How to Build Your First REST API with Node.js and Express (From Someone Who Was Scared at First)
I still remember the first time I opened an empty app.js file in VS Code. No idea where to start. REST API? I barely understood what "route" meant. But I kept going, This is the guide I wish I had when I started my journey into backend development. 1. What is a REST API Think of a REST API like a restaurant experience. You (the client) sit at a table and look at a menu (the API documentation). When you're ready to order, you call over a waiter (send a request) who takes your order to the kitchen (server). The kitchen prepares your food (processes the request), and the waiter returns it (the response). In this analogy: GET is like asking, "What's the special today?" POST is like placing a new order PUT is like saying, "Actually, can I change my side dish?" DELETE is like canceling your order That's REST in a nutshell - a set of conventions for requesting and modifying resources over HTTP. 2. Setting Up Node.js and Express First, make sure you have Node.js installed (check with node -v in your terminal). Then: Create a new folder for your project and navigate to it: mkdir my-first-api cd my-first-api Initialize a Node.js project: npm init -y Install Express: npm install express Create your main file: touch app.js Open app.js and add the following code: const express = require('express'); const app = express(); const PORT = 3000; // Middleware to parse JSON bodies app.use(express.json()); app.listen(PORT, () => { console.log(`Server running on http://localhost:${PORT}`); }); 3. Creating Your First Route Let's add a simple welcome route to make sure everything's working: app.get('/api/welcome', (req, res) => { res.json({ message: "Hello, world!" }); }); Now run your server: node app.js Open your browser and navigate to http://localhost:3000/api/welcome. You should see: {"message":"Hello, world!"}. Congratulations! You've created your first API endpoint!
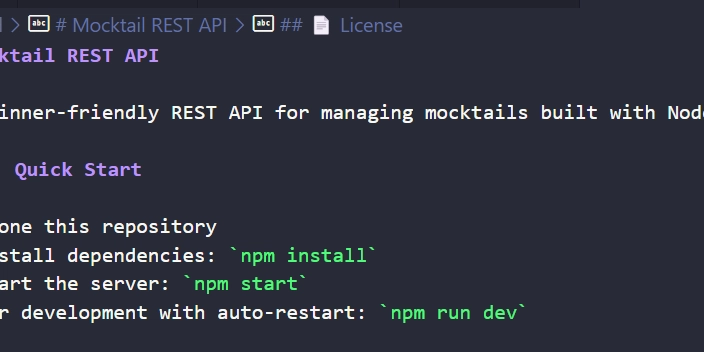
I still remember the first time I opened an empty app.js
file in VS Code. No idea where to start. REST API? I barely understood what "route" meant.
But I kept going, This is the guide I wish I had when I started my journey into backend development.
1. What is a REST API
Think of a REST API like a restaurant experience. You (the client) sit at a table and look at a menu (the API documentation). When you're ready to order, you call over a waiter (send a request) who takes your order to the kitchen (server). The kitchen prepares your food (processes the request), and the waiter returns it (the response).
In this analogy:
- GET is like asking, "What's the special today?"
- POST is like placing a new order
- PUT is like saying, "Actually, can I change my side dish?"
- DELETE is like canceling your order
That's REST in a nutshell - a set of conventions for requesting and modifying resources over HTTP.
2. Setting Up Node.js and Express
First, make sure you have Node.js installed (check with node -v
in your terminal). Then:
- Create a new folder for your project and navigate to it:
mkdir my-first-api
cd my-first-api
- Initialize a Node.js project:
npm init -y
- Install Express:
npm install express
- Create your main file:
touch app.js
- Open
app.js
and add the following code:
const express = require('express');
const app = express();
const PORT = 3000;
// Middleware to parse JSON bodies
app.use(express.json());
app.listen(PORT, () => {
console.log(`Server running on http://localhost:${PORT}`);
});
3. Creating Your First Route
Let's add a simple welcome route to make sure everything's working:
app.get('/api/welcome', (req, res) => {
res.json({ message: "Hello, world!" });
});
Now run your server:
node app.js
Open your browser and navigate to http://localhost:3000/api/welcome
. You should see: {"message":"Hello, world!"}
. Congratulations! You've created your first API endpoint!