Mastering Dart Operators!
When working with Dart in Flutter, operators make our code cleaner, more efficient, and expressive! Let's explore some of the most useful ones with examples. 1️⃣ Null-aware operators (??, ??=, ?.) Dart makes handling null values easy: String? name; print(name ?? 'Guest'); // ➡️ 'Guest' (uses default if null) name ??= 'John'; // Assigns 'John' only if name is null print(name); // ➡️ 'John' int? length = name?.length; // Safe null access print(length); // ➡️ 4 2️⃣ Spread (...) and Null-aware Spread (...?) Great for working with collections: List numbers = [1, 2, 3]; List? nullableList; List allNumbers = [0, ...numbers, 4, ...?nullableList]; print(allNumbers); // ➡️ [0, 1, 2, 3, 4] 3️⃣ Cascade (..) Operator Used for chaining method calls: final controller = TextEditingController() ..text = "Hello, Flutter!" ..selection = TextSelection.collapsed(offset: 5); 4️⃣ Ternary (? :) & Null-aware Conditional (??) Simplifies conditional expressions: int age = 18; String status = age >= 18 ? 'Adult' : 'Minor'; print(status); // ➡️ 'Adult' 5️⃣ Null Check (!) Operator Used when you're sure a value isn’t null: String? nullableString = "Dart"; String nonNullable = nullableString!; print(nonNullable.length); // ➡️ 4 Operators make Dart code cleaner, safer, and more readable—a must for any Flutter Developer!
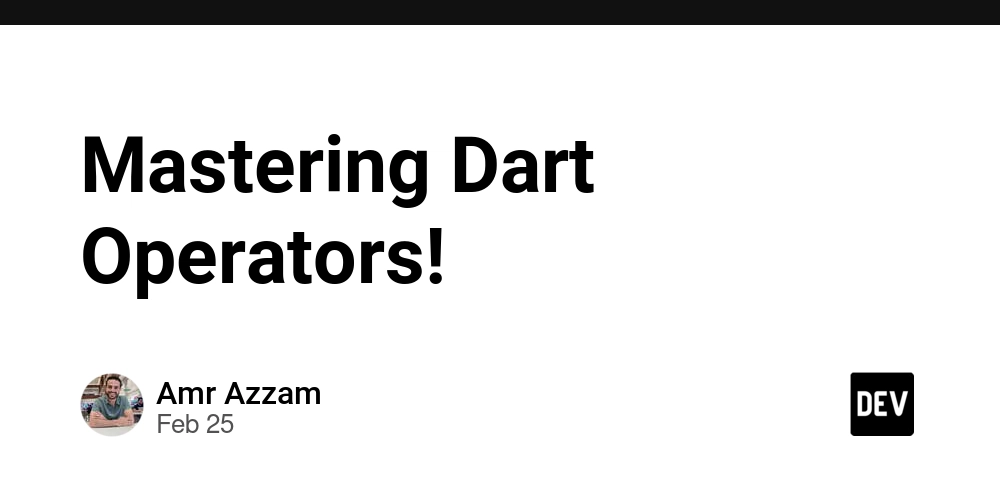
When working with Dart in Flutter, operators make our code cleaner, more efficient, and expressive!
Let's explore some of the most useful ones with examples.
1️⃣ Null-aware operators (??, ??=, ?.)
Dart makes handling null values easy:
String? name;
print(name ?? 'Guest'); // ➡️ 'Guest' (uses default if null)
name ??= 'John'; // Assigns 'John' only if name is null
print(name); // ➡️ 'John'
int? length = name?.length; // Safe null access
print(length); // ➡️ 4
2️⃣ Spread (...) and Null-aware Spread (...?)
Great for working with collections:
List numbers = [1, 2, 3];
List? nullableList;
List allNumbers = [0, ...numbers, 4, ...?nullableList];
print(allNumbers); // ➡️ [0, 1, 2, 3, 4]
3️⃣ Cascade (..) Operator
Used for chaining method calls:
final controller = TextEditingController()
..text = "Hello, Flutter!"
..selection = TextSelection.collapsed(offset: 5);
4️⃣ Ternary (? :) & Null-aware Conditional (??)
Simplifies conditional expressions:
int age = 18;
String status = age >= 18 ? 'Adult' : 'Minor';
print(status); // ➡️ 'Adult'
5️⃣ Null Check (!) Operator
Used when you're sure a value isn’t null:
String? nullableString = "Dart";
String nonNullable = nullableString!;
print(nonNullable.length); // ➡️ 4
Operators make Dart code cleaner, safer, and more readable—a must for any Flutter Developer!