Introduction to React Native for Mobile Development
Introduction to React Native for Mobile Development React Native has revolutionized mobile app development by allowing developers to build cross-platform applications using JavaScript and React. Whether you're a web developer looking to expand your skill set or a mobile developer seeking a more efficient workflow, React Native offers a powerful solution. In this guide, we'll explore the fundamentals of React Native, its advantages, and how you can get started. Plus, if you're looking to monetize your web programming skills, check out MillionFormula for opportunities to turn your expertise into income. What is React Native? React Native is an open-source framework developed by Facebook (now Meta) that enables developers to build native mobile apps using JavaScript and React. Unlike hybrid frameworks that rely on WebView, React Native compiles to native components, providing near-native performance. Key features of React Native include: Cross-platform development – Write once, deploy on both iOS and Android. Hot Reloading – See changes instantly without recompiling. Native Performance – Uses native UI components instead of web-based rendering. Large Ecosystem – Access to third-party libraries via npm. Why Choose React Native? 1. Faster Development Since React Native allows code reuse between iOS and Android, development time is significantly reduced. Companies like Facebook, Instagram, and Airbnb have used React Native to accelerate their mobile app development. 2. Strong Community Support With a thriving community, React Native has extensive documentation, tutorials, and third-party libraries. The React Native GitHub repository is actively maintained, ensuring continuous improvements. 3. Cost-Effective Instead of maintaining two separate codebases (Swift/Kotlin for iOS/Android), a single React Native app can serve both platforms, reducing development costs. Setting Up React Native To get started, you'll need: Node.js (Download here) npm (Comes with Node.js) Expo CLI (For simplified development) or React Native CLI (For more control) Installation Using Expo CLI (Recommended for Beginners) bash Copy npm install -g expo-cli expo init MyFirstApp cd MyFirstApp npm start Using React Native CLI bash Copy npx react-native init MyFirstApp cd MyFirstApp npx react-native run-android # or run-ios Building Your First React Native App Let’s create a simple counter app to understand React Native’s core concepts. Step 1: Create a New Component jsx Copy import React, { useState } from 'react'; import { View, Text, Button, StyleSheet } from 'react-native'; const CounterApp = () => { const [count, setCount] = useState(0); return ( Count: {count} setCount(count + 1)} /> setCount(count - 1)} /> ); }; const styles = StyleSheet.create({ container: { flex: 1, justifyContent: 'center', alignItems: 'center', }, text: { fontSize: 24, marginBottom: 20, }, }); export default CounterApp; Step 2: Run the App If using Expo: bash Copy expo start If using React Native CLI: bash Copy npx react-native run-android # or run-ios React Native vs. Other Frameworks Feature React Native Flutter Ionic Language JavaScript Dart HTML/CSS/JS Performance Near-native High Web-based Learning Curve Moderate Steep Easy Community Large Growing Mature While Flutter offers strong performance, React Native remains a favorite due to its JavaScript foundation and React compatibility. Best Practices for React Native Development Use Functional Components & Hooks – Modern React Native development favors functional components with hooks like useState and useEffect. Optimize Performance – Avoid unnecessary re-renders with React.memo and useCallback. Leverage Native Modules – For complex features (e.g., camera access), use native modules. Test on Real Devices – Emulators are great, but real-world testing ensures better UX. Monetizing Your React Native Skills If you're proficient in React Native, you can freelance, build SaaS products, or teach others. For developers looking to maximize earnings from their web development skills, MillionFormula offers valuable insights and strategies. Conclusion React Native is a powerful tool for mobile app development, combining the flexibility of JavaScript with the performance of native apps. Whether you're building a startup MVP or a large-scale application, React Native provides the efficiency and scalability needed. Start experimenting with React Native today, and explore new ways to monetize your expertise. Happy coding!
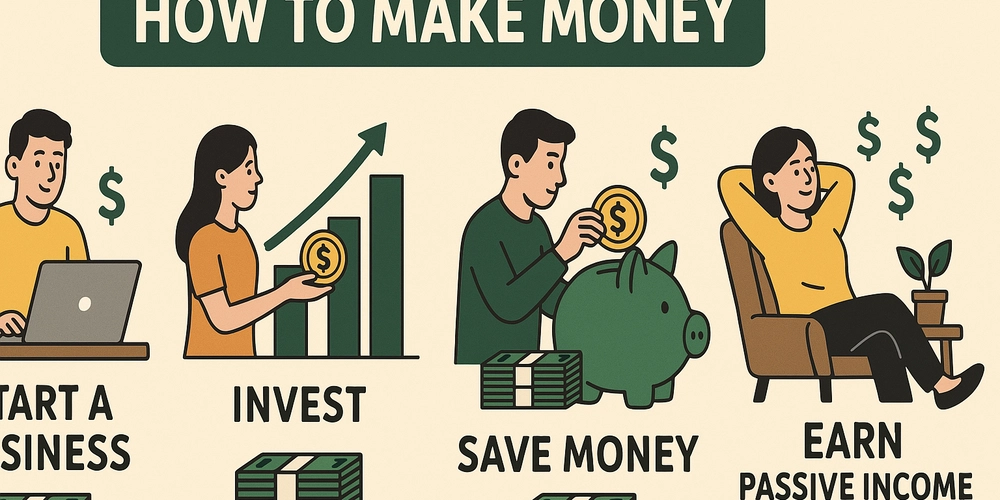
Introduction to React Native for Mobile Development
React Native has revolutionized mobile app development by allowing developers to build cross-platform applications using JavaScript and React. Whether you're a web developer looking to expand your skill set or a mobile developer seeking a more efficient workflow, React Native offers a powerful solution.
In this guide, we'll explore the fundamentals of React Native, its advantages, and how you can get started. Plus, if you're looking to monetize your web programming skills, check out MillionFormula for opportunities to turn your expertise into income.
What is React Native?
React Native is an open-source framework developed by Facebook (now Meta) that enables developers to build native mobile apps using JavaScript and React. Unlike hybrid frameworks that rely on WebView, React Native compiles to native components, providing near-native performance.
Key features of React Native include:
- Cross-platform development – Write once, deploy on both iOS and Android.
- Hot Reloading – See changes instantly without recompiling.
- Native Performance – Uses native UI components instead of web-based rendering.
- Large Ecosystem – Access to third-party libraries via npm.
Why Choose React Native?
1. Faster Development
Since React Native allows code reuse between iOS and Android, development time is significantly reduced. Companies like Facebook, Instagram, and Airbnb have used React Native to accelerate their mobile app development.
2. Strong Community Support
With a thriving community, React Native has extensive documentation, tutorials, and third-party libraries. The React Native GitHub repository is actively maintained, ensuring continuous improvements.
3. Cost-Effective
Instead of maintaining two separate codebases (Swift/Kotlin for iOS/Android), a single React Native app can serve both platforms, reducing development costs.
Setting Up React Native
To get started, you'll need:
- Node.js (Download here)
- npm (Comes with Node.js)
- Expo CLI (For simplified development) or React Native CLI (For more control)
Installation
Using Expo CLI (Recommended for Beginners)
bash
Copy
npm install -g expo-cli expo init MyFirstApp cd MyFirstApp npm start
Using React Native CLI
bash
Copy
npx react-native init MyFirstApp cd MyFirstApp npx react-native run-android # or run-ios
Building Your First React Native App
Let’s create a simple counter app to understand React Native’s core concepts.
Step 1: Create a New Component
jsx
Copy
import React, { useState } from 'react'; import { View, Text, Button, StyleSheet } from 'react-native'; const CounterApp = () => { const [count, setCount] = useState(0); return ( <View style={styles.container}> <Text style={styles.text}>Count: {count}Text> <Button title="Increment" onPress={() => setCount(count + 1)} /> <Button title="Decrement" onPress={() => setCount(count - 1)} /> View> ); }; const styles = StyleSheet.create({ container: { flex: 1, justifyContent: 'center', alignItems: 'center', }, text: { fontSize: 24, marginBottom: 20, }, }); export default CounterApp;
Step 2: Run the App
If using Expo:
bash
Copy
expo start
If using React Native CLI: bash Copy
npx react-native run-android # or run-ios
React Native vs. Other Frameworks
Feature | React Native | Flutter | Ionic |
---|---|---|---|
Language | JavaScript | Dart | HTML/CSS/JS |
Performance | Near-native | High | Web-based |
Learning Curve | Moderate | Steep | Easy |
Community | Large | Growing | Mature |
While Flutter offers strong performance, React Native remains a favorite due to its JavaScript foundation and React compatibility.
Best Practices for React Native Development
-
Use Functional Components & Hooks – Modern React Native development favors functional components with hooks like
useState
anduseEffect
. -
Optimize Performance – Avoid unnecessary re-renders with
React.memo
anduseCallback
. - Leverage Native Modules – For complex features (e.g., camera access), use native modules.
- Test on Real Devices – Emulators are great, but real-world testing ensures better UX.
Monetizing Your React Native Skills
If you're proficient in React Native, you can freelance, build SaaS products, or teach others. For developers looking to maximize earnings from their web development skills, MillionFormula offers valuable insights and strategies.
Conclusion
React Native is a powerful tool for mobile app development, combining the flexibility of JavaScript with the performance of native apps. Whether you're building a startup MVP or a large-scale application, React Native provides the efficiency and scalability needed.
Start experimenting with React Native today, and explore new ways to monetize your expertise. Happy coding!