Prevention If using MongoDB with Mongoose: const User = require('./models/User'); app.get('/user/:id', async (req, res) => { const user = await User.findOne({ _id: req.params.id }).exec(); res.json(user); });
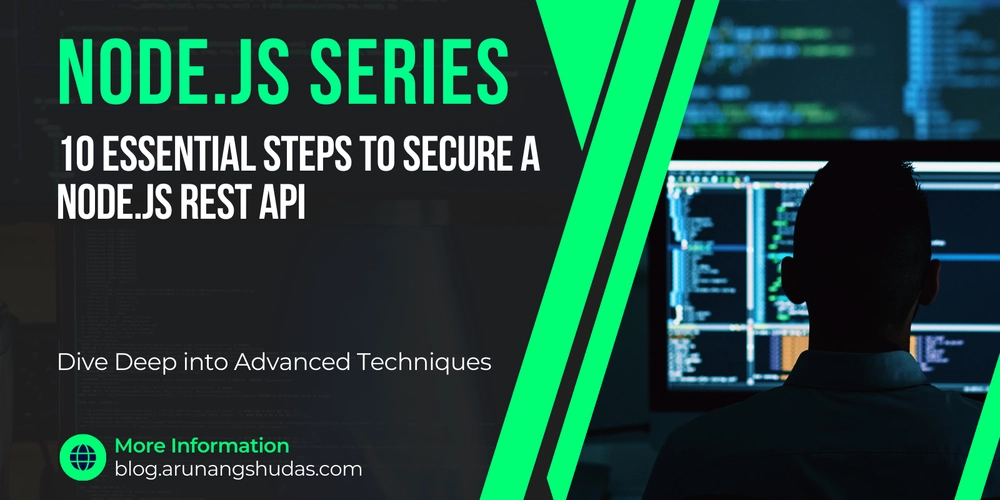
Building a REST API with Node.js is a straightforward process, but making it secure? That’s where things get tricky. In today’s world, APIs are prime targets for attacks, and even a single vulnerability can expose sensitive user data. Whether you’re handling authentication, managing user roles, or protecting against common exploits, security should always be a top priority.
1. Always Use HTTPS
The first and most basic rule of API security is: never expose your API over HTTP. Always use HTTPS to encrypt data in transit, preventing man-in-the-middle (MITM) attacks.
How to Implement HTTPS in Node.js
If you're running your API on a local machine or development server, you can use a self-signed certificate for HTTPS:
const https = require('https');
const fs = require('fs');
const express = require('express');
const app = express();
const options = {
key: fs.readFileSync('server.key'),
cert: fs.readFileSync('server.cert'),
};
https.createServer(options, app).listen(3000, () => {
console.log('Secure server running on https://localhost:3000');
});
In production, you should use Let’s Encrypt or a Cloudflare proxy to enforce HTTPS. If you’re deploying on AWS, Azure, or Google Cloud, use their built-in HTTPS services.
2. Implement API Authentication & Authorization
Without proper authentication and authorization, your API is vulnerable to unauthorized access.
JWT (JSON Web Token) for Authentication
JWT is the industry standard for securing REST APIs. Here’s how you can implement JWT authentication in a Node.js API:
- Install
jsonwebtoken
:
npm install jsonwebtoken
- Generate a token when a user logs in:
const jwt = require('jsonwebtoken');
function generateToken(user) {
return jwt.sign({ id: user.id, role: user.role }, 'your-secret-key', {
expiresIn: '1h',
});
}
- Protect your routes:
function authenticateToken(req, res, next) {
const token = req.headers['authorization'];
if (!token) return res.status(403).json({ message: 'Access denied' });
jwt.verify(token, 'your-secret-key', (err, user) => {
if (err) return res.status(401).json({ message: 'Invalid token' });
req.user = user;
next();
});
}
app.get('/protected', authenticateToken, (req, res) => {
res.json({ message: 'This is a protected route' });
});
For better security, store your secret keys in environment variables instead of hardcoding them.
3. Use Rate Limiting
APIs are often the target of brute-force attacks and DDoS attempts. Rate limiting restricts the number of requests a client can make within a time frame.
How to Implement Rate Limiting in Express.js
You can use express-rate-limit
to limit requests:
npm install express-rate-limit
const rateLimit = require('express-rate-limit');
const limiter = rateLimit({
windowMs: 15 * 60 * 1000, // 15 minutes
max: 100, // limit each IP to 100 requests per windowMs
message: 'Too many requests, please try again later.',
});
app.use(limiter);
This prevents users from sending too many requests in a short period.
4. Validate and Sanitize User Input
User input is a major attack vector for SQL injection, NoSQL injection, and XSS attacks. Always validate and sanitize input.
Using Joi for Validation
npm install joi
const Joi = require('joi');
const userSchema = Joi.object({
username: Joi.string().alphanum().min(3).max(30).required(),
email: Joi.string().email().required(),
password: Joi.string().min(8).required(),
});
app.post('/register', (req, res) => {
const { error } = userSchema.validate(req.body);
if (error) return res.status(400).json({ message: error.details[0].message });
res.json({ message: 'User registered successfully' });
});
Sanitizing Input
Use express-validator
for sanitization:
npm install express-validator
const { body, validationResult } = require('express-validator');
app.post('/login', [
body('email').isEmail().normalizeEmail(),
body('password').trim().escape(),
], (req, res) => {
const errors = validationResult(req);
if (!errors.isEmpty()) return res.status(400).json({ errors: errors.array() });
res.json({ message: 'Login successful' });
});
5. Protect Against SQL & NoSQL Injection
If you’re using a database, prevent injection attacks by using parameterized queries.
SQL Injection Prevention
If using MySQL or PostgreSQL with mysql2
or pg
:
const db = require('mysql2/promise');
async function getUser(username) {
const [rows] = await db.execute('SELECT * FROM users WHERE username = ?', [username]);
return rows;
}
NoSQL Injection Prevention
If using MongoDB with Mongoose:
const User = require('./models/User');
app.get('/user/:id', async (req, res) => {
const user = await User.findOne({ _id: req.params.id }).exec();
res.json(user);
});
6. Secure API Keys & Environment Variables
Never store API keys or database credentials in your code. Use dotenv to manage environment variables.
npm install dotenv
require('dotenv').config();
const dbPassword = process.env.DB_PASSWORD;
const jwtSecret = process.env.JWT_SECRET;
Store secrets in a .env
file and add it to .gitignore
to prevent it from being committed.
7. Implement CORS Properly
Cross-Origin Resource Sharing (CORS) ensures only allowed origins can access your API.
npm install cors
const cors = require('cors');
app.use(cors({ origin: 'https://yourfrontend.com' }));
8. Log and Monitor API Activity
Use winston
for logging API activity.
npm install winston
const winston = require('winston');
const logger = winston.createLogger({
level: 'info',
format: winston.format.json(),
transports: [
new winston.transports.File({ filename: 'logs/api.log' }),
],
});
app.use((req, res, next) => {
logger.info(`${req.method} ${req.url}`);
next();
});
9. Use Helmet to Secure HTTP Headers
Helmet helps protect against Clickjacking, XSS, and other attacks.
npm install helmet
const helmet = require('helmet');
app.use(helmet());
10. Keep Dependencies Updated
Use npm audit
to check for vulnerabilities:
npm audit
Update dependencies:
npm update
Use tools like Snyk to scan for security issues.
Final Thoughts
Securing your Node.js REST API isn’t a one-time task—it’s an ongoing process.
You may also like:
Read more blogs from Here
Share your experiences in the comments, and let’s discuss how to tackle them!
Follow me on Linkedin