How to Structure Repository and Service Interfaces in Go Clean Architecture?
Introduction to Clean Architecture in Go Clean Architecture, introduced by Robert C. Martin (Uncle Bob), emphasizes separation of concerns and independence of frameworks. When applying these principles in Go, particularly for a small accounting web tool with a REST API, it's essential to organize your codebase effectively. This includes thoughtful structuring of repository and service interfaces to align with your domain-driven design. In this article, we'll delve into the best practices for defining interfaces for repositories and services within your application, clarifying common confusions regarding where these interfaces should reside. Understanding the Clean Architecture Layers To effectively design your application, let's briefly recap the layers of Clean Architecture: Domain Layer: This is the core of your application, where your entities and business logic reside. Entities are typically plain structs that encapsulate the business rules surrounding the data. Application Layer: This includes the use cases or application services that orchestrate operations by using the domain entities. It is here that you will interact with your repositories and any necessary services. Infrastructure Layer: This layer implements interfaces defined in the application layer, such as repositories and external service integrations (e.g., databases, message queues). Config Layer: This layer holds configurations relevant to databases, third-party services, and the application setup. Defining Repository Interfaces The repository interfaces are crucial for data handling in Go applications. A common question you might face is, "Where should I define repository interfaces?" Here’s the guided approach: Define Once in the Domain Layer Typically, repository interfaces like UserScopeRepository should be defined in the relevant module, in this case, the User module of your domain layer. This encapsulation fosters reusability across different use cases. For instance, defining your UserScopeRepository interface in the User module makes it accessible to all use cases like CreateUser, RefreshToken, and others. Here’s how you might structure this: package user // UserScopeRepository defines the interface for user data operations. type UserScopeRepository interface { GetUserByID(id string) (*User, error) CreateUser(user *User) error // Other user-related methods } Utilizing the Repository in Use Cases Once your repository is defined, you can inject it into your use cases. For example, here's how you might use it in the RefreshToken use case: package usecases type RefreshToken struct { UserRepo user.UserScopeRepository } func (r *RefreshToken) Execute(token string) (string, error) { // Use UserRepo to refresh the token } Defining Service Interfaces Similar to repositories, the question arises regarding service interfaces such as password hashing or JWT token generation: Generalization in the Application Layer Service interfaces should generally be defined in the application layer. This provides a central location for defining services that can be shared across multiple use cases. For example, you might define a TokenGenerator service as follows: package services // TokenGenerator defines the interface for token generation. type TokenGenerator interface { GenerateToken(userID string) (string, error) } Injecting Services into Use Cases When services are defined in the application layer, they can be injected into your use cases likewise. This reduces redundancy and encourages a clean architecture: package usecases type CreateUser struct { UserRepo user.UserScopeRepository PasswordHasher services.PasswordHasher } func (c *CreateUser) Execute(user *User) error { hashedPassword, err := c.PasswordHasher.HashPassword(user.Password) // Further logic... } Factory Pattern for Service Management You might consider using a factory pattern to manage service creation. This approach creates a central point for constructing the various services as needed, which can help manage dependencies more effectively: package factories type ServiceFactory struct { PasswordHasher services.PasswordHasher TokenGenerator services.TokenGenerator } func NewServiceFactory() *ServiceFactory { return &ServiceFactory{ PasswordHasher: NewScryptHasher(), TokenGenerator: NewJWTGenerator(), } } Passing Around Services Once you have a service factory, inject the factory into your use cases, allowing easy access to all necessary services: package usecases type SomeUseCase struct { ServiceFactory *factories.ServiceFactory } func (s *SomeUseCase) Execute() { // Access services via ServiceFactory } Frequently Asked Questions 1. Should I define repository interfaces in use cases? No, repository interfaces should be defined once in their corresponding module to promote reusability. 2. Where to define service interfaces? Service interfaces should
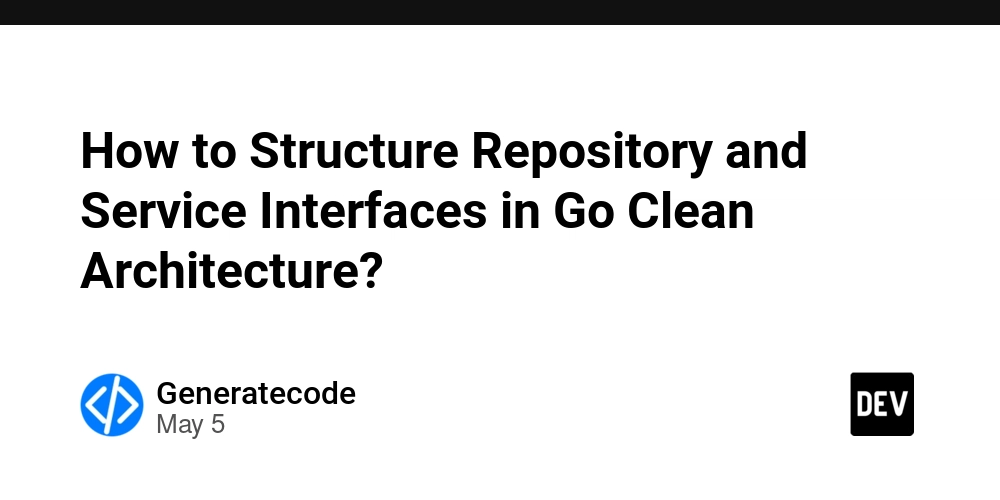
Introduction to Clean Architecture in Go
Clean Architecture, introduced by Robert C. Martin (Uncle Bob), emphasizes separation of concerns and independence of frameworks. When applying these principles in Go, particularly for a small accounting web tool with a REST API, it's essential to organize your codebase effectively. This includes thoughtful structuring of repository and service interfaces to align with your domain-driven design.
In this article, we'll delve into the best practices for defining interfaces for repositories and services within your application, clarifying common confusions regarding where these interfaces should reside.
Understanding the Clean Architecture Layers
To effectively design your application, let's briefly recap the layers of Clean Architecture:
-
Domain Layer: This is the core of your application, where your entities and business logic reside. Entities are typically plain structs that encapsulate the business rules surrounding the data.
-
Application Layer: This includes the use cases or application services that orchestrate operations by using the domain entities. It is here that you will interact with your repositories and any necessary services.
-
Infrastructure Layer: This layer implements interfaces defined in the application layer, such as repositories and external service integrations (e.g., databases, message queues).
-
Config Layer: This layer holds configurations relevant to databases, third-party services, and the application setup.
Defining Repository Interfaces
The repository interfaces are crucial for data handling in Go applications. A common question you might face is, "Where should I define repository interfaces?" Here’s the guided approach:
Define Once in the Domain Layer
Typically, repository interfaces like UserScopeRepository
should be defined in the relevant module, in this case, the User module of your domain layer. This encapsulation fosters reusability across different use cases.
For instance, defining your UserScopeRepository
interface in the User module makes it accessible to all use cases like CreateUser
, RefreshToken
, and others. Here’s how you might structure this:
package user
// UserScopeRepository defines the interface for user data operations.
type UserScopeRepository interface {
GetUserByID(id string) (*User, error)
CreateUser(user *User) error
// Other user-related methods
}
Utilizing the Repository in Use Cases
Once your repository is defined, you can inject it into your use cases. For example, here's how you might use it in the RefreshToken
use case:
package usecases
type RefreshToken struct {
UserRepo user.UserScopeRepository
}
func (r *RefreshToken) Execute(token string) (string, error) {
// Use UserRepo to refresh the token
}
Defining Service Interfaces
Similar to repositories, the question arises regarding service interfaces such as password hashing or JWT token generation:
Generalization in the Application Layer
Service interfaces should generally be defined in the application layer. This provides a central location for defining services that can be shared across multiple use cases. For example, you might define a TokenGenerator
service as follows:
package services
// TokenGenerator defines the interface for token generation.
type TokenGenerator interface {
GenerateToken(userID string) (string, error)
}
Injecting Services into Use Cases
When services are defined in the application layer, they can be injected into your use cases likewise. This reduces redundancy and encourages a clean architecture:
package usecases
type CreateUser struct {
UserRepo user.UserScopeRepository
PasswordHasher services.PasswordHasher
}
func (c *CreateUser) Execute(user *User) error {
hashedPassword, err := c.PasswordHasher.HashPassword(user.Password)
// Further logic...
}
Factory Pattern for Service Management
You might consider using a factory pattern to manage service creation. This approach creates a central point for constructing the various services as needed, which can help manage dependencies more effectively:
package factories
type ServiceFactory struct {
PasswordHasher services.PasswordHasher
TokenGenerator services.TokenGenerator
}
func NewServiceFactory() *ServiceFactory {
return &ServiceFactory{
PasswordHasher: NewScryptHasher(),
TokenGenerator: NewJWTGenerator(),
}
}
Passing Around Services
Once you have a service factory, inject the factory into your use cases, allowing easy access to all necessary services:
package usecases
type SomeUseCase struct {
ServiceFactory *factories.ServiceFactory
}
func (s *SomeUseCase) Execute() {
// Access services via ServiceFactory
}
Frequently Asked Questions
1. Should I define repository interfaces in use cases?
No, repository interfaces should be defined once in their corresponding module to promote reusability.
2. Where to define service interfaces?
Service interfaces should typically be defined in the application layer for broader access across use cases.
3. What advantage does the factory pattern provide?
Using a factory for services centralizes the creation logic and improves dependency management within your application.
Conclusion
Defining repository and service interfaces correctly is essential when implementing Uncle Bob's Clean Architecture in Go. By centralizing your interfaces in their related modules and the application layer, you not only adhere to clean architecture principles but also enhance the maintainability and readability of your code. Additionally, considering a factory for service management can streamline service instantiation and promote a cohesive architecture.
This structure will help you adhere to the principles of clean architecture while effectively using Go’s idioms, enhancing your small accounting web tool as it scales.