How to Enable Inline Variable Values in C# Debugging
Debugging is a crucial phase in software development, allowing developers to track down and fix bugs in their code. One feature that significantly enhances the debugging experience in Visual Studio (VS) is the ability to view objects' values inline while stepping through your C# code. If you've noticed that your colleagues can see values inline when debugging but you can't, don't worry! This article will guide you through the steps necessary to enable this feature and make your debugging sessions more efficient. Understanding Inline Values in Visual Studio Inline values provide a convenient way to monitor variable states without having to hover over them or check the Watch window. This feature is particularly useful for tracking down issues quickly as it allows you to see how variable values change as your code executes. Why You Might Not See Inline Values If you have trouble viewing inline variable values during debugging, it could be due to a few reasons: Debugging Mode: Ensure that you are running your application in Debug mode, not Release mode. In Release mode, optimizations might remove some debugging information. Settings Configuration: Visual Studio's settings may not be set to display inline values. Version of Visual Studio: Inline values are available in newer versions of Visual Studio, so if you are using an outdated version, consider upgrading. Steps to Enable Inline Values in Visual Studio Follow these straightforward steps to enable inline variable values in your Visual Studio environment: Step 1: Open Visual Studio Options Launch Visual Studio. Click on Tools in the menu bar. Select Options from the dropdown list. Step 2: Navigate to Debugging Settings In the Options dialog, expand the Debugging node in the left panel. Click on the General option. Step 3: Enable Inline Values In the General debug settings, look for the option labeled Enable source link support. Ensure this box is checked. Next, find the option Show all variables inline and check this box. Step 4: Save and Restart Click OK to save your settings. Restart Visual Studio to apply the changes. Step 5: Start Debugging Open your C# project and set breakpoints where you want to inspect variable values. Start debugging (F5) and begin stepping through your code using F10 (Step Over) or F11 (Step Into). You should now see the values of your variables displayed inline next to the code. Example Code Snippet Here’s a simple C# code snippet to illustrate inline debugging: using System; namespace InlineDebuggingDemo { class Program { static void Main(string[] args) { int x = 10; int y = 20; int sum = Add(x, y); Console.WriteLine($"Sum of {x} and {y} is {sum}"); } static int Add(int a, int b) { return a + b; } } } When you set a breakpoint in the Main method and step through the code, you should see the values of x, y, and sum inline beside their respective declarations. Frequently Asked Questions Can I view inline values on other IDEs? While inline value viewing is a feature mostly associated with Visual Studio, other IDEs such as JetBrains Rider may offer similar functionality, but the settings will differ. What if I still don't see inline values after enabling them? If inline values do not appear after you've followed the steps, ensure that your Visual Studio is up-to-date, or try resetting your user settings to defaults. Does inline viewing work with all data types? Yes, inline viewing in Visual Studio typically works with primitive data types as well as objects, though complex types may require additional inspection through the Watch window. Conclusion Enabling inline variable values in Visual Studio can greatly enhance your debugging workflow by providing instant feedback without extra steps. By following the steps outlined in this article, you can configure your Visual Studio settings, allowing you to focus on code quality and bug fixing. Remember to ensure you’re in Debug mode and keep your IDE up to date for the best experience.
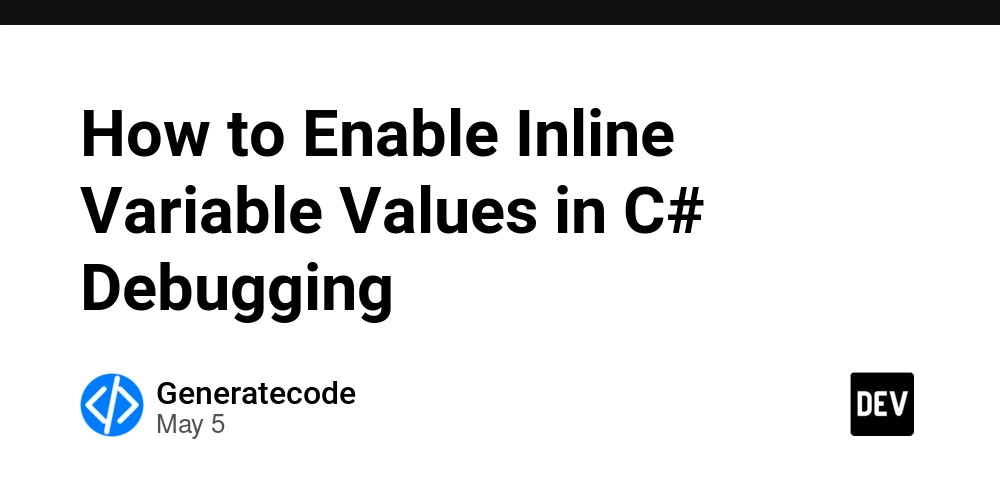
Debugging is a crucial phase in software development, allowing developers to track down and fix bugs in their code. One feature that significantly enhances the debugging experience in Visual Studio (VS) is the ability to view objects' values inline while stepping through your C# code. If you've noticed that your colleagues can see values inline when debugging but you can't, don't worry! This article will guide you through the steps necessary to enable this feature and make your debugging sessions more efficient.
Understanding Inline Values in Visual Studio
Inline values provide a convenient way to monitor variable states without having to hover over them or check the Watch window. This feature is particularly useful for tracking down issues quickly as it allows you to see how variable values change as your code executes.
Why You Might Not See Inline Values
If you have trouble viewing inline variable values during debugging, it could be due to a few reasons:
- Debugging Mode: Ensure that you are running your application in Debug mode, not Release mode. In Release mode, optimizations might remove some debugging information.
- Settings Configuration: Visual Studio's settings may not be set to display inline values.
- Version of Visual Studio: Inline values are available in newer versions of Visual Studio, so if you are using an outdated version, consider upgrading.
Steps to Enable Inline Values in Visual Studio
Follow these straightforward steps to enable inline variable values in your Visual Studio environment:
Step 1: Open Visual Studio Options
- Launch Visual Studio.
- Click on
Tools
in the menu bar. - Select
Options
from the dropdown list.
Step 2: Navigate to Debugging Settings
- In the Options dialog, expand the
Debugging
node in the left panel. - Click on the
General
option.
Step 3: Enable Inline Values
- In the General debug settings, look for the option labeled
Enable source link support
. - Ensure this box is checked.
- Next, find the option
Show all variables inline
and check this box.
Step 4: Save and Restart
- Click
OK
to save your settings. - Restart Visual Studio to apply the changes.
Step 5: Start Debugging
- Open your C# project and set breakpoints where you want to inspect variable values.
- Start debugging (F5) and begin stepping through your code using F10 (Step Over) or F11 (Step Into).
- You should now see the values of your variables displayed inline next to the code.
Example Code Snippet
Here’s a simple C# code snippet to illustrate inline debugging:
using System;
namespace InlineDebuggingDemo
{
class Program
{
static void Main(string[] args)
{
int x = 10;
int y = 20;
int sum = Add(x, y);
Console.WriteLine($"Sum of {x} and {y} is {sum}");
}
static int Add(int a, int b)
{
return a + b;
}
}
}
When you set a breakpoint in the Main
method and step through the code, you should see the values of x
, y
, and sum
inline beside their respective declarations.
Frequently Asked Questions
Can I view inline values on other IDEs?
While inline value viewing is a feature mostly associated with Visual Studio, other IDEs such as JetBrains Rider may offer similar functionality, but the settings will differ.
What if I still don't see inline values after enabling them?
If inline values do not appear after you've followed the steps, ensure that your Visual Studio is up-to-date, or try resetting your user settings to defaults.
Does inline viewing work with all data types?
Yes, inline viewing in Visual Studio typically works with primitive data types as well as objects, though complex types may require additional inspection through the Watch window.
Conclusion
Enabling inline variable values in Visual Studio can greatly enhance your debugging workflow by providing instant feedback without extra steps. By following the steps outlined in this article, you can configure your Visual Studio settings, allowing you to focus on code quality and bug fixing. Remember to ensure you’re in Debug mode and keep your IDE up to date for the best experience.