Managing Docker Images and Containers: A Comprehensive Guide
Docker is widely used for containerization, enabling efficient application deployment. This guide covers essential Docker commands and concepts related to managing images and containers, understanding interactive mode, viewing logs, handling file transfers, and optimizing workflows. Getting Help with Docker Docker provides built-in help for commands. To explore available options, simply run: docker --help For specific commands, use: docker --help This helps in discovering flags and additional functionalities. Start vs Run Command: Attached vs Detached Mode When working with containers, understanding how they run is crucial. Docker Run vs Docker Start docker run → Starts a new container in attached mode, meaning the terminal blocks while logs are printed. docker start → Starts an existing container in detached mode, meaning logs won’t be printed, and interaction isn’t possible. Running a Container in Detached Mode By default, docker run runs in attached mode, but you can force it into detached mode with: docker run -p 8000:80 -d Here: -p 8000:80 → Maps container's port 80 to host port 8000. -d → Runs the container without blocking the terminal. Attaching to a Running Container Even if a container is running in detached mode, you can attach to it anytime: docker container attach Viewing Logs for Containers To see logs, use: docker logs To continuously monitor logs in real-time: docker logs -f Here, -f ensures live log streaming in the terminal. Interactive Mode in Docker Containers Docker isn't just for web applications—it can also be used for interactive scripts. Practice Resource Example: Running a Python Script in Interactive Mode Consider a Python script (rng.py): from random import randint min_number = int(input('Please enter the min number: ')) max_number = int(input('Please enter the max number: ')) if max_number
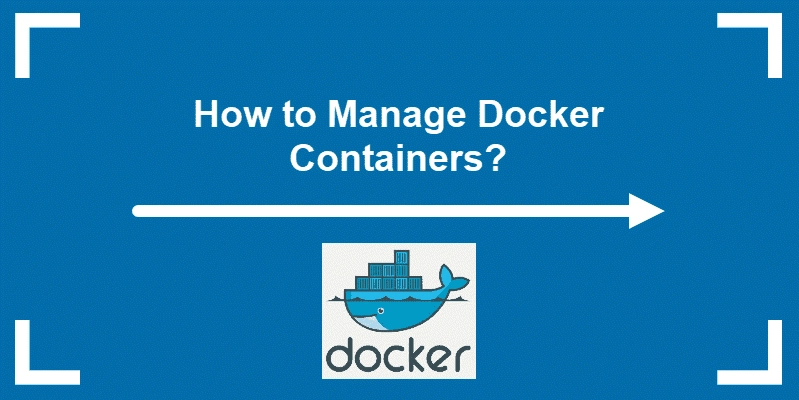
Docker is widely used for containerization, enabling efficient application deployment. This guide covers essential Docker commands and concepts related to managing images and containers, understanding interactive mode, viewing logs, handling file transfers, and optimizing workflows.
Getting Help with Docker
Docker provides built-in help for commands. To explore available options, simply run:
docker --help
For specific commands, use:
docker <command> --help
This helps in discovering flags and additional functionalities.
Start vs Run Command: Attached vs Detached Mode
When working with containers, understanding how they run is crucial.
Docker Run vs Docker Start
-
docker run
→ Starts a new container in attached mode, meaning the terminal blocks while logs are printed. -
docker start
→ Starts an existing container in detached mode, meaning logs won’t be printed, and interaction isn’t possible.
Running a Container in Detached Mode
By default, docker run
runs in attached mode, but you can force it into detached mode with:
docker run -p 8000:80 -d
Here:
-
-p 8000:80
→ Maps container's port 80 to host port 8000. -
-d
→ Runs the container without blocking the terminal.
Attaching to a Running Container
Even if a container is running in detached mode, you can attach to it anytime:
docker container attach
Viewing Logs for Containers
To see logs, use:
docker logs
To continuously monitor logs in real-time:
docker logs -f
Here, -f
ensures live log streaming in the terminal.
Interactive Mode in Docker Containers
Docker isn't just for web applications—it can also be used for interactive scripts.
Example: Running a Python Script in Interactive Mode
Consider a Python script (rng.py
):
from random import randint
min_number = int(input('Please enter the min number: '))
max_number = int(input('Please enter the max number: '))
if max_number < min_number:
print('Invalid input - shutting down...')
else:
rnd_number = randint(min_number, max_number)
print(rnd_number)
Dockerfile for the Python Script
FROM python
WORKDIR /app
COPY . /app
CMD ["python", "rng.py"]
Running the Container
Trying:
docker run
Would result in an error.
Instead, run in interactive mode:
docker run -i -t
Or equivalently:
docker run -it
Explanation of -i
and -t
Flags
-
-i
→ Interactive mode, allowing input from the terminal. -
-t
→ Tty (pseudo-terminal) mode, enabling a terminal interface within the container.
Starting an Interactive Container
By default, docker start
runs a container in detached mode.
To start in interactive mode, use:
docker start -a -i
Where:
-
-a
→ Attaches output to the terminal. -
-i
→ Allows input interaction.
Deleting Containers
Checking Running Containers
docker ps
Stopping a Running Container
docker stop
Removing Containers
docker rm
Automatically Removing Stopped Containers
Instead of manually cleaning up stopped containers, Docker provides the --rm
flag:
docker run -p 3000:80 -d --rm
-
--rm
ensures the container automatically gets deleted once it stops.
Copying Files To and From a Container
Copying Files INTO a Container
docker cp dummy/. :/test
This command:
- Copies all files from the local
dummy/
folder into/test
inside the container. - If
/test
doesn’t exist, Docker automatically creates it. - Use Case: Copy configuration files into a Docker container.
Copying Files FROM a Container
docker cp :/test /dummy
- Copies files from
/test
inside the container todummy/
on the local machine. - Use Case: Extract logs generated inside a container to the local system.
Naming and Tagging Containers & Images
Naming a Running Container
When starting a container, you can assign a custom name instead of a random identifier:
docker run -p 3000:80 --name my-container
Here:
-
--name my-container
assigns a human-friendly name to the container.
Naming (Tagging) an Image
Images can be tagged for easier identification:
docker build -t goals:latest .
Where:
-
-t goals:latest
tags the image as "goals" with version "latest".
To see all images:
docker images
Conclusion
-
Attached vs Detached Mode:
docker run
is attached by default,docker start
is detached. -
Interactive Mode:
-i
and-t
allow input/output within a container. -
Logs Management:
docker logs -f
provides real-time logs. -
File Transfers: Use
docker cp
to move files to/from a container. -
Container Cleanup:
docker rm
removes stopped containers,--rm
ensures automatic removal. -
Naming and Tagging: Use
--name
for containers and-t
for images.
By understanding these commands, managing Docker images and containers becomes effortless. Experiment with these concepts in real-world scenarios to strengthen your containerization expertise.