Java Day 3: Exploring Errors, Instances, Objects, and the Evolution of Java Programming
Java - Day 3: Understanding Errors, Security, and More Introduction Welcome to Day 3 of learning Java! Today, we'll cover key concepts like errors, security, memory management, JDBC, OOP vs Functional Programming, and Java versions. We'll also explore why machines understand only 0s and 1s, and how Java ensures security with bytecode verification. 1️⃣ Compilation vs Runtime Errors In Java, a program may compile successfully but fail to run if there are runtime errors. Example: Code Compiles but Does Not Run class Home { // No main method } ✅ Compiles Successfully ❌ Fails at Runtime because Java looks for a main() method to execute. Code with an Empty main() Method class Home { public static void main(String[] args) { // Empty main method } } ✅ Compiles Successfully ✅ Runs Successfully, but does nothing because main() is empty. 2️⃣ Platform Independence & JVM Architecture JDK - Platform Independent Java source code is written once and compiled into bytecode (.class files). This bytecode can run on any OS with a compatible JVM. JVM - Platform Dependent Each OS has its own version of JVM (Windows JVM, Linux JVM, etc.), making JVM platform dependent. The JVM translates bytecode into machine code that the OS can understand. 3️⃣ Java Security & Bytecode Verification Why is Java Secure? ✔ No Pointers – Prevents direct memory access. ✔ Bytecode Verification – Ensures no malicious bytecode is executed. ✔ Security Manager – Restricts unauthorized access to system resources. Bytecode Verification Process Java compiler converts source code into bytecode. JVM’s Class Loader loads the bytecode. Bytecode Verifier checks for security risks (e.g., invalid access). JVM executes the verified bytecode. 4️⃣ Memory Management & Garbage Collection Memory Management in Java Heap Memory: Stores objects. Stack Memory: Stores method calls and local variables. Garbage Collector (GC) - Automatic Memory Cleanup Daemon Thread: Runs in the background. Frees unused objects automatically, preventing memory leaks. 5️⃣ Why Java Doesn’t Use Pointers Java avoids pointers to prevent direct memory manipulation, reducing security risks. Instead, Java uses references, making code safer and easier to manage. 6️⃣ Binary & Decimal Conversion Binary to Decimal Conversion Example: Convert 1011 (binary) to decimal. (1 × 2³) + (0 × 2²) + (1 × 2¹) + (1 × 2⁰) = 8 + 0 + 2 + 1 = 11 1011 (binary) = 11 (decimal) Decimal to Binary Conversion Example: Convert 13 (decimal) to binary. 13 ÷ 2 = 6 remainder **1** 6 ÷ 2 = 3 remainder **0** 3 ÷ 2 = 1 remainder **1** 1 ÷ 2 = 0 remainder **1** 13 (decimal) = 1101 (binary) 7️⃣ Template in Java A template is a blueprint that allows reusable code structures. Java Generics are an example of templates: class Box { T item; void add(T item) { this.item = item; } } ✔ Allows Box and Box without rewriting code. 8️⃣ Error vs Exception vs Bug Term Definition Example Error A system-level failure, usually unrecoverable. OutOfMemoryError Exception A recoverable issue that can be handled. NullPointerException Bug A logical mistake by the programmer. Wrong calculation logic 9️⃣In Java, **"instance" and "object" are closely related but not exactly the same. 1️⃣ Object An object is a specific entity created from a class. It has identity (name), state (values), and behavior (methods). Objects are stored in heap memory when created. Example of Object class Car { String color; void drive() { System.out.println("Car is driving"); } } public class Main { public static void main(String[] args) { Car myCar = new Car(); // Object creation myCar.color = "Red"; myCar.drive(); } } ✔️ Here, myCar is an object of the Car class. 2️⃣ Instance An instance refers to a specific realization of a class in memory. When we create an object, we say "this is an instance of the class." Every object is an instance, but the term 'instance' emphasizes its existence in memory. Example of Instance Car car1 = new Car(); // Instance 1 Car car2 = new Car(); // Instance 2 ✔️ Here, car1 and car2 are instances of the Car class. Key Differences Between Instance and Object Feature Instance Object Definition A realized version of a class in memory. A physical entity created from a class. Focus Focuses on existence in memory. Focuses on behavior and attributes. Example "car1 is an instance of Car" "car1 is an object of Car" Relation Every object is an instance. Every instance is an object, but the focus is different.
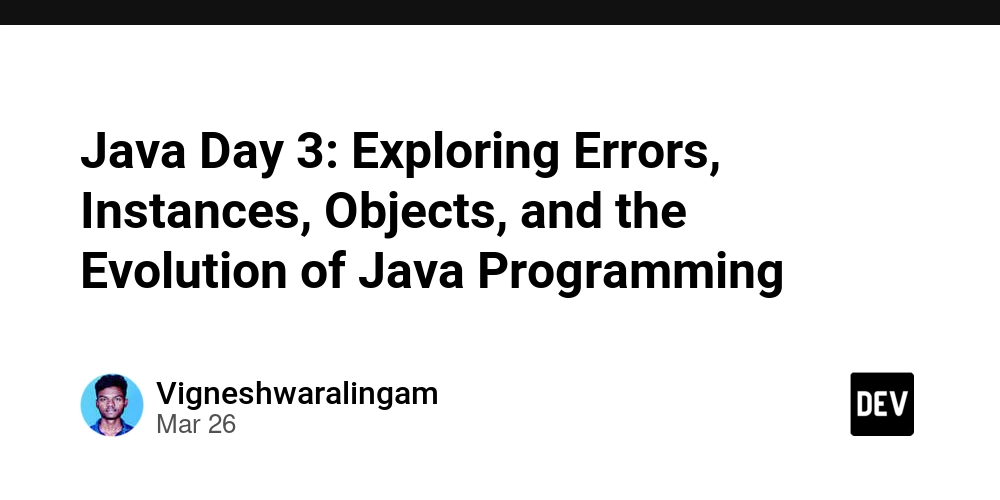
Java - Day 3: Understanding Errors, Security, and More
Introduction
Welcome to Day 3 of learning Java! Today, we'll cover key concepts like errors, security, memory management, JDBC, OOP vs Functional Programming, and Java versions. We'll also explore why machines understand only 0s and 1s, and how Java ensures security with bytecode verification.
1️⃣ Compilation vs Runtime Errors
In Java, a program may compile successfully but fail to run if there are runtime errors.
Example: Code Compiles but Does Not Run
class Home {
// No main method
}
✅ Compiles Successfully
❌ Fails at Runtime because Java looks for a main()
method to execute.
Code with an Empty main()
Method
class Home {
public static void main(String[] args) {
// Empty main method
}
}
✅ Compiles Successfully
✅ Runs Successfully, but does nothing because main()
is empty.
2️⃣ Platform Independence & JVM Architecture
JDK - Platform Independent
- Java source code is written once and compiled into bytecode (.class files).
- This bytecode can run on any OS with a compatible JVM.
JVM - Platform Dependent
- Each OS has its own version of JVM (Windows JVM, Linux JVM, etc.), making JVM platform dependent.
- The JVM translates bytecode into machine code that the OS can understand.
3️⃣ Java Security & Bytecode Verification
Why is Java Secure?
✔ No Pointers – Prevents direct memory access.
✔ Bytecode Verification – Ensures no malicious bytecode is executed.
✔ Security Manager – Restricts unauthorized access to system resources.
Bytecode Verification Process
- Java compiler converts source code into bytecode.
- JVM’s Class Loader loads the bytecode.
- Bytecode Verifier checks for security risks (e.g., invalid access).
- JVM executes the verified bytecode.
4️⃣ Memory Management & Garbage Collection
Memory Management in Java
- Heap Memory: Stores objects.
- Stack Memory: Stores method calls and local variables.
Garbage Collector (GC) - Automatic Memory Cleanup
- Daemon Thread: Runs in the background.
- Frees unused objects automatically, preventing memory leaks.
5️⃣ Why Java Doesn’t Use Pointers
- Java avoids pointers to prevent direct memory manipulation, reducing security risks.
- Instead, Java uses references, making code safer and easier to manage.
6️⃣ Binary & Decimal Conversion
Binary to Decimal Conversion
Example: Convert 1011
(binary) to decimal.
(1 × 2³) + (0 × 2²) + (1 × 2¹) + (1 × 2⁰) = 8 + 0 + 2 + 1 = 11
1011 (binary) = 11 (decimal)
Decimal to Binary Conversion
Example: Convert 13
(decimal) to binary.
13 ÷ 2 = 6 remainder **1**
6 ÷ 2 = 3 remainder **0**
3 ÷ 2 = 1 remainder **1**
1 ÷ 2 = 0 remainder **1**
13 (decimal) = 1101 (binary)
7️⃣ Template in Java
- A template is a blueprint that allows reusable code structures.
- Java Generics are an example of templates:
class Box<T> {
T item;
void add(T item) { this.item = item; }
}
✔ Allows Box
and Box
without rewriting code.
8️⃣ Error vs Exception vs Bug
Term | Definition | Example |
---|---|---|
Error | A system-level failure, usually unrecoverable. | OutOfMemoryError |
Exception | A recoverable issue that can be handled. | NullPointerException |
Bug | A logical mistake by the programmer. | Wrong calculation logic |
9️⃣In Java, **"instance" and "object" are closely related but not exactly the same.
1️⃣ Object
- An object is a specific entity created from a class.
- It has identity (name), state (values), and behavior (methods).
- Objects are stored in heap memory when created.
Example of Object
class Car {
String color;
void drive() {
System.out.println("Car is driving");
}
}
public class Main {
public static void main(String[] args) {
Car myCar = new Car(); // Object creation
myCar.color = "Red";
myCar.drive();
}
}
✔️ Here, myCar
is an object of the Car
class.
2️⃣ Instance
- An instance refers to a specific realization of a class in memory.
- When we create an object, we say "this is an instance of the class."
- Every object is an instance, but the term 'instance' emphasizes its existence in memory.
Example of Instance
Car car1 = new Car(); // Instance 1
Car car2 = new Car(); // Instance 2
✔️ Here, car1
and car2
are instances of the Car
class.
Key Differences Between Instance and Object
Feature | Instance | Object |
---|---|---|
Definition | A realized version of a class in memory. | A physical entity created from a class. |
Focus | Focuses on existence in memory. | Focuses on behavior and attributes. |
Example | "car1 is an instance of Car" |
"car1 is an object of Car" |
Relation | Every object is an instance. | Every instance is an object, but the focus is different. |
3️⃣ Another Example to Understand
class Student {
String name;
}
public class Main {
public static void main(String[] args) {
Student s1 = new Student(); // Instance/Object 1
Student s2 = new Student(); // Instance/Object 2
}
}
✅ s1
and s2
are both objects and instances of the Student
class.
Conclusion
✔️ "Object" focuses on the real-world entity and its behavior.
✔️ "Instance" emphasizes that the object exists in memory as a specific realization of a class.