Why Should a Flask Developer Use MongoDB?
This post is written by Karen Zhang. Flask is one of the most popular Python web frameworks. Its lightweight and flexible nature makes it ideal for small to medium projects and rapid development cycles. With approximately three to 3.5 million users worldwide, Flask is a top choice for developers seeking simplicity without sacrificing functionality. On the other hand, MongoDB is a leading document database that has gained widespread adoption for its schema-less design, scalability, and flexibility. It provides an intuitive way to store and retrieve data, particularly for applications that require dynamic data structures and fast iteration. Flask: The need for a compatible database Flask is classified as a microframework, meaning it provides only the essential components needed to build a web application. One of the key features of Flask is its lack of a built-in database abstraction layer. While this might seem like a limitation, it actually gives developers the freedom to choose the database that best suits their project’s needs. When selecting a database for a Flask application, it’s important to consider one that aligns with Flask’s concept of simplicity and flexibility. This is where MongoDB shines. MongoDB’s schema-less design and ease of use make it an ideal companion for Flask, enabling developers to build scalable and dynamic applications without the constraints of a rigid schema. Why MongoDB is a great fit for Flask developers Schema-less design MongoDB's schema-less architecture is one of its most compelling features, especially for Flask developers who value development agility. Unlike traditional relational databases, which require developers to define strict table structures and relationships upfront, MongoDB allows data to be stored in flexible, JSON-like documents without requiring a predefined schema. This eliminates the need for complex migrations when data structures evolve, saving time and reducing friction during development. This flexibility aligns perfectly with Flask's philosophy of simplicity and adaptability. With MongoDB, developers can modify data models on the fly as application requirements change, without the overhead of writing and executing migration scripts. This is particularly useful during prototyping and early development stages, where requirements often shift as the project evolves. From developers’ experiences, MongoDB allows teams to avoid "planning out the database ahead of time or worrying about migration changes," making it an ideal choice for Flask applications that need to adapt quickly to changing needs. Below is a simple example to demonstrate MongoDB’s schema-less nature. In this example, we'll store user data in a MongoDB collection without defining a fixed schema upfront. Notice how we can add new fields to documents dynamically: from flask import Flask from flask_pymongo import PyMongo app = Flask(__name__) app.config["MONGO_URI"] = "mongodb+srv://:@cluster0.mongodb.net/my_database?retryWrites=true&w=majority" mongo = PyMongo(app) # Add a user with basic information user1 = { "name": "Alice", "email": "alice@example.com" } mongo.db.users.insert_one(user1) # Add another user with additional fields user2 = { "name": "Bob", "email": "bob@example.com", "age": 30, "address": { "city": "New York", "country": "USA" } } mongo.db.users.insert_one(user2) # Retrieve and print all users users = mongo.db.users.find() for user in users: print(user) And the output: {'_id': ObjectId('...'), 'name': 'Alice', 'email': 'alice@example.com'} {'_id': ObjectId('...'), 'name': 'Bob', 'email': 'bob@example.com', 'age': 30, 'address': {'city': 'New York', 'country': 'USA'}} JSON-like document structure MongoDB stores data in BSON (Binary JSON) format, which provides a natural fit for web applications that frequently work with JSON data. Flask applications typically handle JSON for API responses and requests. Python’s dictionary data structure closely mirrors JSON objects. When integrating MongoDB with Flask using libraries like PyMongo or Flask-PyMongo, we can store Python dictionaries directly in MongoDB collections and retrieve them without the need for complex transformations or additional mapping layers. This significantly reduces cognitive overhead, allowing us to focus on crafting application logic rather than navigating the data mapping complexities often encountered with relational databases. Below is a simple example to demonstrate how MongoDB’s JSON-like document structure integrates effortlessly with Flask and Python using flask_pymongo: from flask import Flask, jsonify from flask_pymongo import PyMongo app = Flask(__name__) app.config["MONGO_URI"] = "mongodb+srv://:@cluster0.mongodb.net/my_database?retryWrites=true&w=majority" mongo = PyMongo(app) # Store a JSON-like document in MongoDB product = { "name": "Wireless Mouse", "price": 29.
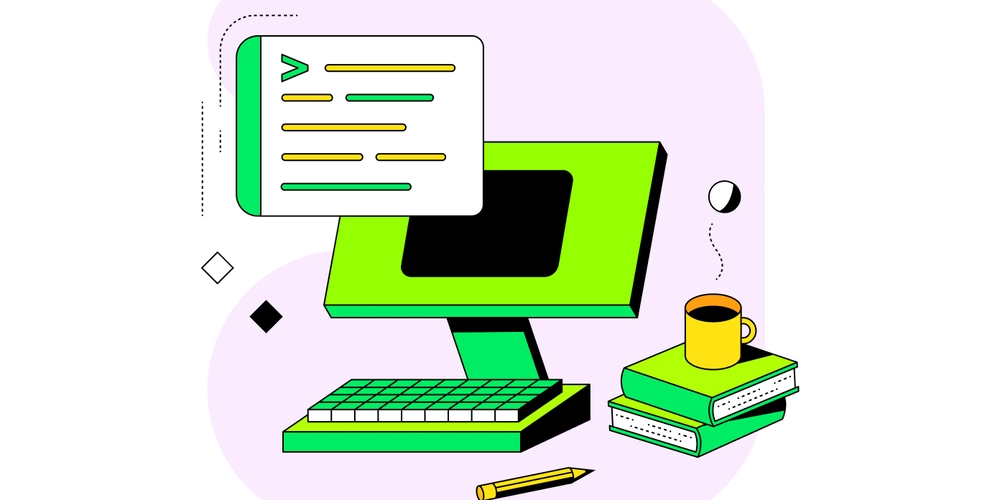
This post is written by Karen Zhang.
Flask is one of the most popular Python web frameworks. Its lightweight and flexible nature makes it ideal for small to medium projects and rapid development cycles. With approximately three to 3.5 million users worldwide, Flask is a top choice for developers seeking simplicity without sacrificing functionality.
On the other hand, MongoDB is a leading document database that has gained widespread adoption for its schema-less design, scalability, and flexibility. It provides an intuitive way to store and retrieve data, particularly for applications that require dynamic data structures and fast iteration.
Flask: The need for a compatible database
Flask is classified as a microframework, meaning it provides only the essential components needed to build a web application. One of the key features of Flask is its lack of a built-in database abstraction layer. While this might seem like a limitation, it actually gives developers the freedom to choose the database that best suits their project’s needs.
When selecting a database for a Flask application, it’s important to consider one that aligns with Flask’s concept of simplicity and flexibility. This is where MongoDB shines. MongoDB’s schema-less design and ease of use make it an ideal companion for Flask, enabling developers to build scalable and dynamic applications without the constraints of a rigid schema.
Why MongoDB is a great fit for Flask developers
Schema-less design
MongoDB's schema-less architecture is one of its most compelling features, especially for Flask developers who value development agility. Unlike traditional relational databases, which require developers to define strict table structures and relationships upfront, MongoDB allows data to be stored in flexible, JSON-like documents without requiring a predefined schema. This eliminates the need for complex migrations when data structures evolve, saving time and reducing friction during development.
This flexibility aligns perfectly with Flask's philosophy of simplicity and adaptability. With MongoDB, developers can modify data models on the fly as application requirements change, without the overhead of writing and executing migration scripts. This is particularly useful during prototyping and early development stages, where requirements often shift as the project evolves. From developers’ experiences, MongoDB allows teams to avoid "planning out the database ahead of time or worrying about migration changes," making it an ideal choice for Flask applications that need to adapt quickly to changing needs.
Below is a simple example to demonstrate MongoDB’s schema-less nature. In this example, we'll store user data in a MongoDB collection without defining a fixed schema upfront. Notice how we can add new fields to documents dynamically:
from flask import Flask
from flask_pymongo import PyMongo
app = Flask(__name__)
app.config["MONGO_URI"] = "mongodb+srv://:@cluster0.mongodb.net/my_database?retryWrites=true&w=majority "
mongo = PyMongo(app)
# Add a user with basic information
user1 = {
"name": "Alice",
"email": "alice@example.com"
}
mongo.db.users.insert_one(user1)
# Add another user with additional fields
user2 = {
"name": "Bob",
"email": "bob@example.com",
"age": 30,
"address": {
"city": "New York",
"country": "USA"
}
}
mongo.db.users.insert_one(user2)
# Retrieve and print all users
users = mongo.db.users.find()
for user in users:
print(user)
And the output:
{'_id': ObjectId('...'), 'name': 'Alice', 'email': 'alice@example.com'}
{'_id': ObjectId('...'), 'name': 'Bob', 'email': 'bob@example.com', 'age': 30, 'address': {'city': 'New York', 'country': 'USA'}}
JSON-like document structure
MongoDB stores data in BSON (Binary JSON) format, which provides a natural fit for web applications that frequently work with JSON data. Flask applications typically handle JSON for API responses and requests. Python’s dictionary data structure closely mirrors JSON objects. When integrating MongoDB with Flask using libraries like PyMongo or Flask-PyMongo, we can store Python dictionaries directly in MongoDB collections and retrieve them without the need for complex transformations or additional mapping layers. This significantly reduces cognitive overhead, allowing us to focus on crafting application logic rather than navigating the data mapping complexities often encountered with relational databases. Below is a simple example to demonstrate how MongoDB’s JSON-like document structure integrates effortlessly with Flask and Python using flask_pymongo:
from flask import Flask, jsonify
from flask_pymongo import PyMongo
app = Flask(__name__)
app.config["MONGO_URI"] = "mongodb+srv://:@cluster0.mongodb.net/my_database?retryWrites=true&w=majority"
mongo = PyMongo(app)
# Store a JSON-like document in MongoDB
product = {
"name": "Wireless Mouse",
"price": 29.99,
"category": "Electronics",
"tags": ["wireless", "ergonomic", "gadget"],
"in_stock": True
}
mongo.db.products.insert_one(product)
# Retrieve and return the document as a JSON response
@app.route('/product', methods=['GET'])
def get_product():
product = mongo.db.products.find_one({"name": "Wireless Mouse"})
return jsonify(product)
if __name__ == '__main__':
app.run(debug=True)
And the output looks like:
{
"_id": {"$oid": "..."},
"name": "Wireless Mouse",
"price": 29.99,
"category": "Electronics",
"tags": ["wireless", "ergonomic", "gadget"],
"in_stock": true
}
Built for modern applications (geospatial queries)
MongoDB is well-equipped to handle modern application requirements, including advanced geospatial queries. This makes it an excellent choice for Flask developers building location-based services or applications. MongoDB's native support for geospatial data allows efficient storage and querying of location-based information.
For example, you can use MongoDB to find all restaurants within a five-mile radius of a user's location or retrieve the closest delivery hubs to optimize logistics. Here's an example of using MongoDB's geospatial query with Flask:
@app.route('/nearby', methods=['GET'])
def find_nearby():
latitude = float(request.args.get('latitude'))
longitude = float(request.args.get('longitude'))
myclient = pymongo.MongoClient("mongodb://localhost:27017/")
mydb = myclient["myapp"]
mycol = mydb["locations"]
nearby_locations = mycol.find({
"location": {
"$near": {
"$geometry": {"type": "Point", "coordinates": [longitude, latitude]},
"$maxDistance": 5000 # Distance in meters
}
}
})
return jsonify(list(nearby_locations))
This capability is particularly valuable for applications such as ride-sharing platforms, delivery services, real estate search tools, and fitness tracking apps.
By leveraging MongoDB's features, Flask developers can build modern, location-aware applications with minimal effort and maximum performance, further enhancing the synergy between Flask and MongoDB for creating cutting-edge web applications.
Time series data
MongoDB’s native time series data support empowers Flask developers to build applications that efficiently handle chronological data streams. This feature is critical for IoT platforms, financial analytics tools, real-time monitoring systems, and other use cases requiring high-volume storage and analysis of time-stamped data. MongoDB’s time series collections are optimized for fast data ingestion and temporal querying, enabling developers to manage metrics, sensor readings, and event logs without compromising performance.
For instance, a Flask application tracking environmental sensors could use MongoDB to store temperature readings and calculate hourly averages. Learn more about the time series feature.
Code example: integrating MongoDB with Flask using libraries like PyMongo—advanced analytics with aggregation pipelines
MongoDB’s compatibility with Flask is reinforced by practical tools like Flask-PyMongo, a library designed to simplify database interactions between Flask and MondoDB. Flask-PyMongo eliminates boilerplate connection logic, allowing developers to interact with MongoDB collections using Python’s native dictionary syntax. Below, we will explore an example with code that demonstrates advanced analytics with an aggregation pipeline, with PyMongo. The following code calculates total sales by product category for the current month using MongoDB’s aggregation pipeline:
from flask import Flask, jsonify
from flask_pymongo import PyMongo
from datetime import datetime
app = Flask(__name__)
app.config["MONGO_URI"] = "mongodb+srv://:@cluster0.mongodb.net/my_database?retryWrites=true&w=majority"
mongo = PyMongo(app)
@app.route('/sales-analytics', methods=['GET'])
def sales_analytics():
# Get first day of current month
first_of_month = datetime(datetime.now().year, datetime.now().month, 1)
pipeline = [
{"$match": {"order_date": {"$gte": first_of_month}}},
{"$unwind": "$items"},
{"$group": {
"_id": "$items.category",
"total_sales": {"$sum": "$items.price"},
"average_quantity": {"$avg": "$items.quantity"},
"total_orders": {"$sum": 1}
}},
{"$sort": {"total_sales": -1}}
]
results = mongo.db.orders.aggregate(pipeline)
return jsonify(list(results))
Sample data structure:
{
"order_id": "ORD-123",
"order_date": datetime(2024, 3, 15),
"items": [
{"product": "Laptop", "category": "Electronics", "price": 999.99, "quantity": 1},
{"product": "Mouse", "category": "Accessories", "price": 29.99, "quantity": 2}
]
}
The output:
[
{
"_id": "Electronics",
"total_sales": 19999.80,
"average_quantity": 1.2,
"total_orders": 20
},
{
"_id": "Accessories",
"total_sales": 3598.80,
"average_quantity": 2.5,
"total_orders": 15
}
]
This code demonstrates MongoDB's robust aggregation framework working seamlessly with Flask-PyMongo. The /sales-analytics endpoint calculates real-time sales metrics for the current month, including total sales per category, average item quantities, and order counts. Using MongoDB's pipeline stages ($match, $unwind, $group, $sort), it processes nested item arrays in orders and performs calculations entirely on the database server. The results are returned as JSON, showcasing how MongoDB's native aggregation capabilities pair perfectly with Flask's API-building simplicity.
Addressing common concerns and limitations
While Flask and MongoDB excel in flexibility, their performance characteristics differ from alternatives like Django or relational databases. Flask’s lightweight nature makes it faster for small-to-medium applications, but Django’s built-in optimizations often outperform it in large-scale projects. MongoDB’s schema-less design can introduce latency for complex queries without proper indexing, but its native indexing capabilities (B-tree, geospatial, text) and query optimizer enable performance comparable to relational databases when configured correctly.
Conclusion
MongoDB’s document model and Flask’s minimalist philosophy create a symbiotic relationship for modern web development. While alternatives like Django+PostgreSQL excel in rigidly structured environments, this stack shines for:
Rapid prototyping.
JSON-native applications (APIs, real-time systems).
For teams prioritizing developer experience and iterative development, the combination delivers unparalleled agility. MongoDB Atlas provides a seamless cloud-hosted solution, while Flask-PyMongo simplifies integration.
Ready to dive deeper?
Now that you’ve seen how MongoDB and Flask work together, take your skills further with these curated resources:
Official MongoDB Flask Integration Guide: Learn to build RESTful APIs with step-by-step examples and advanced data validation techniques.
[MongoDB Atlas + Flask Deployment Guide](https://www.mongodb.com/developer/products/mongodb/flask-mongodb-flyio/?utm_campaign=devrel&utm_source=third-party-content&utm_medium=cta&utm_content=FlaskDeveloperKaren&utm_term=anaiya.raisinghani: Deploy a location-based app on Fly.io with MongoDB’s geospatial features.
Flask-PyMongo Example Project: Explore a full-stack movie database app with aggregation pipelines and real-world use cases.