Optimizing Article URLs for Performance and SEO: Moving Beyond Slugs
When building content-heavy platforms like news websites, URL design plays a crucial role in both SEO and backend performance. Many legacy systems and modern CMSs still rely on slug-based URLs to uniquely identify and route to an article. While great for human readability and SEO, this approach can significantly slow down backend performance at scale. Let’s break down the issue and look at a more efficient alternative. The Problem with Slug-Based URLs Here’s a typical slug-based article URL: https://example.com/news/international/slug-title-goes-here In systems using this approach: The slug (string in the URL) is used to find the article in the database. This typically results in full-text or indexed searches, which can be costly in terms of performance as the data grows. If the slug changes (e.g., after a headline update), old links can break or will result in 404 for the indexed urls. The Solution: Timestamp-Based Numeric IDs To solve this, we moved to a hybrid URL structure using both slug and a 10-digit unique numeric ID appended at the end. Example: https://example.com/news/international/slug-title-1712659384 Here’s how it works: The last segment (1712659384) is a 10-digit numeric ID, generated using the Unix timestamp (in seconds). This ID serves as the primary key to fetch the article directly from the database. The slug is optional for SEO and user readability, but article lookup relies only on the ID. Why This Works Fast Lookups: Numeric ID allows for fast, indexed database queries (e.g., by _id or article_id). SEO-Friendly: Slugs still exist for users and search engines, but aren’t part of the lookup logic. Stable URLs: Even if the title or slug changes, the article ID ensures the URL remains valid. Multiple Access Points: Articles can be accessed from various URLs with different slugs, as long as the ID remains the same. Canonical Tag Usage: To avoid SEO penalties for duplicate content across different slug variations, set a canonical URL using the original permalink. Time-Encoded: Using timestamps ensures uniqueness per second and gives an approximate idea of when the article was created. Implementation Tips ID Generation: const articleId = Math.floor(Date.now() / 1000); // 10-digit Unix timestamp URL Structure: const permalink =/news/${slug}-${articleId}; Backend Routing: Extract the numeric ID from the URL using regex: const id = req.params.slugOrId.match(/(\d{10})$/)[1]; Fallback Logic: If ID is not found, optionally fallback to slug-based search (but cache it). SEO Best Practices: Always include the original permalink in a canonical tag to consolidate SEO ranking to a single source. Final Thoughts This small shift in URL design can dramatically improve performance and maintain SEO benefits. It’s especially powerful for high-traffic sites like news platforms where quick and reliable article lookup is critical. Have you tried similar strategies in your app or CMS? Let’s discuss in the comments!
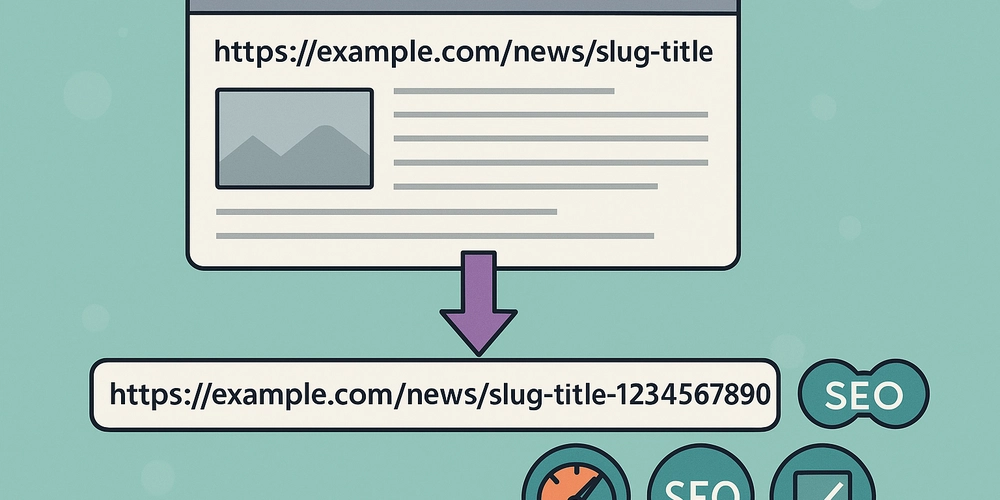
When building content-heavy platforms like news websites, URL design plays a crucial role in both SEO and backend performance. Many legacy systems and modern CMSs still rely on slug-based URLs to uniquely identify and route to an article. While great for human readability and SEO, this approach can significantly slow down backend performance at scale.
Let’s break down the issue and look at a more efficient alternative.
The Problem with Slug-Based URLs
Here’s a typical slug-based article URL:
https://example.com/news/international/slug-title-goes-here
In systems using this approach:
The slug (string in the URL) is used to find the article in the database.
This typically results in full-text or indexed searches, which can be costly in terms of performance as the data grows.
If the slug changes (e.g., after a headline update), old links can break or will result in 404 for the indexed urls.
The Solution: Timestamp-Based Numeric IDs
To solve this, we moved to a hybrid URL structure using both slug and a 10-digit unique numeric ID appended at the end. Example:
https://example.com/news/international/slug-title-1712659384
Here’s how it works:
- The last segment (1712659384) is a 10-digit numeric ID, generated using the Unix timestamp (in seconds).
- This ID serves as the primary key to fetch the article directly from the database.
- The slug is optional for SEO and user readability, but article lookup relies only on the ID.
Why This Works
- Fast Lookups: Numeric ID allows for fast, indexed database queries (e.g., by _id or article_id).
- SEO-Friendly: Slugs still exist for users and search engines, but aren’t part of the lookup logic.
- Stable URLs: Even if the title or slug changes, the article ID ensures the URL remains valid.
- Multiple Access Points: Articles can be accessed from various URLs with different slugs, as long as the ID remains the same.
-
Canonical Tag Usage: To avoid SEO penalties for duplicate content across different slug variations, set a canonical URL using the original permalink.
- Time-Encoded: Using timestamps ensures uniqueness per second and gives an approximate idea of when the article was created.
Implementation Tips
- ID Generation:
const articleId = Math.floor(Date.now() / 1000); // 10-digit Unix timestamp
- URL Structure:
const permalink =
/news/${slug}-${articleId};
- Backend Routing: Extract the numeric ID from the URL using regex:
const id = req.params.slugOrId.match(/(\d{10})$/)[1];
Fallback Logic:
If ID is not found, optionally fallback to slug-based search (but cache it).SEO Best Practices:
Always include the original permalink in a canonical tag to consolidate SEO ranking to a single source.
Final Thoughts
This small shift in URL design can dramatically improve performance and maintain SEO benefits. It’s especially powerful for high-traffic sites like news platforms where quick and reliable article lookup is critical.
Have you tried similar strategies in your app or CMS? Let’s discuss in the comments!