Next.js vs PHP: Real Code Comparison for Web Applications
Leapcell: The Best of Serverless Web Hosting In-depth Comparison of Nextjs and PHP in Web Business Development In the field of web development, Nextjs and PHP are two commonly used technical solutions. Nextjs is a front-end framework based on React, providing powerful page rendering and data processing capabilities; while writing business code directly in PHP pages does not require relying on a framework and can quickly implement dynamic web page functions. This article will conduct an in-depth comparison of the performance of Nextjs and PHP in web business development from multiple dimensions such as business logic implementation, data processing, and page interaction, combined with actual code examples. I. Business Logic Infrastructure 1.1 Business Logic Architecture of Nextjs Nextjs adopts a component-based development model, splitting pages into multiple independent React components, and each component is responsible for specific business logic. The project structure takes the pages folder as the core, and the files in it correspond to different routing pages. For example, to create a simple blog list page, write the following code in pages/blog.js: import React from'react'; const Blog = () => { // Business logic-related states and functions can be defined here return ( Blog List {/* Code to display the blog list... */} ); }; export default Blog; This component-based architecture makes the code highly reusable and maintainable. Different business modules can be encapsulated into independent components, which is convenient to be used in multiple pages. 1.2 Business Logic Architecture of PHP In PHP, business logic is usually written directly in .php files, and a file may contain a mixture of HTML, PHP code, and CSS styles. Taking the creation of a simple user login page as an example, the content of the login.php file is as follows: User Login
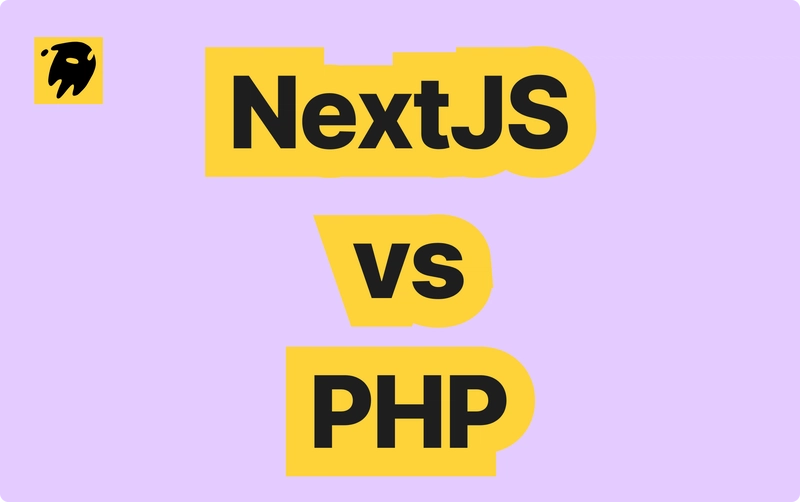
Leapcell: The Best of Serverless Web Hosting
In-depth Comparison of Nextjs and PHP in Web Business Development
In the field of web development, Nextjs and PHP are two commonly used technical solutions. Nextjs is a front-end framework based on React, providing powerful page rendering and data processing capabilities; while writing business code directly in PHP pages does not require relying on a framework and can quickly implement dynamic web page functions. This article will conduct an in-depth comparison of the performance of Nextjs and PHP in web business development from multiple dimensions such as business logic implementation, data processing, and page interaction, combined with actual code examples.
I. Business Logic Infrastructure
1.1 Business Logic Architecture of Nextjs
Nextjs adopts a component-based development model, splitting pages into multiple independent React components, and each component is responsible for specific business logic. The project structure takes the pages
folder as the core, and the files in it correspond to different routing pages. For example, to create a simple blog list page, write the following code in pages/blog.js
:
import React from'react';
const Blog = () => {
// Business logic-related states and functions can be defined here
return (
<div>
<h1>Blog Listh1>
{/* Code to display the blog list... */}
div>
);
};
export default Blog;
This component-based architecture makes the code highly reusable and maintainable. Different business modules can be encapsulated into independent components, which is convenient to be used in multiple pages.
1.2 Business Logic Architecture of PHP
In PHP, business logic is usually written directly in .php
files, and a file may contain a mixture of HTML, PHP code, and CSS styles. Taking the creation of a simple user login page as an example, the content of the login.php
file is as follows:
User Login
This hybrid architecture of PHP pages is relatively straightforward when dealing with simple businesses. However, as the business complexity increases, the readability and maintainability of the code will be affected.
II. Data Processing and Interaction
2.1 Data Processing in Nextjs
Nextjs provides functions such as getStaticProps
and getServerSideProps
to handle data fetching. When it is necessary to obtain and display the blog post list from the backend API, the following code can be written in pages/blog.js
:
export async function getServerSideProps() {
const res = await fetch('https://api.example.com/blogs');
const blogs = await res.json();
return {
props: {
blogs
}
};
}
const Blog = ({ blogs }) => {
return (
<div>
<h1>Blog Listh1>
<ul>
{blogs.map((blog) => (
<li key={blog.id}>{blog.title}li>
))}
ul>
div>
);
};
export default Blog;
In terms of client-side interaction, Nextjs relies on React's state management mechanisms, such as hook functions like useState
and useReducer
, to achieve dynamic data updates. For example, to add a search function to the blog list:
import React, { useState } from'react';
export async function getServerSideProps() {
const res = await fetch('https://api.example.com/blogs');
const blogs = await res.json();
return {
props: {
blogs
}
};
}
const Blog = ({ blogs }) => {
const [searchTerm, setSearchTerm] = useState('');
const filteredBlogs = blogs.filter((blog) =>
blog.title.toLowerCase().includes(searchTerm.toLowerCase())
);
return (
<div>
<input
type="text"
placeholder="Search Blogs"
value={searchTerm}
onChange={(e) => setSearchTerm(e.target.value)}
/>
<h1>Blog Listh1>
<ul>
{filteredBlogs.map((blog) => (
<li key={blog.id}>{blog.title}li>
))}
ul>
div>
);
};
export default Blog;
2.2 Data Processing in PHP
PHP mainly processes data by interacting with the database. Taking the MySQL database as an example, use the mysqli
extension to obtain the blog post list and display it on the page. The code of blog.php
is as follows:
$servername = "localhost";
$username = "root";
$password = "";
$dbname = "myblog";
// Create a connection
$conn = new mysqli($servername, $username, $password, $dbname);
// Check the connection
if ($conn->connect_error) {
die("Connection Failed: ". $conn->connect_error);
}
$sql = "SELECT id, title FROM blogs";
$result = $conn->query($sql);
if ($result->num_rows > 0) {
echo "Blog List
"
;
while($row = $result->fetch_assoc()) {
echo "" . $row["title"]. "";
}
echo "";
} else {
echo "No Blog Posts Found";
}
$conn->close();
?>
When processing user-submitted data, PHP obtains the data through superglobal variables such as $_POST
and $_GET
. For example, to add a search function to the above blog list:
$servername = "localhost";
$username = "root";
$password = "";
$dbname = "myblog";
// Create a connection
$conn = new mysqli($servername, $username, $password, $dbname);
// Check the connection
if ($conn->connect_error) {
die("Connection Failed: ". $conn->connect_error);
}
$searchTerm = isset($_GET["search"])?. $_GET["search"] : "";
$sql = "SELECT id, title FROM blogs WHERE title LIKE '%$searchTerm%'";
$result = $conn->query($sql);
if ($result->num_rows > 0) {
echo "Blog List
"
;
while($row = $result->fetch_assoc()) {
echo "" . $row["title"]. "";
}
echo "";
} else {
echo "No Blog Posts Found";
}
$conn->close();
?>
However, this method of directly concatenating SQL statements has the risk of SQL injection, and strict data filtering and parameterized queries are required in actual development.
III. Page Rendering and Update
3.1 Page Rendering in Nextjs
Nextjs supports multiple rendering modes. In the Static Site Generation (SSG) mode, the getStaticProps
function will run during the build process, rendering the page into a static HTML file. For example:
export async function getStaticProps() {
const res = await fetch('https://api.example.com/blogs');
const blogs = await res.json();
return {
props: {
blogs
},
revalidate: 60 // Revalidate the data every 60 seconds
};
}
const Blog = ({ blogs }) => {
return (
<div>
<h1>Blog Listh1>
<ul>
{blogs.map((blog) => (
<li key={blog.id}>{blog.title}li>
))}
ul>
div>
);
};
export default Blog;
In the Server-Side Rendering (SSR) mode, the getServerSideProps
function runs on each request, fetching data in real-time and rendering the page. Client-Side Rendering (CSR) is to dynamically update the page content through JavaScript after the page has been loaded.
3.2 Page Rendering in PHP
PHP dynamically generates HTML pages on the server side and then returns them to the client. When the page data changes, the page needs to be refreshed to see the updated content. For example, for the above blog list page, each search or data update requires reloading the entire page, which results in a relatively poor user experience. However, PHP can also achieve partial page refresh through AJAX technology to improve the user experience. For example, use the AJAX function of jQuery to achieve partial refresh of the blog list:
Blog List
$(document).ready(function() {
$('#search-form').submit(function(event) {
event.preventDefault();
const searchTerm = $('#search').val();
$.ajax({
type: 'GET',
url: 'blog.php',
data: { search: searchTerm },
success: function(response) {
$('#blog-list').html(response);
},
error: function() {
console.log('Request Failed');
}
});
});
});
id="blog-list">
connect_error) {
die("Connection Failed: ". $conn->connect_error);
}
$searchTerm = isset($_GET["search"])?. $_GET["search"] : "";
$sql = "SELECT id, title FROM blogs WHERE title LIKE '%$searchTerm%'";
$result = $conn->query($sql);
if ($result->num_rows > 0) {
echo "Blog List
";
while($row = $result->fetch_assoc()) {
echo "- ". $row["title"]. "
";
}
echo "
";
} else {
echo "No Blog Posts Found";
}
$conn->close();
?>
IV. Error Handling and Debugging
4.1 Error Handling and Debugging in Nextjs
In Nextjs, React's Error Boundaries mechanism can catch errors in child components and handle them. For example, create an error boundary component:
class ErrorBoundary extends React.Component {
constructor(props) {
super(props);
this.state = { hasError: false };
}
static getDerivedStateFromError(error) {
return { hasError: true };
}
componentDidCatch(error, errorInfo) {
console.log(error, errorInfo);
}
render() {
if (this.state.hasError) {
return <div>An Error Occurred. Please Try Again Later.div>;
}
return this.props.children;
}
}
export default ErrorBoundary;
Use this component in the page:
import React from'react';
import ErrorBoundary from './ErrorBoundary';
const Blog = () => {
// Assume there is code here that may cause an error...
return (
<ErrorBoundary>
<div>
<h1>Blog Listh1>
div>
ErrorBoundary>
);
};
export default Blog;
In terms of debugging, Nextjs supports breakpoint debugging in the browser developer tools, and with the help of React DevTools, it is convenient to view the state and Props of components.
4.2 Error Handling and Debugging in PHP
PHP uses try...catch
statement blocks for error handling, and at the same time, the error reporting level can be set through the error_reporting
and ini_set
functions. For example:
try {
$servername = "localhost";
$username = "root";
$password = "";
$dbname = "myblog";
// Create a connection
$conn = new mysqli($servername, $username, $password, $dbname);
// Check the connection
if ($conn->connect_error) {
throw new Exception("Connection Failed: ". $conn->connect_error);
}
// Perform database operations...
$conn->close();
} catch (Exception $e) {
echo "Error: ". $e->getMessage();
}
When debugging, developers can use functions such as var_dump
and print_r
to output the contents of variables, and can also use debugging tools such as Xdebug for breakpoint debugging.
V. Security
5.1 Security in Nextjs
In terms of security, Nextjs requires developers to strictly verify and filter input data to prevent XSS attacks. When interacting with the backend API, it is necessary to ensure that the API's authentication and authorization mechanisms are reliable to prevent data leakage. At the same time, use the HTTPS protocol to transmit data to avoid the theft of sensitive information.
5.2 Security in PHP
PHP faces more challenges in terms of security. In addition to common SQL injection attacks, there may also be vulnerabilities such as file inclusion vulnerabilities and command execution vulnerabilities. To prevent SQL injection, parameterized queries should be used. For example, use the prepared statements of mysqli
:
$servername = "localhost";
$username = "root";
$password = "";
$dbname = "myblog";
// Create a connection
$conn = new mysqli($servername, $username, $password, $dbname);
// Check the connection
if ($conn->connect_error) {
die("Connection Failed: ". $conn->connect_error);
}
$searchTerm = isset($_GET["search"])?. $_GET["search"] : "";
$sql = "SELECT id, title FROM blogs WHERE title LIKE?";
$stmt = $conn->prepare($sql);
$stmt->bind_param("s", $searchTerm);
$stmt->execute();
$result = $stmt->get_result();
if ($result->num_rows > 0) {
echo "Blog List
"
;
while($row = $result->fetch_assoc()) {
echo "" . $row["title"]. "";
}
echo "";
} else {
echo "No Blog Posts Found";
}
$stmt->close();
$conn->close();
In addition, strictly verify and filter user-uploaded files to avoid file inclusion vulnerabilities; strictly check user-entered commands to prevent command execution vulnerabilities.
VI. Maintainability and Extensibility
6.1 Maintainability and Extensibility of Nextjs
Nextjs's component-based architecture and clear project structure make the code highly maintainable. When business requirements change, it can be achieved by modifying or adding new components. At the same time, the Nextjs ecosystem is rich, with a large number of third-party libraries and plugins available for use, making it convenient to expand functions. For example, to add a complex chart display function, libraries such as react-chartjs-2
can be introduced.
6.2 Maintainability and Extensibility of PHP
Writing business code directly in PHP pages will make the code lengthy and chaotic as the project scale increases, reducing its maintainability. In terms of extensibility, although PHP has rich extension libraries, due to the unclear code structure, a large number of modifications may be required to the original code when adding new functions, increasing the development cost and the risk of errors.
VII. Conclusion
Nextjs and writing business code directly in PHP pages each have their own characteristics. Nextjs is suitable for building modern and highly interactive web applications, and it performs excellently in performance optimization, component reuse, and development efficiency, but it requires a higher level of front-end technology stack from developers; PHP, with its simple and direct approach, has certain advantages in quickly building dynamic web pages and handling traditional business logic. However, developers need to be more cautious in terms of code maintainability and security. When choosing, developers should comprehensively consider factors such as the specific requirements of the project, the team's technical capabilities, and future extensibility to select the most suitable technical solution for web business development.
Leapcell: The Best of Serverless Web Hosting
Finally, I would like to recommend a platform that is most suitable for deploying Nodejs services: Leapcell