CSS Performance Tips: Writing Faster, More Efficient Styles
In the fast-paced world of frontend development, writing performant CSS can significantly impact your app's speed and user experience. Poorly optimized CSS may cause layout shifts, slow rendering, and increased load times. This article explores actionable CSS performance tips to help you write cleaner, faster, and more efficient styles. 1. Avoid Universal Selectors and Overly Deep Nesting Deeply nested CSS selectors or using universal selectors (e.g., *) can degrade performance because browsers have to traverse the DOM more extensively. Bad Example: /* Overly specific and nested selectors */ .container .header .nav .link { color: blue; } Better Example: /* Keep it flat and less specific */ .nav-link { color: blue; } 2. Use Logical Properties for Layout Efficiency CSS logical properties like margin-inline, padding-block, and inset improve maintainability and can handle left-to-right (LTR) and right-to-left (RTL) layouts automatically. Example: /* Logical properties replace traditional directional properties */ .container { margin-inline: 16px; /* Handles both left and right margins */ padding-block: 8px; /* Applies to both top and bottom padding */ } 3. Minimize Reflows with CSS Grid and Flexbox Reflows occur when the DOM has to recalculate element positions. Using modern layout techniques like CSS Grid and Flexbox can minimize reflows and improve rendering speed. Grid Example: .grid { display: grid; grid-template-columns: repeat(3, 1fr); /* Efficiently handles dynamic layout */ } 4. Leverage CSS Variables (Custom Properties) CSS variables reduce duplication and enhance performance by enabling real-time updates without recalculating the entire stylesheet. Example: :root { --primary-color: #4CAF50; } .button { background-color: var(--primary-color); } 5. Use Hardware-Accelerated Animations For smoother animations, animate properties like transform and opacity, which utilize the GPU instead of the CPU. Bad Example (CPU-bound animation): .element { animation: move 2s infinite; } @keyframes move { from { left: 0px; } to { left: 100px; } } Better Example (GPU-accelerated animation): .element { animation: move 2s infinite; } @keyframes move { from { transform: translateX(0); } to { transform: translateX(100px); } } 6. Defer Non-Critical CSS Load only critical CSS upfront and defer non-essential styles using techniques like media queries or dynamic imports. Example: 7. Reduce Unused CSS (Tree-Shaking) Use tools like PurgeCSS or Tailwind CSS to eliminate unused CSS and reduce file size. 8. Optimize Images and Fonts in CSS Large background images or unoptimized fonts can slow down your styles. Use webp or avif formats for background images. Apply font-display: swap; for faster font rendering. Final Thoughts By applying these CSS performance tips, you can improve page load speed, minimize layout shifts, and enhance the overall user experience. Remember, performant CSS is as much about simplicity as it is about leveraging modern techniques.
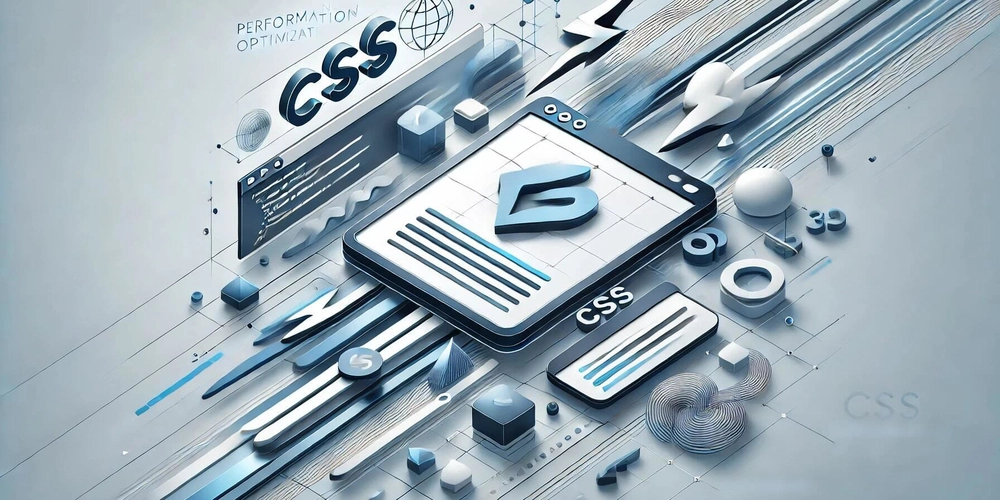
In the fast-paced world of frontend development, writing performant CSS can significantly impact your app's speed and user experience. Poorly optimized CSS may cause layout shifts, slow rendering, and increased load times. This article explores actionable CSS performance tips to help you write cleaner, faster, and more efficient styles.
1. Avoid Universal Selectors and Overly Deep Nesting
Deeply nested CSS selectors or using universal selectors (e.g., *
) can degrade performance because browsers have to traverse the DOM more extensively.
Bad Example:
/* Overly specific and nested selectors */
.container .header .nav .link {
color: blue;
}
Better Example:
/* Keep it flat and less specific */
.nav-link {
color: blue;
}
2. Use Logical Properties for Layout Efficiency
CSS logical properties like margin-inline
, padding-block
, and inset
improve maintainability and can handle left-to-right (LTR) and right-to-left (RTL) layouts automatically.
Example:
/* Logical properties replace traditional directional properties */
.container {
margin-inline: 16px; /* Handles both left and right margins */
padding-block: 8px; /* Applies to both top and bottom padding */
}
3. Minimize Reflows with CSS Grid and Flexbox
Reflows occur when the DOM has to recalculate element positions. Using modern layout techniques like CSS Grid
and Flexbox
can minimize reflows and improve rendering speed.
Grid Example:
.grid {
display: grid;
grid-template-columns: repeat(3, 1fr); /* Efficiently handles dynamic layout */
}
4. Leverage CSS Variables (Custom Properties)
CSS variables reduce duplication and enhance performance by enabling real-time updates without recalculating the entire stylesheet.
Example:
:root {
--primary-color: #4CAF50;
}
.button {
background-color: var(--primary-color);
}
5. Use Hardware-Accelerated Animations
For smoother animations, animate properties like transform
and opacity
, which utilize the GPU instead of the CPU.
Bad Example (CPU-bound animation):
.element {
animation: move 2s infinite;
}
@keyframes move {
from { left: 0px; }
to { left: 100px; }
}
Better Example (GPU-accelerated animation):
.element {
animation: move 2s infinite;
}
@keyframes move {
from { transform: translateX(0); }
to { transform: translateX(100px); }
}
6. Defer Non-Critical CSS
Load only critical CSS upfront and defer non-essential styles using techniques like media
queries or dynamic imports.
Example:
rel="stylesheet" href="critical-styles.css">
rel="stylesheet" href="non-critical-styles.css" media="print" onload="this.media='all';">
7. Reduce Unused CSS (Tree-Shaking)
Use tools like PurgeCSS or Tailwind CSS to eliminate unused CSS and reduce file size.
8. Optimize Images and Fonts in CSS
Large background images or unoptimized fonts can slow down your styles.
- Use
webp
oravif
formats for background images. - Apply
font-display: swap;
for faster font rendering.
Final Thoughts
By applying these CSS performance tips, you can improve page load speed, minimize layout shifts, and enhance the overall user experience. Remember, performant CSS is as much about simplicity as it is about leveraging modern techniques.