Copying objects in JavaScript. Shallow Copy vs Deep Copy
When working with objects and arrays in JavaScript, understanding the difference between shallow copy and deep copy is crucial to avoid unintended side effects. Let's break it down with explanations and examples. What is a Shallow Copy? A shallow copy creates a new object or array, but it only copies the references of nested objects rather than duplicating them. This means changes to nested objects in the copied structure will reflect in the original object. Example of a Shallow Copy: let original = { name: "Alice", details: { age: 25, city: "New York" } }; let shallowCopy = { ...original }; shallowCopy.details.city = "Los Angeles"; console.log(original.details.city); // Output: "Los Angeles" Why does this happen? The spread operator (...) creates a new top-level object, but the nested details object is still referenced, meaning modifications in shallowCopy.details affect original.details as well. Common Methods for Shallow Copy: Spread Operator (...) Object.assign() Array.slice() or Array.concat() for arrays What is a Deep Copy? A deep copy creates an entirely independent clone of the original object, including all nested structures. Modifications in the copied object will not affect the original. Example of a Deep Copy: let deepCopy = JSON.parse(JSON.stringify(original)); deepCopy.details.city = "Chicago"; console.log(original.details.city); // Output: "New York" console.log(deepCopy.details.city); // Output: "Chicago" Common Methods for Deep Copy: JSON.parse(JSON.stringify(obj)) - Simple and effective, but has the following limitations: Loses functions Converts dates to strings Doesn't handle Maps, Sets, RegExp, etc. Can't handle circular references (throws error) Lodash's cloneDeep()- A robust method that handles all data types. let deepCopy = _.cloneDeep(original); StructuredClone() (modern browsers) - A built-in method for deep cloning: let deepCopy = structuredClone(original); Performance: Shallow copies are generally more performant since they don't have to recursively copy nested structures. When to Use Which? Shallow Copy: When working with simple objects that don’t contain nested structures. Real-world scenarios: React Component State Updates: When updating React state that contains objects, shallow copies help maintain immutability principles Form Data Management: When you need to collect user input but preserve the original data API Data Transformation: When you need to slightly modify API response data without changing the original Redux Reducers: Redux requires immutable state updates, making shallow copies essential Configuration Object Overrides: When you need to merge default settings with user preferences Caching with Modification: When implementing simple caching systems Undo Functionality: Creating a simple undo history for UI interactions API responses: Making copies of data before manipulation In these examples, shallow copying provides the right balance between performance and functionality when you only need to modify top-level properties of objects. Deep Copy: When modifying an object independently without affecting the original, especially for nested objects or complex data structures. Real-world scenarios: Complex State Management: When working with nested state objects that need independent manipulation: javascriptCopyfunction editUserProfile(user) { // Deep clone to ensure complete independence from original const workingCopy = structuredClone(user); // Now we can modify deeply nested properties safely workingCopy.preferences.notifications.marketing.email = false; workingCopy.preferences.notifications.marketing.sms = false; // Original user object remains unchanged return workingCopy; } Saving Game State When implementing save/load functionality in browser games: javascriptCopyfunction saveGameState() { // Deep clone the current game state with all nested objects const savedState = structuredClone(gameState); // Add metadata savedState.savedAt = new Date().toISOString(); savedState.version = "1.2.0"; localStorage.setItem('savedGame', JSON.stringify(savedState)); } Multi-step Form Wizards When implementing forms with multiple steps and draft saving: javascriptCopyfunction FormWizard() { const [masterData, setMasterData] = useState(initialData); const [draftData, setDraftData] = useState({}); // Create completely independent draft to work with useEffect(() => { setDraftData(structuredClone(masterData)); }, [masterData]); const saveDraft = () => { // Save draft without committing localStorage.setItem('formDraft', JSON.stringify(draftData)); }; const commitChanges = () => { // Only update master data when form is submitted setMasterData(structuredClone(draftData)); }; } Undo/Redo Functionality When implementing robust undo/redo features: javascriptCopyclass DocumentEditor { constructor(initial
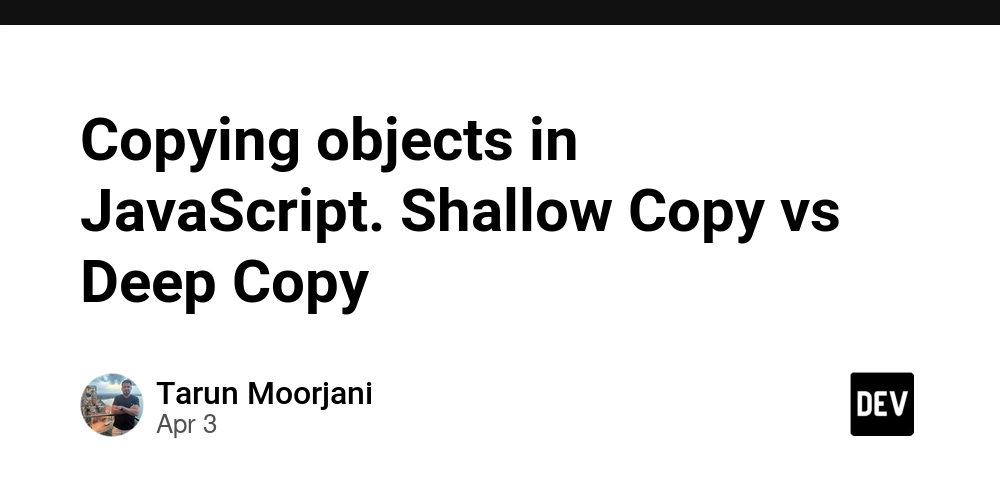
When working with objects and arrays in JavaScript, understanding the difference between shallow copy and deep copy is crucial to avoid unintended side effects. Let's break it down with explanations and examples.
What is a Shallow Copy?
A shallow copy creates a new object or array, but it only copies the references of nested objects rather than duplicating them. This means changes to nested objects in the copied structure will reflect in the original object.
Example of a Shallow Copy:
let original = {
name: "Alice",
details: {
age: 25,
city: "New York"
}
};
let shallowCopy = { ...original };
shallowCopy.details.city = "Los Angeles";
console.log(original.details.city); // Output: "Los Angeles"
Why does this happen?
The spread operator (...) creates a new top-level object, but the nested details object is still referenced, meaning modifications in shallowCopy.details affect original.details as well.
Common Methods for Shallow Copy:
Spread Operator (...)
Object.assign()
Array.slice() or Array.concat() for arrays
What is a Deep Copy?
A deep copy creates an entirely independent clone of the original object, including all nested structures. Modifications in the copied object will not affect the original.
Example of a Deep Copy:
let deepCopy = JSON.parse(JSON.stringify(original));
deepCopy.details.city = "Chicago";
console.log(original.details.city); // Output: "New York"
console.log(deepCopy.details.city); // Output: "Chicago"
Common Methods for Deep Copy:
JSON.parse(JSON.stringify(obj)) - Simple and effective, but has the following limitations:
- Loses functions
- Converts dates to strings
- Doesn't handle Maps, Sets, RegExp, etc.
- Can't handle circular references (throws error)
Lodash's cloneDeep()- A robust method that handles all data types.
let deepCopy = _.cloneDeep(original);
StructuredClone() (modern browsers) - A built-in method for deep cloning:
let deepCopy = structuredClone(original);
Performance:
Shallow copies are generally more performant since they don't have to recursively copy nested structures.
When to Use Which?
Shallow Copy: When working with simple objects that don’t contain nested structures.
Real-world scenarios:
- React Component State Updates: When updating React state that contains objects, shallow copies help maintain immutability principles
- Form Data Management: When you need to collect user input but preserve the original data
- API Data Transformation: When you need to slightly modify API response data without changing the original
- Redux Reducers: Redux requires immutable state updates, making shallow copies essential
- Configuration Object Overrides: When you need to merge default settings with user preferences
- Caching with Modification: When implementing simple caching systems
- Undo Functionality: Creating a simple undo history for UI interactions
- API responses: Making copies of data before manipulation
In these examples, shallow copying provides the right balance between performance and functionality when you only need to modify top-level properties of objects.
Deep Copy: When modifying an object independently without affecting the original, especially for nested objects or complex data structures.
Real-world scenarios:
- Complex State Management: When working with nested state objects that need independent manipulation:
javascriptCopyfunction editUserProfile(user) {
// Deep clone to ensure complete independence from original
const workingCopy = structuredClone(user);
// Now we can modify deeply nested properties safely
workingCopy.preferences.notifications.marketing.email = false;
workingCopy.preferences.notifications.marketing.sms = false;
// Original user object remains unchanged
return workingCopy;
}
- Saving Game State When implementing save/load functionality in browser games:
javascriptCopyfunction saveGameState() {
// Deep clone the current game state with all nested objects
const savedState = structuredClone(gameState);
// Add metadata
savedState.savedAt = new Date().toISOString();
savedState.version = "1.2.0";
localStorage.setItem('savedGame', JSON.stringify(savedState));
}
- Multi-step Form Wizards When implementing forms with multiple steps and draft saving:
javascriptCopyfunction FormWizard() {
const [masterData, setMasterData] = useState(initialData);
const [draftData, setDraftData] = useState({});
// Create completely independent draft to work with
useEffect(() => {
setDraftData(structuredClone(masterData));
}, [masterData]);
const saveDraft = () => {
// Save draft without committing
localStorage.setItem('formDraft', JSON.stringify(draftData));
};
const commitChanges = () => {
// Only update master data when form is submitted
setMasterData(structuredClone(draftData));
};
}
- Undo/Redo Functionality When implementing robust undo/redo features:
javascriptCopyclass DocumentEditor {
constructor(initialDoc) {
this.history = [structuredClone(initialDoc)];
this.currentIndex = 0;
}
makeChange(updateFn) {
// Create completely new document state
const newDoc = structuredClone(this.history[this.currentIndex]);
updateFn(newDoc);
// Add to history stack
this.history = this.history.slice(0, this.currentIndex + 1);
this.history.push(newDoc);
this.currentIndex++;
}
undo() {
if (this.currentIndex > 0) {
this.currentIndex--;
return structuredClone(this.history[this.currentIndex]);
}
}
redo() {
if (this.currentIndex < this.history.length - 1) {
this.currentIndex++;
return structuredClone(this.history[this.currentIndex]);
}
}
}
- Data Manipulation Before Visualization When preparing complex data for charts or visualizations:
javascriptCopyfunction prepareDataForVisualization(rawData) {
// Start with complete copy to preserve original data
const processedData = structuredClone(rawData);
// Transform deeply nested structures
processedData.datasets.forEach(dataset => {
dataset.points = dataset.points.map(point => ({
...point,
size: calculatePointSize(point.value),
color: determineColor(point.category, point.value)
}));
dataset.metadata.lastProcessed = new Date();
});
return processedData;
}
- Test Fixtures When setting up test cases with complex data:
javascriptCopyfunction createTestFixture() {
const baseFixture = {
user: {
id: "test-123",
profile: {
preferences: { /* complex nested settings */ }
},
cart: [
{ product: { /* product data */ }, quantity: 2 }
]
}
};
return {
getFixtureForTest(customizations = {}) {
// Return fresh copy each time to avoid test interference
const fixture = structuredClone(baseFixture);
// Apply any test-specific customizations
deepMerge(fixture, customizations);
return fixture;
}
};
}
- Configuration Management Systems When managing complex application configurations:
javascriptCopyclass ConfigManager {
constructor(defaultConfig) {
this.defaultConfig = defaultConfig;
this.currentConfig = structuredClone(defaultConfig);
}
saveUserConfig(userConfig) {
// Deep merge user settings with defaults
this.currentConfig = this.mergeConfigs(
structuredClone(this.defaultConfig),
userConfig
);
}
resetToDefaults() {
// Create fresh copy of defaults
this.currentConfig = structuredClone(this.defaultConfig);
}
}
Deep cloning is critical in these scenarios because it ensures complete data independence, preventing unintended side effects when modifying complex nested data structures.
Conclusion
Understanding the difference between shallow and deep copies in JavaScript is essential to prevent unintended mutations in your data structures. Depending on your use case, choosing the right method can optimize performance and ensure data integrity.