Day-07: Unpacking Java: How Classes, Objects, and Methods Work Together
Java: From Source Code to Execution Compilation (Source Code to Byte Code) Source Code → Written in Java, with human-readable syntax. Compiler → Translates the source code into Byte Code. Compile Time → Occurs when the Java compiler processes the source code. Execution (Byte Code to Binary Code) Byte Code → The intermediate code generated by the compiler. JRE (Java Runtime Environment) → Interprets the byte code and converts it into machine-readable binary code. Run Time → Happens when the program is executed by the JRE. JDK (Java Development Kit) The JDK contains the Compiler and JRE for both compiling and running Java applications. Classes, Objects, and Methods in Real-Time Example Class A class is a blueprint or template for creating objects. It defines properties (attributes) and methods (functions) that the objects created from the class can have. **Real-world analogy:** **A Class can be compared to a Company,** which defines what an employee can do but doesn't perform tasks itself. Object An object is an instance of a class. It represents a real-world entity created from the class, and it holds the state and behaviors defined in the class. ** Real-world analogy:** An Object is like an Employee in a company. The employee is an actual worker who performs tasks, which are defined by the company's blueprint. Method A method defines the actions or tasks that objects can perform. It's like a function that operates on the data of the object. ** Real-world analogy:** A Method is like a Task that an employee performs based on their role in the company. For example, the task could be to process documents or manage finances. Example Code: Calculator Class In the following example, we'll define a Calculator class with methods that represent tasks like adding two numbers. public class Calculator { // Main method: The entry point of the program public static void main(String[] args) { Calculator casio = new Calculator(); // Creating an object 'casio' of the Calculator class casio.add(10, 20); // Calling the 'add' method with 10 and 20 as parameters } // Method to add two numbers public void add(int num1, int num2) { System.out.println(num1 + num2); // Printing the result of the addition } // You can define other methods like subtract, multiply, divide here } Breakdown of the Code Class: Calculator This class serves as the blueprint. It defines methods like add which will perform specific tasks. Object: casio casio is an object created from the Calculator class. It's the physical representation of the class and can perform the tasks defined in it. Method: add The add method takes two parameters (num1 and num2) and outputs their sum. This method defines the task the casio object performs. Summary: A Class is a blueprint or template. An Object is a real-world instance of a class. A Method defines tasks that objects can perform.
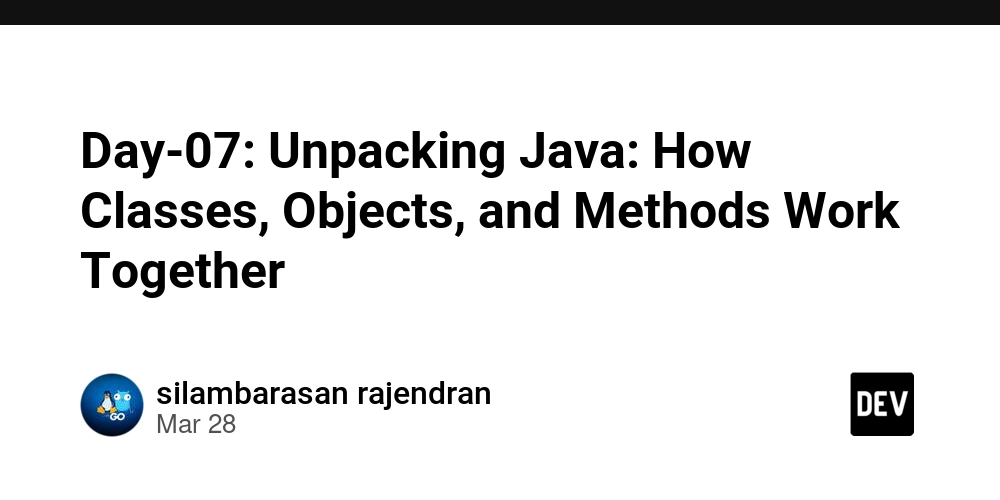
Java: From Source Code to Execution
Compilation (Source Code to Byte Code)
Source Code → Written in Java, with human-readable syntax.
Compiler → Translates the source code into Byte Code.
Compile Time → Occurs when the Java compiler processes the source code.
Execution (Byte Code to Binary Code)
Byte Code → The intermediate code generated by the compiler.
JRE (Java Runtime Environment) → Interprets the byte code and converts it into machine-readable binary code.
Run Time → Happens when the program is executed by the JRE.
JDK (Java Development Kit)
The JDK contains the Compiler and JRE for both compiling and running Java applications.
Classes, Objects, and Methods in Real-Time Example
Class
A class is a blueprint or template for creating objects. It defines properties (attributes) and methods (functions) that the objects created from the class can have.
**Real-world analogy:**
**A Class can be compared to a Company,** which defines what an employee can do but doesn't perform tasks itself.
Object
An object is an instance of a class. It represents a real-world entity created from the class, and it holds the state and behaviors defined in the class.
** Real-world analogy:**
An Object is like an Employee in a company. The employee is an actual worker who performs tasks, which are defined by the company's blueprint.
Method
A method defines the actions or tasks that objects can perform. It's like a function that operates on the data of the object.
** Real-world analogy:**
A Method is like a Task that an employee performs based on their role in the company. For example, the task could be to process documents or manage finances.
Example Code: Calculator Class
In the following example, we'll define a Calculator class with methods that represent tasks like adding two numbers.
public class Calculator {
// Main method: The entry point of the program
public static void main(String[] args) {
Calculator casio = new Calculator(); // Creating an object 'casio' of the Calculator class
casio.add(10, 20); // Calling the 'add' method with 10 and 20 as parameters
}
// Method to add two numbers
public void add(int num1, int num2) {
System.out.println(num1 + num2); // Printing the result of the addition
}
// You can define other methods like subtract, multiply, divide here
}
Breakdown of the Code
Class: Calculator
This class serves as the blueprint. It defines methods like add which will perform specific tasks.
Object: casio
casio is an object created from the Calculator class. It's the physical representation of the class and can perform the tasks defined in it.
Method: add
The add method takes two parameters (num1 and num2) and outputs their sum. This method defines the task the casio object performs.
Summary:
A Class is a blueprint or template.
An Object is a real-world instance of a class.
A Method defines tasks that objects can perform.