When Your Code Works But You Have No Idea Why: A Developer’s Survival Guide
Introduction Every developer, at some point, has written code that miraculously works but defies all logic. Maybe it was a rogue semicolon, a mysterious cache issue, or a divine intervention from the coding gods. Whatever the case, this moment of confusion can be both hilarious and terrifying. So, let's dive into the art of debugging when your code works... but you have no clue why. The "I’ll Fix It Later" Trap We’ve all been there. You add a comment like this: // TODO: Figure out why this works And six months later, you’re staring at the same line, now deeply regretting your life choices. If future-you is currently cursing past-you, congratulations—you've entered the debugging paradox. Debugging Techniques for the Clueless 1. The "Print Debugging" Method Forget fancy debugging tools—just throw in a bunch of console logs like a true warrior: console.log("Why is this working?"); console.log("No, seriously, WHY?"); console.log("Send help."); If the console starts looking like a horror movie script, you know you’re on the right track. 2. The Rubber Duck Method Grab a rubber duck (or any inanimate object) and start explaining your code to it. At some point, you’ll realize your mistake, and the duck will have saved the day—again. "Wait… so if x is undefined, but y is returning true… Oh no." 3. Blaming the Compiler If all else fails, assume it’s the compiler’s fault. The syntax is fine. The logic makes sense. Clearly, the universe is broken. 4. Sacrificing to the Code Gods As a last resort, write a short prayer: "Oh mighty Stack Overflow, guide my keystrokes and bless my commits." The Accidental Genius Moment Sometimes, in a moment of sheer desperation, you comment out random lines and—boom—it works. Do you understand why? Absolutely not. Do you take the win? Every time. // Some random line of code // that I commented out // and now everything works "If it ain’t broke, don’t fix it." — Every developer, ever. Conclusion: Embrace the Chaos Debugging is a rite of passage. If your code works and you have no idea why, cherish the moment. One day, you’ll look back and laugh (or cry). Until then, keep console.logging, trust the rubber duck, and remember—sometimes, the best coders aren’t the ones who understand everything, but the ones who never give up. What’s Your Most Ridiculous Debugging Story? Have you ever fixed a bug by accident? Or discovered that your code was working purely out of luck? Share your funniest debugging moments in the comments below!
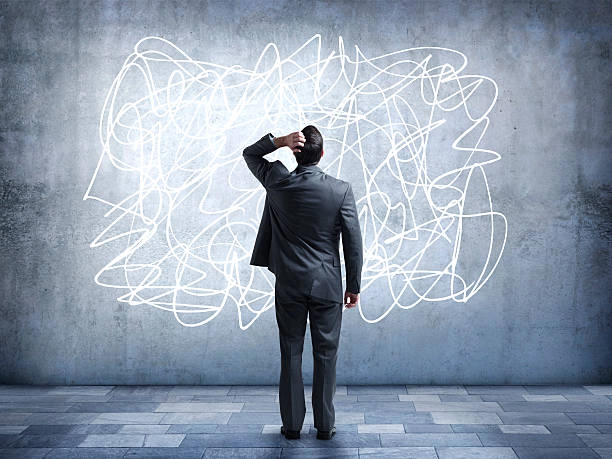
Introduction
Every developer, at some point, has written code that miraculously works but defies all logic. Maybe it was a rogue semicolon, a mysterious cache issue, or a divine intervention from the coding gods. Whatever the case, this moment of confusion can be both hilarious and terrifying. So, let's dive into the art of debugging when your code works... but you have no clue why.
The "I’ll Fix It Later" Trap
We’ve all been there. You add a comment like this:
// TODO: Figure out why this works
And six months later, you’re staring at the same line, now deeply regretting your life choices. If future-you is currently cursing past-you, congratulations—you've entered the debugging paradox.
Debugging Techniques for the Clueless
1. The "Print Debugging" Method
Forget fancy debugging tools—just throw in a bunch of console logs like a true warrior:
console.log("Why is this working?");
console.log("No, seriously, WHY?");
console.log("Send help.");
If the console starts looking like a horror movie script, you know you’re on the right track.
2. The Rubber Duck Method
Grab a rubber duck (or any inanimate object) and start explaining your code to it. At some point, you’ll realize your mistake, and the duck will have saved the day—again.
"Wait… so if x is undefined, but y is returning true… Oh no."
3. Blaming the Compiler
If all else fails, assume it’s the compiler’s fault. The syntax is fine. The logic makes sense. Clearly, the universe is broken.
4. Sacrificing to the Code Gods
As a last resort, write a short prayer:
"Oh mighty Stack Overflow, guide my keystrokes and bless my commits."
The Accidental Genius Moment
Sometimes, in a moment of sheer desperation, you comment out random lines and—boom—it works. Do you understand why? Absolutely not. Do you take the win? Every time.
// Some random line of code
// that I commented out
// and now everything works
"If it ain’t broke, don’t fix it." — Every developer, ever.
Conclusion: Embrace the Chaos
Debugging is a rite of passage. If your code works and you have no idea why, cherish the moment. One day, you’ll look back and laugh (or cry). Until then, keep console.logging, trust the rubber duck, and remember—sometimes, the best coders aren’t the ones who understand everything, but the ones who never give up.
What’s Your Most Ridiculous Debugging Story?
Have you ever fixed a bug by accident? Or discovered that your code was working purely out of luck? Share your funniest debugging moments in the comments below!