Integrating Git API into a Spring Boot Application: A Comprehensive Guide
Git APIs allow developers to interact with repositories programmatically, enabling automation of workflows like creating branches, retrieving repository details, or committing changes. This blog covers step-by-step instructions to integrate Git API into a Spring Boot application. Step 1: Setup Your Spring Boot Application Start by creating a Spring Boot project. You can generate the boilerplate code using Spring Initializr. Add dependencies like Spring Web, Spring Boot Starter JSON, and optionally Spring Security for authentication. Add the Maven dependencies in your pom.xml: org.springframework.boot spring-boot-starter-web org.springframework.boot spring-boot-starter-json org.springframework.boot spring-boot-starter-security Step 2: Register a Personal Access Token (PAT) GitHub APIs require authentication via a Personal Access Token (PAT). Navigate to your GitHub account settings and generate a PAT with the necessary permissions (e.g., repo permissions to access repositories). Step 3: Create a Service to Handle Git API Integration Create a service class responsible for making HTTP requests to the Git API. Use RestTemplate or WebClient for HTTP communication. Here’s an example using RestTemplate: import org.springframework.stereotype.Service; import org.springframework.web.client.RestTemplate; import org.springframework.http.*; import java.util.Collections; @Service public class GitService { private final RestTemplate restTemplate; private static final String GITHUB_API_URL = "https://api.github.com"; public GitService() { this.restTemplate = new RestTemplate(); } public String getRepositoryDetails(String owner, String repo, String token) { String url = GITHUB_API_URL + "/repos/" + owner + "/" + repo; // Set up headers HttpHeaders headers = new HttpHeaders(); headers.set("Authorization", "Bearer " + token); headers.setAccept(Collections.singletonList(MediaType.APPLICATION_JSON)); HttpEntity entity = new HttpEntity(headers); // Make the API call ResponseEntity response = restTemplate.exchange(url, HttpMethod.GET, entity, String.class); return response.getBody(); } } Step 4: Create a Controller to Expose Endpoints Now, create a REST controller that maps the service methods to HTTP endpoints: import org.springframework.beans.factory.annotation.Autowired; import org.springframework.web.bind.annotation.*; @RestController @RequestMapping("/api/git") public class GitController { @Autowired private GitService gitService; @GetMapping("/repo-details/{owner}/{repo}") public String getRepositoryDetails( @PathVariable String owner, @PathVariable String repo, @RequestHeader("Authorization") String token) { return gitService.getRepositoryDetails(owner, repo, token); } } Step 5: Testing Your Integration Start the Spring Boot application. Use tools like Postman or curl to test the endpoint. Set the Authorization header with your PAT. Example request URL: http://localhost:8080/api/git/repo-details/{owner}/{repo}. Sample curl command: curl -H "Authorization: Bearer YOUR_PAT" http://localhost:8080/api/git/repo-details/{owner}/{repo} Step 6: Enhance and Customize You can expand the implementation to include other Git API functionalities like: Creating a new branch: public String createBranch(String owner, String repo, String token, String branchName, String sha) { String url = GITHUB_API_URL + "/repos/" + owner + "/" + repo + "/git/refs"; HttpHeaders headers = new HttpHeaders(); headers.set("Authorization", "Bearer " + token); headers.setAccept(Collections.singletonList(MediaType.APPLICATION_JSON)); Map body = Map.of( "ref", "refs/heads/" + branchName, "sha", sha ); HttpEntity entity = new HttpEntity(body, headers); ResponseEntity response = restTemplate.exchange(url, HttpMethod.POST, entity, String.class); return response.getBody(); } Making commits or handling pull requests using appropriate endpoints. Conclusion Integrating Git API into a Spring Boot application opens doors to automating repository management, enabling dynamic workflows. With a robust setup like this, you can build advanced tools and services tailored to your needs. Have fun coding your next Git-integrated Spring Boot project!
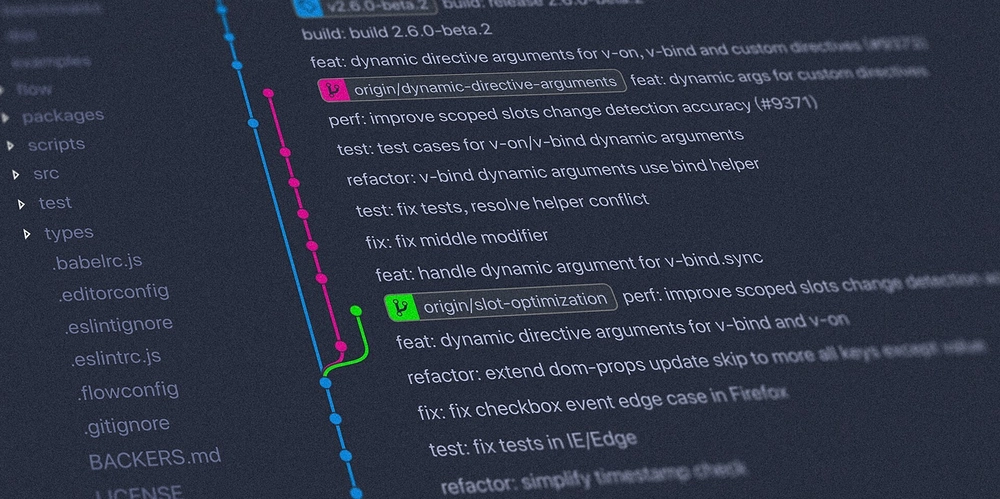
Git APIs allow developers to interact with repositories programmatically, enabling automation of workflows like creating branches, retrieving repository details, or committing changes. This blog covers step-by-step instructions to integrate Git API into a Spring Boot application.
Step 1: Setup Your Spring Boot Application
Start by creating a Spring Boot project. You can generate the boilerplate code using Spring Initializr. Add dependencies like Spring Web, Spring Boot Starter JSON, and optionally Spring Security for authentication.
Add the Maven dependencies in your pom.xml
:
org.springframework.boot
spring-boot-starter-web
org.springframework.boot
spring-boot-starter-json
org.springframework.boot
spring-boot-starter-security
Step 2: Register a Personal Access Token (PAT)
GitHub APIs require authentication via a Personal Access Token (PAT). Navigate to your GitHub account settings and generate a PAT with the necessary permissions (e.g., repo permissions to access repositories).
Step 3: Create a Service to Handle Git API Integration
Create a service class responsible for making HTTP requests to the Git API. Use RestTemplate
or WebClient
for HTTP communication.
Here’s an example using RestTemplate
:
import org.springframework.stereotype.Service;
import org.springframework.web.client.RestTemplate;
import org.springframework.http.*;
import java.util.Collections;
@Service
public class GitService {
private final RestTemplate restTemplate;
private static final String GITHUB_API_URL = "https://api.github.com";
public GitService() {
this.restTemplate = new RestTemplate();
}
public String getRepositoryDetails(String owner, String repo, String token) {
String url = GITHUB_API_URL + "/repos/" + owner + "/" + repo;
// Set up headers
HttpHeaders headers = new HttpHeaders();
headers.set("Authorization", "Bearer " + token);
headers.setAccept(Collections.singletonList(MediaType.APPLICATION_JSON));
HttpEntity<String> entity = new HttpEntity<>(headers);
// Make the API call
ResponseEntity<String> response = restTemplate.exchange(url, HttpMethod.GET, entity, String.class);
return response.getBody();
}
}
Step 4: Create a Controller to Expose Endpoints
Now, create a REST controller that maps the service methods to HTTP endpoints:
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.web.bind.annotation.*;
@RestController
@RequestMapping("/api/git")
public class GitController {
@Autowired
private GitService gitService;
@GetMapping("/repo-details/{owner}/{repo}")
public String getRepositoryDetails(
@PathVariable String owner,
@PathVariable String repo,
@RequestHeader("Authorization") String token) {
return gitService.getRepositoryDetails(owner, repo, token);
}
}
Step 5: Testing Your Integration
- Start the Spring Boot application.
- Use tools like Postman or curl to test the endpoint.
- Set the Authorization header with your PAT.
- Example request URL:
http://localhost:8080/api/git/repo-details/{owner}/{repo}
.
Sample curl command:
curl -H "Authorization: Bearer YOUR_PAT" http://localhost:8080/api/git/repo-details/{owner}/{repo}
Step 6: Enhance and Customize
You can expand the implementation to include other Git API functionalities like:
- Creating a new branch:
public String createBranch(String owner, String repo, String token, String branchName, String sha) {
String url = GITHUB_API_URL + "/repos/" + owner + "/" + repo + "/git/refs";
HttpHeaders headers = new HttpHeaders();
headers.set("Authorization", "Bearer " + token);
headers.setAccept(Collections.singletonList(MediaType.APPLICATION_JSON));
Map<String, String> body = Map.of(
"ref", "refs/heads/" + branchName,
"sha", sha
);
HttpEntity<Map<String, String>> entity = new HttpEntity<>(body, headers);
ResponseEntity<String> response = restTemplate.exchange(url, HttpMethod.POST, entity, String.class);
return response.getBody();
}
- Making commits or handling pull requests using appropriate endpoints.
Conclusion
Integrating Git API into a Spring Boot application opens doors to automating repository management, enabling dynamic workflows. With a robust setup like this, you can build advanced tools and services tailored to your needs.
Have fun coding your next Git-integrated Spring Boot project!