Add 1 to the list
Problem tc :O(n), sc:O(1) /* class Node{ int data; Node next; Node(int x){ data = x; next = null; } } */ class Solution { public Node addOne(Node head) { // code here. //reverse the list //add 1 ///reverse back again and return Node reverseNode = reverse(head); head = reverseNode; Node prev = null; int carry = 1; while(head!=null && carry!=0){ if(head.data + carry > 9){ int val = head.data + carry; head.data = (val) % 10; carry = (val)/10; } else { head.data = head.data+carry; carry =0; } prev = head; head = head.next; } if(carry!=0){ prev.next = new Node(carry); } return reverse(reverseNode); } public Node reverse(Node node){ Node prev = null; while(node!=null){ Node next = node.next; node.next = prev; prev = node; node = next; } return prev; } }
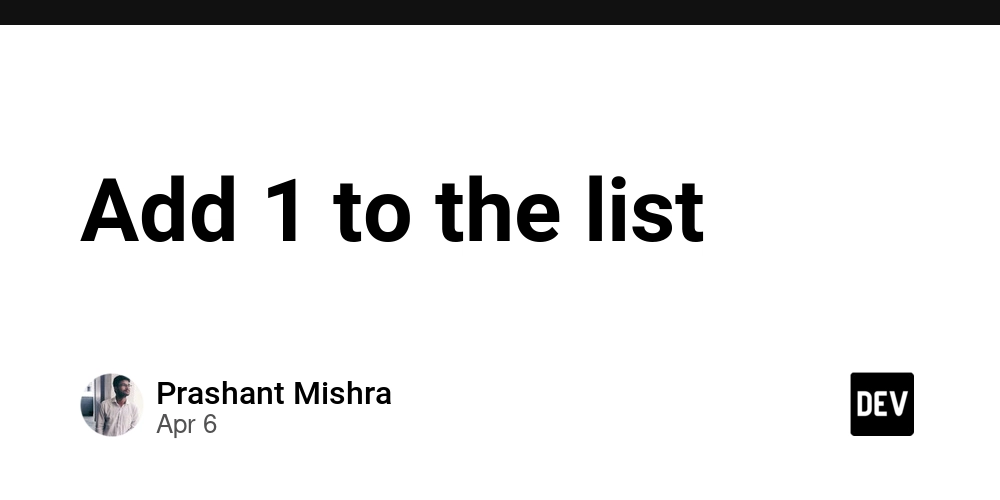
tc :O(n), sc:O(1)
/*
class Node{
int data;
Node next;
Node(int x){
data = x;
next = null;
}
}
*/
class Solution {
public Node addOne(Node head) {
// code here.
//reverse the list
//add 1
///reverse back again and return
Node reverseNode = reverse(head);
head = reverseNode;
Node prev = null;
int carry = 1;
while(head!=null && carry!=0){
if(head.data + carry > 9){
int val = head.data + carry;
head.data = (val) % 10;
carry = (val)/10;
}
else {
head.data = head.data+carry;
carry =0;
}
prev = head;
head = head.next;
}
if(carry!=0){
prev.next = new Node(carry);
}
return reverse(reverseNode);
}
public Node reverse(Node node){
Node prev = null;
while(node!=null){
Node next = node.next;
node.next = prev;
prev = node;
node = next;
}
return prev;
}
}