Comparison Between Fetch and Axios: Usage, Differences, and Real-World Applications
In modern web development, fetching data from APIs is an essential task. Two widely used methods for making HTTP requests in JavaScript and TypeScript are fetch and axios. While both serve the same fundamental purpose, they differ in functionality, ease of use, and performance. This article explores their key differences, usage, and practical applications to help developers choose the right tool based on their requirements. Key Differences Feature fetch axios Default Behaviour Supports only GET requests by default; additional configuration is required for POST, PUT, and DELETE requests. Supports GET, POST, PUT, DELETE requests without extra configuration. Response Handling Requires explicit conversion to JSON using .json(). Automatically converts responses to JSON. Error Handling Only fails on network errors; HTTP errors (e.g., 404, 500) must be handled manually. Automatically detects and handles HTTP errors. Configuration Headers and request body must be manually configured. Provides default configurations with extensive customisation options. Request Cancellation No built-in cancellation support; requires AbortController. Supports request cancellation with built-in cancelToken. Timeout Handling No built-in timeout support. Allows setting request timeouts. Browser Support Supported by most modern browsers. Similar browser support, but better compatibility with older versions. Real-World Applications When to Use fetch Lightweight applications where minimal overhead is required. Simple GET requests without additional configurations. Modern browser-based applications that do not require extensive request handling. When to Use axios Applications requiring advanced error handling and automatic JSON parsing. Complex API interactions involving request cancellations and timeouts. Server-side rendering (SSR) and Node.js environments where browser-native fetch is not available. Practical Examples in TypeScript 1. Fetch API Implementation async function getDataWithFetch(url: string): Promise { try { const response = await fetch(url); if (!response.ok) { throw new Error(`HTTP error! Status: ${response.status}`); } const data = await response.json(); console.log("Fetched Data:", data); } catch (error) { console.error("Fetch Error:", error); } } // API Call getDataWithFetch("https://jsonplaceholder.typicode.com/posts/1"); Explanation: The fetch function is used to make an HTTP request. The .json() method is required to parse the response into JSON format. HTTP status codes are manually checked to determine if an error has occurred. 2. Axios API Implementation import axios from 'axios'; async function getDataWithAxios(url: string): Promise { try { const response = await axios.get(url); console.log("Axios Data:", response.data); } catch (error) { console.error("Axios Error:", error); } } // API Call getDataWithAxios("https://jsonplaceholder.typicode.com/posts/1"); Explanation: axios automatically handles JSON parsing, eliminating the need for .json(). It provides built-in error handling for HTTP status codes. The code is more concise and easier to maintain. In summary, fetch is a lightweight and browser-native option suitable for simple API requests, whereas axios provides a more feature-rich solution with automatic error handling, response parsing, and request cancellation capabilities. Selecting between them depends on the complexity of the application and the developer's specific requirements. Both tools have their advantages, and understanding their differences ensures the most efficient choice for web development projects.
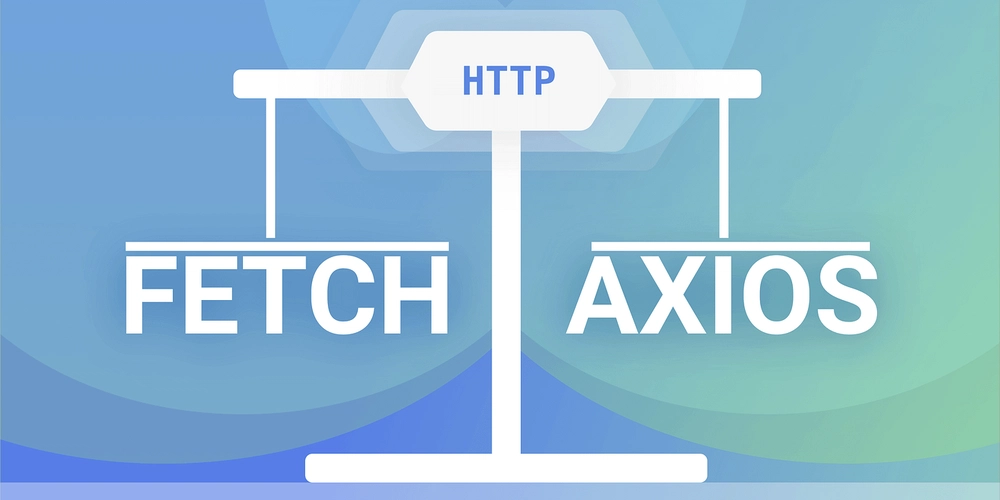
In modern web development, fetching data from APIs is an essential task. Two widely used methods for making HTTP requests in JavaScript and TypeScript are fetch
and axios
. While both serve the same fundamental purpose, they differ in functionality, ease of use, and performance. This article explores their key differences, usage, and practical applications to help developers choose the right tool based on their requirements.
Key Differences
Feature | fetch |
axios |
---|---|---|
Default Behaviour | Supports only GET requests by default; additional configuration is required for POST , PUT , and DELETE requests. |
Supports GET , POST , PUT , DELETE requests without extra configuration. |
Response Handling | Requires explicit conversion to JSON using .json() . |
Automatically converts responses to JSON. |
Error Handling | Only fails on network errors; HTTP errors (e.g., 404, 500) must be handled manually. | Automatically detects and handles HTTP errors. |
Configuration | Headers and request body must be manually configured. | Provides default configurations with extensive customisation options. |
Request Cancellation | No built-in cancellation support; requires AbortController . |
Supports request cancellation with built-in cancelToken . |
Timeout Handling | No built-in timeout support. | Allows setting request timeouts. |
Browser Support | Supported by most modern browsers. | Similar browser support, but better compatibility with older versions. |
Real-World Applications
When to Use fetch
- Lightweight applications where minimal overhead is required.
- Simple
GET
requests without additional configurations. - Modern browser-based applications that do not require extensive request handling.
When to Use axios
- Applications requiring advanced error handling and automatic JSON parsing.
- Complex API interactions involving request cancellations and timeouts.
- Server-side rendering (SSR) and Node.js environments where browser-native
fetch
is not available.
Practical Examples in TypeScript
1. Fetch API Implementation
async function getDataWithFetch(url: string): Promise<void> {
try {
const response = await fetch(url);
if (!response.ok) {
throw new Error(`HTTP error! Status: ${response.status}`);
}
const data = await response.json();
console.log("Fetched Data:", data);
} catch (error) {
console.error("Fetch Error:", error);
}
}
// API Call
getDataWithFetch("https://jsonplaceholder.typicode.com/posts/1");
Explanation:
- The
fetch
function is used to make an HTTP request. - The
.json()
method is required to parse the response into JSON format. - HTTP status codes are manually checked to determine if an error has occurred.
2. Axios API Implementation
import axios from 'axios';
async function getDataWithAxios(url: string): Promise<void> {
try {
const response = await axios.get(url);
console.log("Axios Data:", response.data);
} catch (error) {
console.error("Axios Error:", error);
}
}
// API Call
getDataWithAxios("https://jsonplaceholder.typicode.com/posts/1");
Explanation:
-
axios
automatically handles JSON parsing, eliminating the need for.json()
. - It provides built-in error handling for HTTP status codes.
- The code is more concise and easier to maintain.
In summary, fetch
is a lightweight and browser-native option suitable for simple API requests, whereas axios
provides a more feature-rich solution with automatic error handling, response parsing, and request cancellation capabilities. Selecting between them depends on the complexity of the application and the developer's specific requirements.
Both tools have their advantages, and understanding their differences ensures the most efficient choice for web development projects.