“Async vs. Sync: The Dysfunctional Love Triangle in Your Codebase”
There are only two types of devs in the world: Those who misuse async/await like it’s going out of style. And liars. Welcome to the eternal battle between synchronous and asynchronous code—where your choices define whether you deliver a performant app or a sentient, bug-producing AI that crashes more than a Mac with 2GB of RAM. Let’s break it down, with all the nuance of a DevOps guy watching you scp a file to production. ⸻ Synchronous Code: The Reliable But Clingy Ex Sync code is like that person who texts “where r u” every 5 minutes. It waits for your full attention, won’t move until you answer, and god help you if it gets stuck—everything else stops. Great for control freaks. Easy to debug—stack traces actually mean something. But as slow as a junior dev copy-pasting code from Stack Overflow with zero understanding. Synchronous is like standing in line at the DMV: nothing else happens until you finish your turn. It works, but dear lord is it painful under pressure. ⸻ Asynchronous Code: The Cool Kid Who Sets Everything on Fire Async is fast, loose, and has commitment issues. It’s the rockstar that kicks off 12 background tasks, disappears for a beer, and shows back up with errors you didn’t even know were possible. Perfect for APIs, I/O, file ops. Super performant when not handled like a wet sock. Also perfect for birthing race conditions, memory leaks, and broken dreams. Every junior dev’s journey: “Wow async is cool” → “Why is my app crashing?” → “WTF is the event loop?” → “I hate everything.” ⸻ Top 5 Ways to Ruin Your Day with Async 1 awaiting nothing You’ve seen it. Someone slaps await on a non-Promise like it’s seasoning. await console.log("hi"); This is like putting on a seatbelt to use a blender. Stop it. 2 Not awaiting actual Promises You forgot to await. Now everything runs, nothing finishes, and your server crashes faster than a crypto coin in 2022. 3 Using forEach with async This one’s a classic. Nothing says “I don’t understand JavaScript” like this: array.forEach(async (item) => { await doSomething(item); }); Enjoy your unordered chaos, king. Your loop didn’t await anything, but your error logs sure will. 4 Catching errors like you catch sleep on release week: not at all Async errors are like silent farts. You don’t know they happened until everything smells like regret. 5 Using async where sync would work just fine “Hey let’s make this utility function async because… vibes.” Yeah, and let’s replace your if statements with AI-generated haikus while we’re at it. ⸻ Sync Ain’t Dead, It’s Just Misunderstood Sometimes you don’t need concurrency. Sometimes, the right move is to do things one at a time like a responsible adult. If your operation takes 2ms, introducing async overhead is like hiring a team of consultants to change a lightbulb. ⸻ The TL;DR That Saves Careers Use sync when you care about order, simplicity, and not setting your app on fire. Use async when you’re juggling I/O, need performance, and are emotionally ready for stack traces that make no sense. Don’t sprinkle async/await everywhere like it’s garlic powder. You still need to know what the hell you’re cooking. ⸻ Final Thought: If your async code is harder to read than your company’s healthcare policy, you’ve done something wrong. Write code like the next poor soul reading it is a sleep-deprived junior dev with anxiety and a looming deadline. Because they probably are. And sometimes… that dev is you in 3 months. ⸻ Follow me if you’ve ever rage-quit over a Promise chain and cried into your console.log. Or if you just like your tech advice with a side of sarcasm:
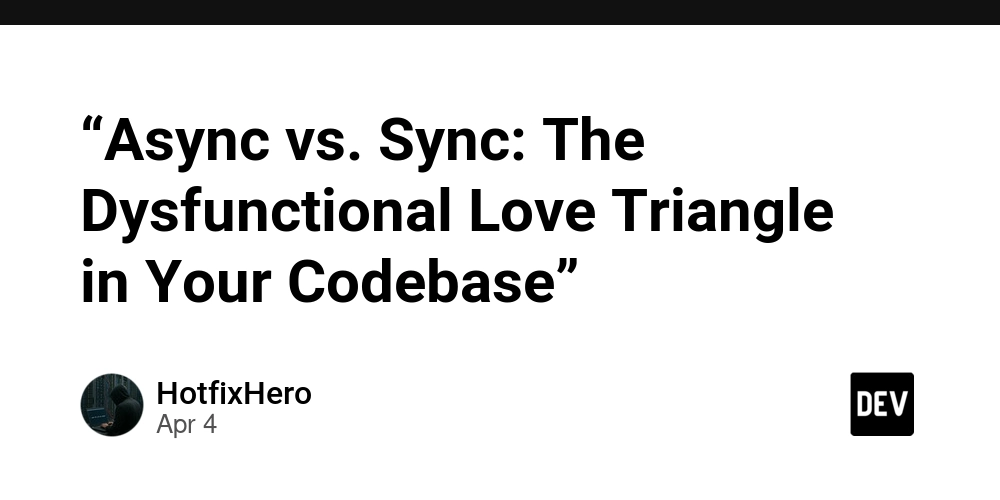
There are only two types of devs in the world:
- Those who misuse async/await like it’s going out of style.
- And liars.
Welcome to the eternal battle between synchronous and asynchronous code—where your choices define whether you deliver a performant app or a sentient, bug-producing AI that crashes more than a Mac with 2GB of RAM.
Let’s break it down, with all the nuance of a DevOps guy watching you scp a file to production.
⸻
Synchronous Code: The Reliable But Clingy Ex
Sync code is like that person who texts “where r u” every 5 minutes. It waits for your full attention, won’t move until you answer, and god help you if it gets stuck—everything else stops.
- Great for control freaks.
- Easy to debug—stack traces actually mean something.
- But as slow as a junior dev copy-pasting code from Stack Overflow with zero understanding.
Synchronous is like standing in line at the DMV: nothing else happens until you finish your turn. It works, but dear lord is it painful under pressure.
⸻
Asynchronous Code: The Cool Kid Who Sets Everything on Fire
Async is fast, loose, and has commitment issues.
It’s the rockstar that kicks off 12 background tasks, disappears for a beer, and shows back up with errors you didn’t even know were possible.
- Perfect for APIs, I/O, file ops.
- Super performant when not handled like a wet sock.
- Also perfect for birthing race conditions, memory leaks, and broken dreams.
Every junior dev’s journey:
“Wow async is cool” → “Why is my app crashing?” → “WTF is the event loop?” → “I hate everything.”
⸻
Top 5 Ways to Ruin Your Day with Async
1 awaiting nothing
You’ve seen it. Someone slaps await on a non-Promise like it’s seasoning.
await console.log("hi");
This is like putting on a seatbelt to use a blender. Stop it.
2 Not awaiting actual Promises
You forgot to await. Now everything runs, nothing finishes, and your server crashes faster than a crypto coin in 2022.
3 Using forEach with async
This one’s a classic. Nothing says “I don’t understand JavaScript” like this:
array.forEach(async (item) => {
await doSomething(item);
});
Enjoy your unordered chaos, king. Your loop didn’t await anything, but your error logs sure will.
4 Catching errors like you catch sleep on release week: not at all
Async errors are like silent farts. You don’t know they happened until everything smells like regret.
5 Using async where sync would work just fine
“Hey let’s make this utility function async because… vibes.”
Yeah, and let’s replace your if statements with AI-generated haikus while we’re at it.
⸻
Sync Ain’t Dead, It’s Just Misunderstood
Sometimes you don’t need concurrency. Sometimes, the right move is to do things one at a time like a responsible adult.
If your operation takes 2ms, introducing async overhead is like hiring a team of consultants to change a lightbulb.
⸻
The TL;DR That Saves Careers
- Use sync when you care about order, simplicity, and not setting your app on fire.
- Use async when you’re juggling I/O, need performance, and are emotionally ready for stack traces that make no sense.
- Don’t sprinkle async/await everywhere like it’s garlic powder. You still need to know what the hell you’re cooking.
⸻
Final Thought:
If your async code is harder to read than your company’s healthcare policy, you’ve done something wrong.
Write code like the next poor soul reading it is a sleep-deprived junior dev with anxiety and a looming deadline. Because they probably are. And sometimes… that dev is you in 3 months.
⸻
Follow me if you’ve ever rage-quit over a Promise chain and cried into your console.log. Or if you just like your tech advice with a side of sarcasm: