DevOps Made Simple: A Beginner’s Guide to Deploying a Scalable Web App on AWS with Terraform & Docker
Introduction In today’s fast-paced software development landscape, deploying scalable web applications efficiently is a crucial skill for DevOps engineers. AWS, Terraform, and Docker are three powerful technologies that simplify infrastructure management, automation, and containerization. In this beginner-friendly guide, we’ll walk through the process of deploying a scalable web application on AWS using Terraform and Docker. Whether you’re new to DevOps or looking to refine your skills, this guide will help you understand and implement a real-world deployment scenario. Understanding the Core Technologies 1. AWS Amazon Web Services (AWS) provides cloud computing solutions, making it easy to deploy, manage, and scale applications. 2. Terraform Terraform is an Infrastructure-as-Code (IaC) tool that automates the provisioning of cloud resources. 3. Docker Docker is a containerization tool that enables developers to package applications along with their dependencies, ensuring consistency across environments. Step-by-Step Guide to Deployment Step 1: Setting Up AWS Credentials Before using Terraform, configure your AWS credentials to allow Terraform to access your AWS account. aws configure Provide your AWS Access Key, Secret Key, Region, and Output Format. Step 2: Writing a Terraform Script for AWS Infrastructure Create a Terraform script (main.tf) to define AWS resources such as EC2 instances and security groups. provider "aws" { region = "us-east-1" } resource "aws_instance" "web" { ami = "ami-12345678" instance_type = "t2.micro" key_name = "my-key" tags = { Name = "Terraform-Docker-Instance" } } Step 3: Initializing and Applying Terraform Run the following Terraform commands to set up the infrastructure: terraform init terraform apply -auto-approve This will provision an EC2 instance on AWS. Step 4: Installing Docker on the AWS Instance SSH into the instance and install Docker: ssh -i my-key.pem ec2-user@ Then, run the following commands: sudo yum update -y sudo yum install docker -y sudo systemctl start docker sudo systemctl enable docker Step 5: Running a Dockerized Web App Create a simple Dockerfile for a Node.js web application: FROM node:14 WORKDIR /app COPY . . RUN npm install CMD ["node", "server.js"] EXPOSE 3000 Build and run the Docker container: docker build -t my-web-app . docker run -d -p 80:3000 my-web-app Real-World Applications Deploying web applications with AWS, Terraform, and Docker is widely used in production environments. Some real-world use cases include: Microservices Deployment: Running scalable microservices in isolated Docker containers. CI/CD Pipelines: Automating deployments with Terraform and integrating Dockerized applications. Multi-Cloud Deployments: Using Terraform to provision resources across different cloud providers. Common Mistakes & Best Practices Mistakes to Avoid Hardcoding AWS Credentials: Always use environment variables or IAM roles. Ignoring Security Groups: Ensure correct inbound/outbound rules to prevent security vulnerabilities. Not Using State Management: Store Terraform state files remotely (e.g., in AWS S3) for collaboration. Best Practices Use modular Terraform scripts to keep configurations clean and reusable. Follow Docker best practices, such as using lightweight base images. Implement auto-scaling and load balancing for high availability. Conclusion & Call to Action Deploying a scalable web application on AWS using Terraform and Docker is an essential skill for DevOps engineers. With the step-by-step guide, real-world applications, and best practices outlined in this post, you can confidently implement these technologies in your projects. Do you have any questions or want to share your experience? Drop a comment below and let’s discuss! Also, check out more DevOps tutorials to deepen your knowledge. Happy coding!
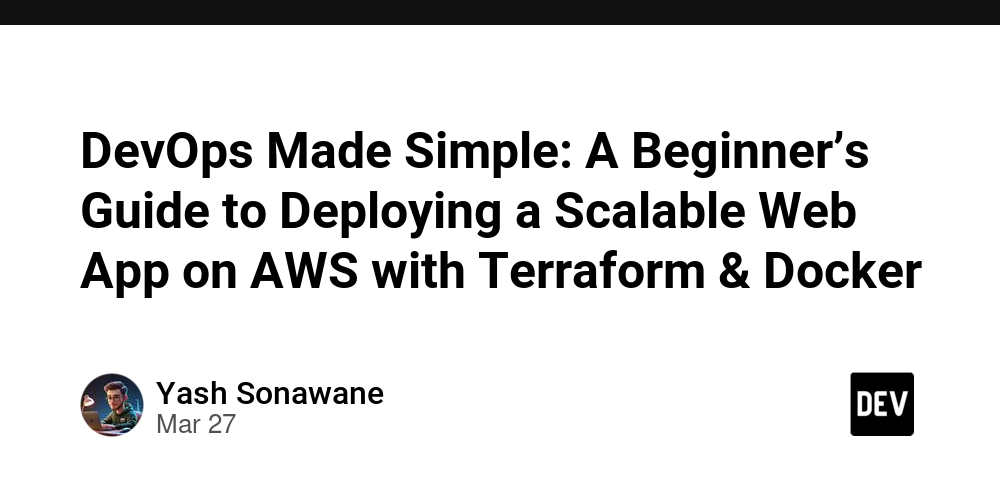
Introduction
In today’s fast-paced software development landscape, deploying scalable web applications efficiently is a crucial skill for DevOps engineers. AWS, Terraform, and Docker are three powerful technologies that simplify infrastructure management, automation, and containerization.
In this beginner-friendly guide, we’ll walk through the process of deploying a scalable web application on AWS using Terraform and Docker. Whether you’re new to DevOps or looking to refine your skills, this guide will help you understand and implement a real-world deployment scenario.
Understanding the Core Technologies
1. AWS
Amazon Web Services (AWS) provides cloud computing solutions, making it easy to deploy, manage, and scale applications.
2. Terraform
Terraform is an Infrastructure-as-Code (IaC) tool that automates the provisioning of cloud resources.
3. Docker
Docker is a containerization tool that enables developers to package applications along with their dependencies, ensuring consistency across environments.
Step-by-Step Guide to Deployment
Step 1: Setting Up AWS Credentials
Before using Terraform, configure your AWS credentials to allow Terraform to access your AWS account.
aws configure
Provide your AWS Access Key, Secret Key, Region, and Output Format.
Step 2: Writing a Terraform Script for AWS Infrastructure
Create a Terraform script (main.tf
) to define AWS resources such as EC2 instances and security groups.
provider "aws" {
region = "us-east-1"
}
resource "aws_instance" "web" {
ami = "ami-12345678"
instance_type = "t2.micro"
key_name = "my-key"
tags = {
Name = "Terraform-Docker-Instance"
}
}
Step 3: Initializing and Applying Terraform
Run the following Terraform commands to set up the infrastructure:
terraform init
terraform apply -auto-approve
This will provision an EC2 instance on AWS.
Step 4: Installing Docker on the AWS Instance
SSH into the instance and install Docker:
ssh -i my-key.pem ec2-user@
Then, run the following commands:
sudo yum update -y
sudo yum install docker -y
sudo systemctl start docker
sudo systemctl enable docker
Step 5: Running a Dockerized Web App
Create a simple Dockerfile
for a Node.js web application:
FROM node:14
WORKDIR /app
COPY . .
RUN npm install
CMD ["node", "server.js"]
EXPOSE 3000
Build and run the Docker container:
docker build -t my-web-app .
docker run -d -p 80:3000 my-web-app
Real-World Applications
Deploying web applications with AWS, Terraform, and Docker is widely used in production environments. Some real-world use cases include:
- Microservices Deployment: Running scalable microservices in isolated Docker containers.
- CI/CD Pipelines: Automating deployments with Terraform and integrating Dockerized applications.
- Multi-Cloud Deployments: Using Terraform to provision resources across different cloud providers.
Common Mistakes & Best Practices
Mistakes to Avoid
- Hardcoding AWS Credentials: Always use environment variables or IAM roles.
- Ignoring Security Groups: Ensure correct inbound/outbound rules to prevent security vulnerabilities.
- Not Using State Management: Store Terraform state files remotely (e.g., in AWS S3) for collaboration.
Best Practices
- Use modular Terraform scripts to keep configurations clean and reusable.
- Follow Docker best practices, such as using lightweight base images.
- Implement auto-scaling and load balancing for high availability.
Conclusion & Call to Action
Deploying a scalable web application on AWS using Terraform and Docker is an essential skill for DevOps engineers. With the step-by-step guide, real-world applications, and best practices outlined in this post, you can confidently implement these technologies in your projects.
Do you have any questions or want to share your experience? Drop a comment below and let’s discuss! Also, check out more DevOps tutorials to deepen your knowledge.
Happy coding!