How to Add Unique ID to Each Row in CSV with C#
Introduction In this article, we will explore how to assign a unique ID to each row when storing information into a CSV file using C# with the CsvHelper library. You are currently using the CsvHelper in a Blazor web app to append records to a CSV file but facing challenges in generating unique IDs for new entries. Let’s dive into a step-by-step guide on how to achieve this seamlessly. Understanding the Unique ID Requirement When working with datasets, ensuring that each record has a unique identifier is crucial for various reasons, such as data integrity and easy retrieval later. Since you are using a CSV file for storage, we need a solution that increments the ID automatically based on the existing records in your CSV file. This will help you avoid duplicate IDs and keep your data organized. Step-by-Step Solution to Add Unique IDs To implement a unique ID, we will modify your existing AddArtwork method. This method currently creates a new instance of Artwork and tries to append it without generating a proper unique identifier. Below are the changes you should make: 1. Read the Existing Data First, we need to read the current entries in your CSV file to determine the highest existing ID and increment from there. 2. Modify the AddArtwork Method Here’s the updated AddArtwork method: using Microsoft.EntityFrameworkCore; using BlazorApp.Models; using BlazorApp.Data; using CsvHelper; using System.Globalization; using CsvHelper.Configuration; using System.IO; using System.Linq; public async Task AddArtwork(Artwork artwork) { var existingArtworks = new List(); // Step 1: Read existing records to find the highest ID using (var reader = new StreamReader("Artworks.csv")) using (var csv = new CsvReader(reader, new CsvConfiguration(CultureInfo.InvariantCulture))) { existingArtworks = csv.GetRecords().ToList(); } // If there are existing records, find the maximum ID int newId = (existingArtworks.Any()) ? existingArtworks.Max(a => a.Id) + 1 : 1; // Step 2: Create a new artwork entry with a unique ID artwork.Id = newId; var records = new List { artwork }; bool append = true; // Step 3: Prepare CSV configuration var config = new CsvConfiguration(CultureInfo.InvariantCulture) { HasHeaderRecord = true, // Set to true if headers are required }; // Step 4: Write the new entry to the CSV using (var writer = new StreamWriter("Artworks.csv", append)) using (var csv = new CsvWriter(writer, config)) { // Check if header needs to be written if (existingArtworks.Count == 0) { csv.WriteHeader(); csv.NextRecord(); } csv.WriteRecords(records); } } 3. Explanation of the Changes Reading Existing Records: We use the CsvReader to read the contents of the Artworks.csv file, which allows us to retrieve existing records and determine the highest ID. Generating New ID: Using LINQ's Max method, we obtain the highest ID current in the list, then increment it by one to generate a new unique ID. If the list is empty, we start from 1. Writing Records: Finally, we adjust the CSV writing mechanism to check whether we need to write headers or not. Frequently Asked Questions (FAQ) 1. What happens if I delete a record? If you delete a record directly from the CSV file, the next entry will continue the ID sequence by incrementing from the maximum ID found, which means there could be 'gaps' in the ID sequence. If continuous IDs are essential, you might need to consider a more robust database solution. 2. Can I use a database instead of CSV? Yes, while CSV is suitable for small datasets, a database offers more flexibility regarding complex queries and data integrity. 3. What if two users try to add an entry simultaneously? To handle concurrent writes, consider using a database with transaction capabilities or implement file locking mechanisms when accessing the CSV file. Conclusion By implementing the above changes in your AddArtwork method, you will successfully add unique IDs to each artwork entry in your CSV file using C#. This will not only simplify your data management process but also maintain the integrity of your records. If you encounter further issues, don’t hesitate to reach out to the programming community for support. Happy coding!
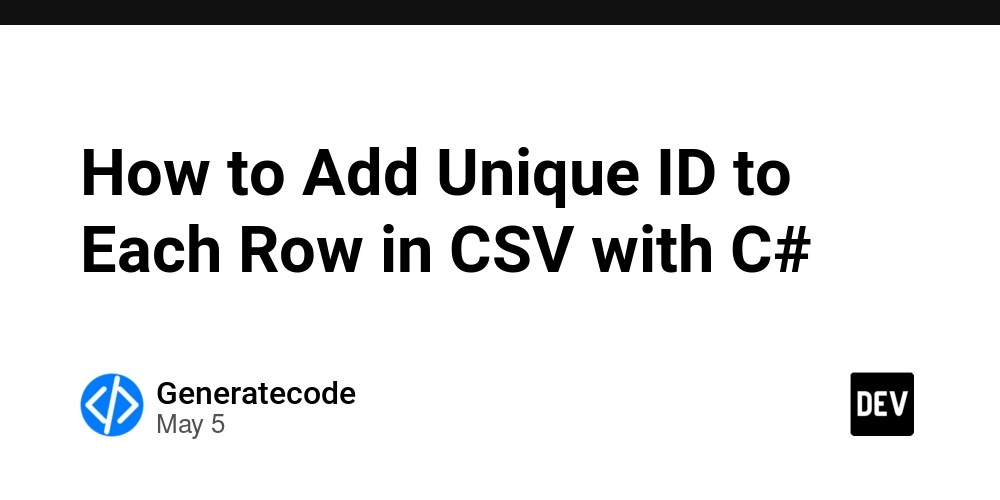
Introduction
In this article, we will explore how to assign a unique ID to each row when storing information into a CSV file using C# with the CsvHelper library. You are currently using the CsvHelper in a Blazor web app to append records to a CSV file but facing challenges in generating unique IDs for new entries. Let’s dive into a step-by-step guide on how to achieve this seamlessly.
Understanding the Unique ID Requirement
When working with datasets, ensuring that each record has a unique identifier is crucial for various reasons, such as data integrity and easy retrieval later. Since you are using a CSV file for storage, we need a solution that increments the ID automatically based on the existing records in your CSV file. This will help you avoid duplicate IDs and keep your data organized.
Step-by-Step Solution to Add Unique IDs
To implement a unique ID, we will modify your existing AddArtwork
method. This method currently creates a new instance of Artwork
and tries to append it without generating a proper unique identifier. Below are the changes you should make:
1. Read the Existing Data
First, we need to read the current entries in your CSV file to determine the highest existing ID and increment from there.
2. Modify the AddArtwork Method
Here’s the updated AddArtwork
method:
using Microsoft.EntityFrameworkCore;
using BlazorApp.Models;
using BlazorApp.Data;
using CsvHelper;
using System.Globalization;
using CsvHelper.Configuration;
using System.IO;
using System.Linq;
public async Task AddArtwork(Artwork artwork)
{
var existingArtworks = new List();
// Step 1: Read existing records to find the highest ID
using (var reader = new StreamReader("Artworks.csv"))
using (var csv = new CsvReader(reader, new CsvConfiguration(CultureInfo.InvariantCulture)))
{
existingArtworks = csv.GetRecords().ToList();
}
// If there are existing records, find the maximum ID
int newId = (existingArtworks.Any()) ? existingArtworks.Max(a => a.Id) + 1 : 1;
// Step 2: Create a new artwork entry with a unique ID
artwork.Id = newId;
var records = new List { artwork };
bool append = true;
// Step 3: Prepare CSV configuration
var config = new CsvConfiguration(CultureInfo.InvariantCulture)
{
HasHeaderRecord = true, // Set to true if headers are required
};
// Step 4: Write the new entry to the CSV
using (var writer = new StreamWriter("Artworks.csv", append))
using (var csv = new CsvWriter(writer, config))
{
// Check if header needs to be written
if (existingArtworks.Count == 0)
{
csv.WriteHeader();
csv.NextRecord();
}
csv.WriteRecords(records);
}
}
3. Explanation of the Changes
-
Reading Existing Records: We use the
CsvReader
to read the contents of theArtworks.csv
file, which allows us to retrieve existing records and determine the highest ID. -
Generating New ID: Using LINQ's
Max
method, we obtain the highest ID current in the list, then increment it by one to generate a new unique ID. If the list is empty, we start from 1. - Writing Records: Finally, we adjust the CSV writing mechanism to check whether we need to write headers or not.
Frequently Asked Questions (FAQ)
1. What happens if I delete a record?
If you delete a record directly from the CSV file, the next entry will continue the ID sequence by incrementing from the maximum ID found, which means there could be 'gaps' in the ID sequence. If continuous IDs are essential, you might need to consider a more robust database solution.
2. Can I use a database instead of CSV?
Yes, while CSV is suitable for small datasets, a database offers more flexibility regarding complex queries and data integrity.
3. What if two users try to add an entry simultaneously?
To handle concurrent writes, consider using a database with transaction capabilities or implement file locking mechanisms when accessing the CSV file.
Conclusion
By implementing the above changes in your AddArtwork
method, you will successfully add unique IDs to each artwork entry in your CSV file using C#. This will not only simplify your data management process but also maintain the integrity of your records. If you encounter further issues, don’t hesitate to reach out to the programming community for support. Happy coding!