Calm in Code: Building an Interactive Breathing Exercise Web App
Ever feel like your coding sessions leave you tense and stressed? I've been there too! That's why I created a breathing exercise web application that helps developers (and everyone else) practice mindful breathing techniques with visual guidance. In this post, I'll walk through how I built this app using vanilla JavaScript, CSS animations, and a clean, responsive design. Whether you're looking to build something similar or just want to add some interactive animations to your projects, there's something here for you! Demo You can try the live app here: Breathing Exercise Guide The Why Behind the App As developers, we often forget to breathe properly while focusing intensely on debugging or building new features. Research shows that controlled breathing can: Reduce mental fatigue and stress Improve problem-solving abilities Increase focus and concentration Lower physical tension from long coding sessions Each breathing pattern in the app serves a specific purpose: 4-7-8 Breathing: Great for rapid relaxation and breaking stress cycles Box Breathing: Enhances focus and clarity (used by Navy SEALs!) Diaphragmatic Breathing: Improves oxygen flow and reduces shallow breathing habits App Features Here's what our breathing app includes: Multiple breathing patterns with different timing sequences Three difficulty levels to accommodate users of all experience levels Visual animations that expand and contract with breathing rhythm A countdown timer for each breathing phase Session tracking to monitor practice time Dark mode toggle for reduced eye strain (especially important for night coding!) The HTML Structure Let's start with the HTML backbone of our application: Breathing Exercise Guide Breathing Guide 4-7-8 Breathing Box Breathing Diaphragmatic Beginner Intermediate Advanced Start Toggle Dark Mode Breathe In 4 Session: 0:00 The HTML structure is intentionally simple, with: A control section for selecting patterns and difficulty levels A central breathing circle that provides visual guidance Text instructions and a countdown timer A progress tracking section CSS: Bringing the Breath to Life The visual magic happens in the CSS: * { margin: 0; padding: 0; box-sizing: border-box; font-family: 'Poppins', sans-serif; } body { background: linear-gradient(135deg, #e0f2fe, #bae6fd); min-height: 100vh; transition: background 0.3s; } body.dark { background: linear-gradient(135deg, #1e3a8a, #3b82f6); } .container { max-width: 800px; margin: 0 auto; padding: 20px; } .controls { text-align: center; margin-bottom: 40px; color: #1e3a8a; } body.dark .controls { color: #f1f5f9; } h1 { font-family: 'Poppins', sans-serif; font-weight: 700; font-size: 2.8rem; margin-bottom: 20px; letter-spacing: 1px; } .settings { display: flex; justify-content: center; gap: 15px; flex-wrap: wrap; } select, button { padding: 10px 20px; border: none; border-radius: 25px; background: #ffffff; cursor: pointer; font-family: 'Lora', serif; font-size: 1rem; font-weight: 400; transition: all 0.3s ease; } body.dark select, body.dark button { background: #1e40af; color: #ffffff; } select:hover, button:hover { transform: translateY(-2px); box-shadow: 0 4px 15px rgba(0,0,0,0.1); } .breathing-container { position: relative; height: 400px; display: flex; justify-content: center; align-items: center; } .circle { width: 200px; height: 200px; background: radial-gradient(circle, #3b82f6 0%, #93c5fd 70%, transparent 100%); border-radius: 50%; position: absolute; transition: all 1s ease-in-out; } body.dark .circle { background: radial-gradient(circle, #60a5fa 0%, #1e40af 70%, transparent 100%); } .inhale { transform: scale(1.5); } .hold { transform: scale(1.2); } .exhale { transform: scale(1); } .instructions { position: relative; text-align: center; color: #1e3a8a; z-index: 1; } body.dark .instructions { color: #f1f5f9; } #breathText { display: block; font-family: 'Poppins', sans-serif; font-weight: 300; font-size: 2.2rem; margin-bottom: 10px; letter-spacing: 0.5px; } #timer { font-family: 'Lora', serif; font-weight: 700; font-size: 3.5rem; line-height: 1; } .progress { margin-top: 40px; text-align: center; } .progress-bar { width:
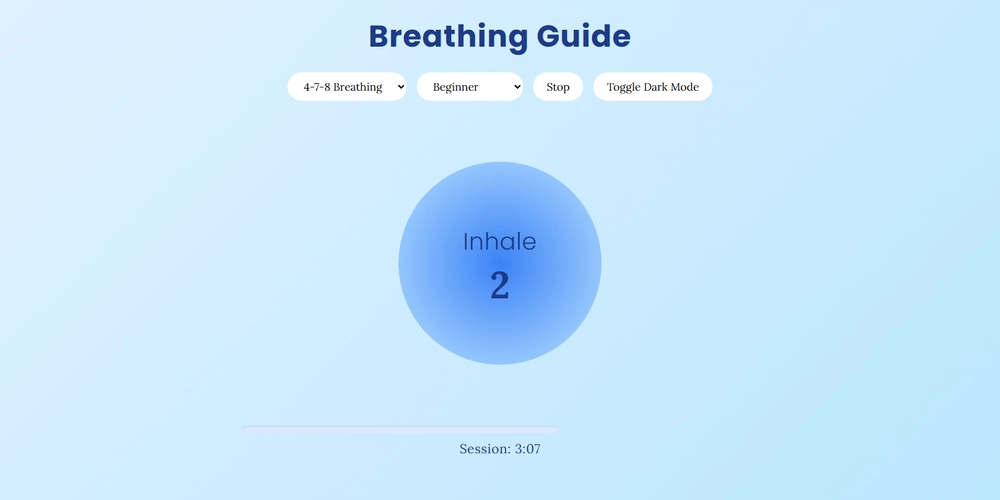
Ever feel like your coding sessions leave you tense and stressed? I've been there too! That's why I created a breathing exercise web application that helps developers (and everyone else) practice mindful breathing techniques with visual guidance.
In this post, I'll walk through how I built this app using vanilla JavaScript, CSS animations, and a clean, responsive design. Whether you're looking to build something similar or just want to add some interactive animations to your projects, there's something here for you!
Demo
You can try the live app here: Breathing Exercise Guide
The Why Behind the App
As developers, we often forget to breathe properly while focusing intensely on debugging or building new features. Research shows that controlled breathing can:
- Reduce mental fatigue and stress
- Improve problem-solving abilities
- Increase focus and concentration
- Lower physical tension from long coding sessions
Each breathing pattern in the app serves a specific purpose:
- 4-7-8 Breathing: Great for rapid relaxation and breaking stress cycles
- Box Breathing: Enhances focus and clarity (used by Navy SEALs!)
- Diaphragmatic Breathing: Improves oxygen flow and reduces shallow breathing habits
App Features
Here's what our breathing app includes:
- Multiple breathing patterns with different timing sequences
- Three difficulty levels to accommodate users of all experience levels
- Visual animations that expand and contract with breathing rhythm
- A countdown timer for each breathing phase
- Session tracking to monitor practice time
- Dark mode toggle for reduced eye strain (especially important for night coding!)
The HTML Structure
Let's start with the HTML backbone of our application:
charset="UTF-8">
name="viewport" content="width=device-width, initial-scale=1.0">
Breathing Exercise Guide
rel="stylesheet" href="styles.css">
href="https://fonts.googleapis.com/css2?family=Poppins:wght@300;400;700&family=Lora:wght@400;700&display=swap" rel="stylesheet">
class="container">
class="controls">
Breathing Guide
class="settings">
class="breathing-container">
class="circle">
class="instructions">
id="breathText">Breathe In
id="timer">4
class="progress">
class="progress-bar" id="progressBar">
id="sessionTime">Session: 0:00