Assertion Testing: Techniques for Ensuring Software Reliability
Assertions play a crucial role in software testing, helping developers verify that their code behaves as expected. By using assertion techniques effectively, teams can detect bugs early, improve test accuracy, and build more robust applications. Explore assertion testing techniques. What is Assertion Testing? Assertion testing is the process of verifying that a program's actual output matches the expected result. Assertions help validate conditions in unit tests, integration tests, and functional tests. Learn more about test planning. Why Use Assertions in Testing? ✅ Detects Bugs Early – Catches incorrect behavior before production. ✅ Improves Test Accuracy – Ensures expected results in automated tests. ✅ Enhances Code Stability – Helps prevent unintended changes in the codebase. ✅ Supports Continuous Integration – Automates testing in DevOps pipelines. Discover the role of assertions in unit testing. Types of Assertions 1. Boolean Assertions Verifies whether a condition is True or False. assert True # Passes assert False # Fails 2. Equality Assertions Ensures expected values match actual values. assert 5 == 5 # Passes assert "Hello" == "World" # Fails 3. Exception Assertions Confirms that a function raises the correct exception. import pytest def divide(x, y): return x / y with pytest.raises(ZeroDivisionError): divide(1, 0) 4. API Response Assertions Checks if an API request returns the expected response. import requests response = requests.get("https://api.example.com/data") assert response.status_code == 200 Explore API testing automation. Best Practices for Assertion Testing ✅ Use Descriptive Error Messages – Make failures easier to debug. ✅ Keep Assertions Simple – Avoid complex logic inside tests. ✅ Mock External Dependencies – Isolate units of code using tools like Keploy. ✅ Automate Assertions – Integrate tests into CI/CD pipelines. ✅ Test Edge Cases – Ensure the system handles unexpected inputs correctly. Learn how Keploy automates test case generation. Top Tools for Assertion Testing Keploy – AI-powered test case generation and API mocking. See how Keploy enhances assertion testing. PyTest – Powerful assertion framework for Python. JUnit – Java testing framework with built-in assertions. Mocha & Chai – JavaScript testing tools for assertions and validation. Conclusion Using assertions effectively ensures that your software remains reliable and bug-free. By incorporating assertion techniques in unit tests, API tests, and CI/CD workflows, teams can enhance test automation and debugging. Keploy further simplifies the process by generating intelligent test cases. Explore assertion testing techniques.
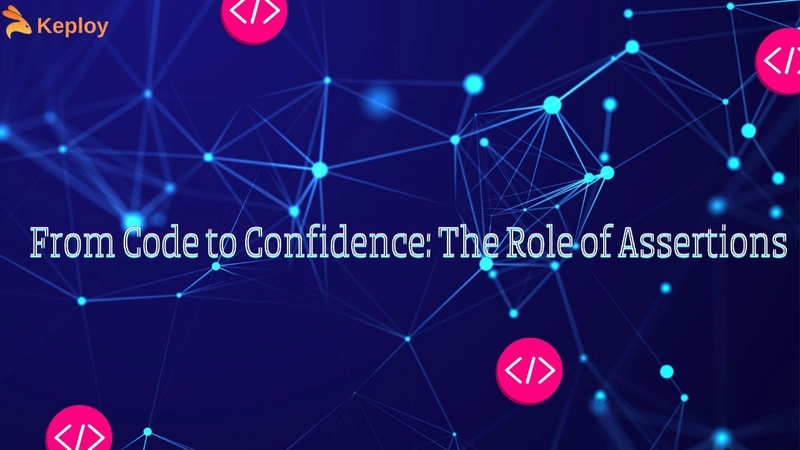
Assertions play a crucial role in software testing, helping developers verify that their code behaves as expected. By using assertion techniques effectively, teams can detect bugs early, improve test accuracy, and build more robust applications. Explore assertion testing techniques.
What is Assertion Testing?
Assertion testing is the process of verifying that a program's actual output matches the expected result. Assertions help validate conditions in unit tests, integration tests, and functional tests. Learn more about test planning.
Why Use Assertions in Testing?
✅ Detects Bugs Early – Catches incorrect behavior before production. ✅ Improves Test Accuracy – Ensures expected results in automated tests. ✅ Enhances Code Stability – Helps prevent unintended changes in the codebase. ✅ Supports Continuous Integration – Automates testing in DevOps pipelines.
Discover the role of assertions in unit testing.
Types of Assertions
1. Boolean Assertions
Verifies whether a condition is True
or False
.
assert True # Passes
assert False # Fails
2. Equality Assertions
Ensures expected values match actual values.
assert 5 == 5 # Passes
assert "Hello" == "World" # Fails
3. Exception Assertions
Confirms that a function raises the correct exception.
import pytest
def divide(x, y):
return x / y
with pytest.raises(ZeroDivisionError):
divide(1, 0)
4. API Response Assertions
Checks if an API request returns the expected response.
import requests
response = requests.get("https://api.example.com/data")
assert response.status_code == 200
Explore API testing automation.
Best Practices for Assertion Testing
✅ Use Descriptive Error Messages – Make failures easier to debug. ✅ Keep Assertions Simple – Avoid complex logic inside tests. ✅ Mock External Dependencies – Isolate units of code using tools like Keploy. ✅ Automate Assertions – Integrate tests into CI/CD pipelines. ✅ Test Edge Cases – Ensure the system handles unexpected inputs correctly.
Learn how Keploy automates test case generation.
Top Tools for Assertion Testing
Keploy – AI-powered test case generation and API mocking. See how Keploy enhances assertion testing.
PyTest – Powerful assertion framework for Python.
JUnit – Java testing framework with built-in assertions.
Mocha & Chai – JavaScript testing tools for assertions and validation.
Conclusion
Using assertions effectively ensures that your software remains reliable and bug-free. By incorporating assertion techniques in unit tests, API tests, and CI/CD workflows, teams can enhance test automation and debugging. Keploy further simplifies the process by generating intelligent test cases. Explore assertion testing techniques.