Optimizing String Comparisons Using compareTo for Better Performance
String comparison is a fundamental operation in programming, used in sorting, searching, and organizing textual data. One of the most commonly used methods for comparing strings is the compareTo function. Efficient string comparisons are crucial for improving application performance, reducing computational overhead, and ensuring faster execution times. In this blog, we will explore how to optimize string comparisons using string compareTo, discuss its significance in various scenarios, and provide best practices for ensuring efficiency. Understanding String Comparison Before diving into optimization techniques, it is important to understand how string comparison works. The string compareTo method is used to compare two strings lexicographically based on their character values. This method follows a predefined order to determine whether one string is less than, equal to, or greater than another. Efficient string comparison is essential in applications that handle large amounts of textual data, such as databases, search engines, and data sorting algorithms. Poorly optimized comparisons can slow down execution and lead to unnecessary processing delays. Challenges in String Comparisons String comparisons can become computationally expensive when dealing with large data sets. Several factors impact the performance of string comparisons, including: String Length – Comparing long strings takes more processing time than shorter ones. Case Sensitivity – Depending on the application, case-sensitive comparisons may be required, increasing complexity. Encoding Issues – Unicode and special character handling can add overhead to comparisons. Data Volume – Comparing thousands or millions of strings in real-time can lead to performance bottlenecks. Memory Consumption – Inefficient comparison methods can lead to increased memory usage. Addressing these challenges requires careful optimization techniques to ensure smooth and fast execution. Best Practices for Optimizing String Comparisons Using compareTo 1. Minimize Unnecessary Comparisons One of the simplest ways to optimize string comparison operations is to minimize the number of comparisons performed. Instead of comparing every string in a data set, implement techniques like: Early termination in loops – If the required condition is met, break out of the loop instead of continuing unnecessary comparisons. Sorting before comparison – Sorting data in advance reduces the number of comparisons needed in certain operations. 2. Use Case-Insensitive Comparisons When Possible If case sensitivity is not a requirement, performing case-insensitive comparisons can speed up execution. Converting strings to a uniform case before comparison reduces complexity and ensures consistency. 3. Optimize for Short Strings First When comparing multiple strings, prioritizing shorter strings can improve efficiency. Since string compareTo compares characters sequentially, shorter strings are processed faster, allowing early termination when possible. 4. Leverage Indexing and Caching For applications that repeatedly compare the same strings, implementing indexing and caching mechanisms can prevent redundant computations. Storing previous results in a cache allows for faster retrieval and reduces processing time. 5. Use Efficient Data Structures Selecting the right data structure can significantly impact the performance of string comparisons. Consider using: Hash maps or dictionaries for quick lookups. Trie structures for efficient searching and comparison in large text-based applications. Binary trees for structured comparison and sorting operations. 6. Avoid Repetitive Computation When working with large text data, avoid unnecessary recalculations. Instead of recomputing string comparisons every time, store results and reuse them when needed. 7. Use Lazy Evaluation for Large Data Sets Lazy evaluation techniques allow comparisons to be deferred until absolutely necessary, reducing processing overhead. This approach is useful in scenarios where only a subset of comparisons needs to be evaluated. 8. Utilize Parallel Processing for Bulk Comparisons For applications that require extensive string comparisons, leveraging parallel processing can enhance performance. Distributing comparison operations across multiple threads or processors reduces execution time, making the system more efficient. Practical Applications of Optimized String Comparisons Optimizing string comparisons using string compareTo can be beneficial in various real-world applications, including: Database Query Optimization – Efficient string comparisons help improve query performance, reducing the time needed to filter and retrieve data. Search Engine Performance – Optimized comparisons ensure faster indexing and retrieval of search results. Sorting Algorithms – Many sor
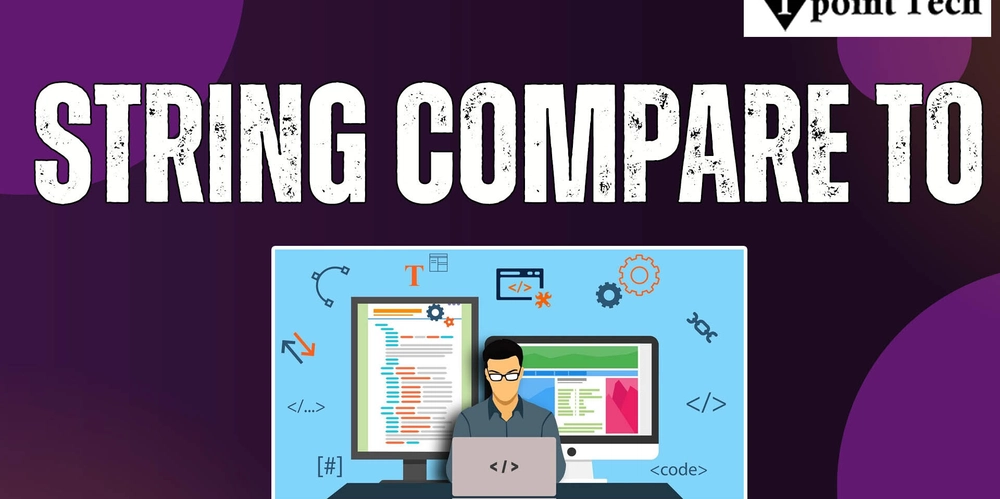
String comparison is a fundamental operation in programming, used in sorting, searching, and organizing textual data. One of the most commonly used methods for comparing strings is the compareTo
function. Efficient string comparisons are crucial for improving application performance, reducing computational overhead, and ensuring faster execution times.
In this blog, we will explore how to optimize string comparisons using string compareTo, discuss its significance in various scenarios, and provide best practices for ensuring efficiency.
Understanding String Comparison
Before diving into optimization techniques, it is important to understand how string comparison works. The string compareTo
method is used to compare two strings lexicographically based on their character values. This method follows a predefined order to determine whether one string is less than, equal to, or greater than another.
Efficient string comparison is essential in applications that handle large amounts of textual data, such as databases, search engines, and data sorting algorithms. Poorly optimized comparisons can slow down execution and lead to unnecessary processing delays.
Challenges in String Comparisons
String comparisons can become computationally expensive when dealing with large data sets. Several factors impact the performance of string comparisons, including:
- String Length – Comparing long strings takes more processing time than shorter ones.
- Case Sensitivity – Depending on the application, case-sensitive comparisons may be required, increasing complexity.
- Encoding Issues – Unicode and special character handling can add overhead to comparisons.
- Data Volume – Comparing thousands or millions of strings in real-time can lead to performance bottlenecks.
- Memory Consumption – Inefficient comparison methods can lead to increased memory usage.
Addressing these challenges requires careful optimization techniques to ensure smooth and fast execution.
Best Practices for Optimizing String Comparisons Using compareTo
1. Minimize Unnecessary Comparisons
One of the simplest ways to optimize string comparison operations is to minimize the number of comparisons performed. Instead of comparing every string in a data set, implement techniques like:
- Early termination in loops – If the required condition is met, break out of the loop instead of continuing unnecessary comparisons.
- Sorting before comparison – Sorting data in advance reduces the number of comparisons needed in certain operations.
2. Use Case-Insensitive Comparisons When Possible
If case sensitivity is not a requirement, performing case-insensitive comparisons can speed up execution. Converting strings to a uniform case before comparison reduces complexity and ensures consistency.
3. Optimize for Short Strings First
When comparing multiple strings, prioritizing shorter strings can improve efficiency. Since string compareTo
compares characters sequentially, shorter strings are processed faster, allowing early termination when possible.
4. Leverage Indexing and Caching
For applications that repeatedly compare the same strings, implementing indexing and caching mechanisms can prevent redundant computations. Storing previous results in a cache allows for faster retrieval and reduces processing time.
5. Use Efficient Data Structures
Selecting the right data structure can significantly impact the performance of string comparisons. Consider using:
- Hash maps or dictionaries for quick lookups.
- Trie structures for efficient searching and comparison in large text-based applications.
- Binary trees for structured comparison and sorting operations.
6. Avoid Repetitive Computation
When working with large text data, avoid unnecessary recalculations. Instead of recomputing string comparisons every time, store results and reuse them when needed.
7. Use Lazy Evaluation for Large Data Sets
Lazy evaluation techniques allow comparisons to be deferred until absolutely necessary, reducing processing overhead. This approach is useful in scenarios where only a subset of comparisons needs to be evaluated.
8. Utilize Parallel Processing for Bulk Comparisons
For applications that require extensive string comparisons, leveraging parallel processing can enhance performance. Distributing comparison operations across multiple threads or processors reduces execution time, making the system more efficient.
Practical Applications of Optimized String Comparisons
Optimizing string comparisons using string compareTo
can be beneficial in various real-world applications, including:
- Database Query Optimization – Efficient string comparisons help improve query performance, reducing the time needed to filter and retrieve data.
- Search Engine Performance – Optimized comparisons ensure faster indexing and retrieval of search results.
- Sorting Algorithms – Many sorting techniques rely on string comparisons for ordering textual data efficiently.
- Real-Time Data Processing – Applications that handle streaming data benefit from optimized comparisons to maintain speed and responsiveness.
- Machine Learning and Natural Language Processing (NLP) – Large-scale text analysis and pattern recognition depend on fast and efficient string comparisons.
Monitoring and Measuring Performance
To ensure that string comparisons are optimized, it is essential to monitor and measure performance using:
- Profiling tools to analyze execution times.
- Benchmarking techniques to compare different optimization strategies.
- Load testing to simulate real-world scenarios and identify bottlenecks.
Regular monitoring helps in identifying areas of improvement and maintaining optimal performance in applications relying on string comparisons.
Conclusion
String comparisons using string compareTo are fundamental operations in various applications, from database management to text processing. Optimizing these comparisons can lead to significant improvements in performance, reducing computational overhead and enhancing efficiency.
By following best practices such as minimizing unnecessary comparisons, leveraging caching, using efficient data structures, and utilizing parallel processing, developers can optimize string comparisons for better performance. Implementing these strategies ensures faster execution times and a more responsive application, making it essential for large-scale text processing and data management.
Whether you are working on search algorithms, database queries, or machine learning applications, optimizing string comparisons is a crucial step toward building high-performance software solutions. By continuously monitoring and refining your approach, you can achieve optimal results and improve overall application efficiency.