I Made a TUI in C... and regretted it
Hey! You! If you would prefer to consume this article as a video, check this out! I know what you're thinking... You might've clicked on this article because there are so many better tools to build a TUI with. Or, you might say if I’m this desperate to do something with C, why not just make something simple, like a hashmap? But no, I have the compulsive need to go big or go home. I chose to (kind of) completely reimplement my favorite todo app, Todoist, in the terminal (and yes, I know this exists already). I hadn’t used C in about two years, and instead of getting warmed up to it with a few Easy Leetcode problems, I dove directly into the deep end. What's the problem with C? It’s supposed to be this beautifully crafted, logical, low level programming language. Wait, hold on... there’s the problem. It’s a low level programming language. With C, this doesn’t just mean you control your program's memory, segfault after segfault after segfault. This means you control everything yourself. And yes, this applies to everything. Wanna create a JSON object with one value in it? // Ok, so maybe like 10 lines, if we're smart. cJSON *monitor = cJSON_CreateObject(); if (monitor == NULL) { goto end; } name = cJSON_CreateString("Awesome 4K"); if (name == NULL) { goto end; } cJSON_AddItemToObject(monitor, "name", name); // From cJSON repo Awesome, that’ll be about 20 lines of code. This isn’t the fault of C, of course. This is how it’s meant to work. It just means that the most menial features take hours to complete. The libraries So with all that being said, let’s dig into the libraries used to make this TUI, what they do, and their biggest pain points. Ncurses If you’ve ever worked with C before, this is likely a name that scares you a little bit. Don’t get me wrong, Ncurses was amazing for its time… which was about 30 years ago. Every state change, every update to the UI, down to the exact row and column, is up to you to handle. I recommend that you refer to the video for this part - specifically 1:11 And how exactly does it work, you ask? Well, there’s a lot going on behind the scenes that I certainly don’t understand, but generally speaking, Ncurses maintains something of a buffer that tracks the state of the currently used Ncurses Window – which in this case, refers to the Ncurses buffer, and not a window on your desktop – and then allows you to efficiently render and update that buffer and consequently re-render it to your terminal window by using refresh() #include int main() { initscr(); /* Start curses mode */ printw("Hello World !!!"); /* Print Hello World */ refresh(); /* Print it on to the real screen */ getch(); /* Wait for user input */ endwin(); /* End curses mode */ return 0; } // Courtesy of Pradeep Padala Ncurses also provides a bunch of out-of-the-box tools like menus and forms, similar to those that you would find in HTML and JavaScript. But as with anything else C related, you, the programmer, are expected to handle and deal with the guts of each of these tools. For instance, it takes about 50 lines of C and Ncurses to render a simple menu, and don’t even get me started on how one would actually make this menu reactive (well, do, because we’ll talk about this later). cJSON The second big library that I used is cJSON. Now, I don’t know if this really falls under the definition of a “library” for C. It’s a single file, but it is about 5000 lines, and probably about 25% of my code involves cJSON, so I’m counting it as a quote-unquote important library. Libcurl Now the other really big library that I used for this TUI is libcurl. As with Ncurses, it’s a wonderful library... that requires you to do everything yourself. Wanna make some headers? struct curl_slist *markCompleteHeaders = NULL; markCompleteHeaders = curl_slist_append(markCompleteHeaders, curlArgs.headers->data); markCompleteHeaders = curl_slist_append(markCompleteHeaders, "Content-Type: application/json"); markCompleteHeaders = curl_slist_append(markCompleteHeaders, "Accept: application/json"); Wonderful, that’ll be four function calls. Again, libcurl is a wonderful tool, it’s just a large step in a different direction from making an API request in something like Python or JavaScript. Documentation Finally, I want to mention documentation. If you’ve ever used a more modern language, framework, or library, which most of you probably have, you’re probably used to really beautiful, interactive, and consistently updated documentation. Take React for example. Almost every page has a small built-in tutorial as well as a font and font size that’s completely optimized for reading. But… that’s not the case for anything involved with Ncurses or Libcurl. Ncurses doesn’t
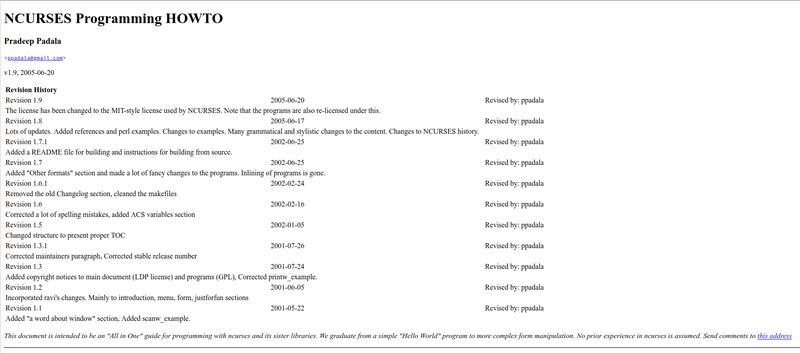
Hey! You! If you would prefer to consume this article as a video, check this out!
I know what you're thinking...
You might've clicked on this article because there are so many better tools to build a TUI with. Or, you might say if I’m this desperate to do something with C, why not just make something simple, like a hashmap?
But no, I have the compulsive need to go big or go home. I chose to (kind of) completely reimplement my favorite todo app, Todoist, in the terminal (and yes, I know this exists already). I hadn’t used C in about two years, and instead of getting warmed up to it with a few Easy Leetcode problems, I dove directly into the deep end.
What's the problem with C?
It’s supposed to be this beautifully crafted, logical, low level programming language. Wait, hold on... there’s the problem. It’s a low level programming language. With C, this doesn’t just mean you control your program's memory, segfault after segfault after segfault. This means you control everything yourself.
And yes, this applies to everything. Wanna create a JSON object with one value in it?
// Ok, so maybe like 10 lines, if we're smart.
cJSON *monitor = cJSON_CreateObject();
if (monitor == NULL)
{
goto end;
}
name = cJSON_CreateString("Awesome 4K");
if (name == NULL)
{
goto end;
}
cJSON_AddItemToObject(monitor, "name", name);
// From cJSON repo
Awesome, that’ll be about 20 lines of code. This isn’t the fault of C, of course. This is how it’s meant to work. It just means that the most menial features take hours to complete.
The libraries
So with all that being said, let’s dig into the libraries used to make this TUI, what they do, and their biggest pain points.
Ncurses
If you’ve ever worked with C before, this is likely a name that scares you a little bit. Don’t get me wrong, Ncurses was amazing for its time… which was about 30 years ago. Every state change, every update to the UI, down to the exact row and column, is up to you to handle.
I recommend that you refer to the video for this part - specifically 1:11
And how exactly does it work, you ask? Well, there’s a lot going on behind the scenes that I certainly don’t understand, but generally speaking, Ncurses maintains something of a buffer that tracks the state of the currently used Ncurses Window – which in this case, refers to the Ncurses buffer, and not a window on your desktop – and then allows you to efficiently render and update that buffer and consequently re-render it to your terminal window by using refresh()
#include
int main()
{
initscr(); /* Start curses mode */
printw("Hello World !!!"); /* Print Hello World */
refresh(); /* Print it on to the real screen */
getch(); /* Wait for user input */
endwin(); /* End curses mode */
return 0;
}
// Courtesy of Pradeep Padala
Ncurses also provides a bunch of out-of-the-box tools like menus and forms, similar to those that you would find in HTML and JavaScript. But as with anything else C related, you, the programmer, are expected to handle and deal with the guts of each of these tools. For instance, it takes about 50 lines of C and Ncurses to render a simple menu, and don’t even get me started on how one would actually make this menu reactive (well, do, because we’ll talk about this later).
cJSON
The second big library that I used is cJSON. Now, I don’t know if this really falls under the definition of a “library” for C. It’s a single file, but it is about 5000 lines, and probably about 25% of my code involves cJSON, so I’m counting it as a quote-unquote important library.
Libcurl
Now the other really big library that I used for this TUI is libcurl. As with Ncurses, it’s a wonderful library... that requires you to do everything yourself. Wanna make some headers?
struct curl_slist *markCompleteHeaders = NULL;
markCompleteHeaders =
curl_slist_append(markCompleteHeaders, curlArgs.headers->data);
markCompleteHeaders =
curl_slist_append(markCompleteHeaders, "Content-Type: application/json");
markCompleteHeaders =
curl_slist_append(markCompleteHeaders, "Accept: application/json");
Wonderful, that’ll be four function calls. Again, libcurl is a wonderful tool, it’s just a large step in a different direction from making an API request in something like Python or JavaScript.
Documentation
Finally, I want to mention documentation. If you’ve ever used a more modern language, framework, or library, which most of you probably have, you’re probably used to really beautiful, interactive, and consistently updated documentation. Take React for example. Almost every page has a small built-in tutorial as well as a font and font size that’s completely optimized for reading.
But… that’s not the case for anything involved with Ncurses or Libcurl. Ncurses doesn’t even have a dedicated site. There’s one hosted by Pradeep Padala (I think, at least) and another hosted by Thomas Dickey, who, as far as I know, is the current maintainer of Ncurses. While these sites are awesome, you’re really expected to work off of man ncurses
which is not wrong, so to speak, it’s just vastly different from what I’m used to.
As I said, you can also take a look at the Libcurl docs as another example. Again, there’s nothing inherently wrong with them – it’s just vastly different from what I’m used to. Lots of walls of text, and lots of “here’s some info, good luck” kind of documentation.
Why would I do this?
Before we dive into the code itself, I wanna answer one really important question. Why would I do this to myself?
I’m sure this is something that’s come to your mind! As I previously mentioned, there are so, so, so many better options out there when it comes to making a TUI. You could do something in Rust, Python, or really any language, even JavaScript.
Well, I’ve spent the last 5 months of my life working on byeAI, and after 500 commits, I feel… tired. There’s not really a better way to describe it. The last half of those commits didn’t involve me learning anything or doing anything new, just copying and pasting information from my brain. I wanted a break, and since I had a teensy tiny bit of previous experience with C, I wanted to do something with it.
Also, I felt a little bit of “impostor syndrome” because a lot of the tools that I used to build byeAI were… kind of just handed to me. I don’t have to think about the nitty gritty details of security when I’m using React. I don’t have to write raw SQL when I’m using Django. I don’t even have to think about how to structure my code and project – Django is opinionated, in that it’s built to be used with the Model-View-Template architecture.
Anyways, all that is absolutely terrific and wonderful, and don’t get me wrong, these tools are the reason why we have what we have today. You can learn Django in a few months and whip up a delightful website without having to worry about how the heck your code handles an HTTP request, how it efficiently interacts with your database, or how the floobleflorp triggers a flooblegast. But sometimes you just wanna go back and prove to yourself that you can do what is being done for you, and while a TUI written in C certainly doesn’t do what Django does, it helps to get rid of that nasty, lurking feeling.
Disclaimer
I’m a beginner when it comes to C. I’m sure there’s some awful code that could be completely improved or changed, and honestly, I’m sure that there are some memory leaks – and yes, I’m aware of Valgrind. So please don’t use this article to start learning C.
Furthermore, I’ll cover the first part of the program in a lot of depth, but I won’t do so for the rest of the program. That would just be… way too much information. I just wanna cover the first few lines so you can get a good gist of how Ncurses and Libcurl is supposed to work.
Todoist?
I should also take a moment to explain how Todoist functions. Todoist has projects, and “todos”, also known as tasks or items, and those tasks can be put into projects. Those tasks can also have a due date, and if the due date is today, they’re also displayed in the “Today” tab.
The first major step
So with that, the first major step is to grab the list of projects from the API, display them to the user, and let the user choose what project they want to view specifically including the “Today” tab.
Naturally, we start with the main()
function. We initialize Ncurses in this blob of code, and initialize a CURL handle with the curl_easy_init()
function. Because this is C, we have to check and C (pun intended) if the handle initialized properly. If it did, we continue with our program.
int main(void) {
// ncurses. stdscr acts as the "background", and everything else sits on top
// of it.
initscr();
raw();
noecho();
printw("Loading current projects. Press q to exit.\n");
refresh();
int row, col;
getmaxyx(stdscr, row, col);
keypad(stdscr, TRUE);
// curl
CURL *curl = curl_easy_init();
curl_global_init(CURL_GLOBAL_DEFAULT);
if (!curl) {
printf("curl didn't initalize correctly.\n");
return 1;
} else {
// Carry on!
Next, we get the auth token from the environment. This could certainly be done with a config file somewhere in the home directory or ~/.config
, but as I’ll mention later, my primary objective with this program is simply to have a proof of concept and prove to myself that I can actually make a functional TUI.
// ...
// Get auth token from environment
char *authToken = getenv("TODOIST_AUTH_TOKEN");
if (authToken == NULL) {
displayMessage("Unable to find auth token. Press any button to end the "
"program.\n");
curl_easy_cleanup(curl);
curl_global_cleanup();
endwin();
return 1;
}
// ...
Anyways, after we grab this authentication token, we slap it into a curl_slist
, which is basically just an HTTP header that we’ll add to the request in just a second.
// ...
char *authHeader = combineString("Authorization: Bearer ", authToken);
struct curl_slist *baseHeaders = NULL;
baseHeaders = curl_slist_append(baseHeaders, authHeader);
// ...
Then, we create the URL with one of my helper functions.
// ...
char *allProjectsUrl = combineString(BASE_REST_URL, "projects");
// ...
Finally, we get around to actually making the request. This is one of my structs called curlArgs
, and it just contains all of the essential stuff to make HTTP requests to a REST compliant API. If you’re unfamiliar, REST is kinda just a general API standard that generally supports GET
, POST
, PUT
, and DELETE
requests.
// ...
struct curlArgs allProjectsCurlArgs = {curl, baseHeaders, "GET",
allProjectsUrl};
cJSON *projectsJson = makeRequest(allProjectsCurlArgs);
int numOfProjects = cJSON_GetArraySize(projectsJson);
// ...
Now, what about the makeRequest()
function? This is one of my functions, and it makes about 50 lines of code nice and reusable. If we take a look inside, we can see that it just sets a bunch of options of the curl handle (which is basically just options for the request we’re about to make), makes the request, and then handles the output.
(If you'd like to see more, check out line 350 in main.c)
// ...
// The options being set
curl_easy_setopt(curl, CURLOPT_WRITEFUNCTION, curlWriteHelper);
curl_easy_setopt(curl, CURLOPT_HTTPHEADER, curlArgs.headers);
curl_easy_setopt(curl, CURLOPT_URL, curlArgs.url);
curl_easy_setopt(curl, CURLOPT_WRITEDATA, (void *)&requestData);
curl_easy_setopt(curl, CURLOPT_CUSTOMREQUEST, curlArgs.method);
// ...
Now, I want to make one thing very clear while we’re here. We use the same curl handle throughout our entire program. That means that we need to manually set or clear every field every time we make a request, otherwise we’re going to end up with fields that simply aren’t what they should be. And yes, libcurl’s documentation does recommend this as it seems to be a lot more efficient to do this rather than create a new curl handle every single time we make a request.
The easy handle is used to hold and control a single network transfer. It is encouraged to reuse easy handles for repeated transfers.
Anyways, back to the main function. Once we make the request and use cJSON to gather the data from it, we add one more field manually called today
. This is because of Todoist’s Today section that I find myself using like, 99% of the time, but their API doesn’t count it as an actual project.
// ...
// Adding a cJSON object so the user can also view active tasks (AKA the
// today section)
cJSON *today = cJSON_CreateObject();
if (today == NULL) {
goto end;
}
if (cJSON_AddStringToObject(today, "name", "Today") == NULL) {
goto end;
}
// Adding a "fake" ID field to help with managing menu (see event loop ~20
// lines down)
if (cJSON_AddStringToObject(today, "id", "999") == NULL) {
goto end;
}
cJSON_AddItemToArray(projectsJson, today);
// ...
Once we have that, we render the projects menu. But don’t forget, simply rendering a menu doesn’t mean that the user can actually see the menu. We need to call refresh()
in order to actually display the menu to the screen.
// ...
MENU *projectsMenu = renderMenuFromJson(projectsJson, "name");
if (projectsMenu == NULL) {
goto end;
}
// Render
clear();
set_menu_mark(projectsMenu, NULL);
post_menu(projectsMenu);
refresh();
// For free()-ing
ITEM **projectsItems = menu_items(projectsMenu);
// ...
And finally, we start what I somewhat appropriately call an event loop. This while loop runs until the user presses q, which then causes the program to exit. Oh, and when that happens, there’s a whole bunch of cleanup that has to be done, of course.
// ...
int getchChar;
while ((getchChar = getch()) != 'q') {
if (getchChar == 'j') {
menu_driver(projectsMenu, REQ_DOWN_ITEM);
} else if (KEY_UP || getchChar == 'k') {
menu_driver(projectsMenu, REQ_UP_ITEM);
// ...
Let's see what we have!
Oh… that’s it. Yeah, again, for the 30th time, we’re working with C here, which means that we’re doing the heavy lifting, so there’s not much to C yet. Anyways, what’s actually happening with this thing that I labeled as an “event loop”?
Well, firstly, we of course need to have vim bindings! If the user presses k or j, the menu will go up or down, respectively. But that’s not the meat of the logic. The really important stuff happens when the user presses l.
// ...
} else if (getchChar == 'l') {
// Find project ID, and call projectPanel with that project ID in a
// curlArgs struct
cJSON *currentItemJson = getCurrentItemJson(projectsMenu, projectsJson);
if (currentItemJson == NULL) {
displayMessage(
"Something went wrong when trying to access the current "
"item of the Ncurses menu. Press any key to quit.");
goto end;
}
//...
projectPanel(projectPanelCurlArgs, row, col);
// ...
This is the equivalent of opening a Todoist project and viewing the tasks inside it. Our code does this with Todoist’s API, and calls one really important function: projectPanel
. You can think of this as a copy of the main()
function. What it does is it makes a new Ncurses Window, which means that there’s now another buffer for Ncurses to write to. You can kinda think of this like a pop up, except for the fact that it takes up the entire screen.
Then, as I just said, it does something very similar to what the main()
function does. It calls the Todoist API, and slaps the result into a menu. There’s a lot going on here, so I want to make a few notes.
void projectPanel(struct curlArgs curlArgs, int row, int col) {
PANEL *projectPanel;
WINDOW *projectWindow;
// Query for list of currently open tasks
char *tasksUrl = combineString(BASE_REST_URL, "tasks");
cJSON *unsortedTasksJson = makeRequest(curlArgs);
//...
Firstly, we’re going to need the data from each respective task, specifically the content, the id, and the priority number. We have access to this data when we call renderMenuFromJson
, which naturally renders a… menu from JSON. Then, in this function, we do a few different things. If there’s nothing in the given JSON, we create a so-called blank array of elements and return it. If there is something there, we call createItemsFromJson
, and return a menu with those items.
MENU *renderMenuFromJson(cJSON *json, char *query) {
cJSON *currentTask = NULL;
int itemsLength = cJSON_GetArraySize(json);
// QOL
if (itemsLength == 0) {
ITEM **blankItems = (ITEM **)malloc(2 * sizeof(struct ITEM *));
blankItems[0] = new_item(NO_TASKS_TO_COMPLETE_MESSAGE, "");
blankItems[1] = (ITEM *)NULL;
MENU *blankMenu = new_menu(blankItems);
return blankMenu;
}
Now, in regard to the data from each respective task, we need to store it somewhere.
So how do we do so? Well, when we create an Ncurses Menu item with createItemsFromJson
, we’re only given two fields to fill out. But, we can use the user pointer functions to store quote-unquote metadata for each Menu item. Now this took me way too long to figure out, and I’m still not sure if I’m doing it correctly, but here’s how I’m doing it. You do also have to free()
this when you get rid of the menu, which isn’t that hard to do, but it should be noted.
Ok, that was a lot. If you'd like to take a look at some visuals of how this works, check out this part of the video.
When the user presses l, the Todoist API is called, and relevant tasks are grabbed from the response. These tasks are then rendered in a panel (remember that a panel is basically just another Ncurses Window), and rendered into a Menu with renderMenuFromJson
, which calls createItemsFromJson
, which adds metadata to each item.
Managing single tasks
We’re finally at the point where we can manage single tasks.
We see an event loop here very similar to that of main()
, except for a few differences. If the user presses h or q, the event loop will end, closing the panel, and taking the user back to the main menu (AKA the projects menu). If they press p, the program will close the current task, which is basically just marking it as not due until the next day. If they press o, the task will be reopened – naturally, this is the inverse of closing a task. And finally, if they press d, the task will be permanently deleted, after confirmation from the user, of course.
// ...
// Event loop (ish?). Think of 'break' as going back to the projects menu.
int getchChar;
while ((getchChar = getch()) != 'q') {
if (getchChar == KEY_DOWN || getchChar == 'j') {
menu_driver(tasksMenu, REQ_DOWN_ITEM);
} else if (getchChar == KEY_UP || getchChar == 'k') {
menu_driver(tasksMenu, REQ_UP_ITEM);
} else if (getchChar == 'h') {
break;
} else if (getchChar == 'p') {
ITEM **newItems = closeTask(tasksJson, tasksMenu, curlArgs);
if (newItems == NULL) {
break;
}
setItemsAndRepostMenu(tasksMenu, newItems);
refresh();
} else if (getchChar == 'o') {
// ... and more
On a slight tangent, I want to take this time to again mention how much heavy lifting we’re doing here. Take a look at how the 10 lines of JSON for the API request for updating the TODO date of a task is made. It’s about… 70 lines, maybe. And really, that’s not even the worst of it. Just another glaring reminder of the niceties of modern languages (and as I try to keep in mind, say thank you open source maintainers. Most languages are open source, and programming would suck if the majority of languages were proprietary).
Anyways, those functions are great and all, but they aren’t all that intricate. All that they involve is taking an item’s previously mentioned metadata, using it to call an API, and updating the items/menu if necessary.
So let’s take a look at something that’s a bit more intricate: creating a task. We need to prompt the user for a task, and then create it, and manually append the new task onto the updated list of items.
The prompting and appending are the fun parts here, so let’s take a deeper dive into that. Firstly, we need to get the input from the user. We can do so with a very nice out-of-the-box Ncurses tool called Fields. With this, we can pretty easily gather input data from the user. Do note that I’m using my own helper function here called displayInputField
.
char *displayInputField(char *infoText) {
// Fields
const int lineLength = 50;
FIELD *input[2];
input[0] = new_field(1, lineLength, 0, 0, 0, 0);
set_field_back(input[0], A_UNDERLINE);
input[1] = NULL;
// Forms
FORM *form = new_form(input);
// Render
clear();
post_form(form);
refresh();
// ...
It does exactly what the name suggests: displays an input field, gathers data, and returns the data when it’s done.
So, we add a bit of styling to the form, and then render it. This is where things get a bit weird, and to be completely frank, I don’t think this is the intended way of doing things. If the user presses backspace, we update the field accordingly, and if the user presses a key other than enter, we again update the field accordingly. You’ll also notice that we maintain our own string of data. Once the user presses enter, we send this data back to the function it was originally called from, which is createTask
.
Then, as we’ve done about 93 times before, we make an API request and create this task.
// ...
cJSON *result = makeRequest(createTaskCurlArgs);
free(commands);
if (result == NULL) {
displayMessage(
"Something went wrong when making the request to close the "
"task. Press any key to return to the projects menu.");
return NULL;
}
// tmp_uuid will be free later on
free(uuid);
// ...
But now we have to manually update this list of items. Now, I think there might be a more efficient way to do this with realloc()
, but look, this is what I’m rolling with for the moment.
// ...
ITEM **newItems =
createItemsFromJson(tasksJson, curItemsLength + 2, "content");
newItems[curItemsLength] = new_item(newTaskName, "1");
// ...
Then, we have to do this funky little thing where we append a new item onto the end of this array, and append new meta data to said item. Finally, we’re able to return the new array of items, and update the menu.
// ...
struct taskMetaData *newTaskMetaData =
(struct taskMetaData *)malloc(sizeof(struct taskMetaData));
newTaskMetaData->content = newTaskName;
// Get variables (redacted for sake of time)
free(tmp_uuid);
char *id = idJson->valuestring;
newTaskMetaData->id = id;
newTaskMetaData->priority = 1;
set_item_userptr(newItems[curItemsLength], newTaskMetaData);
newItems[curItemsLength + 1] = (ITEM *)NULL;
return newItems;
// ...
We're kinda done
Now with that, we’re… kind of done. We’ve covered all of the really nasty and interesting stuff, and we’ve successfully created a working TUI, even though it might not look that good.
On that note, what else can we do to make this look better? Well, there’s one main thing that comes to mind, and that’s color.
Unfortunately, coloring an Ncurses menu is a pain, at best. And even then, you’re really not able to separately color that much, so I chose to leave everything unstyled and leave the customization up to the terminal emulator itself – in other words, transparency and stuff.
With all that being said...
This program is not intended for general use.
It’s a proof of concept, if anything. If you want a Todoist TUI, there are plenty of better options. There’s one written in Rust, which is actively maintained and sits at a healthy 1.5 thousand stars, and I’m sure you could find others if you look around enough.
And with that being said, I don’t plan to improve this any further. If this is something that you, for some reason, really want to see grow, please feel free to fork it and develop it in your own time.
peace