Rust Ownership and Borrowing Explained
Introduction In Rust, ownership is a mechanism for managing memory, used to determine which variable has control over a piece of memory at any given time. Rust checks ownership relationships at compile time and informs the programmer when a variable can be used and when memory needs to be released. Every value has an owner, and at any given time, there can be only one owner. When the owner goes out of scope, the memory it owns is automatically released. If you attempt to access memory that has already been released, Rust will refuse to compile. Rust’s ownership mechanism is implemented through borrowing. Borrowing means accessing a variable via references rather than taking ownership. Through borrowing, multiple variables can access the same memory simultaneously, but they cannot modify it at the same time. The ownership mechanism is a key feature of Rust that ensures programs are free from common memory safety issues such as null pointer references, memory leaks, and data races. Ownership Determination Examples In Rust, ownership relationships can be identified through the following scenarios: When a variable is declared, it takes ownership of the value. let s = String::from("hello"); // s owns the value "hello" When assigning a value with ownership to another variable, ownership is transferred to the new variable. This is called a move. let s1 = String::from("hello"); let s2 = s1; // s2 takes ownership from s1; s1 no longer owns "hello" A reference (&) can be used to borrow the value of a variable without taking ownership. When accessing a value through a reference, the ownership does not change. let s1 = String::from("hello"); let len = calculate_length(&s1); // &s1 borrows s1, but s1 still owns the value A mutable reference (&mut) allows modification of the variable’s value, but only one mutable reference can exist at a time, and all immutable references must be disconnected. let mut s = String::from("hello"); let r1 = &s; // immutable reference let r2 = &s; // immutable reference let r3 = &mut s; // mutable reference // Compilation error: r1 and r2 conflict with r3 In summary, in Rust, ownership relationships can be determined through variable binding, variable assignment, and mutable borrowing. So how should we understand mutable and immutable references? Mutable and Immutable References In Rust, mutable and immutable references are crucial components of memory management and ownership. They allow programmers to access and modify variables in a controlled manner. Immutable references provide read-only access to a variable. They can be shared among multiple concurrent readers but cannot modify the value. The benefit of immutable references is that they prevent data races — errors that occur in concurrent environments and are hard to debug. Mutable references provide read and write access but can only be held by one borrower at a time. They offer more flexibility in managing a variable’s value but require caution to avoid data races. A data race can occur when multiple accesses are made to a variable concurrently and at least one access is a write. Here are some core concepts and rules to help you understand mutable and immutable references in depth: Mutable and immutable references can exist, but at any given moment, only one mutable reference or any number of immutable references may exist. let mut s = String::from("hello"); let r1 = &s; // immutable reference let r2 = &mut s; // compilation error: r1 has already borrowed s, so a mutable reference cannot coexist The lifetime of a reference must match the lifetime of the referenced variable, i.e., a reference cannot outlive the variable it points to. fn main() { let r; { let x = 5; r = &x; // compilation error: x’s lifetime is shorter than r’s; r would point to invalid memory } println!("r: {}", r); } Mutable and immutable references cannot be converted into each other, but a mutable reference can be converted into another mutable reference. let mut s = String::from("hello"); let r1 = &s; // immutable reference let r2 = &mut s; // mutable reference // Compilation error: cannot create a mutable reference while an immutable one exists Multiple mutable references are not allowed in the same scope. let mut s = String::from("hello"); let r1 = &mut s; // mutable reference let r2 = &mut s; // compilation error: multiple mutable references in the same scope are not allowed In summary, mutable and immutable references are vital parts of Rust’s memory management and ownership system. They allow for more flexible and maintainable code. However, they must be used carefully to avoid data races and memory safety issues. We are Leapcell, your top choice for hosting Rust projects. Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis: Multi-Language Support Develop with N
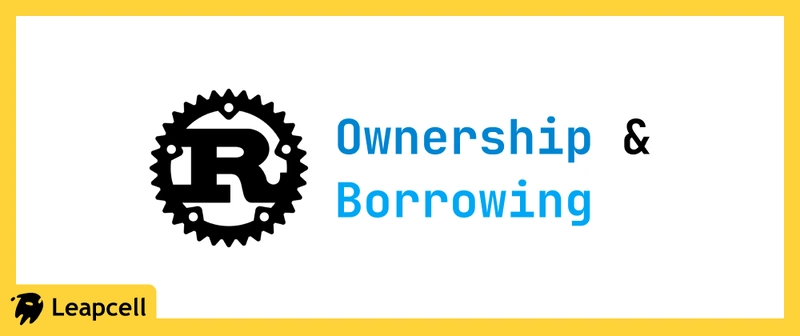
Introduction
In Rust, ownership is a mechanism for managing memory, used to determine which variable has control over a piece of memory at any given time. Rust checks ownership relationships at compile time and informs the programmer when a variable can be used and when memory needs to be released.
Every value has an owner, and at any given time, there can be only one owner. When the owner goes out of scope, the memory it owns is automatically released. If you attempt to access memory that has already been released, Rust will refuse to compile.
Rust’s ownership mechanism is implemented through borrowing. Borrowing means accessing a variable via references rather than taking ownership. Through borrowing, multiple variables can access the same memory simultaneously, but they cannot modify it at the same time.
The ownership mechanism is a key feature of Rust that ensures programs are free from common memory safety issues such as null pointer references, memory leaks, and data races.
Ownership Determination Examples
In Rust, ownership relationships can be identified through the following scenarios:
When a variable is declared, it takes ownership of the value.
let s = String::from("hello"); // s owns the value "hello"
When assigning a value with ownership to another variable, ownership is transferred to the new variable. This is called a move.
let s1 = String::from("hello");
let s2 = s1; // s2 takes ownership from s1; s1 no longer owns "hello"
A reference (&
) can be used to borrow the value of a variable without taking ownership. When accessing a value through a reference, the ownership does not change.
let s1 = String::from("hello");
let len = calculate_length(&s1); // &s1 borrows s1, but s1 still owns the value
A mutable reference (&mut
) allows modification of the variable’s value, but only one mutable reference can exist at a time, and all immutable references must be disconnected.
let mut s = String::from("hello");
let r1 = &s; // immutable reference
let r2 = &s; // immutable reference
let r3 = &mut s; // mutable reference
// Compilation error: r1 and r2 conflict with r3
In summary, in Rust, ownership relationships can be determined through variable binding, variable assignment, and mutable borrowing. So how should we understand mutable and immutable references?
Mutable and Immutable References
In Rust, mutable and immutable references are crucial components of memory management and ownership. They allow programmers to access and modify variables in a controlled manner.
Immutable references provide read-only access to a variable. They can be shared among multiple concurrent readers but cannot modify the value. The benefit of immutable references is that they prevent data races — errors that occur in concurrent environments and are hard to debug.
Mutable references provide read and write access but can only be held by one borrower at a time. They offer more flexibility in managing a variable’s value but require caution to avoid data races. A data race can occur when multiple accesses are made to a variable concurrently and at least one access is a write.
Here are some core concepts and rules to help you understand mutable and immutable references in depth:
Mutable and immutable references can exist, but at any given moment, only one mutable reference or any number of immutable references may exist.
let mut s = String::from("hello");
let r1 = &s; // immutable reference
let r2 = &mut s; // compilation error: r1 has already borrowed s, so a mutable reference cannot coexist
The lifetime of a reference must match the lifetime of the referenced variable, i.e., a reference cannot outlive the variable it points to.
fn main() {
let r;
{
let x = 5;
r = &x; // compilation error: x’s lifetime is shorter than r’s; r would point to invalid memory
}
println!("r: {}", r);
}
Mutable and immutable references cannot be converted into each other, but a mutable reference can be converted into another mutable reference.
let mut s = String::from("hello");
let r1 = &s; // immutable reference
let r2 = &mut s; // mutable reference
// Compilation error: cannot create a mutable reference while an immutable one exists
Multiple mutable references are not allowed in the same scope.
let mut s = String::from("hello");
let r1 = &mut s; // mutable reference
let r2 = &mut s; // compilation error: multiple mutable references in the same scope are not allowed
In summary, mutable and immutable references are vital parts of Rust’s memory management and ownership system. They allow for more flexible and maintainable code. However, they must be used carefully to avoid data races and memory safety issues.
We are Leapcell, your top choice for hosting Rust projects.
Leapcell is the Next-Gen Serverless Platform for Web Hosting, Async Tasks, and Redis:
Multi-Language Support
- Develop with Node.js, Python, Go, or Rust.
Deploy unlimited projects for free
- pay only for usage — no requests, no charges.
Unbeatable Cost Efficiency
- Pay-as-you-go with no idle charges.
- Example: $25 supports 6.94M requests at a 60ms average response time.
Streamlined Developer Experience
- Intuitive UI for effortless setup.
- Fully automated CI/CD pipelines and GitOps integration.
- Real-time metrics and logging for actionable insights.
Effortless Scalability and High Performance
- Auto-scaling to handle high concurrency with ease.
- Zero operational overhead — just focus on building.
Explore more in the Documentation!
Follow us on X: @LeapcellHQ