How to Build a WhatsApp Marketing Automation Tool Using Node.js and the WhatsApp Business API
WhatsApp has become one of the most popular messaging apps worldwide. With over 2 billion active users, it’s now a key channel for marketing. Businesses see the value in sending personalized, timely messages straight to customers’ phones. Automating these messages saves time, boosts engagement, and can lead to more sales. If you want to create your own Bulk WhatsApp marketing tool, using Node.js and the WhatsApp Business API is a smart move. This step-by-step guide walks you through building a custom automation system from scratch. Ready to get started? Let’s dive in. Understanding WhatsApp Business API and Its Marketing Potential Overview of WhatsApp Business API The WhatsApp Business API is a powerful tool for businesses. Unlike the regular WhatsApp Business app, it’s designed for large-scale communication. It allows companies to send messages, receive responses, and automate conversations. The API supports templates, quick replies, and media sharing. This API is especially useful for customer support, updates, and marketing campaigns. It lets you send personalized offers or reminders without manual effort. Imagine sending hundreds of follow-up messages automatically—it’s possible with this API. Benefits of Using WhatsApp for Marketing WhatsApp gets high open rates—up to 98%. That means most people see your message if you send it on the right platform. Engagement rates are also high because customers prefer messaging over email or calls. Look at KLM Airlines: they use WhatsApp to confirm bookings and send updates. It’s easy for customers to reply, and airlines get quick feedback. But, remember, there are rules. You need user consent, and messages must follow WhatsApp’s policies. Requirements for Integration *To connect WhatsApp API to your system, you need a few things: * Business verification on Facebook Business Manager Approval from Facebook or a WhatsApp API provider A server with Node.js installed An SSL certificate for secure communication Once you get API access, you can start building your custom tool. Setting Up the Development Environment Installing and Configuring Node.js First, download Node.js from the official website. Follow the simple instructions for your operating system. After installation, open your terminal and run: node -v npm -v This checks if Node.js and npm (Node package manager) are ready. For editing code, I recommend Visual Studio Code—it’s free and user-friendly. Securing API Access Register your business on Facebook Business Manager and apply for WhatsApp API access. Once approved, generate your API tokens. Keep these secrets safe—they give access to your account. Use environment variables to store credentials securely in your code. Essential Libraries and Tools For HTTP requests, use axios. It simplifies sending messages and fetching data. Also, set up a .env file to store secrets like API tokens safely. Optional tools include SDKs or wrappers that make API calls easier. Connecting to the WhatsApp Business API with Node.js Establishing a Secure Connection Your app needs to communicate securely over HTTPS. Set the appropriate headers, including your API token, for each request. The API endpoints are usually like https://your-server/v1/messages for sending messages. Sending and Receiving Messages Programmatically To send messages, craft a payload. For example, a simple text message requires: { "to": "customer-phone-number", "type": "text", "text": { "body": "Hello! How can we assist you today?" } } Use axios to send this data. Also, set up webhooks to get inbound messages and delivery updates automatically—so your system stays in sync. Managing User Sessions and Data Track conversations to know where each customer is in your flow. Store user info and chat states in a database like MongoDB. This helps personalize messages and manage multiple interactions simultaneously. Building Core Automation Features Creating Message Templates and Manual Triggers Design message templates that fit WhatsApp guidelines. These are pre-approved messages used for automation. Based on user actions, trigger these templates automatically. For example, send a promo code when a user clicks a link. Implementing Workflow Automation Develop flows such as onboarding sequences or support chats. Set up rules so follow-up messages go out at specific times or after certain triggers. Use timers or user responses to guide conversations. Integrating with External Systems Tie your automation to customer data. Connect with your CRM or eCommerce platform to send personalized offers. Use APIs to fetch user info or purchase history before messaging. Handling User Opt-outs and Compliance Always ask users for permission before sending messages. Make it easy for them to opt out. Follow privacy laws like GDPR.
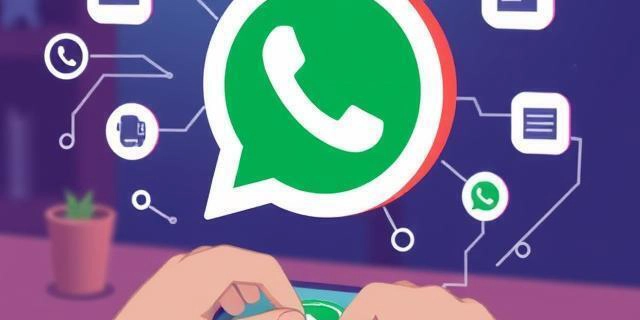
WhatsApp has become one of the most popular messaging apps worldwide. With over 2 billion active users, it’s now a key channel for marketing. Businesses see the value in sending personalized, timely messages straight to customers’ phones. Automating these messages saves time, boosts engagement, and can lead to more sales.
If you want to create your own Bulk WhatsApp marketing tool, using Node.js and the WhatsApp Business API is a smart move. This step-by-step guide walks you through building a custom automation system from scratch. Ready to get started? Let’s dive in.
Understanding WhatsApp Business API and Its Marketing Potential
Overview of WhatsApp Business API
The WhatsApp Business API is a powerful tool for businesses. Unlike the regular WhatsApp Business app, it’s designed for large-scale communication. It allows companies to send messages, receive responses, and automate conversations. The API supports templates, quick replies, and media sharing.
This API is especially useful for customer support, updates, and marketing campaigns. It lets you send personalized offers or reminders without manual effort. Imagine sending hundreds of follow-up messages automatically—it’s possible with this API.
Benefits of Using WhatsApp for Marketing
WhatsApp gets high open rates—up to 98%. That means most people see your message if you send it on the right platform. Engagement rates are also high because customers prefer messaging over email or calls.
Look at KLM Airlines: they use WhatsApp to confirm bookings and send updates. It’s easy for customers to reply, and airlines get quick feedback. But, remember, there are rules. You need user consent, and messages must follow WhatsApp’s policies.
Requirements for Integration
*To connect WhatsApp API to your system, you need a few things:
*
- Business verification on Facebook Business Manager
- Approval from Facebook or a WhatsApp API provider
- A server with Node.js installed
- An SSL certificate for secure communication Once you get API access, you can start building your custom tool.
Setting Up the Development Environment
Installing and Configuring Node.js
First, download Node.js from the official website. Follow the simple instructions for your operating system. After installation, open your terminal and run:
node -v
npm -v
This checks if Node.js and npm (Node package manager) are ready. For editing code, I recommend Visual Studio Code—it’s free and user-friendly.
Securing API Access
Register your business on Facebook Business Manager and apply for WhatsApp API access. Once approved, generate your API tokens. Keep these secrets safe—they give access to your account. Use environment variables to store credentials securely in your code.
Essential Libraries and Tools
For HTTP requests, use axios. It simplifies sending messages and fetching data. Also, set up a .env file to store secrets like API tokens safely. Optional tools include SDKs or wrappers that make API calls easier.
Connecting to the WhatsApp Business API with Node.js
Establishing a Secure Connection
Your app needs to communicate securely over HTTPS. Set the appropriate headers, including your API token, for each request. The API endpoints are usually like https://your-server/v1/messages for sending messages.
Sending and Receiving Messages Programmatically
To send messages, craft a payload. For example, a simple text message requires:
{
"to": "customer-phone-number",
"type": "text",
"text": { "body": "Hello! How can we assist you today?" }
}
Use axios to send this data. Also, set up webhooks to get inbound messages and delivery updates automatically—so your system stays in sync.
Managing User Sessions and Data
Track conversations to know where each customer is in your flow. Store user info and chat states in a database like MongoDB. This helps personalize messages and manage multiple interactions simultaneously.
Building Core Automation Features
Creating Message Templates and Manual Triggers
Design message templates that fit WhatsApp guidelines. These are pre-approved messages used for automation. Based on user actions, trigger these templates automatically. For example, send a promo code when a user clicks a link.
Implementing Workflow Automation
Develop flows such as onboarding sequences or support chats. Set up rules so follow-up messages go out at specific times or after certain triggers. Use timers or user responses to guide conversations.
Integrating with External Systems
Tie your automation to customer data. Connect with your CRM or eCommerce platform to send personalized offers. Use APIs to fetch user info or purchase history before messaging.
Handling User Opt-outs and Compliance
Always ask users for permission before sending messages. Make it easy for them to opt out. Follow privacy laws like GDPR. Keep your data secure and avoid spammy tactics.
Testing, Deployment, and Monitoring
Testing the Automation Tool
Use test numbers or sandbox environments to try your system. Check if messages send correctly and if webhooks work. Debug errors like failed deliveries or missing data.
Deploying for Production Use
Host your app on a trusted server, like AWS or Heroku. Secure your endpoints with SSL. Scale your system as your customer base grows, ensuring reliable delivery and fast responses.
Monitoring Performance and Engagement
Track open rates, reply rates, and opt-outs. Use analytics tools to understand what works. Adjust your campaigns based on real data to improve ROI.
Advanced Tips and Best Practices
Enhancing User Experience
Make your messages more personal. Use customer data to customize greetings or offers. Add images, videos, or buttons to make interactions richer and more engaging.
Scaling Automation Efforts
As your list grows, automate campaigns responsibly. Use queues or batch sending to avoid overload. Always keep user experience in mind.
Staying Up-to-Date with API Changes
Follow WhatsApp’s official channels for updates. New features and policies appear often. Keep your system current so you can use the latest tools.
Conclusion
Building a WhatsApp marketing automation tool with Node.js is a powerful way to connect with customers. It involves understanding the API, setting up a development environment, and creating workflows that serve your goals. Remember to follow compliance rules and prioritize user experience.
Start experimenting today. Integrate the WhatsApp Business API into your system and open a new channel of communication. With time and care, your automation can boost engagement and grow your business.