How to Position a Highlighted Block in Jetpack Compose LazyColumn?
Introduction If you're working with Jetpack Compose, you may have come across a situation where you want a specific item, referred to as a "highlighted block," to always remain at the bottom of the visible viewport within a LazyColumn. Unlike a regular Column, implementing this feature in a LazyColumn might not be as straightforward. In this article, we will explore how to achieve this layout effectively. Understanding Jetpack Compose LazyColumn Before diving into the solution, it's crucial to understand the difference between Column and LazyColumn. While a Column displays its children in a vertical sequence without recycling items, a LazyColumn is optimized for lists with potentially large data sets, creating and disposing of views as they are scrolled into and out of sight. This aspect of LazyColumn can complicate positioning items, especially for use cases like our highlighted block. A typical Column implementation might look like this: Column(modifier = Modifier.fillMaxSize()) { items.forEach { item -> ListItem(item) } Spacer(modifier = Modifier.weight(1f)) HighlightedBlock() } In the code above, the Spacer uses Modifier.weight(1f) to push the HighlightedBlock down to the bottom of the screen, filling up the remaining space. The Challenge with LazyColumn While you might try a similar approach in LazyColumn, using Spacer() with Modifier.weight() doesn't work as intended because LazyColumn optimizes its items for performance. Here’s an initial attempt: LazyColumn(modifier = Modifier.fillMaxSize()) { items(itemsList) { item -> ListItem(item) } item { Spacer(modifier = Modifier.weight(1f)) } item { HighlightedBlock() } } In this case, you won't see the desired effect because the Spacer does not maintain a fixed height; it’s treated differently in the context of a lazy list where recycling happens. Step-by-Step Solution to Keep the Highlighted Block at the Bottom To ensure that the highlighted block stays at the bottom regardless of the number of items in the LazyColumn, we need to leverage another composable structure. Here’s a more effective approach: 1. Use a Box as a Wrapper Use a Box to wrap your LazyColumn and position your highlighted block outside the LazyColumn. This allows you to make use of absolute positioning for the highlighted block. 2. Implementing the Solution Here’s a full working example: @Composable fun MyScreen() { Box(modifier = Modifier.fillMaxSize()) { LazyColumn(modifier = Modifier.fillMaxSize()) { items(itemsList) { item -> ListItem(item) } } // Use the Modifier.align() to position the highlighted block at the bottom HighlightedBlock(modifier = Modifier.align(Alignment.BottomCenter)) } } 3. The Highlighted Block Implementation You can define your HighlightedBlock() composable separately: @Composable fun HighlightedBlock(modifier: Modifier = Modifier) { Box(modifier = modifier.padding(16.dp).background(Color.Yellow)) { Text(text = "This is the highlighted block", fontSize = 20.sp) } } Conclusion By using a Box to contain the LazyColumn and positioning the highlighted block with Modifier.align(), you can effectively keep the highlighted block at the bottom of the viewport in Jetpack Compose. This method ensures that regardless of the number of items in your list, the highlighted block will always be visible to users. Frequently Asked Questions Can I use other layouts instead of Box? Yes, other layouts like ConstraintLayout can be used, but the Box approach is the simplest for this scenario. Is there any performance impact using Box? Using a Box in this context does not significantly impact performance and resolves the layout issue elegantly. How does LazyColumn handle larger datasets? LazyColumn is optimized for larger datasets by only composing items that are on the screen, which minimizes the impact on memory and performance. Implementing these techniques will provide better user experience while maintaining performance with LazyColumn layouts in Jetpack Compose.
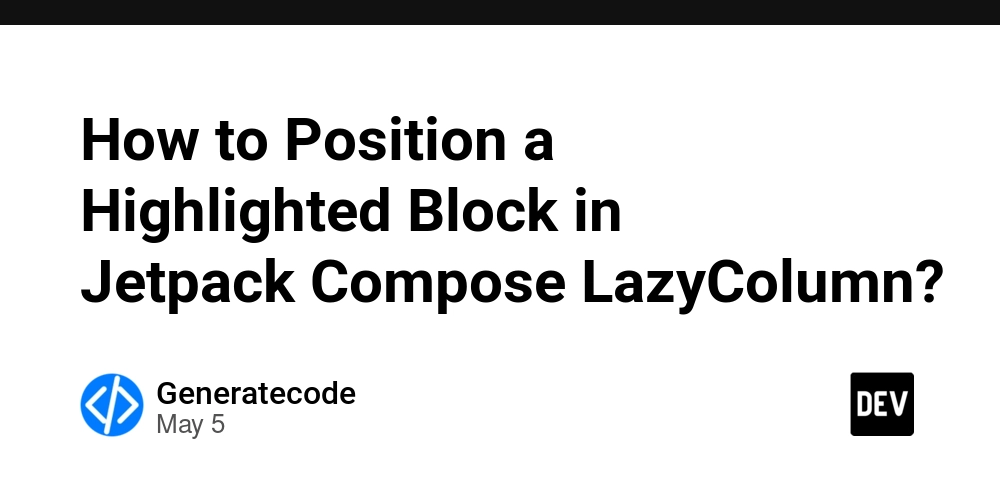
Introduction
If you're working with Jetpack Compose, you may have come across a situation where you want a specific item, referred to as a "highlighted block," to always remain at the bottom of the visible viewport within a LazyColumn
. Unlike a regular Column
, implementing this feature in a LazyColumn
might not be as straightforward. In this article, we will explore how to achieve this layout effectively.
Understanding Jetpack Compose LazyColumn
Before diving into the solution, it's crucial to understand the difference between Column
and LazyColumn
. While a Column
displays its children in a vertical sequence without recycling items, a LazyColumn
is optimized for lists with potentially large data sets, creating and disposing of views as they are scrolled into and out of sight. This aspect of LazyColumn
can complicate positioning items, especially for use cases like our highlighted block.
A typical Column
implementation might look like this:
Column(modifier = Modifier.fillMaxSize()) {
items.forEach { item ->
ListItem(item)
}
Spacer(modifier = Modifier.weight(1f))
HighlightedBlock()
}
In the code above, the Spacer
uses Modifier.weight(1f)
to push the HighlightedBlock
down to the bottom of the screen, filling up the remaining space.
The Challenge with LazyColumn
While you might try a similar approach in LazyColumn
, using Spacer()
with Modifier.weight()
doesn't work as intended because LazyColumn
optimizes its items for performance. Here’s an initial attempt:
LazyColumn(modifier = Modifier.fillMaxSize()) {
items(itemsList) { item ->
ListItem(item)
}
item {
Spacer(modifier = Modifier.weight(1f))
}
item {
HighlightedBlock()
}
}
In this case, you won't see the desired effect because the Spacer
does not maintain a fixed height; it’s treated differently in the context of a lazy list where recycling happens.
Step-by-Step Solution to Keep the Highlighted Block at the Bottom
To ensure that the highlighted block stays at the bottom regardless of the number of items in the LazyColumn
, we need to leverage another composable structure. Here’s a more effective approach:
1. Use a Box as a Wrapper
Use a Box
to wrap your LazyColumn
and position your highlighted block outside the LazyColumn
. This allows you to make use of absolute positioning for the highlighted block.
2. Implementing the Solution
Here’s a full working example:
@Composable
fun MyScreen() {
Box(modifier = Modifier.fillMaxSize()) {
LazyColumn(modifier = Modifier.fillMaxSize()) {
items(itemsList) { item ->
ListItem(item)
}
}
// Use the Modifier.align() to position the highlighted block at the bottom
HighlightedBlock(modifier = Modifier.align(Alignment.BottomCenter))
}
}
3. The Highlighted Block Implementation
You can define your HighlightedBlock()
composable separately:
@Composable
fun HighlightedBlock(modifier: Modifier = Modifier) {
Box(modifier = modifier.padding(16.dp).background(Color.Yellow)) {
Text(text = "This is the highlighted block", fontSize = 20.sp)
}
}
Conclusion
By using a Box
to contain the LazyColumn
and positioning the highlighted block with Modifier.align()
, you can effectively keep the highlighted block at the bottom of the viewport in Jetpack Compose. This method ensures that regardless of the number of items in your list, the highlighted block will always be visible to users.
Frequently Asked Questions
Can I use other layouts instead of Box?
Yes, other layouts like ConstraintLayout
can be used, but the Box
approach is the simplest for this scenario.
Is there any performance impact using Box?
Using a Box
in this context does not significantly impact performance and resolves the layout issue elegantly.
How does LazyColumn handle larger datasets?
LazyColumn
is optimized for larger datasets by only composing items that are on the screen, which minimizes the impact on memory and performance.
Implementing these techniques will provide better user experience while maintaining performance with LazyColumn
layouts in Jetpack Compose.