Deploying a Node.js Application using Docker on AWS EC2 & Kubernetes (EKS)
Deploying a Node.js application using Docker, Kubernetes, and AWS is a multi-step process that requires building the Docker image, configuring Kubernetes for deployment, and setting up AWS services. Below is an process and commands involved I'll walk through the steps of deploying a simple Node.js application using Docker, Kubernetes, and AWS. Step 1: Set Up Node.js Application First, create a simple Node.js application. For this example, we'll use a basic Express server. Create a server.js file: // server.js const express = require('express'); const app = express(); const port = 3000; app.get('/', (req, res) => { res.send('First Service from Node.js!'); }); app.listen(port, () => { console.log(`Server is running on port ${port}`); }); Step 2: Dockerize the Node.js Application Create a Dockerfile: The Dockerfile defines the environment to run your application. # Step 1: Use the official Node.js image FROM node:14 # Step 2: Set the working directory inside the container WORKDIR /usr/src/app # Step 3: Copy the application code into the container COPY . . # Step 4: Install dependencies RUN npm install # Step 5: Expose the application port EXPOSE 3000 # Step 6: Run the application CMD ["npm", "start"] Build the Docker image using below command: docker build -t node-docker-app . docker run -p 3000:3000 node-docker-app Now we can check our application should now be accessible at http://localhost:3000. Step 3: Push Docker Image to AWS ECR (Elastic Container Registry) First, create a repository in ECR on AWS Console: Go to the ECR section of AWS. Create a new repository, e.g., node-docker-app. Then, authenticate Docker with AWS ECR: aws ecr get-login-password --region us-west-2 | docker login --username AWS --password-stdin .dkr.ecr.us-west-2.amazonaws.com Reference Link: link:https://docs.aws.amazon.com/AmazonECR/latest/userguide/docker-push-ecr-image.html Tag the Docker image: docker tag node-docker-app:latest .dkr.ecr.us-west-2.amazonaws.com/node-docker-app:latest Push the Docker image to ECR: docker push .dkr.ecr.us-west-2.amazonaws.com/node-docker-app:latest Step 4: Create Kubernetes Deployment Configuration Create a Kubernetes deployment YAML file to manage your Dockerized app. Create a deployment.yaml file: apiVersion: apps/v1 kind: Deployment metadata: name: node-docker-app-deployment spec: replicas: 2 selector: matchLabels: app: node-docker-app template: metadata: labels: app: node-docker-app spec: containers: - name: node-docker-app image: .dkr.ecr.us-west-2.amazonaws.com/node-docker-app:latest ports: - containerPort: 3000 Create a Kubernetes service YAML file: This exposes your application to the outside world. Create a service.yaml file: apiVersion: v1 kind: Service metadata: name: node-docker-app-service spec: selector: app: node-docker-app ports: - protocol: TCP port: 80 targetPort: 3000 type: LoadBalancer Step 5: Set Up Kubernetes on AWS (EKS) Install kubectl and Install eksctl (for creating an EKS cluster) brew install kubectl brew install eksctl Create an EKS cluster: eksctl create cluster --name nodejs-cluster --region us-west-2 --nodegroup-name nodegroup --node-type t2.micro --nodes 2 Configure kubectl to use your EKS cluster: aws eks --region us-west-2 update-kubeconfig --name nodejs-cluster Step 6: Deploy the Application to Kubernetes Apply the deployment and service files: kubectl apply -f deployment.yaml kubectl apply -f service.yaml Check the status of the deployment: kubectl get deployments kubectl get pods Get the LoadBalancer IP: kubectl get svc node-docker-app-service The external IP address will be listed under the EXTERNAL-IP column. You can now access the application via the LoadBalancer's IP. Step 7: Clean Up When you're done, you can delete the Kubernetes cluster on AWS to avoid any ongoing charges: `eksctl delete cluster --name nodejs-cluster --region us-west-2
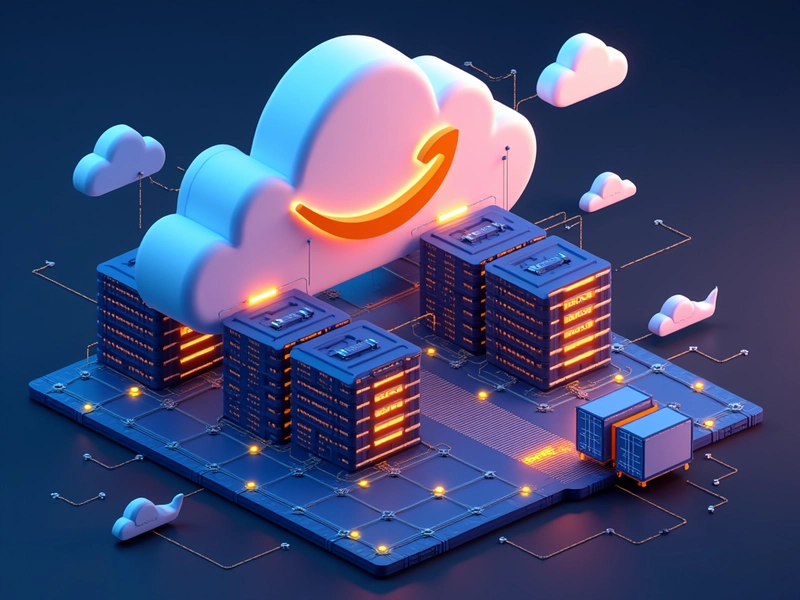
Deploying a Node.js application using Docker, Kubernetes, and AWS is a multi-step process that requires building the Docker image, configuring Kubernetes for deployment, and setting up AWS services. Below is an process and commands involved
I'll walk through the steps of deploying a simple Node.js application using Docker, Kubernetes, and AWS.
Step 1: Set Up Node.js Application
First, create a simple Node.js application. For this example, we'll use a basic Express server.
Create a server.js file:
// server.js
const express = require('express');
const app = express();
const port = 3000;
app.get('/', (req, res) => {
res.send('First Service from Node.js!');
});
app.listen(port, () => {
console.log(`Server is running on port ${port}`);
});
Step 2: Dockerize the Node.js Application
Create a Dockerfile:
The Dockerfile defines the environment to run your application.
# Step 1: Use the official Node.js image
FROM node:14
# Step 2: Set the working directory inside the container
WORKDIR /usr/src/app
# Step 3: Copy the application code into the container
COPY . .
# Step 4: Install dependencies
RUN npm install
# Step 5: Expose the application port
EXPOSE 3000
# Step 6: Run the application
CMD ["npm", "start"]
Build the Docker image using below command:
docker build -t node-docker-app .
docker run -p 3000:3000 node-docker-app
Now we can check
our application should now be accessible at http://localhost:3000.
Step 3: Push Docker Image to AWS ECR (Elastic Container Registry)
First, create a repository in ECR on AWS Console:
Go to the ECR section of AWS.
Create a new repository, e.g., node-docker-app.
Then, authenticate Docker with AWS ECR:
aws ecr get-login-password --region us-west-2 | docker login --username AWS --password-stdin
Reference Link: link:https://docs.aws.amazon.com/AmazonECR/latest/userguide/docker-push-ecr-image.html
Tag the Docker image:
docker tag node-docker-app:latest
Push the Docker image to ECR:
docker push
Step 4: Create Kubernetes Deployment Configuration
Create a Kubernetes deployment YAML file to manage your Dockerized app.
Create a deployment.yaml file:
apiVersion: apps/v1
kind: Deployment
metadata:
name: node-docker-app-deployment
spec:
replicas: 2
selector:
matchLabels:
app: node-docker-app
template:
metadata:
labels:
app: node-docker-app
spec:
containers:
- name: node-docker-app
image: .dkr.ecr.us-west-2.amazonaws.com/node-docker-app:latest
ports:
- containerPort: 3000
Create a Kubernetes service YAML file:
This exposes your application to the outside world.
Create a service.yaml file:
apiVersion: v1
kind: Service
metadata:
name: node-docker-app-service
spec:
selector:
app: node-docker-app
ports:
- protocol: TCP
port: 80
targetPort: 3000
type: LoadBalancer
Step 5: Set Up Kubernetes on AWS (EKS)
Install kubectl and Install eksctl (for creating an EKS cluster)
brew install kubectl
brew install eksctl
Create an EKS cluster:
eksctl create cluster --name nodejs-cluster --region us-west-2 --nodegroup-name nodegroup --node-type t2.micro --nodes 2
Configure kubectl to use your EKS cluster:
aws eks --region us-west-2 update-kubeconfig --name nodejs-cluster
Step 6: Deploy the Application to Kubernetes
Apply the deployment and service files:
kubectl apply -f deployment.yaml
kubectl apply -f service.yaml
Check the status of the deployment:
kubectl get deployments
kubectl get pods
Get the LoadBalancer IP:
kubectl get svc node-docker-app-service
The external IP address will be listed under the EXTERNAL-IP column. You can now access the application via the LoadBalancer's IP.
Step 7: Clean Up
When you're done, you can delete the Kubernetes cluster on AWS to avoid any ongoing charges:
`eksctl delete cluster --name nodejs-cluster --region us-west-2