Create Your Own JavaScript Game: Memory Match in Under 100 Lines
Gamification grabs attention! If you’ve ever wanted to build your own game using JavaScript, a memory match game is a perfect beginner-friendly project that’s both fun and practical. With fewer than 100 lines of code, you can develop an interactive and rewarding experience for players—no frameworks needed. Whether you're learning JavaScript or just want to sharpen your DOM manipulation skills, this project will get you hands-on with: HTML/CSS layout JavaScript event handling Shuffling arrays Matching logic By the end, you’ll not only have a fully functional game but also the confidence to expand or even customize it your own way. What You’ll Build A Memory Match Game (a.k.a. Concentration) where: Players flip two cards at a time. If the cards match, they stay flipped. If not, they flip back after a short delay. The goal is to match all pairs. Project Setup Here’s a super minimal file structure: memory-game/ │ ├── index.html ├── style.css └── script.js 1. index.html Memory Match Memory Match 2. style.css body { font-family: sans-serif; display: flex; flex-direction: column; align-items: center; } h1 { margin-top: 20px; } .game-board { display: grid; grid-template-columns: repeat(4, 100px); gap: 10px; margin-top: 30px; } .card { width: 100px; height: 100px; background: #444; color: white; display: flex; align-items: center; justify-content: center; font-size: 24px; cursor: pointer; user-select: none; } .card.flipped { background: #0f0; } 3. script.js const board = document.getElementById("game-board"); const values = ["A", "B", "C", "D", "E", "F", "G", "H"]; let cards = [...values, ...values].sort(() => 0.5 - Math.random()); let firstCard = null; let lock = false; cards.forEach(val => { const card = document.createElement("div"); card.classList.add("card"); card.dataset.value = val; card.textContent = ""; board.appendChild(card); card.addEventListener("click", () => { if (lock || card.classList.contains("flipped")) return; card.textContent = val; card.classList.add("flipped"); if (!firstCard) { firstCard = card; } else { if (firstCard.dataset.value === card.dataset.value) { firstCard = null; } else { lock = true; setTimeout(() => { firstCard.textContent = ""; card.textContent = ""; firstCard.classList.remove("flipped"); card.classList.remove("flipped"); firstCard = null; lock = false; }, 1000); } } }); }); Expand It! Once you're done, try adding: Move counter or timer Restart button Scoreboard Sound effects or animations And guess what? This is just one of the 200 JavaScript projects waiting for you. Want More Projects Like This? If you enjoyed this and want to supercharge your learning with real-world apps, check out my ebook: 200 JavaScript Projects: Build, Learn & Master Real-World Apps You'll get: Beginner to advanced projects Code snippets Practical use cases Organized chapters for easy reference Start building. Start mastering JavaScript!
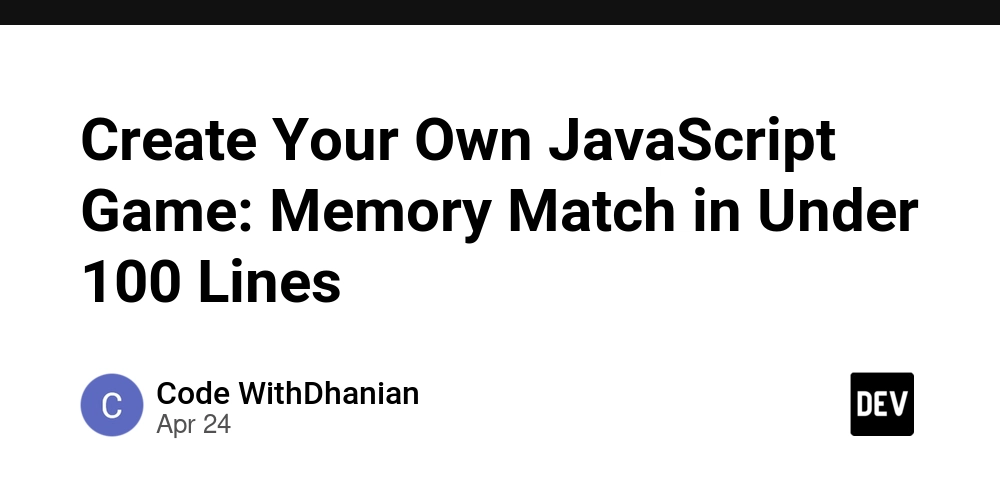
Gamification grabs attention!
If you’ve ever wanted to build your own game using JavaScript, a memory match game is a perfect beginner-friendly project that’s both fun and practical. With fewer than 100 lines of code, you can develop an interactive and rewarding experience for players—no frameworks needed.
Whether you're learning JavaScript or just want to sharpen your DOM manipulation skills, this project will get you hands-on with:
- HTML/CSS layout
- JavaScript event handling
- Shuffling arrays
- Matching logic
By the end, you’ll not only have a fully functional game but also the confidence to expand or even customize it your own way.
What You’ll Build
A Memory Match Game (a.k.a. Concentration) where:
- Players flip two cards at a time.
- If the cards match, they stay flipped.
- If not, they flip back after a short delay.
- The goal is to match all pairs.
Project Setup
Here’s a super minimal file structure:
memory-game/
│
├── index.html
├── style.css
└── script.js
1. index.html
lang="en">
charset="UTF-8" />
name="viewport" content="width=device-width, initial-scale=1.0" />
Memory Match
rel="stylesheet" href="style.css" />
Memory Match
class="game-board" id="game-board">
2. style.css
body {
font-family: sans-serif;
display: flex;
flex-direction: column;
align-items: center;
}
h1 {
margin-top: 20px;
}
.game-board {
display: grid;
grid-template-columns: repeat(4, 100px);
gap: 10px;
margin-top: 30px;
}
.card {
width: 100px;
height: 100px;
background: #444;
color: white;
display: flex;
align-items: center;
justify-content: center;
font-size: 24px;
cursor: pointer;
user-select: none;
}
.card.flipped {
background: #0f0;
}
3. script.js
const board = document.getElementById("game-board");
const values = ["A", "B", "C", "D", "E", "F", "G", "H"];
let cards = [...values, ...values].sort(() => 0.5 - Math.random());
let firstCard = null;
let lock = false;
cards.forEach(val => {
const card = document.createElement("div");
card.classList.add("card");
card.dataset.value = val;
card.textContent = "";
board.appendChild(card);
card.addEventListener("click", () => {
if (lock || card.classList.contains("flipped")) return;
card.textContent = val;
card.classList.add("flipped");
if (!firstCard) {
firstCard = card;
} else {
if (firstCard.dataset.value === card.dataset.value) {
firstCard = null;
} else {
lock = true;
setTimeout(() => {
firstCard.textContent = "";
card.textContent = "";
firstCard.classList.remove("flipped");
card.classList.remove("flipped");
firstCard = null;
lock = false;
}, 1000);
}
}
});
});
Expand It!
Once you're done, try adding:
- Move counter or timer
- Restart button
- Scoreboard
- Sound effects or animations
And guess what? This is just one of the 200 JavaScript projects waiting for you.
Want More Projects Like This?
If you enjoyed this and want to supercharge your learning with real-world apps, check out my ebook:
200 JavaScript Projects: Build, Learn & Master Real-World Apps
You'll get:
- Beginner to advanced projects
- Code snippets
- Practical use cases
- Organized chapters for easy reference
Start building. Start mastering JavaScript!