Go's Garbage Collector: How It Keeps Your Code Clean
Hi there! I'm Shrijith Venkatrama, founder of Hexmos. Right now, I’m building LiveAPI, a tool that makes generating API docs from your code ridiculously easy. Garbage collection in Go is like an unsung hero that keeps your programs running smoothly by cleaning up unused memory. It’s a complex system, but it doesn’t have to feel like a black box. This article explains how Go’s garbage collector works in plain English with analogies, diverse examples, and a technical deep dive. Whether you’re a Go newbie or a seasoned developer, you’ll get a clear picture of what’s happening under the hood and how to work with it. What Is Garbage Collection in Simple Terms? Imagine a shared workspace with whiteboards (memory) used for brainstorming. As you scribble ideas, the boards fill up. If you don’t erase old, unused notes, you’ll run out of space for new ideas. The garbage collector (GC) is like an assistant who spots boards no longer needed and wipes them clean for reuse. In Go, when your program creates objects like slices, structs, or pointers, they take up memory. The GC’s job is to find and free memory that your program no longer references, preventing crashes due to memory exhaustion. Example: Imagine a function that processes temporary data: func processData() { temp := make([]int, 1000) // Allocates memory // Use temp for calculations } // temp goes out of scope When processData finishes, temp is no longer accessible. The GC will eventually free its memory. Why Go Needs a Garbage Collector Go prioritizes simplicity and concurrency, and manually managing memory (like in C) is error-prone. Forgetting to free memory can cause leaks, while freeing it too early can crash your program. The GC automates this, letting you focus on logic, especially in concurrent programs with goroutines. Table: Manual vs. Automatic Memory Management Feature Manual (C) Go’s GC Allocation Developer calls malloc Runtime handles it Deallocation Developer calls free GC frees unused memory Risk of Errors High (leaks, dangling pointers) Low (automated cleanup) Developer Effort High (track every allocation) Low (focus on code logic) The Big Picture: Go’s Concurrent, Tri-Color GC Go’s garbage collector is a concurrent, tri-color, mark-and-sweep, non-moving system. Let’s unpack that: Concurrent: Runs alongside your program, minimizing pauses. Tri-color: Uses white (potential garbage), gray (being checked), and black (in use) to track objects. Mark-and-sweep: Identifies used memory (mark) and frees unused memory (sweep). Non-moving: Doesn’t relocate objects in memory, keeping pointers stable. Think of it like sorting laundry: you tag clothes you’re keeping (mark), then toss the rest (sweep), all while still doing other chores (concurrent). Example: A web server creating temporary response buffers: func handleRequest() { buffer := make([]byte, 1024) // Heap-allocated // Write response to buffer } // buffer goes out of scope The GC tracks buffer and frees it after the function ends. For more, see Go’s GC Guide. How Mark-and-Sweep Works: Timing and Process The mark-and-sweep process has two distinct steps, and their timing is critical: Mark Activity: Happens when the GC cycle starts. The GC traces from "roots" (global variables, stack, goroutine stacks) to find all reachable objects. It marks them as “alive” using the tri-color system (white → gray → black). This runs concurrently with your program, but a brief stop-the-world pause (microseconds) occurs at the start to initialize. Sweep Activity: Occurs after marking is complete. The GC scans the heap and frees unmarked (white) objects, adding their memory to a free list. This also runs concurrently, with minimal disruption. A stop-the-world pause may happen at the cycle’s start or end, but Go optimizes these to be under a millisecond since Go 1.9. Example: A goroutine creating a struct: type Item struct { ID int Data string } func processItem() { item := &Item{ID: 1, Data: "example"} // Heap-allocated // Process item } // item goes out of scope The GC marks item as reachable during its lifetime. Once it’s out of scope, it’s unmarked and swept. Heap vs. Stack: What Does the GC Track? The GC only tracks heap allocations, not stack allocations. The Go compiler uses escape analysis to decide where objects live: Stack: Fast, temporary storage for variables that don’t “escape” their scope. Not tracked by GC. Heap: For objects that outlive their scope or are shared across goroutines. Tracked by GC. Table: Heap vs. Stack Allocations Code Example Location GC Tracked? x := 10 Stack No x := new(int) Heap Yes x := make([]int, 100) Heap Yes var x [100]int Stack No To see escape analysis: go build -gcflags='-m' main.go Example: A function returning a pointer: func createItem() *Item { return &Item{ID: 2, Data
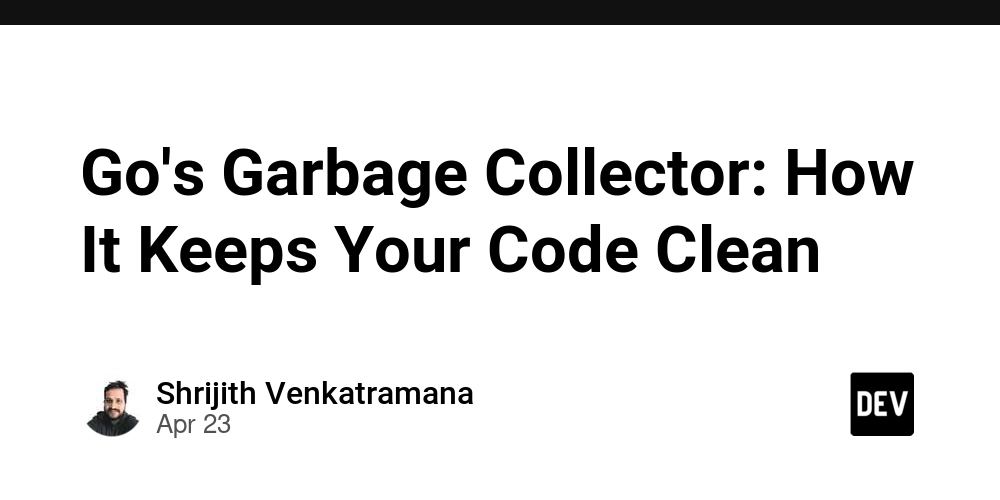
Hi there! I'm Shrijith Venkatrama, founder of Hexmos. Right now, I’m building LiveAPI, a tool that makes generating API docs from your code ridiculously easy.
Garbage collection in Go is like an unsung hero that keeps your programs running smoothly by cleaning up unused memory.
It’s a complex system, but it doesn’t have to feel like a black box.
This article explains how Go’s garbage collector works in plain English with analogies, diverse examples, and a technical deep dive.
Whether you’re a Go newbie or a seasoned developer, you’ll get a clear picture of what’s happening under the hood and how to work with it.
What Is Garbage Collection in Simple Terms?
Imagine a shared workspace with whiteboards (memory) used for brainstorming. As you scribble ideas, the boards fill up. If you don’t erase old, unused notes, you’ll run out of space for new ideas. The garbage collector (GC) is like an assistant who spots boards no longer needed and wipes them clean for reuse.
In Go, when your program creates objects like slices, structs, or pointers, they take up memory. The GC’s job is to find and free memory that your program no longer references, preventing crashes due to memory exhaustion.
Example: Imagine a function that processes temporary data:
func processData() {
temp := make([]int, 1000) // Allocates memory
// Use temp for calculations
} // temp goes out of scope
When processData
finishes, temp
is no longer accessible. The GC will eventually free its memory.
Why Go Needs a Garbage Collector
Go prioritizes simplicity and concurrency, and manually managing memory (like in C) is error-prone. Forgetting to free memory can cause leaks, while freeing it too early can crash your program. The GC automates this, letting you focus on logic, especially in concurrent programs with goroutines.
Table: Manual vs. Automatic Memory Management
Feature | Manual (C) | Go’s GC |
---|---|---|
Allocation | Developer calls malloc
|
Runtime handles it |
Deallocation | Developer calls free
|
GC frees unused memory |
Risk of Errors | High (leaks, dangling pointers) | Low (automated cleanup) |
Developer Effort | High (track every allocation) | Low (focus on code logic) |
The Big Picture: Go’s Concurrent, Tri-Color GC
Go’s garbage collector is a concurrent, tri-color, mark-and-sweep, non-moving system. Let’s unpack that:
- Concurrent: Runs alongside your program, minimizing pauses.
- Tri-color: Uses white (potential garbage), gray (being checked), and black (in use) to track objects.
- Mark-and-sweep: Identifies used memory (mark) and frees unused memory (sweep).
- Non-moving: Doesn’t relocate objects in memory, keeping pointers stable.
Think of it like sorting laundry: you tag clothes you’re keeping (mark), then toss the rest (sweep), all while still doing other chores (concurrent).
Example: A web server creating temporary response buffers:
func handleRequest() {
buffer := make([]byte, 1024) // Heap-allocated
// Write response to buffer
} // buffer goes out of scope
The GC tracks buffer
and frees it after the function ends.
For more, see Go’s GC Guide.
How Mark-and-Sweep Works: Timing and Process
The mark-and-sweep process has two distinct steps, and their timing is critical:
- Mark Activity: Happens when the GC cycle starts. The GC traces from "roots" (global variables, stack, goroutine stacks) to find all reachable objects. It marks them as “alive” using the tri-color system (white → gray → black). This runs concurrently with your program, but a brief stop-the-world pause (microseconds) occurs at the start to initialize.
- Sweep Activity: Occurs after marking is complete. The GC scans the heap and frees unmarked (white) objects, adding their memory to a free list. This also runs concurrently, with minimal disruption.
A stop-the-world pause may happen at the cycle’s start or end, but Go optimizes these to be under a millisecond since Go 1.9.
Example: A goroutine creating a struct:
type Item struct {
ID int
Data string
}
func processItem() {
item := &Item{ID: 1, Data: "example"} // Heap-allocated
// Process item
} // item goes out of scope
The GC marks item
as reachable during its lifetime. Once it’s out of scope, it’s unmarked and swept.
Heap vs. Stack: What Does the GC Track?
The GC only tracks heap allocations, not stack allocations. The Go compiler uses escape analysis to decide where objects live:
- Stack: Fast, temporary storage for variables that don’t “escape” their scope. Not tracked by GC.
- Heap: For objects that outlive their scope or are shared across goroutines. Tracked by GC.
Table: Heap vs. Stack Allocations
Code Example | Location | GC Tracked? |
---|---|---|
x := 10 |
Stack | No |
x := new(int) |
Heap | Yes |
x := make([]int, 100) |
Heap | Yes |
var x [100]int |
Stack | No |
To see escape analysis:
go build -gcflags='-m' main.go
Example: A function returning a pointer:
func createItem() *Item {
return &Item{ID: 2, Data: "shared"} // Escapes to heap
}
Since the Item
is returned, it’s heap-allocated and tracked by the GC.
When and Why the GC Runs
The GC runs based on heap growth or time intervals:
-
Heap Trigger: When the heap grows to a multiple of its size after the last GC (controlled by
GOGC
). DefaultGOGC=100
means GC runs when the heap doubles. - Periodic Check: Roughly every 2 minutes, the GC checks if it’s needed.
You can tweak this with GOGC
:
GOGC=50 go run main.go # More frequent GC
GOGC=200 go run main.go # Less frequent GC
Example: A loop creating large slices:
func generateData() {
for i := 0; i < 100; i++ {
data := make([]int, 10000) // Heap-allocated
_ = data
}
}
Large allocations trigger the GC more often unless you increase GOGC
.
See GOGC Tuning Guide.
Writing GC-Friendly Code
To reduce GC workload:
- Reuse buffers: Avoid repeated allocations.
-
Use
sync.Pool
: For frequently used objects. - Clear references: Don’t hold onto objects in caches unnecessarily.
- Minimize pointers: Fewer pointers mean less for the GC to trace.
Example: Using sync.Pool
var pool = sync.Pool{
New: func() interface{} {
return make([]byte, 1024)
},
}
func process() {
buf := pool.Get().([]byte)
// Use buf
pool.Put(buf) // Return to pool
}
This reuses buffers, reducing heap allocations.
Learn more about sync.Pool.
Tuning and Monitoring the GC
You can monitor and tune the GC:
-
GOGC
: Adjusts GC frequency (lower = more frequent, higher = less frequent). -
runtime.MemStats
: Shows heap size, GC cycles, and pauses. -
pprof
: Visualizes memory usage and GC behavior.
Example: Checking GC Stats
import (
"fmt"
"runtime"
)
func main() {
var ms runtime.MemStats
runtime.ReadMemStats(&ms)
fmt.Printf("HeapAlloc: %v bytes, GCCount: %v\n", ms.HeapAlloc, ms.NumGC)
}
To visualize with pprof:
go tool pprof -http=:8080 ./yourbinary heap.prof
See Go’s pprof guide.
Technical Deep Dive: Under the Hood
For the curious, here’s the nitty-gritty:
- Write Barrier: During marking, the GC uses a write barrier to track pointer updates, ensuring no reachable objects are missed.
-
Pacing Algorithm: Balances GC frequency by predicting heap growth based on allocation rates and
GOGC
. - Tri-Color Algorithm: Objects move from white (unchecked) to gray (being traced) to black (alive). This enables concurrent marking.
- Non-Moving: Objects stay in place, simplifying pointer management but potentially fragmenting memory.
Example: Closure Capturing Variables
func makeCounter() func() int {
count := 0 // Escapes to heap
return func() int {
count++
return count
}
}
The count
variable is heap-allocated because it’s captured by the closure, and the GC tracks it.
Explore the Go source code for implementation details.
In Conclusion...
I hope this article gave you a practical and friendly guide to Go’s garbage collector.
By understanding its mechanics and following GC-friendly practices, you can write more efficient Go programs.
Questions or want to dive deeper? Let me know!